7 Key Patterns for Building Video Processing CLI Tools with Commanderjs
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Setting Up Basic Video Processing Commands with Commander Program Class
Building a video processing CLI often starts with laying a solid foundation using Commander.js's program class. Commander simplifies the creation of command-line interfaces by handling command definitions, argument parsing, and help generation, making it user-friendly. When it comes to video processing, tools like OpenCV are crucial for handling video capture and manipulation, enabling tasks ranging from basic playback to complex analysis. The combination of Commander and libraries like OpenCV can empower developers to quickly assemble functional video processing commands, optimizing workflows and streamlining tasks. While these basics provide a strong starting point, Commander's capabilities extend beyond the simple. As your project develops, consider how Commander can facilitate more intricate interactions, allowing you to create a more sophisticated and robust video processing CLI.
Let's delve into the fundamentals of setting up basic video processing commands within the Commander program class. Commander, being a tool for constructing CLIs, offers a neat way to define commands and options, making the development of command-line applications less of a headache.
The `program.parse(process.argv)` function lies at the heart of this setup, acting as the entry point for parsing command-line arguments fed to our application. We then introduce libraries like OpenCV—a robust open-source toolkit mainly written in C, with Python being a prominent language for its usage in image and video manipulation—which allows us to interact with video files, whether from disk, camera feeds, or even image sequences via the `cv2.VideoCapture` function.
Commander also handles the command name in a rather intuitive way: the `Command` constructor accepts the desired command name, and the script name—extracted from the full set of command-line arguments—is used during the parsing process. This seemingly straightforward behavior can simplify things. But the behavior of standalone executable subcommands and the reliance on the entry script's directory for their discovery could introduce subtle complexities when dealing with projects with intricate structures.
On the subject of interacting with hardware, OpenCV offers the `CameraServer` class which includes functionalities like `startAutomaticCapture`. This can be beneficial for integrating and managing USB cameras, particularly in environments where a server infrastructure is in place for managing or viewing captured streams. It is important to consider the limitations and potential complexity when handling camera-related functionalities. While using a library such as OpenCV may offer benefits, it can also add complexity to the process of managing input devices from within our command-line environment. The nature of integrating with external hardware introduces challenges that we should consider, and it’s a constant reminder that while libraries provide incredible capabilities, these are often achieved through complex operations that can be challenging to debug.
We can see the potential of Commander. It's capable of both simple CLI applications and complex ones, but it is crucial that we consider the implications and potential challenges when using tools that interact with other codebases (such as OpenCV) and hardware.
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Building Custom File Format Detection for Video Input Files

When building video processing tools, correctly identifying the format of input video files is essential for smooth and efficient operation. This format detection process, which involves recognizing unique patterns within the file structure, plays a vital role in ensuring that the video data is handled properly throughout the entire processing pipeline. Video processing itself involves a sequence of steps—decoding the compressed video into a raw format, performing computations on individual frames, and finally encoding the processed frames back into a compressed format. Each of these steps can be significantly impacted by the input format, affecting things like processing speed and overall compatibility with other tools and systems.
While leveraging existing libraries and tools can definitely help in building this file format detection mechanism, it's important to be mindful of the complexities that can arise. Integrating these external tools into your own application can introduce dependencies and challenges that need to be accounted for. Carefully considering how your file format detection will interact with these external components is key to building a robust and reliable video processing system. Ultimately, by prioritizing a strong foundation for file format detection, we can avoid common errors and build a more efficient and versatile video processing system.
Detecting the format of a video file can be surprisingly intricate. While file extensions like ".mp4" or ".avi" give us a hint, the reality is that several formats can employ the same underlying codec. For instance, a video using the H.264 codec might be packaged within an MP4 or MKV container. This overlap means relying solely on file extensions for format detection isn't always reliable.
One approach to custom format detection hinges on FourCC codes. These four-character codes, often embedded within the file header, identify the specific video codec being used. This provides a more precise way to determine the type of encoding used, compared to simply looking at the file extension.
Furthermore, video formats typically have unique identifiers, sometimes called "magic numbers," at the start of their binary data. These signatures provide a very quick check, helping us determine the format even before analyzing codec information or metadata. However, the metadata within video files can be quite complex. It includes aspects like frame rate, aspect ratio, and embedded subtitles, but its organization and structure can vary greatly between different file formats. Any custom detection process needs to consider this variability.
In the process of building a custom file format detection system, it is essential to acknowledge the risk of errors. A single corrupted frame or flawed metadata element could break the whole process, so a robust error handling mechanism is paramount. Additionally, dealing with video files, especially high-definition ones, can be computationally demanding. Processing the headers and metadata can lead to significant resource utilization. Efficient algorithms are therefore crucial to prevent bottlenecks in the detection process.
Despite standardization efforts in video compression like MPEG-4 or H.264, variations between implementations can still create headaches. This means a custom detection solution should be able to account for these nuances in how files are encoded. It's also important to consider modern video streaming protocols like RTMP or HLS. These methods, often dynamic and packet-based, do not always fit within traditional file format structures. This adds a further level of complexity to detection.
Building a truly effective format detection mechanism requires cross-platform compatibility. A video file interpreted correctly on one operating system might behave differently on another. Operating system-specific methods of accessing metadata or encoding details create unique challenges.
And finally, the landscape of video formats is always changing. Newer codecs like AV1 are consistently being introduced, which means the detection algorithm needs to be updated regularly to accommodate these changes. The flexibility and adaptability of the detection system will be crucial for keeping pace with this evolving ecosystem.
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Creating Progress Bar Indicators for Long Running Video Tasks
When dealing with video processing tasks that take a noticeable amount of time, providing visual feedback through a progress bar is a great way to keep users informed and engaged. Especially for tasks lasting longer than 10 seconds, a progress bar becomes vital to avoid users feeling like the program is frozen or stalled. These visual indicators, which can range from traditional progress bars to more dynamic representations like spinners or liquid-fill charts, help communicate the stage and estimated completion time of a video operation.
Having a robust way to report progress is crucial for any long-running task; without it, users are left in the dark about what's happening. Tools like cliprogress can make implementing progress bars in command-line applications less of a hassle, letting developers focus on the actual video processing logic. Ultimately, the ability to see progress in real-time can turn what might feel like a long, unpredictable wait into something much more manageable and understandable for the user. While this doesn't speed up the processing itself, it enhances the user experience and helps reduce frustration with the wait times.
Progress bars, when used for tasks exceeding 10 seconds, serve as a valuable tool for keeping users engaged and informed during extended waits. It's a design principle that acknowledges the human need for feedback and understanding in situations involving uncertainty. Having a reliable mechanism to report progress is fundamental for any lengthy process. Without it, providing accurate information to the user about the progress of the task becomes impossible.
Commonly, we see progress bars utilized in situations like application initialization, managing large data imports, preparing files for download, and managing user queues. Similarly, command-line interfaces (CLIs) often use spinners, "X of Y" counters, and traditional progress bars to enhance the user experience in these situations.
The most direct method for updating a progress bar in a long-running task is to carry out the task directly on the primary UI thread, making progress updates within the same thread. This keeps things relatively simple. However, some tasks might be more complicated and would require alternative design patterns. For instance, progress indicators can be extended to represent things like liquid-fill charts that provide more visual ways to signal project goals or overall project status.
We can also consider dynamic progress bars, which are particularly beneficial in short-form video platforms like TikTok or YouTube, where they help viewers grasp the duration of the content. Tools and libraries like `cliprogress` have been developed to handle the display of progress bars within command-line tools, making it easy to incorporate progress feedback and enhancing compatibility across different environments.
Using a progress bar can make the waiting time more manageable for users. When people can see progress, they are often more receptive to delays, viewing them as a less disruptive and more understandable experience. Visual indicators like loading spinners or progress bars are common design choices in web applications. They function to signal that a process is underway, effectively mitigating user uncertainty and frustration during interactions.
Progress bars, in essence, are a way to inject some predictability into what may otherwise feel like an indefinite wait. They can significantly improve user experience, particularly in scenarios involving lengthy processing or operations. But there are a few potential issues to consider. For example, progress indicators can be sensitive to how the processing is structured. If the tasks are handled in background threads (a common practice for computationally intensive operations, especially when libraries like OpenCV are in use), we need to pay close attention to synchronization issues. We must account for the possibility of deadlocks or race conditions if we aren't careful in the design.
Another challenge is the accurate prediction of the time remaining. Many factors, including frame complexity and overall system load, can introduce significant variability. Advanced statistical methods can help us get a better handle on these estimations, further improving the user experience. Additionally, the design of progress bars can influence how they are perceived. It's worth experimenting with various styles through techniques like A/B testing, which can reveal interesting aspects of how different user groups engage with these visual cues.
While progress bars enhance user experience, it's critical to assess their impact on performance, particularly in CLIs where efficient resource management is crucial. The frequent updates necessary to maintain a dynamic bar can introduce overhead that needs to be factored into the design. Furthermore, some tasks, due to the very nature of the data being processed, may not lend themselves to linear progress indicators. These non-linear variations should be communicated to the user, as doing so builds a more informed user experience.
It’s interesting to ponder the design tradeoffs when developing these types of visual cues for the user. The challenges, including concurrency issues and the complexities of generating accurate time estimates, underline the fact that building tools is about balancing between desirable user experience features and practical constraints within an application’s structure.
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Implementing Error Handling for Failed Video Processing Operations
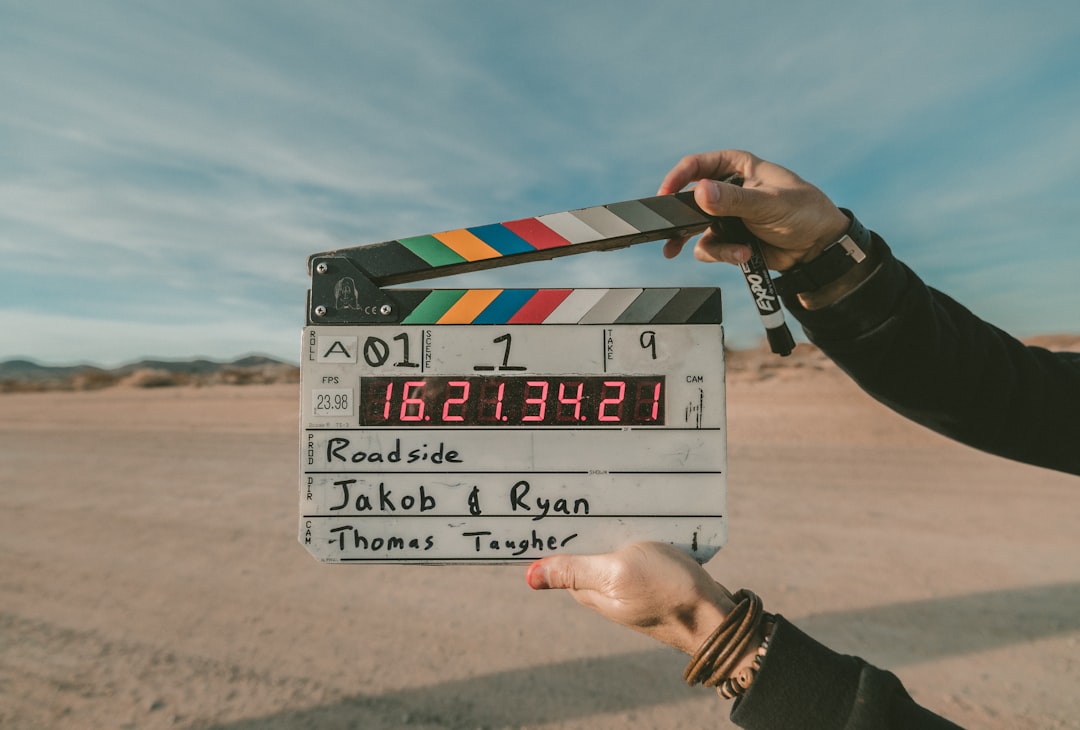
When processing video data within a command-line interface (CLI) tool, it's crucial to implement comprehensive error handling to ensure the tool remains reliable and user-friendly. Video processing can encounter a wide range of errors, including issues with the code syntax, problems that arise during runtime, or even flaws in the logical design of the processing steps. External factors, such as unreliable network connections or database problems, can also contribute to processing failures.
Handling these errors gracefully is essential. One approach is to structure the processing in phases, from initialization to cleanup. By carefully managing resources at each stage, you minimize the risk of problems spiraling out of control and impacting the entire system. Furthermore, tools like Commander.js can be employed to structure your CLI tools. However, it's vital to integrate thoughtful error handling mechanisms within the Commander framework to effectively manage and report any processing failures.
Building resilience into your CLI requires considering patterns like event-driven architectures. These can help the system react to failed processing events, network hiccups, or unavailable services. The ability to handle unexpected situations is paramount. While a Dead Letter Queue (DLQ) can help capture errors, it's crucial to proactively monitor this queue to avoid situations where processing failures go unnoticed.
In essence, a robust error handling strategy is a cornerstone of any reliable video processing CLI. By anticipating potential problems and designing mechanisms to manage them, you'll create tools that are both functional and trustworthy. Without solid error handling, even the most powerful processing tools can become unreliable, leading to frustration for users and potential system instability.
When working with video processing, errors can pop up in various ways, from simple syntax mistakes to more complex issues like network hiccups or database problems. Understanding the different types of errors and how they can impact our video processing operations is crucial for building reliable tools.
For example, a single corrupted frame in a video stream could cause a domino effect, leading to a chain of errors in later parts of the processing pipeline. We must create systems that can isolate errors and prevent this kind of cascade failure.
Furthermore, video processing is quite sensitive to delays. Even a small amount of lag can impact the viewer's experience. So, we must design our error handling to keep the process smooth, without sacrificing quality. And, it's important to remember that video processing is very demanding on computer hardware. We must understand the limitations of our systems, or risk failures and even crashes when things go wrong.
The fact that many modern videos use a variable frame rate adds to the complexity. If we don't correctly manage frame timing, we can end up with audio and video that aren't in sync. This is a prime example of how careful error detection is necessary.
And external factors can cause trouble too. If a video source suddenly disconnects, for instance, our tools should be equipped to recover and ensure processing continues uninterrupted. We can't just ignore the fact that the way we handle errors will depend on the specific video format and codec we are working with. Each format has its own quirks that influence error patterns.
One significant difference between video processing and processing static files is that video is sequential. If an error happens, we're often left with few choices for recovery. We may be forced to restart the entire processing task from the beginning. This really underscores the importance of designing smart error handling practices before we even encounter a problem.
Adding to the challenge, using multiple different frameworks—like Commander.js and OpenCV—can create more potential issues. The interactions between these different environments can be difficult to manage, leading to errors that might not be immediately apparent.
If we don't handle errors properly, it can lead to silent failures, where the output of a video processing task looks unchanged, even though it's been affected by an error. Users might not even notice it. So, it's essential to find ways to clearly communicate errors and provide useful feedback.
Ultimately, one of the best things we can do is learn from the errors we encounter. If we actively analyze these errors and collect data about what happened, we can gain valuable insights that can inform future design decisions. This process of continuous improvement is key to making our video processing applications more reliable.
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Adding FFmpeg Integration Through Command Line Arguments
Extending your video processing CLI with FFmpeg, through command line arguments, unlocks a powerful set of tools for video manipulation. FFmpeg, a command-line tool without a graphical interface, offers a wide range of features for video processing, including file format conversion, adjusting bitrates, and controlling frame rates. Its commands, while effective, can also be intricate. A basic conversion might look like `ffmpeg -i input_file output_file`, with additional options like `-b` for controlling bitrate or `-r` for manipulating the frame rate. While this expands your CLI's capabilities significantly, integrating FFmpeg demands careful consideration. You'll need to address potential error scenarios and think through how FFmpeg commands will work together within your tool. Utilizing principles from frameworks like Commander.js can help ensure your CLI structure remains well-organized, making it easier to manage and build upon the powerful features FFmpeg provides. This approach creates maintainable command-line applications while making it easier to utilize FFmpeg's full potential.
FFmpeg, a command-line tool with no graphical interface, offers extensive video processing capabilities. Its command-line approach involves using a terminal or command prompt to interact with it, enabling manipulation of video and audio files across a vast array of formats. The core FFmpeg command structure is simple: `ffmpeg -i input_file output_file`, which can be used for basic media file conversions.
FFmpeg provides many customizable options, like the `-b` option for controlling bitrate and impacting output quality. Users can manipulate video frame rates with commands like `ffmpeg -i input.mp4 -r 24 output.mp4`, forcing the output to a specific frame rate. Even concatenating multiple video files is possible by using a text file listing the inputs and then using that file with FFmpeg.
Manipulating video speed is also within FFmpeg's abilities, achieved through filters like `setpts`. For instance, `ffmpeg -i input.mp4 -filter:v 'setpts=0.5*PTS' output.mp4` would double the video's speed. Although FFmpeg offers a wide range of options and filters, integrating it into more advanced video editing pipelines might require external libraries like Editly, which provide higher-level tools.
Understanding how FFmpeg functions is facilitated by its documentation and built-in help commands. `ffmpeg -h` and `man ffmpeg` provide access to FFmpeg's features and options.
Integrating FFmpeg into a CLI tool presents particular challenges. There's a need for robust error handling, especially considering that FFmpeg error messages can be quite detailed and complex. This is where tools like Commander.js are quite valuable. Commander.js makes it much easier to create structured CLIs that can interact with FFmpeg, avoiding the complications that come with dealing with the inherent complexities of subprocesses and interpreting command-line outputs correctly.
Furthermore, the sheer variety of codecs and options FFmpeg provides might lead to difficulties if the application isn't well-designed. Carefully considering how options are presented to the user via the CLI and managing the different FFmpeg subcommands, flags, and settings is a real challenge. Additionally, because video processing is inherently I/O intensive, concurrent FFmpeg processes can lead to bottlenecks or other issues that developers need to be mindful of.
While the potential for using FFmpeg to do real-time processing exists, achieving that can be difficult. The ability to seamlessly integrate into scripting environments, like Bash or PowerShell, is an advantage that developers should consider when developing FFmpeg-based applications.
One thing that's worth noting is that encoding settings directly impact file size and processing speeds. This suggests a trade-off between video quality and the speed and resource demands of the process. Open-source FFmpeg itself may require alterations and custom configurations depending on the specific use case or performance requirements of an application.
Ultimately, utilizing best practices and tools, including Commander.js, helps mitigate some of these complexities, leading to better-structured and maintainable applications when dealing with video processing through the command line. It's clear that FFmpeg is a powerful tool with the potential for substantial impact, but it can be quite challenging to integrate effectively into applications due to the complexities of both its interface and the inherent characteristics of video processing.
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Managing Concurrent Video Processing with Queue Systems
When building video processing tools, handling multiple tasks simultaneously (concurrency) is essential for speed and efficiency. Queue systems provide a robust way to manage this complexity. These systems act as a buffer, accepting processing requests, storing them, and then delivering them to available processing resources. This helps prevent bottlenecks and improves the overall responsiveness of the tools, especially when dealing with large or complex video files.
Using frameworks like NestJS can help make video processing applications more scalable, allowing them to handle a growing workload without significant performance degradation. Integrating with queue systems like RabbitMQ or cloud services like AWS Lambda further supports this scalability. These frameworks and services enable a more modular architecture, where video processing tasks are broken down into smaller, independent units that can run concurrently.
However, concurrent processing introduces its own set of challenges. Maintaining the correct order of processed frames can be difficult when operations happen out of sequence. Ensuring seamless communication and synchronization between the different components of a system, particularly when tasks are distributed across multiple threads or services, becomes critical.
Properly designing a queue-based system helps to address these concurrency challenges. By prioritizing the order of tasks, ensuring reliable communication pathways, and managing potential errors, you can avoid issues like out-of-sequence frame processing or lost data. Through careful consideration of these complexities, you can create video processing applications that are both fast and reliable, leading to more efficient workflows.
When dealing with video processing, which often involves computationally heavy tasks like decoding and encoding, we can often see substantial performance gains by using concurrent processing techniques. Queue systems are great for managing this concurrent nature of the work. We can push jobs into the queue, pull them out for processing, or handle failed, deleted, or cleared jobs. This approach can often cut processing time in half when compared to doing things sequentially. However, the more we utilize these queues, the more complicated things can get. We might end up with different jobs needing to be done at different priorities. This requires using priority queues or a similar strategy so we don't get bogged down in dealing with lots of smaller jobs before dealing with critical tasks. Furthermore, the nature of concurrent processing itself also introduces the challenge of managing memory usage across different tasks and processes. Each concurrent task that is launched often requires resources, and it's important to design your application in a way that doesn't lead to excessive resource consumption or unexpected crashes related to memory issues. There are a number of techniques such as load shedding that can help here.
Another challenge we encounter when working with concurrent processing is the possibility of running into race conditions. If multiple threads or processes are simultaneously accessing the same parts of the program or data, it can quickly become difficult to get accurate results. This is why we need to be mindful of thread safety and employ strategies like using thread-safe queues to minimize these types of issues. On the subject of queues, we also need to consider the latency that is inherent in the way queues are used to orchestrate tasks. While queues are helpful in ensuring that tasks don't interfere with each other, this also means we introduce a waiting period. We must try to minimize these delays so that our application can maintain a high rate of processing, while not sacrificing the reliability or correctness of the processing steps.
It's important to consider the impact of errors when implementing concurrent processing. When an error occurs in one process, we don't want that error to cascade into other unrelated tasks. Robust error handling needs to be in place so that we can gracefully handle failure. For instance, when a job fails, it's crucial that the rest of the queue can continue its operations. This is part of managing the tradeoff between system efficiency and robustness. It's a good idea to consider methods for retriggering failed tasks as part of this error handling mechanism.
Distributing processing tasks across different machines or processing cores can also help improve throughput. Static allocation might not be the most efficient solution, so dynamic load balancing is often beneficial for handling tasks of variable size or duration. When developing applications that rely on concurrency for video processing, we also need to consider how the application can scale. Scaling the application might mean distributing tasks across more servers or processors. It is important to account for the potential for queues to overflow if not carefully designed. We'd want to avoid a scenario where the queue grows unbounded and ultimately results in a failure condition.
Providing feedback to the users can be beneficial in helping them understand how the application is doing. This could be implemented through something like callbacks or a user interface element such as a dashboard that displays the status of tasks. Finally, the sheer volume of data often associated with video files requires us to carefully consider the implications of bandwidth consumption. If we don't manage bandwidth carefully, particularly when deploying to a distributed system, it could result in undesirable behavior. Adaptive strategies, where bandwidth allocation changes dynamically depending on the network conditions, can be useful to avoid these scenarios.
In closing, when developing video processing CLIs, it's important to fully consider the implications of using concurrent processing, queues, and related patterns. There is a balance between efficiency and reliability that needs to be considered during the design phase. It's likely that these issues will require you to make a number of design choices, and a lot of experimentation before you are completely satisfied with the outcome.
7 Key Patterns for Building Video Processing CLI Tools with Commanderjs - Writing Video Metadata Output to Standard JSON Format
When working with video, consistently managing and sharing information across different systems is important. Using a standard format, like JSON, for storing video metadata helps with this. Standardized metadata schemas, such as the IPTC Video Metadata Hub, play a crucial role in making sure tools and systems can understand each other when working with video files. As video files become more complex, using a standard format becomes even more necessary for interoperability. JSON is a good choice for storing metadata because it's easy to read and work with. This makes tasks like editing and validating metadata simpler. Tools like FFmpeg can be integrated to automate extracting the metadata, simplifying the overall process. But developers need to be careful and handle errors properly when writing the metadata to a JSON file. Taking these steps when designing a command line tool with Commander.js promotes better data management and aligns with best practices in software development for video processing.
When it comes to video processing, storing metadata in a structured, easily accessible format is key. JSON, with its hierarchical structure, offers a compelling alternative to older formats like XML, which can become quite verbose and less efficient. Using JSON allows for a more streamlined representation of video properties, such as codecs, resolutions, and bitrates, by nesting related attributes under a single parent object.
One of the primary advantages of JSON is its promotion of interoperability. By standardizing on JSON for video metadata, we pave the way for seamless exchange of information between different processing tools and libraries. This can greatly simplify the workflow, as video processed using one tool can be easily analyzed or modified by another without requiring complex parsing routines.
The structured nature of JSON also helps us catch errors early on. Because of JSON's rigid syntax, any malformed data can be quickly flagged during the parsing process. This is vital for preventing downstream issues that could result from missing or faulty metadata, ensuring the integrity of video processing operations.
Furthermore, the adoption of JSON in modern APIs makes it a natural fit for integrating our CLI tools with cloud services. Many platforms that deal with video processing rely on JSON for data exchange, so adhering to the JSON standard simplifies integrating our CLI tools into these environments.
Because it's easily readable by humans, JSON also makes debugging much easier. Developers can quickly inspect the output of video metadata and grasp the configuration details without requiring specialized tools. This can be a real time-saver when trying to identify and fix issues in our processing pipelines.
Beyond readability, JSON's serialization capabilities are a significant advantage. Efficient serialization and deserialization of data during processing are crucial for managing large video datasets, which are becoming increasingly common. JSON's efficiency in this area can optimize data handling and reduce the I/O overhead involved in video operations.
Another benefit of using JSON is its flexibility. The ability to easily define custom video metadata attributes is crucial in the constantly evolving world of video technology. This extensibility allows us to adapt our tools to new formats, codecs, or features without breaking existing data structures.
Moreover, a robust ecosystem of libraries and frameworks exists for parsing and manipulating JSON data across a wide range of programming languages. This extensive support minimizes the implementation hurdles, making JSON a practical choice for CLI tool developers.
JSON also facilitates real-time metadata updates, a valuable feature for live video processing applications. By employing technologies like WebSockets, changes to video metadata can be dynamically reflected in JSON format, allowing clients to receive instant updates without needing a refresh.
While JSON is a text format, compression techniques are available to minimize its size. This can be critical in video processing scenarios where metadata can grow quite large, reducing the impact on storage and bandwidth usage.
Overall, adopting JSON for video metadata output offers a multitude of advantages in terms of structure, interoperability, error handling, API integration, and tooling support. As video processing becomes increasingly complex and interwoven with cloud and web-based services, utilizing JSON's strengths in this space will continue to be valuable for ensuring efficient and robust applications. However, while the benefits are clear, researchers and engineers should remain aware that any reliance on specific technologies or formats may introduce risks of future incompatibility with evolving standards or practices.
More Posts from whatsinmy.video: