C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms
C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms - Understanding Range Checking in C++ for Video Processing

Within the realm of C++ video processing, understanding and employing range checking is vital for safeguarding data integrity. This practice involves setting boundaries for input values, preventing them from exceeding predefined limits. This step is crucial to avoid the pitfalls of integer overflow, a common issue in C++ where operations surpass the capacity of the data type, potentially yielding inaccurate or unexpected outcomes.
By rigorously enforcing these limits, range checking strengthens the reliability of video processing applications. It acts as a filter, preventing invalid data from entering the processing pipeline and reducing the risk of crashes or unpredictable behavior. This becomes particularly important when dealing with extensive and intricate video datasets, highlighting the necessity for automated validation methods. These automated processes not only enhance efficiency but also ensure accuracy throughout the development process.
The onus falls on developers to establish and uphold these data boundaries within their video processing algorithms. This deliberate approach ensures that applications are built upon a foundation of robust and reliable performance, guaranteeing the integrity and trustworthiness of the resulting video processing outputs.
Data validation through range checking plays a key role in the efficiency of video processing algorithms in C++. By ensuring that data stays within predefined limits, it avoids accessing memory beyond allocated boundaries, mitigating a common source of performance slowdowns caused by memory-related errors.
While C++ offers the performance needed for systems programming, its lack of native range checking mechanisms introduces a vulnerability to buffer overflows. This is particularly concerning for real-time video processing, which demands both speed and reliability.
The benefits of range checking extend beyond preventing crashes; it's also vital for safeguarding image quality. By restricting pixel values to appropriate ranges, potential visual artifacts that degrade the video can be avoided.
C++'s ability to use templates and inline functions can be harnessed for implementing effective range checking. These techniques provide compile-time checks, thereby minimizing the runtime performance overhead that traditional runtime checks can impose.
Despite C++'s appeal for video processing due to its hardware proximity, the responsibility for implementing range checks manually can introduce a significant debugging challenge. Errors often surface only under specific input conditions, making it harder to track their origins.
Utilizing assertions or exceptions for range checking offers more than just debugging assistance. These mechanisms also act as valuable documentation, making the maintenance of intricate video processing algorithms more manageable.
However, careful consideration is necessary when applying range checks as excessive or inefficient checks can create latency in processing pipelines. Performing checks only when absolutely necessary and optimising those checks for speed is critical to prevent frame rate drops.
Modern C++ standards like C++20 introduce features that could streamline range checking through concepts and enhanced type safety. These advancements can offer benefits in the development of video processing algorithms.
Many cross-platform video processing libraries leverage C++ and embed range checking within their APIs. This standardization of data integrity helps developers by reducing their workload and ensuring consistency across projects.
Lastly, it's worth exploring the relationship between range checking and SIMD techniques within the context of C++ for video processing. Ensuring data ranges are properly handled can optimize parallel processing performance, leading to notable gains in efficiency.
C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms - Implementing Boundary Checks in Video Frame Analysis
Within the context of video frame analysis, implementing boundary checks is a critical step in ensuring the integrity of the processed data. These checks serve as safeguards against invalid input values that can potentially disrupt the video processing pipeline and degrade output quality. It's vital to prevent situations where data overflows or falls outside acceptable ranges, especially when working with extensive video datasets or in real-time applications. Such violations can manifest as visual distortions in the processed video or even cause unexpected system crashes.
However, implementing boundary checks must be done judiciously, with careful attention to their potential impact on performance. Excessively frequent or poorly optimized boundary checks can introduce latency into the video processing pipeline, impacting frame rates and overall smoothness of operation. Striking a balance between rigorous data validation and efficient execution is essential for maintaining a smooth and reliable video processing experience.
As the C++ language evolves, newer features and approaches for range checking and data validation can be integrated into video processing algorithms to enhance their robustness. This continuous evolution presents opportunities for developers to refine their boundary check implementation, further enhancing both data integrity and performance within their video analysis applications.
Within video frame analysis, implementing boundary checks isn't solely about preventing crashes; it's fundamental to preserving the integrity of the visual output. When pixel values stray beyond defined boundaries, it can trigger artifacts that drastically diminish the image quality, potentially jeopardizing the entire processing pipeline. This isn't just a theoretical issue, but a real-world concern.
Integer overflow, often disregarded as a theoretical issue, can create vulnerabilities in video processing. A seemingly minor overflow might lead to unexpected behavior in a video player, potentially creating exploitable security risks. Thus, robust boundary checks become crucial.
However, the approach to range checking isn't without its challenges. Manual boundary checks, if not handled delicately, can introduce performance penalties, especially in real-time video processing where every millisecond matters. The overhead from excessive checks can easily outweigh their benefits, leading to slower processing times.
The intricate connection between boundary checks and SIMD instructions underscores an optimization trade-off. While SIMD streamlines parallel processing of data, insufficient range checks can disrupt the parallel execution, hindering the potential performance gains that SIMD offers. So, finding the balance between robust checks and maintaining speed is essential.
Utilizing C++ assertions or exceptions for boundary checks is beneficial beyond simply debugging. These mechanisms also serve as a form of self-documentation, enabling easier maintenance of intricate video processing code, a common challenge in this field.
Modern C++ features like C++20's concepts provide a new angle for implementing range checks. By enforcing data constraints during compilation, these features lessen the burden of runtime checks, contributing to the overall robustness of video processing algorithms.
However, the application of boundary checks isn't universally applicable across all video data types. For instance, dealing with compression parameters necessitates distinct strategies from checking pixel color ranges. It's crucial to tailor the approach based on the specific data context.
This need for context is further magnified in multi-threaded video processing. Depending on the approach to implementing checks, they can introduce points of contention that restrict parallel execution, ultimately impeding overall processing speed.
While several cross-platform libraries integrate automatic range checking, the inconsistencies in implementation can cause complications across different projects. This can pose a challenge for developers trying to enforce a uniform approach to data integrity throughout their projects.
Lastly, it's important to acknowledge that even experienced developers can overlook specific edge cases in boundary checks. This human element can lead to bugs that might only appear under certain circumstances, making debugging in video frame analysis particularly complex.
C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms - Optimizing Memory Access with Safe Array Indexing

Efficient memory access is crucial for the performance of video processing algorithms in C++. Safe array indexing plays a key role in achieving this efficiency by preventing memory access errors. When accessing array elements, developers should implement checks that ensure indices remain within the valid bounds of the array. Failing to do so can lead to crashes or unexpected program behavior.
Using data structures like SAFEARRAY can provide a helpful mechanism for enforcing these boundaries. These structures often include metadata describing the array's dimensions and limits, which can be used during operations to verify that accesses are valid. However, it is essential to understand that the benefits of robust error checking need to be weighed against potential performance drawbacks. Excessive checks can introduce overhead that can be detrimental to real-time video processing.
Therefore, developers should carefully consider where and when to implement these checks. By striking the right balance between safety and speed, we can optimize memory access while maintaining the integrity of video processing systems. This balance is critical for ensuring that these systems are reliable and efficient enough to meet the demands of modern video applications.
Optimizing memory access is paramount in video processing, especially given that memory operations often dominate execution time. Using safe array indexing can potentially improve performance significantly by leveraging the way modern CPUs manage memory. When data is accessed sequentially within an array, the CPU's cache can operate more efficiently. This is because the cache is designed to store nearby data, leading to fewer cache misses and lower latency. However, we need to consider the overhead that bounds checks introduce, which, if not implemented carefully, can lead to performance penalties and, ultimately, reduce efficiency, especially in real-time applications.
On the other hand, if done well, safe indexing gives the compiler more information about the program's behavior. The compiler can then make more informed choices regarding optimizations that potentially eliminate unnecessary checks, further enhancing performance. But caution is needed when working with SIMD (Single Instruction, Multiple Data) parallelism. Improperly implemented array indexing can lead to divergent execution paths across threads, thus potentially undermining any performance gains intended by SIMD.
In the context of real-time applications, such as those involved in video processing, even slight delays caused by boundary checks can lead to noticeable frame drops and affect the user experience negatively. Given that unsafe array indexing is a major cause of bugs and vulnerabilities in C++, it's crucial to be mindful of memory safety. Security audits frequently reveal that a large percentage of issues stem from memory access errors. Furthermore, when we introduce multi-threading, implementing safe array indexing could potentially create bottlenecks and reduce the performance benefits of parallelism.
Debugging in video processing can be tricky because boundary conditions can sometimes lead to hidden problems. The output might appear normal until the algorithm is exposed to certain edge cases, which can make these bugs extremely challenging to diagnose and fix. This underlines the importance of ensuring safe and robust implementation of array indexing from the very start.
Interestingly, adopting a hybrid approach that combines both compile-time and runtime checking offers a potential avenue for achieving a good balance. This hybrid approach aims to mitigate the performance costs of runtime checks while retaining the safety guarantees they provide. This can be a very valuable method for achieving both performance and safety in video processing applications.
In conclusion, the optimization potential of safe array indexing is compelling, but it requires careful consideration of various factors, including caching behavior, compiler optimizations, the impact on SIMD and multi-threading, and the complexity of debugging. Through thoughtful implementation and the use of hybrid techniques, we can strive to ensure that safe array indexing enhances performance without sacrificing the integrity of our video processing algorithms.
C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms - Handling Overflow and Underflow in Pixel Value Calculations
When processing video data, ensuring the accuracy of pixel value calculations is paramount. One key challenge is handling potential overflow and underflow situations. Overflow occurs when the result of a calculation exceeds the maximum value a data type can represent, leading to incorrect pixel values. This is particularly problematic with integer types, where exceeding the limit can lead to unexpected wraparound behavior. Underflow, more common with floating-point numbers, involves results approaching zero to the point where they become smaller than the smallest representable value, often resulting in a loss of precision.
To maintain data integrity, developers should proactively include checks before performing pixel calculations. These checks should verify that the inputs and anticipated outputs fall within the valid range of the chosen data type. Implementing these checks helps ensure that calculations produce accurate results, preventing visual artifacts and system errors in video processing algorithms. While preventing these issues is crucial, it's important to also consider that adding too many checks can impact performance, so a balance is essential.
When calculating pixel values, exceeding the representable range for a data type can lead to noticeable visual problems. For example, integer overflow can cause unexpected color distortions, like pixels suddenly changing to completely different hues or rendering parts of the image unidentifiable. These distortions are a direct consequence of values "wrapping around" outside the intended range.
Similarly, underflow can create more than just transparency issues. It can force pixel values towards zero, potentially leading to a substantial loss of detail, especially in darker areas of an image, resulting in reduced image fidelity.
Commonly, 8-bit pixel channels have a range of 0 to 255. If computations exceed these limits, they can produce colors that cannot be displayed on a screen. Consequently, implementing range checks becomes crucial for accurate color representation and a positive user experience.
Managing overflow and underflow requires a thorough understanding of how data types are represented at a low level. Overflow behavior, for instance, differs significantly between signed and unsigned integers, which necessitates specific handling of pixel values based on the chosen data type.
There's a noticeable performance trade-off associated with range checking. While skipping checks leads to faster computations, it introduces the risk of runtime errors. Determining where and when to check for potential overflows and underflows is a key consideration to ensure both speed and safety.
Modern hardware acceleration through techniques like SIMD presents unique challenges. If a single range check fails within a SIMD operation, the error can potentially cascade across multiple threads, potentially causing a complete breakdown of the processing pipeline. This highlights the need for very careful handling of range checking in this context.
Optimization techniques, such as lookup tables, which are frequently used for pixel processing to avoid repeated calculations, can be adapted to incorporate range checking mechanisms, thus improving both the speed and safety of pixel transformations.
Pixel value compression, frequently used in lossy video formats, can worsen underflow problems by modifying original values beyond their typical ranges. This increases the importance of strict validation during video processing to maintain the integrity of the data.
Pixel encoding formats, such as RGB or YUV, interact with overflow and underflow in unique ways. Therefore, dedicated boundary checks are needed for each format, as standard checks might not accommodate the specific ways color information is represented within each.
Utilizing assertions in C++ for debugging builds is helpful in identifying overflow and underflow issues early on. However, relying solely on assertions in release builds can limit the scope for performance optimization if robust runtime checks are not carefully implemented. This again underscores the tension between performance and reliability, necessitating careful engineering choices.
C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms - Ensuring Data Integrity During Video Codec Operations
Maintaining the integrity of video data during codec operations is crucial for ensuring high-quality and reliable video processing. This means carefully overseeing both encoding and decoding processes to prevent errors and maintain the intended video quality. Methods like identifying anomalies through statistical analysis or detecting disruptions in the video stream can aid in detecting potential tampering or failures in compressed video. It's also necessary to include continuous checks and validation steps throughout the codec process to guarantee overall data integrity. These measures not only improve video quality but also contribute to the overall stability and dependability of the video codec systems being used. However, there's a potential tradeoff between these safeguards and performance, as excessive checks could impact speed and efficiency. Finding a good balance here is key.
Maintaining data integrity during video codec operations is critical to preserving video quality and preventing unexpected behavior. One crucial aspect is the interplay between integer and floating-point calculations when manipulating pixel values. If an integer operation overflows, resulting in a negative value, subsequent floating-point calculations can generate erroneous results. This underscores the complexity of ensuring data integrity across different data types.
Overflow errors aren't just theoretical; they cause noticeable visual artifacts. Besides distorting colors, overflow can lead to color banding, where smooth gradients are replaced with abrupt transitions. This typically occurs when pixel values exceed the displayable range and "wrap around," leading to jarring visual effects in the output video.
Underflow, more common with floating-point data, can significantly impact the details in video. It can cause loss of precision, particularly in darker regions of an image, making them appear flat or detail-less. This is especially problematic when pixel values fall below zero in shadows, resulting in a noticeable drop in image fidelity.
SIMD (Single Instruction Multiple Data) instructions, while boosting performance, introduce new challenges to range checking. A failed range check in one lane can potentially affect all lanes in the SIMD operation, potentially causing widespread artifacts or a complete performance degradation. This compels developers to design rigorous pre-checks for overflow and underflow in SIMD environments.
The choice of using signed or unsigned integers heavily influences how overflow is handled. While unsigned integers prevent negative results, they can lead to unforeseen wrapping behaviors if not properly constrained, resulting in incorrect data. Historically, the trade-offs between signed and unsigned integers have guided implementation choices, and a thorough understanding of this is key to maintain data integrity.
Lookup tables, a common optimization technique for pixel processing, can be adapted to incorporate range checking as well. Lookup tables avoid redundant calculations, accelerating processing, and simultaneously guarantee pixel values stay within safe ranges. This approach provides an effective method for improving both speed and safety.
Some developers employ conditional compilation for range checks. By using preprocessor directives, they can compile range checks for debugging builds but exclude them from optimized production builds. This allows for thorough testing without sacrificing performance in the final version. However, it requires meticulous planning to ensure that edge cases aren't overlooked.
Each pixel encoding format, whether it's RGB or YUV, has unique operational characteristics. As a result, individual range checks need to be carefully designed for each. Failing to do so can result in notable color reproduction errors across different formats.
Video compression techniques, particularly lossy formats, introduce a new dimension to range checking challenges. Compression methods often modify pixel values beyond typical limits, potentially exacerbating underflow issues. This emphasizes the need to maintain strict data integrity during both encoding and decoding processes to safeguard data quality.
Balancing range checks and runtime performance remains a central challenge in video processing. Although enforcing checks increases reliability, neglecting optimization can introduce severe latency in real-time applications, negatively impacting the user experience in critical areas such as live broadcasting and interactive gaming. Developers must walk a fine line to guarantee both a quality viewing experience and reliable processing.
C++ Range Checking Ensuring Data Integrity in Video Processing Algorithms - Best Practices for Exception Handling in C++ Video Algorithms
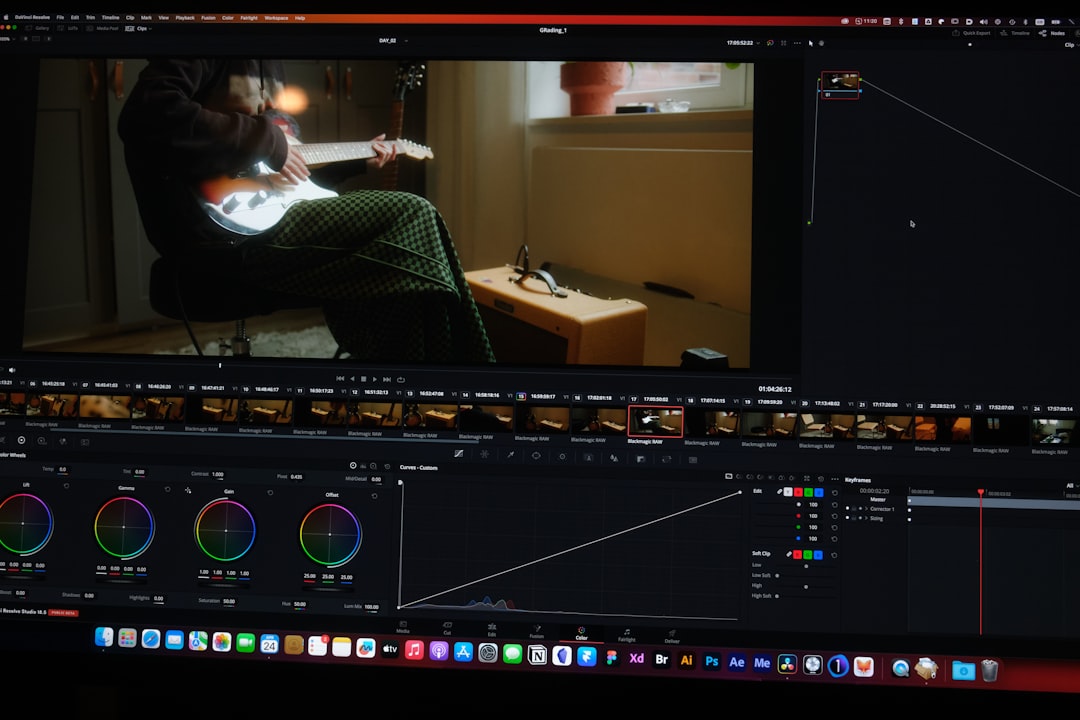
Within C++ video algorithms, managing unexpected events and errors during runtime is essential for maintaining data integrity. This is achieved primarily through exception handling, using `try-catch` blocks to intercept and process errors. While C++ doesn't have the automatic error handling some languages offer, developers can leverage techniques like returning error codes or employing the `errno` variable to manage errors effectively. It's crucial for video algorithms, particularly given their sensitivity to data issues, to implement error checks at various points in the data processing pipeline, ideally at both the input and output stages. By separating the core logic of the algorithm from the exception handling code, developers can create algorithms that are easier to understand, modify, and maintain, promoting the development of a more reliable and stable video processing environment. This approach also contributes to preventing errors from cascading through the program, leading to more predictable and resilient video algorithms. However, this separation needs to be done carefully, ensuring that the exception handling doesn't itself introduce unexpected delays.
1. **Balancing Act**: In C++ video algorithms, achieving the desired processing speed often clashes with the need for careful range checking. Even slight performance boosts can be crucial, but overlooking validation can lead to major issues with image quality, creating a tricky balance.
2. **Hidden Bugs**: Research suggests a lot of bugs in video algorithms stem from unnoticed violations of data boundaries. Developers frequently miss edge cases, leading to problems that only appear under specific conditions, emphasizing the need for comprehensive testing.
3. **SIMD's Double-Edged Sword**: Employing SIMD (Single Instruction, Multiple Data) in video processing can greatly increase speed. However, a single range check failure in a SIMD operation can spread errors across multiple data lanes, significantly hindering the efficiency gains achieved through parallelization.
4. **Assertions: A Tool, Not a Solution**: Assertions are helpful for developers to pinpoint range-related problems during debugging. However, relying solely on them in production can lead to significant performance bottlenecks. Striking a balance between safety and efficiency is necessary.
5. **Compiler's Advantage**: Using safe array indexing not only prevents memory errors but also provides vital information to the compiler. This allows for optimization by eliminating unnecessary bounds checks in cases where the program logic already ensures safety.
6. **Signed vs. Unsigned Quandary**: The choice between signed and unsigned integers when manipulating pixel data is not straightforward. While signed integers offer a wider range including negative values, they can lead to wraparound problems, requiring tailored solutions to avoid artifacts caused by overflow.
7. **Data-Specific Boundaries**: Effective boundary checks can't be applied universally; they must be tailored to the data they protect. Strategies for validating pixel color ranges differ significantly from those needed for compression parameters, highlighting the need for customized checks.
8. **Lookup Tables: A Safety Net**: Adapting lookup tables to include range checking can deliver both speed and safety benefits. This combined approach mitigates the performance cost of repeated calculations while keeping pixel transformations within valid limits.
9. **Flexibility in Checks**: The hybrid approach of mixing compile-time and runtime checks offers a balanced solution to performance concerns. It allows developers to implement effective safety validation while satisfying real-time processing requirements.
10. **Format-Specific Validation**: Pixel encoding formats such as RGB and YUV need specific range checks due to their unique characteristics. Generic range checks might lead to substantial color reproduction errors if not tailored to each format's particular handling of color data.
More Posts from whatsinmy.video: