Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts - Understanding the Invalid Index to Scalar Variable Error
When working with video data in Python, particularly using NumPy, encountering the "Invalid Index to Scalar Variable" error can be a common stumbling block. This error signifies that you're trying to access elements within a single value, like a scalar (e.g., a simple integer or float), as if it were an array or list. This typically occurs because your code incorrectly assumes that the variable you're indexing is a collection of elements rather than a standalone value.
The root of this problem often lies in how you're iterating through or manipulating data. You might be inadvertently treating a single value as a multi-dimensional structure, leading to an indexing error. Recognizing that NumPy arrays have defined dimensions is key. Trying to access elements in a 2D array with incorrect indices will naturally trigger this error. Similarly, using multidimensional indexing on lower-dimensional data can also cause this issue.
Preventing these errors involves a blend of careful coding practices and understanding your data. Understanding the structure and type of your variables is paramount. If you're intending to index into a sequence of data, ensure that the variable you're working with is indeed an array or list, not a scalar. It's also prudent to thoroughly test code segments involving indexing, to catch any potential mismatches between your expectations and the actual data type. By diligently focusing on these points, you can improve the robustness and efficiency of your video data analysis workflows.
When we encounter the "Invalid Index to Scalar Variable" error in Python, it generally means we're trying to treat a single value (like a number or a single character) as if it were a sequence of values, like a list or array. This error often reveals an incorrect assumption about the type of data we're handling.
This type of error frequently arises in scenarios where data types are handled implicitly, especially when combining or interchanging different data structures like lists and scalars. This highlights the importance of Python's dynamic typing and the need to be mindful of how data types are interpreted and potentially converted during processing.
It's not unusual to encounter this error when using libraries like NumPy where a single-element array might act more like a scalar than a list. In those situations, the dynamic nature of Python can lead us astray if we expect array-like indexing behavior.
While Python's indexing starts at 0, attempting to access an index that's beyond the bounds of a sequence will yield an "index out of range" error. It's important to differentiate between these types of errors, as the latter signifies a valid attempt to index but an invalid index value, not necessarily a scalar variable issue.
It's worth noting that the error doesn't usually appear when working directly with Python's basic types like integers, floats, or strings. It's more likely to arise when dealing with complex or custom data structures which might be inappropriately categorized as scalars.
Therefore, a solid understanding of various data structure types like lists, tuples, and dictionaries is vital. Mistaking a scalar for an indexable collection can easily lead to perplexing errors.
Troubleshooting this error generally involves carefully scrutinizing the data types involved. Python's built-in `type()` function is invaluable for verifying whether a variable is indeed a scalar or a collection.
In the realm of data analysis, these errors can be particularly disruptive when handling intricate data extraction steps where variables are dynamically assigned. They can obstruct analysis workflows and necessitate debugging.
This type of error underscores the critical role of data validation within our processing pipelines. Unverified assumptions regarding variable types can easily introduce runtime errors, often making debugging a challenging task.
Ultimately, appreciating the relationships between Python's data types and structures not only averts this specific error but contributes to more efficient coding practices. By being mindful of data handling, we can reduce redundancies and improve our code's clarity and reliability.
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts - Common Scenarios Leading to This Error in Video Data Analysis
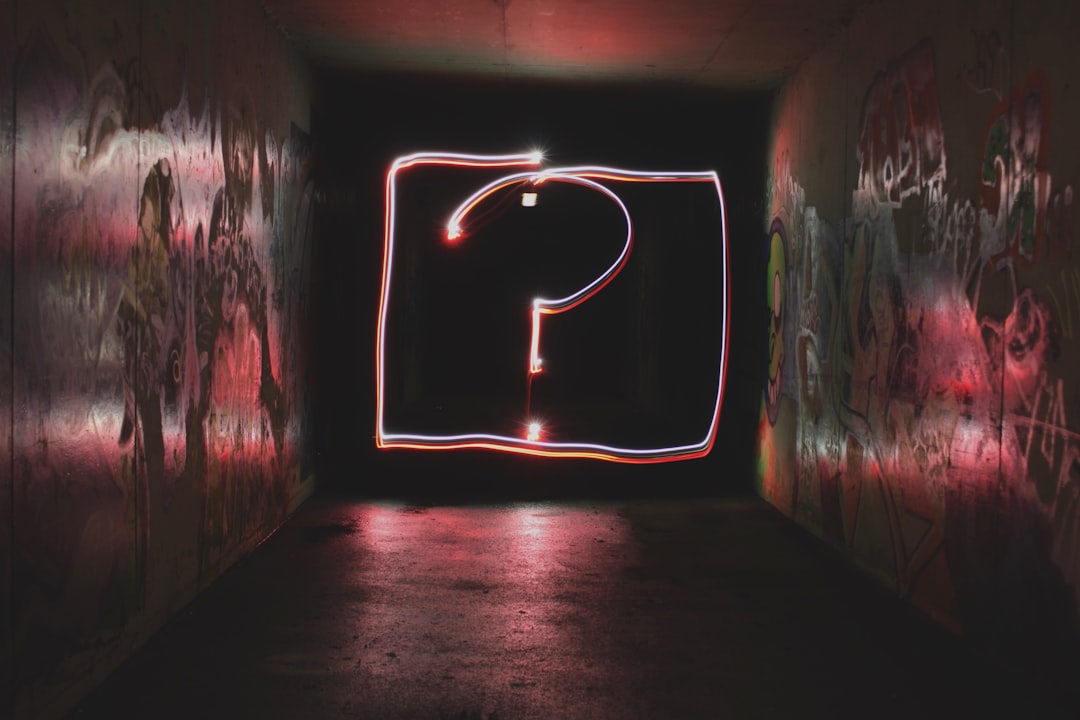
Within the realm of video data analysis, the "Invalid Index to Scalar Variable" error often arises from typical missteps in how we handle data. A frequent source is mistakenly treating simple values like integers or decimals as if they were collections like arrays or lists. This naturally leads to attempts to index into these singular values, triggering the error. Another common culprit involves improperly managing data during processing. For example, looping through a variable and then trying to index a value that no longer exists within a multi-element structure can cause this error. It's crucial to be acutely aware of the data's format, especially when utilizing tools like NumPy. Preventing these problems involves carefully checking the shape of variables and their specific data types. Through diligence in these areas, one can sidestep the common confusion that comes from unintentionally combining single values with data collections within analysis workflows.
1. **Data Type Inconsistencies**: A frequent cause of this error stems from inadvertently mixing different data types. When your code expects a single value (like an integer) but receives a list or array instead, the mismatch can lead to failure. It reinforces the need to pay close attention to the consistency of data types while manipulating them.
2. **The Curious Case of NumPy's Single-Element Arrays**: NumPy introduces an interesting wrinkle: a one-element array (e.g., `np.array([5])`) can act a little differently from a simple scalar. If you try to access this array using a standard index, thinking of it as a scalar, it can trigger the error. This underscores the subtleties of data structures in numerical computing.
3. **When Functions Don't Return What You Expect**: Many NumPy functions, when dealing with a single value, might return a scalar instead of maintaining the array structure. For example, using `np.mean()` on a single-element array yields a scalar, which can be a problem if the subsequent code expects an array.
4. **Indexing vs. Slicing: A Fine Line**: It's easy to get indexing and slicing confused, particularly with multi-dimensional arrays. For instance, using `array[0][0]` when you meant `array[0, 0]` can trigger the scalar index error. This reinforces the need to be precise with these distinct mathematical operations.
5. **The Labyrinth of Nested Data**: Dealing with nested lists or dictionaries adds another layer of complexity to data manipulation. When navigating deep structures, if you don't verify their form, you might end up treating a single returned value as a multi-dimensional entity, causing the error.
6. **List Comprehensions and Unexpected Scalars**: Problems can pop up in list comprehensions if you expect to be iterating over collections but inadvertently receive scalars. This often happens when conditional statements within the comprehension return a single item rather than a list or array, leading to unpredictable results.
7. **The Perils of Dynamic Data**: When you're dynamically generating data using loops, it's possible to end up with an unexpected scalar when an array was intended. A loop that inadvertently modifies the structure of your data can produce confusing behavior and ultimately lead to errors.
8. **Debugging's Best Friend: `print()`**: The simple `print()` function remains a powerful tool for debugging this particular error. Printing the variable types and their values just before the point where the error occurs can reveal unexpected data manipulations, guiding you to the root of the issue.
9. **The Fallout from Concatenation**: Concatenating arrays of differing shapes can produce unexpected scalar values. If, during concatenation, one array is unintentionally reshaped into a scalar, subsequent code expecting an array will likely fail. Maintaining consistent array shapes during modifications is crucial to avoid such errors.
10. **Errors Begetting Errors**: The "Invalid Index to Scalar Variable" error isn't always about the line of code where it's raised. It can be a symptom of a mistake earlier in your code. An earlier modification that silently changes data types can lead to these types of indexing failures down the line, making debugging a bit more challenging.
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts - Distinguishing Between NumPy Scalars and Standard Python Integers
When working with numerical data in Python, particularly within the realm of video analysis using libraries like NumPy, understanding the subtle difference between NumPy scalars and standard Python integers is crucial. NumPy scalars, unlike their Python counterparts, are essentially zero-dimensional arrays. This means they represent a single value but come with a broader set of associated data types including integer, float, and complex number formats. Standard Python integers, on the other hand, represent just a single integer type. While these two might appear interchangeable in some basic operations, their fundamental difference becomes apparent when dealing with operations that require strict type consistency, especially within indexing and array manipulation.
Failing to recognize this distinction can lead to the dreaded "Invalid Index to Scalar Variable" error. This error often pops up when your code inadvertently treats a NumPy scalar as if it were a standard Python integer and attempts to index into it like a list or array. It's a reminder that NumPy, designed for scientific computing, is more meticulous about data types than core Python. Understanding how NumPy handles data types and their representation is vital to ensure that your video analysis workflows avoid unexpected behaviors, maintain accuracy, and improve your overall code's reliability. Video analysts can significantly improve their coding efficiency and minimize debugging time by developing a strong understanding of the nuances of scalar data types and recognizing the potential consequences when they are incorrectly interpreted in their analyses.
NumPy's scalars, while appearing similar to standard Python integers, are fundamentally different. NumPy scalars, like `numpy.int32`, represent zero-dimensional arrays and offer a range of types including boolean, integer, unsigned integer, floating-point, and complex numbers. This contrast can lead to unexpected behaviors, particularly when interacting with array-based operations. For instance, while Python's `int` type is flexible, NumPy scalars are more rigid and optimized for efficiency within scientific computing, often using low-level optimizations not accessible to Python integers.
Interestingly, these types can sometimes be used interchangeably in Python. However, automatic type promotion can also create issues. NumPy might adjust data types during operations, potentially altering the expected outcome. Combining a floating-point NumPy scalar with an integer array, for example, will often yield a floating-point array, deviating from what you might expect from similar operations in standard Python.
Comparisons between NumPy scalars and Python integers can also become tricky due to differences in type handling, leading to inconsistencies in conditional checks. This can introduce subtle bugs, particularly in situations requiring precise type evaluations.
Even memory management can be influenced by this distinction. NumPy scalars are designed for space-efficiency, but that can introduce new types of problems if not accounted for. It is possible to have integer overflow errors due to limitations of the NumPy scalar's data type.
Additionally, the way these types interact with the broader array structures of NumPy is important. You will often find it useful to ask about the shape or dimensionality of your data using `.shape`. However, if you apply `.shape` to a NumPy scalar, it'll trigger an error. There's no shape or structure to a scalar!
Another area where this distinction matters is interoperability. Many scientific computing libraries built on top of NumPy have expectations for data to be in specific types. Feeding regular Python integers to them can lead to crashes or failures. This can also manifest in more subtle ways with NumPy's own functions or methods when they don't receive what they expect.
NumPy's indexing and slicing behaviors provide another demonstration of the divergence between the two scalar types. If you try indexing with an index that's outside of a NumPy array or with a scalar where one isn't expected you get errors. Slicing a scalar has no meaning, and trying to do so won't work and will error out.
Beyond basic indexing, interactions with NumPy scalars are worth keeping in mind when you develop your own customized types, or code. This can be problematic since it may trigger unexpected behavior when NumPy tries to operate on objects you've built if they do not conform to the NumPy scalar framework.
Understanding these nuances can help video data analysts avoid common pitfalls. This includes the need to carefully track the data types when indexing and avoid erroneous assumptions about how data is represented. With a mindful approach, these potential sources of errors can be avoided, and NumPy can continue to be a powerful tool within your video analysis arsenal.
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts - Proper Indexing Techniques for One-Dimensional Arrays
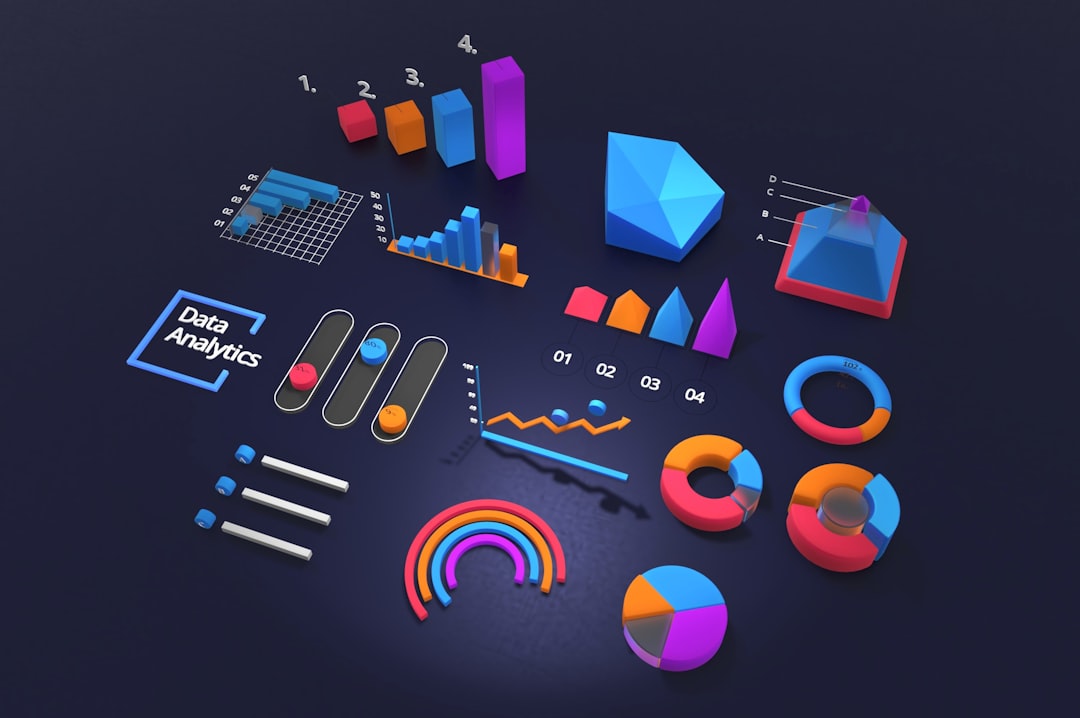
When analyzing video data with Python, particularly using libraries like NumPy, understanding how to properly index one-dimensional arrays is vital. This is especially important to avoid common errors like the "Invalid Index to Scalar Variable" error. One-dimensional arrays, which are fundamental data structures in this domain, require careful consideration when accessing their elements. Remember that Python uses zero-based indexing, meaning the first element is at index 0. A common mistake for those new to array manipulation is to try and access a scalar value (a single number) as if it were an array. This incorrect assumption directly leads to indexing errors, and hence the error. Consequently, it's essential to always verify the type and structure of your data before applying indexing operations. Being able to readily differentiate between scalar values and arrays is critical for preventing unnecessary debugging and enhancing the reliability of your analysis workflows. Leveraging NumPy's array indexing tools in an accurate way can significantly improve efficiency and eliminate common misconceptions that can trip up video data analysts.
When working with one-dimensional arrays, particularly within the context of video data analysis using NumPy, understanding how indexing works is crucial to avoid unexpected behavior. While indexing in Python typically starts at zero, NumPy introduces some subtle complexities that can lead to confusion, especially when dealing with scalar values.
One of the common pitfalls is that NumPy scalars, which represent single values like integers or floats, have no dimensions. Unlike a one-dimensional array with a shape like (3,), a NumPy scalar has a shape of (). Trying to access elements within a scalar using indexing, like you would with an array, will naturally lead to the infamous "Invalid Index to Scalar Variable" error. This is because indexing requires a sequence with a specific structure, and scalars simply don't have that.
Furthermore, NumPy's automatic broadcasting can also lead to problems. Broadcasting allows operations to be performed on arrays of differing shapes, including scalars. However, if not handled carefully, this can result in unintended behavior because scalars can be promoted to arrays of various dimensions during operations. Therefore, it's important to be aware of how this automatic data type promotion can affect the output of operations.
Another aspect to be cautious of is that NumPy scalar types can significantly impact computational performance. The type you choose for a scalar, whether `np.int32` or `np.int64`, can influence the memory used to store the value and ultimately, performance. Using a smaller data type for situations requiring a larger range can cause overflow errors, introducing unpredictable behavior when indexing, and potentially compromising the integrity of your calculations.
When working with dynamic data, like during loops, it's essential to verify the shape of the arrays you're using. If the shape of a variable unintentionally changes due to intermediate manipulations within a loop, indexing based on prior assumptions could lead to errors. Regularly checking the shape can mitigate the likelihood of these scenarios.
Interoperability with other libraries can also be problematic if they expect Python integers instead of NumPy scalars. Passing a NumPy scalar to a library that is designed for standard Python integers might lead to unpredictable results or failures. Therefore, explicit type checking and conversions are essential when collaborating with external libraries.
Similarly, type-checking with conditional statements can be challenging when dealing with NumPy scalars because subtle differences in how NumPy and standard Python handle data types can lead to logical errors. It's best to avoid relying on Python's implicit type conversions for critical comparisons and instead use explicit type-checking functions for these evaluations.
Reshaping arrays can also introduce new indexing issues. If an array is inadvertently reshaped into a scalar during an operation, subsequent code assuming it's still an array will fail. This emphasizes the importance of carefully understanding your data’s structure at each stage of a calculation.
It's equally important to realize that scalars are immutable in NumPy. This means modifying a scalar creates a new object instead of modifying the original, which is something you'd naturally expect with many other Python types.
In conclusion, proper indexing techniques, specifically understanding the differences between NumPy scalars and standard Python integers along with the behavior of NumPy's broadcasting and scalar types, are key to avoiding the "Invalid Index to Scalar Variable" error. Paying close attention to the dimensionality and data type of variables, and regularly checking their shape within dynamic contexts, can help prevent these errors and ensure your video analysis code remains robust and reliable.
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts - Verifying Data Types and Structures Before Indexing
When working with data, particularly in Python's numerical libraries like NumPy, it's vital to confirm the data type and structure before attempting to index it. This is a crucial step to prevent encountering the common "Invalid Index to Scalar Variable" error. This error typically surfaces when you try to index a single value, a scalar, as if it were an array or list. Scalars, unlike arrays, can't be indexed because they don't represent a collection of items.
To avoid this pitfall, it's necessary to ensure that the variable you are indexing is actually a valid data structure, like a list, array, or another collection designed for indexing. Additionally, understanding the dimensionality of your data, especially within multi-dimensional arrays, is vital for correctly indexing elements. This includes recognizing if your data structure has a shape like (3,) or a NumPy scalar with a shape of (). When working with dynamic data or nested structures, frequently checking data types for consistency is also important to prevent errors related to the underlying data structures. If these steps are taken, you can build more resilient and efficient video data analysis workflows in Python.
1. **The Zero-Dimensionality of Scalars:** NumPy scalars are fundamentally different from standard Python integers because they possess zero dimensions. This means they don't have a shape attribute, unlike arrays. Attempting to index into a scalar, expecting array-like behavior, will trigger the indexing error we're discussing. It's important to recognize this distinction when transitioning between using scalar values and arrays in your code.
2. **Hidden Type Conversions:** NumPy's automatic type promotion during calculations can introduce a subtle but crucial element of unpredictability. When combining different data types (like integers and floating-point numbers), NumPy might implicitly change a scalar's data type without any explicit notice. This change can unexpectedly affect your results, so keeping an eye on potential type promotions is critical.
3. **Optimizing Memory Usage:** Choosing the correct data type for your NumPy scalars (e.g., `np.int32` vs. `np.int64`) directly affects memory allocation and performance. An improper choice might lead to situations where you either waste memory with excessively large data types or face overflow errors because a data type is too small for the values involved. These unexpected behaviors can impact accuracy and cause issues during indexing, highlighting the importance of carefully selecting scalar data types.
4. **Dynamic Data's Pitfalls:** When working with dynamically generated data—often the case within loops—it's easy to inadvertently modify the shape of an array. If this occurs, relying on previous indexing assumptions can quickly lead to errors. Continuously monitoring the shape of arrays as they're manipulated within dynamic sections of your code can help prevent these issues, especially when dealing with scalar-related transformations.
5. **Collaboration Across Libraries:** NumPy's close relationship with other scientific computing libraries can be a source of confusion. If you're feeding standard Python integers to a library expecting a NumPy scalar, it might cause a crash or unpredictable results. Type consistency between NumPy and other libraries is important to avoid such issues and maintain the stability of your analysis workflows.
6. **Slicing's Structural Context:** Applying slicing operations to a scalar is fundamentally incorrect, as slicing implies that the data has dimensions. Because scalars are zero-dimensional, attempting to slice one will yield an error. Understanding this basic difference in behavior can help us avoid errors when performing manipulations on scalar values compared to arrays.
7. **Unchanging Scalars:** Unlike many other data structures in Python, scalars are immutable. Any modification to a scalar results in creating a completely new scalar, instead of changing the original object in place. This can be counterintuitive to programmers accustomed to mutable objects, so it's useful to keep this characteristic in mind to prevent confusion when modifying scalars in your video analysis code.
8. **Shape Inspection Before Indexing:** Before indexing into any array, it's a good practice to check its shape. A simple check using `.shape` can quickly reveal whether you're dealing with an array (which has a shape) or a scalar (which doesn't). Making this a habit helps to avoid common indexing errors when your code incorrectly expects an array.
9. **Type Comparisons Can Be Deceptive:** Conditionals in code can get tricky when relying on automatic type conversions between NumPy scalars and standard Python integers. There are subtle differences in how the two types are handled, which can introduce unexpected behavior in conditional statements. It is best to be explicit about your data types when doing type checking within loops, conditional statements, and other logical parts of your program to avoid mistakes.
10. **Print Statements for Debugging:** If you encounter the error, a simple `print()` statement can be your best friend. Printing the type and shape of variables just before an indexing operation allows you to detect discrepancies between your expectations (e.g., expecting an array) and the actual data (e.g., a scalar). This can pinpoint the root cause of your issue, allowing for quicker resolution.
Demystifying the Invalid Index to Scalar Variable Error in Python A Guide for Video Data Analysts - Practical Solutions for Resolving Invalid Index Errors
When tackling the "Invalid Index to Scalar Variable" error, it's crucial to recognize that it often arises from incorrectly assuming a scalar value (like a simple number) is an indexable collection like an array or list. The core of the solution is to confirm the data type and structure of the variable before attempting to index it. This means making sure the variable is indeed an array or another suitable structure, not a scalar. This pre-indexing check prevents type mismatches that are a leading cause of the error. Using Python's built-in `type()` function can be a useful tool for verifying a variable's data type.
Beyond type verification, it's equally important to have a firm grasp of the dimensions of your data. A frequent error is to try indexing a scalar, which, by definition, has no shape or structure, leading to the error. Applying methods like shape inspection and diligent type checks before indexing operations helps to prevent these errors. Adapting your indexing methods based on the structure of the data you're working with – arrays, lists, dictionaries, etc. – ensures that the indexing operations match the intended data, resulting in more reliable and precise analysis. This attention to detail minimizes data management issues, promoting smoother and more accurate workflows in video data analysis.
When diving into the world of video data analysis using Python, particularly with NumPy, understanding how to handle indexing errors becomes crucial. One common stumbling block is the "Invalid Index to Scalar Variable" error. This typically crops up when you try to index a single value (like a simple integer or float) as if it were a collection of values like an array or list. This usually occurs due to incorrect assumptions about the structure of your data.
Here's a peek into the 10 surprising ways this issue can manifest itself, highlighting the intricacies of working with data in this context:
1. NumPy scalars are zero-dimensional entities, meaning they don't possess a shape like arrays do. If you try to access elements within a scalar as if it were a multi-dimensional structure, you'll get the "Invalid Index to Scalar Variable" error.
2. Leveraging Python's type-checking capabilities, like the `type()` function, can be incredibly helpful in avoiding these errors. It's a great way to verify if a variable is indeed an array or if it's actually a scalar before you attempt any indexing.
3. NumPy's broadcasting can be a bit of a double-edged sword. While it simplifies many operations, if not carefully managed, it can cause problems when scalars and arrays interact in ways you didn't expect, especially during indexing.
4. NumPy scalars are designed for efficiency, and they take up a fixed amount of memory based on their declared type. This contrasts with the way Python's integer types can grow dynamically. If you're not careful about choosing your NumPy scalar type, it can lead to problems that might appear as indexing errors.
5. Speaking of performance, the type of scalar you use can significantly impact how fast your code runs. Higher precision scalars can slow things down when not needed, emphasizing the importance of selecting the most efficient type for the job.
6. If you're struggling with an invalid index error, a print statement placed right before the indexing step can illuminate a lot about the situation. This simple debugging technique can often reveal hidden discrepancies between what your code *thinks* the data looks like and what it *actually* is.
7. When working with nested structures (lists within lists, or dictionaries), it's easy to get confused about the dimensionality of your data. Indexing assumptions based on incorrect perceptions of these structures can lead to problems.
8. Oftentimes, an invalid index error is a symptom of a more fundamental problem that occurred earlier in your code. Fixing the error might not be as simple as just changing the line where it popped up—it could require you to trace the issue back to a prior stage in the data flow.
9. The interchangeable nature of Python's integers and NumPy's scalars can be deceiving. Some functions in libraries that are built on NumPy are designed to work specifically with NumPy scalars. Providing them with a standard Python integer could lead to errors or unexpected outcomes.
10. When working with NumPy arrays, it's essential to understand the difference between slicing and indexing, and avoid applying them incorrectly. Using either of these improperly can result in unnecessary invalid index errors, particularly when operating on scalars.
By keeping these insights in mind, you can significantly minimize the chances of encountering this error in your video data analysis workflows. It's all about understanding the nuances of how data structures are handled, especially when it comes to indexing and interacting with NumPy scalars. With a bit of extra awareness, you can write code that is both more robust and more efficient!
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: