Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python - Understanding the 'nothing to repeat' error in Python regex
When working with Python regular expressions, the "nothing to repeat" error can be a frustrating hurdle. This error crops up when you try to use a quantifier like `*`, `+`, or `?` without a character or group in front of it that it can actually repeat. It often appears at the beginning of your regular expression (position 0), highlighting the fact that the quantifier is essentially lost without a preceding element.
One common source of this problem is the improper use of escape sequences, especially when backslashes are unintentionally placed in a regex without a preceding character. The heart of the issue is that regex quantifiers demand something to quantify – a character, a group, or a pattern. Patterns like `*a` or `?b` are flawed because `*` and `?` need a valid element to precede them.
Luckily, Python's `re` module gives you tools to compile regular expression patterns and detect these errors during compilation instead of runtime, smoothing out the debugging process. While Python and Perl regex share similarities, being mindful of Perl's behavior can help clarify and fix Python-related issues. Taking the time to ensure every quantifier has a preceding character or group within your regular expressions is crucial for preventing this type of error in the future.
1. Python's regex engine throws the "nothing to repeat" error when it encounters quantifiers like `*`, `+`, or `?` without a valid preceding element. It's a fundamental constraint of how these quantifiers are designed to work—they need something to repeatedly match.
2. The error's frequent appearance at the beginning of the regex suggests the engine stumbles upon a quantifier right away, before encountering any character or group it's meant to quantify. This highlights a structural issue within the pattern.
3. While the error itself may not be directly related to finite state machines, its occurrence does point towards an issue in how the pattern translates into the internal representation used by the engine. This translation process may be viewed as a transition from a human-readable form to a computational one, where quantifiers require clear definition for successful transitions.
4. A pitfall leading to this error involves using backslashes to escape characters that don't need escaping. This results in an unexpected and likely invalid construct in the pattern, creating confusion for the engine. It's like adding extra steps in a routine that weren't necessary.
5. Understanding the "nothing to repeat" error is more than just fixing a syntax mistake; it encourages a deeper understanding of the underlying structure and logic within our regex expressions. It provides insights into where issues originate in the design phase.
6. A common misconception is to think that the error's position always pinpoints the mistake directly. While it usually provides a general area, it's often the first point where the engine detects an invalid state, not where the error was originally introduced in the pattern.
7. This error becomes more likely to occur in complex patterns where many quantifiers, character classes, and other constructs are combined. The more complex the regex, the more opportunities for subtle errors in the pattern's sequencing.
8. In an attempt to circumvent the error, some might compromise by disabling specific regex checks or using overly simplistic patterns. However, this might mask deeper issues and could lead to potential bugs or unpredictable behaviour, especially when regex plays a central role in an application.
9. Different regex engines may have distinct ways of handling this type of error, but Python's approach emphasizes pattern correctness, which promotes a more predictable behavior for users. This enforced structure, in essence, enhances our reliance on the engine as a predictable component in the software development process.
10. Confronting this specific regex error fosters a greater appreciation for careful pattern design and testing. These practices encourage us to create cleaner regexes, which in turn contribute to a more maintainable codebase. We are forced to consider the intended logic of the pattern and validate its expression.
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python - Common causes of the position 0 regex error
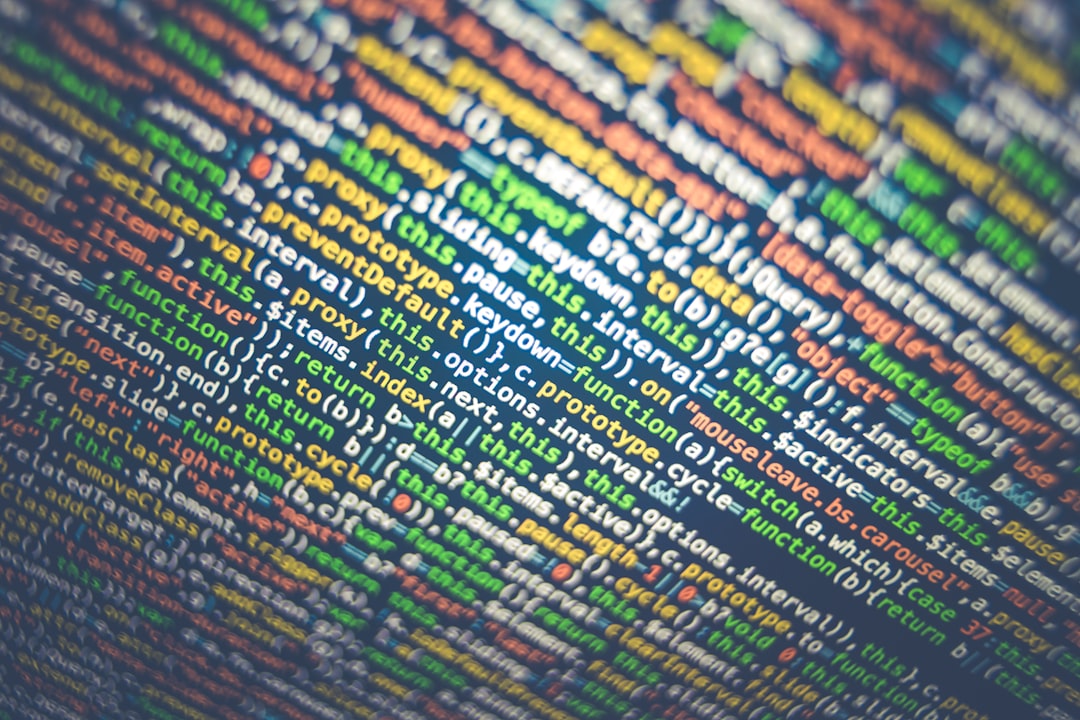
The "nothing to repeat" error at position 0, commonly encountered when working with Python's regular expression module, typically stems from misusing quantifiers like `*`, `+`, or `?`. These quantifiers need a preceding character or group to specify what they're supposed to repeat. The error arises when they're inappropriately placed at the start of the regex pattern, effectively attempting to repeat nothing. For instance, patterns like `*abc` or `+def` demonstrate this error—the quantifiers are used without a preceding element to repeat. Moreover, employing empty character classes or groups can introduce ambiguity, adding another layer of complexity that the regex engine struggles to interpret. The core solution to avoiding this issue is to carefully examine regex patterns, ensuring each quantifier has a clear, valid element it's meant to repeat. By carefully structuring and reviewing regex patterns, you can prevent these common errors from arising.
1. The "nothing to repeat" error acts as a safety net within regex engines, ensuring patterns are structurally sound before they're interpreted. This helps avoid misunderstandings that could stem from improperly formed syntax.
2. Each regex quantifier has a specific purpose – in Python's world, these roles are well-defined, making them ineffective without a valid expression in front of them. As a result, getting a handle on what each quantifier is supposed to do is crucial for building correct patterns.
3. Many patterns that cause this error often come from a misunderstanding of how regex quantification works, particularly with optional aspects. For instance, writing `??` doesn't make logical sense because there's nothing to designate as optional at that spot.
4. The common occurrence of these types of syntax errors, like misplaced quantifiers, highlights why regex patterns truly benefit from rigorous testing. A surprising number of developers skip this step, wrongly assuming a quick visual check is enough to validate things.
5. Interestingly, this error can serve as a teaching moment, emphasizing the value of regex learning and iterative refinement. Developers often find themselves re-examining their understanding of regex's more intricate aspects when they hit this frequent issue.
6. Some regex structures, like character classes (`[ ]`), can unintentionally avoid the "nothing to repeat" error by placing modifiers within appropriate contexts. This not only helps sidestep the error but can also make the pattern more powerful.
7. It's easy to get a false sense of security when building complex regexes; however, as the complexity increases, so does the likelihood of triggering this error. It underscores the need for streamlined and clearly designed regexes.
8. Users not fully comfortable with the subtleties of regex handling might view the error message more as an annoyance than a chance to critically evaluate their coding methods. Recognizing the significance of the error can push for better coding approaches and habits.
9. Patterns using negative lookaheads or lookbehinds can ironically mask the "nothing to repeat" error since they necessitate careful positioning, but they can still lead to major logic errors if not structured correctly.
10. Ultimately, repeatedly encountering the "nothing to repeat" error can foster a coding culture that prioritizes clarity and strong testing, ultimately improving the overall quality of regular expression usage in projects.
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python - Proper escaping techniques for special characters in regex
When crafting regular expressions, it's crucial to handle special characters, also known as metacharacters, correctly. These characters, like `.`, `*`, `+`, and `?`, have predefined meanings within the regex syntax. If you want these characters to be matched literally instead of triggering their special functions, you need to escape them. This is usually done with a backslash (`\`). For example, if you want to match a literal dot (`.`) in your pattern, you'd write `\.`.
Python's `re` module offers a convenient way to manage these escape sequences: raw string notation. By prefixing your regex string with `r`, you tell Python to treat backslashes literally. This can greatly simplify writing regular expressions, especially when you have a lot of backslashes in your patterns.
Additionally, Python provides the `re.escape()` function. This tool automatically handles escaping all special characters in a string, making it ideal for when you need to incorporate user-supplied strings or variables directly into your regex patterns.
Understanding these escaping techniques helps prevent errors like "nothing to repeat at position 0", which often stems from issues with quantifiers and escaped characters. Moreover, grasping how escaping works promotes a better grasp of the underlying structure and logic of regular expressions, leading to more efficient and robust pattern matching.
1. In the realm of regular expressions, characters like `.` and `*` have specific roles. While `.` typically matches any character (excluding newline), `*` signifies repetition of the preceding element. Simply using them together doesn't automatically translate to "match any character zero or more times"—it's about how you structure the pattern.
2. The backslash (`\`) is frequently a source of confusion within regex. It's used to escape characters, essentially telling the regex engine to treat the following character literally instead of as a regex operator. This can lead to unexpected results when backslashes are used unnecessarily to "escape" characters that aren't special in the first place.
3. Constructs like `\d` (digits), `\w` (word characters), and `\s` (whitespace) illustrate that regex includes shorthand elements to boost readability and efficiency. Overdoing it with backslashes can introduce complexity and increase the likelihood of errors.
4. Regex needs context to function correctly. Grouping with parentheses, for instance, not only adjusts the order of operations but also acts as an independent entity that quantifiers can operate on, proving essential for handling larger and more complicated regexes.
5. The introduction of greedy and lazy quantifiers adds yet another dimension of complexity: `.*` (greedy) gobbles up as much as it can, whereas `.*?` (lazy) consumes the minimum required. Misinterpreting these can cause patterns to appear correct while actually producing unintended outputs or errors.
6. Character classes offer a way to circumvent the "nothing to repeat" errors. By grouping characters within square brackets, you create a unit that the quantifier can operate on. For example, `[a-z]*` is valid because `*` modifies the whole class rather than a nonexistent preceding element.
7. Testing regex patterns in online sandboxes before implementing them into production code is crucial. It helps reveal escaping mistakes or structural issues that might otherwise go unnoticed during development, underscoring the value of experimentation in mastering regex.
8. Understanding the order of operations (precedence) of operators and quantifiers in regex is essential for dissecting why some patterns produce errors. For example, a quantifier in the wrong spot can cause cascading mismatches that ripple through the entire regex.
9. The "nothing to repeat" error isn't simply a syntax problem; it forces developers to reflect on their overall approach to designing regexes. It pushes for cleaner, more well-commented code, making maintenance easier in collaborative projects.
10. The evolving ecosystem of regex libraries—some offering advanced syntax or debugging features—motivates engineers to stay informed and use the best tools available. This helps minimize common errors associated with escaping and quantification, ultimately leading to more robust and reliable regex patterns.
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python - Handling escape sequences at the start of regex patterns
When creating regular expressions in Python, correctly handling escape sequences, especially at the pattern's start, is crucial. The "nothing to repeat at position 0" error often appears when a quantifier like `*` or `+` is used without a character or group it can operate on. This usually happens at the beginning of the pattern. It highlights the need to understand the interplay of escape sequences with quantifiers and other special characters. The `re` module offers useful tools, such as raw strings (`r'...'`) and the `re.escape()` function, to help you manage these escape sequences and prevent errors caused by accidentally escaping the wrong things. Using these tools can improve your regex development, making your patterns more accurate and less error-prone. Thoroughly inspecting your regex patterns to ensure proper syntax and structure is essential not only for preventing this common error but also for fostering a stronger understanding of regular expression construction. While it's tempting to rush through writing regexes, a moment's pause to check for issues like these often avoids larger problems later on.
1. While regex offers a wide range of escape sequences, it's important to remember that not every character needs escaping. Over-escaping can lead to unintended consequences, creating confusing regex patterns that are more prone to errors like "nothing to repeat."
2. Using raw strings (prefixing your regex with `r`) in Python is critical. It stops Python from interpreting backslashes as escape characters within the string itself, which can cause problems with the actual regex pattern. This straightforward approach greatly simplifies things and avoids potential syntax snags.
3. Python's `re.escape()` function acts as a safety net when building regex dynamically. It automatically escapes special characters in any given string, which is incredibly helpful when building patterns from user input or variables. This way, you avoid the regex engine misinterpreting the pattern due to unusual characters.
4. The nuances of greedy and lazy quantifiers can get tricky when it comes to escaping and pattern construction. It's easy to misunderstand how these quantifiers grab and process input, leading to unexpected behavior and harder-to-find errors.
5. Regex shortcuts like `\d` (digits), `\w` (word characters), and `\s` (whitespace) are helpful for readability and conciseness. However, if you throw in unnecessary escapes along with these, you're introducing complexity that can trigger errors or make the pattern harder to understand.
6. Building intricate regex patterns is common, but grouping elements with parentheses not only tidies up the structure but also ensures that quantifiers always have a valid element to act upon. This careful handling helps reduce the likelihood of encountering "nothing to repeat" errors.
7. The order in which different regex elements and operators are processed (operator precedence) can cause unexpected results. If you don't keep track of how these elements interact, you can get errors like "nothing to repeat." This highlights the importance of arranging elements thoughtfully within your pattern.
8. It's a mistake to assume that an error will only appear where it originates. Often, a problem like "nothing to repeat" is a cascade effect from an earlier error in the regex pattern. This underlines the importance of testing and validating the complete expression for potential issues.
9. Before deploying a regex in a real-world application, using online testing tools or validators is a good habit. They can identify escaping mistakes and syntax errors that might slip through during development. Preventing these problems in advance can save a lot of headaches later.
10. It's important to remember that different regex engines handle complex patterns and escaping rules in different ways. This can lead to unique errors depending on the environment. Being aware of these nuances is particularly crucial when moving code between systems or integrating regex components from various sources.
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python - Tools for debugging and testing regular expressions
When working with regular expressions, debugging and testing can be challenging. Fortunately, various tools exist to assist in this process. Online platforms like Regex101 have gained popularity for their ability to highlight syntax and provide explanations, allowing you to quickly see how your regex patterns behave. Regexr offers similar capabilities with its real-time matching features and support for multiple regex flavors. If you're primarily working with JavaScript, RegViz provides a unique visual approach to debugging, revealing the structure and behavior of the regex pattern. Even Python's built-in `re` module contributes to better debugging by offering the option to preemptively detect errors during compilation, avoiding runtime issues. While there's a wealth of information on regex available, the right tools can significantly enhance your experience. Using them can not only speed up debugging but also foster a better comprehension of regex concepts, reducing the risk of common issues like the "nothing to repeat at position 0" error.
1. Many regex debugging tools provide visual aids, allowing users to see how a string matches against a regex in real-time. This can significantly aid in understanding complex patterns and pinpoint errors like "nothing to repeat," especially in intricate expressions. It's like watching the regex engine's thought process in action.
2. Several development environments include built-in regex testers that highlight errors immediately as you write the expression. This immediate feedback is much faster than the traditional trial-and-error debugging often done through a terminal. It's a small change, but it can improve the debugging workflow.
3. Online regex testers and interactive tools are becoming increasingly popular because they often support various regex "flavors," showing you how your pattern might behave in different programming languages. This is important because regex syntax can differ slightly between languages, which can lead to unexpected "nothing to repeat" errors when you move code between projects using different languages. This is a good reminder that one size doesn't fit all when dealing with regex.
4. There's a growing use of debugging libraries that specifically focus on regex, allowing developers not only to catch syntax errors but also to visualize the regex engine's processing of the pattern. It provides an insight into how the regex engine works, leading to a deeper understanding and potentially preventing many common mistakes. A helpful perspective to prevent re-inventing the wheel, especially in collaborative projects.
5. Modern IDEs (Integrated Development Environments) frequently incorporate regex linting features that pinpoint syntax errors as you write your code. This continuous feedback creates a more robust coding practice and reduces reliance on the somewhat tedious trial-and-error debugging process. This can really help accelerate your learning curve and improve coding quality.
6. Often, you can make subtle changes to your regex patterns, like using named groups or splitting functions, without dramatically impacting the overall logic. This can simplify the pattern, make it easier to manage, and minimize the chances of encountering errors like "nothing to repeat." This shows that even complex patterns can be refactored, making them easier to read and maintain.
7. The process of debugging regex can inadvertently teach pattern theory. There are tools specifically designed to illustrate how a pattern behaves when matched against a series of example strings. This interactive learning can deepen your understanding and lead to fewer future syntax errors. This approach focuses on experiential learning, which is known to be a very effective method of learning complex concepts.
8. Many regex debuggers let you create test cases to evaluate the pattern before you put it into production code. This gives you a way to verify that the regex operates as expected in various situations. Preventing problems ahead of time can save a lot of trouble down the road. This demonstrates the value of proactive testing and helps instill a more defensive approach to coding.
9. A growing number of regex debugging tools include collaborative features, enabling teams to share and review regex patterns together. This open dialogue can help prevent and resolve potential pitfalls and improve everyone's understanding of regex. When you collaborate, you learn from the experience of others, fostering shared knowledge and better practices.
10. Advancements in technology have led to the development of AI-powered regex tools. These tools use machine learning to suggest fixes and optimize regex patterns based on common errors and typical usage patterns. It's an interesting development that attempts to automate a traditionally human-driven process of learning and debugging, leveraging the collective knowledge of many users to improve the experience. Such tools might help minimize common errors, including the "nothing to repeat" error, by providing intelligent assistance and guidance during development.
Debugging Regular Expressions Understanding the 'reerror nothing to repeat at position 0' in Python - Best practices for avoiding regex syntax errors in Python
When using regular expressions in Python, avoiding syntax errors is crucial, especially the common "nothing to repeat at position 0" error. This error typically arises when a quantifier like `*`, `+`, or `?` is used without a valid character or group before it. It's a reminder that these quantifiers need something to quantify. To sidestep these issues, it's good practice to make sure quantifiers always have something to repeat. Utilizing tools like raw strings or the `re.escape()` function for managing special characters within your patterns is a helpful strategy. Also, pre-compiling your regexes with `re.compile()` can improve performance and allow for quicker error detection. Furthermore, the use of debugging tools and comprehensive testing is highly recommended to help prevent issues from slipping into production code. By establishing these practices, you'll develop cleaner and easier to understand regular expressions.
1. The "nothing to repeat at position 0" error frequently arises when quantifiers like `*`, `+`, or `?` are placed at the beginning of a regex pattern without a valid character or group to operate on. Understanding the fundamental structure of regex patterns is key to preventing this particular error.
2. While regex syntax might seem straightforward at first glance, each symbol carries a specialized meaning. Failing to grasp the context in which these symbols are used can quickly lead to unforeseen issues. Quantifiers, in particular, need a clear target (a character, a group, or another pattern) to repeat, and omitting this leads to errors like "nothing to repeat".
3. Backslashes are often used to escape characters in regex. However, overusing them for no apparent reason can create a pattern that's difficult to decipher and debug. Avoiding excessive escaping simplifies the expression, improving readability and potentially mitigating the "nothing to repeat" error.
4. Python's raw string notation (using the `r` prefix) is a powerful tool for crafting regex patterns. By employing `r'...'` for regex strings, developers avoid Python's default interpretation of backslashes as escape sequences within the string itself. This approach significantly helps with accuracy and error reduction in regex development.
5. Character classes, represented within square brackets (`[ ]`), can help overcome the "nothing to repeat" error in specific situations. They allow developers to bundle characters together, effectively providing a distinct entity that quantifiers can modify. Utilizing character classes can make regex patterns both more expressive and less susceptible to the "nothing to repeat" issue.
6. While the temptation to quickly write and deploy regex patterns is understandable, thorough testing is a vital step that many overlook. A surprising number of regex errors might stem from a lack of rigorous testing. Taking time to test the pattern across a range of possible inputs can often unveil hidden issues like misplaced quantifiers.
7. Real-time regex debuggers and testing platforms have transformed the debugging experience. They allow developers to see the pattern matching process unfold as it happens. This instant feedback can make spotting errors like "nothing to repeat" much easier, eliminating time wasted on lengthy debugging cycles.
8. Greedy and lazy quantifiers might seem simple at first but can produce unintended results if not carefully considered. Understanding the distinct behavior of greedy (`.*`) and lazy quantifiers (`.*?`) is essential for precise regex pattern design. These quantifiers have significant ramifications for match behavior and neglecting to acknowledge them could easily lead to the "nothing to repeat" error, amongst others.
9. Regex debugging, especially when encountering errors like "nothing to repeat", is an opportunity for continuous learning. It emphasizes the importance of regularly revisiting regex concepts, refining one's understanding of pattern construction, and improving coding practices related to regex. Recognizing that regex errors are opportunities for deeper understanding can push developers to strengthen their overall regex skills.
10. AI-powered debugging tools are gaining traction in assisting regex development. These tools are built with machine learning that can analyze regex patterns and offer insights into possible fixes or optimizations. Such advancements might significantly enhance regex development by helping resolve issues like "nothing to repeat" in an automated or semi-automated fashion, providing guidance to developers and ultimately increasing the overall quality and efficiency of regex usage. While the human component remains central, the evolution of regex debugging tools utilizing AI may be a welcome trend for some.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: