Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling
Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling - Optimizing key selection for Python dictionaries in video metadata
When working with video metadata stored in Python dictionaries, the selection of keys plays a crucial role in maximizing performance and usability. Choosing keys that are meaningful and unique improves the readability and organization of your metadata. Since Python dictionaries rely on hashing for efficient key lookups, employing immutable data types like strings or numbers as keys is often beneficial. This is because immutable data types provide consistent hash values, enabling faster key lookups.
Beyond basic key-value pairs, you can build more complex data structures by using dictionary comprehensions and nesting techniques. This can help to capture intricate relationships and dependencies within your video metadata. While Python dictionaries excel in data handling, the potential for performance bottlenecks exists. Understanding how key selection impacts performance is therefore important when aiming for efficient metadata storage and retrieval.
1. The performance of Python dictionary key lookups is heavily reliant on the hash table implementation, making key characteristics like distribution and collision rates crucial considerations. Understanding these aspects is vital for efficient retrieval.
2. While Python's dictionary keys need to be hashable, favoring immutable types like strings and tuples is a best practice. Using mutable types like lists or custom classes without proper hashing can introduce unexpected performance issues, something to always be wary of.
3. The memory footprint of dictionary keys is influenced by their size. Shorter keys inherently consume less memory. This can be significant for large video metadata datasets, potentially improving cache utilization and overall performance.
4. A trade-off exists when selecting keys: using human-readable strings enhances readability, whereas numeric keys offer better memory efficiency. Choosing the appropriate key type depends on your priorities: easily understood data or reduced memory usage.
5. The order of keys in a dictionary impacts performance during iteration, especially for extensive datasets. Python 3.7 onwards guarantees insertion order, which can be leveraged for predictable performance. However, it also calls for careful key management strategies.
6. Frequent dynamic key changes can trigger hash table resizing, a potentially expensive operation. Having a good understanding of the dataset's anticipated size and growth can help in mitigating this cost.
7. Employing nested dictionaries (multi-level) instead of flat dictionaries offers a level of structure, but comes with potential performance trade-offs. Retrieving data from deeply nested structures can be computationally more intensive. This trade-off should be carefully considered.
8. Although we might not often think about it, Python's internal string representation is thoughtfully designed for efficiency. The variable-length encoding in Python 3 can lead to faster string operations than one might initially expect.
9. Maintaining consistent key formats, like using only lowercase strings, helps improve lookup consistency. It can also minimize the likelihood of key collisions, especially in large-scale systems with similar-sounding key names. This becomes particularly important in scenarios with a lot of data.
10. Evaluating key access patterns through profiling and benchmarking can unearth hidden performance bottlenecks. This highlights the need for continuous evaluation of key selection strategies, not just during initial implementation, but also throughout the entire development lifecycle of a project like whatsinmy.video.
Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling - Immutable data types as keys for efficient hashing in metadata handling
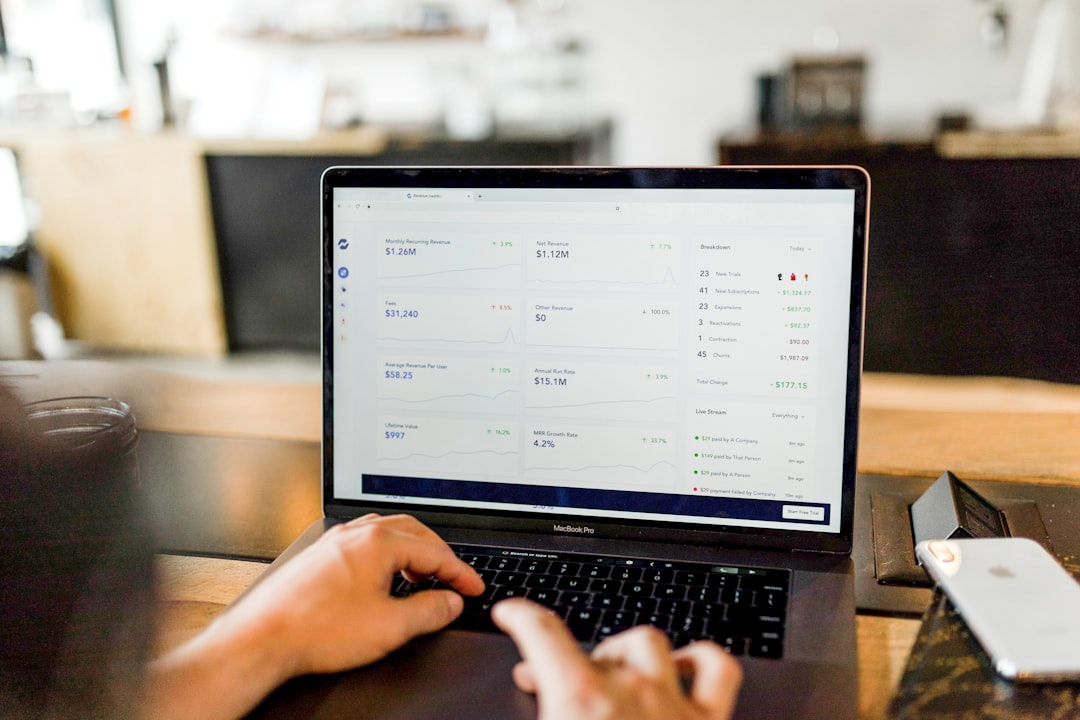
When managing video metadata within Python dictionaries, leveraging immutable data types as keys is crucial for efficient hashing and, consequently, improved performance. Immutable types like strings, numbers, or tuples maintain a consistent hash value throughout their existence. This consistency is essential for hash tables, which rely on a stable hash to rapidly locate a key. The sheer volume of data commonly associated with video metadata makes this efficiency critical, as even small inefficiencies can impact retrieval speed and data accuracy. Moreover, the selection of keys themselves is a balancing act between human readability and memory usage. Understanding how these factors interact is vital for building metadata storage systems capable of efficiently managing complex relationships within video data while avoiding common performance pitfalls. The need to strike this balance is particularly important when dealing with large volumes of metadata.
1. Immutable data types like strings and tuples offer not just consistent hash values but also simplified debugging. Since their state doesn't change, you're less likely to introduce errors by unintentionally modifying dictionary keys during program execution.
2. Python often optimizes the hashing of immutable keys using "hash memoization". This means the hash value is cached after the first calculation, speeding up subsequent lookups for frequently used keys.
3. While beneficial, using lengthy strings as keys can lead to increased memory usage. This can slow things down, especially in applications dealing with massive datasets where millions of keys might be in play.
4. Numeric keys are memory-efficient, but they can obscure the meaning of the data, making it harder to debug or comprehend, especially in team projects. It's difficult to understand numeric identifiers without some kind of additional context.
5. Python dictionaries use "open addressing" to deal with collisions. Using a range of immutable key types helps to minimize collision frequency, and this directly improves hash table performance.
6. Python strings have an optimization called "string interning". Identical strings are stored as a single object in memory, leading to faster access and reduced memory consumption when the same string is repeatedly used as a key.
7. Using tuples as keys adds some complexity to the hashing process, as each element within the tuple needs to be hashed. While handy for representing combined keys, it can potentially result in more costly hash computations in situations where there are a lot of lookups.
8. Hash tables generally give you O(1) average-case lookup time with immutable types. However, performance can degrade to O(n) if there are many collisions. This underlines the importance of good key design and carefully chosen hashing algorithms.
9. Creating custom hash functions for user-defined immutable types can lead to substantial performance gains. This allows for specialized key management tailored to the specifics of your data.
10. It's useful to understand the limitations of Python's hash table implementation. Some data types might need special handling to prevent issues, especially when mixing mutable and immutable keys within the same dictionary.
Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling - Addressing linear search limitations in large video metadata dictionaries
When dealing with substantial video metadata dictionaries, the limitations of linear search become apparent. Linear search involves sequentially examining each key until a match is found, a process that's inefficient when dealing with large amounts of data. This inefficiency arises from the time it takes to iterate through potentially countless keys, especially when lookups are frequent. Python dictionaries, by utilizing hash tables, are a more efficient way to manage video metadata. These hash tables provide rapid access to information, making retrieval far quicker than with a linear approach. However, the growing size and complexity of video data collections necessitate innovative solutions that go beyond the simple structure of a hash table. This need for improvement is driving a search for advanced techniques such as indexing methods and other data structures that can streamline the search process. To handle this scale effectively, exploring alternatives like nested dictionaries or other specialized structures for data storage is becoming increasingly crucial. It emphasizes the need for more sophisticated search strategies when managing extensive collections of video metadata.
When dealing with the vast amount of data found in large video metadata dictionaries, the inherent limitations of linear search become increasingly apparent. Even small improvements in how we retrieve keys can translate to significant performance gains, particularly when applications are processing millions of entries. This is because the time spent on repeated lookups can quickly add up.
As the size of our video metadata grows, the linear search approach quickly becomes inefficient. It's like searching for a specific book in a giant library by looking at every single title one by one. More structured ways to organize and access data, such as tries or hash tables, can dramatically reduce the search time. Instead of having to potentially check every key (O(n)), we can get the average lookup time down to O(log n) or even O(1).
Hash collisions are a common issue in hash tables. If multiple keys end up hashing to the same location, it can cause a performance hit as the system needs to look through multiple locations to find the correct value. Having a good hashing algorithm is essential for large dictionaries because it helps minimize these situations and keeps key retrieval efficient.
To address these limitations, we can utilize more advanced data structures like balanced trees or hash tables with chaining. These techniques can help manage large and rapidly growing collections of video metadata much more efficiently than simple linear searches.
Once video metadata dictionaries grow past a few thousand entries, it becomes crucial to perform some benchmarking and see how linear search holds up compared to other search methods. Doing this can give us a clear understanding of the impact on our applications and ensure everything is as responsive as we need.
In systems where the metadata is frequently changing, the linear search can quickly become a performance bottleneck. These constantly changing conditions call for a rethink of how we access the data so that updates can be handled efficiently.
Bloom filters can help reduce unnecessary searches by providing a quick initial check to see if a key might be present. While they're not perfect and might have false positives, using them together with dictionaries can quickly filter out a large portion of irrelevant keys, relieving some of the pressure on our linear search.
Blending immutable keys with dictionary optimizations and searching techniques can lead to a hybrid approach that provides a good user experience. This means faster retrieval of video metadata and less time waiting for results.
Allowing for concurrent access to keys through multi-threaded applications can help overcome the limitations of linear search, which often becomes a bottleneck when multiple processes need access to a shared dictionary.
Understanding how users are likely to interact with the video metadata is critical for designing and optimizing our search strategies. By predicting the types of queries most likely to be used, we can move away from potential bottlenecks caused by linear searches and, instead, create efficient custom solutions that fit the needs of our video metadata system.
Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling - Leveraging dictionary comprehensions for complex video metadata structures
Dictionary comprehensions offer a powerful approach for managing the intricate structures often encountered in video metadata. By enabling the concise construction and manipulation of dictionaries, they help represent the complex relationships within video data. This ability to build nested or layered dictionaries without explicit loops greatly enhances code clarity and readability. The resulting organized structure can then lead to more efficient retrieval of specific metadata elements. However, it's important to be mindful that overly complex structures, while potentially more expressive, can introduce performance bottlenecks. Achieving a balance between the descriptive power of complex structures and the need for efficient data access is a key consideration for developers working with large video datasets. By understanding these trade-offs, developers can manage vast amounts of data while maintaining optimal performance within their applications.
Dictionary comprehensions offer a powerful way to build intricate video metadata structures within Python. They let you create nested dictionaries in a concise and readable manner, essentially condensing multiple lines of code into a single expression. This not only makes code cleaner but also allows for on-the-fly filtering and transformation of metadata, potentially speeding up processing and eliminating the need for temporary data structures.
When working with hierarchical metadata, dictionary comprehensions provide clarity by allowing us to visually express the relationships and dependencies within our data. Moreover, they enable us to extract only the necessary metadata, preventing unnecessary storage and memory bloat. Since dictionary comprehensions avoid generating intermediary data structures, performance can be significantly enhanced by reducing processing overhead.
This approach is particularly beneficial when converting flat data representations into more structured nested formats. By leveraging comprehensions, we can guarantee that relationships inherent in our metadata – like genre associations with video titles – are easily accessible and maintained throughout the process. When dealing with large datasets, comprehensions offer more predictable and stable memory allocation compared to traditional methods, which can lead to unpredictable memory growth and performance drops due to excessive allocations.
Furthermore, the readability afforded by comprehensions simplifies the management of deeply nested metadata structures, facilitating a better understanding of the intricate relationships within the data. Python's automatic garbage collection further complements this approach, effectively managing memory, especially crucial in video metadata applications where frequent updates and lookups might otherwise lead to memory fragmentation.
However, a word of caution is warranted: overly complex comprehensions can negatively impact performance if not carefully designed. There's a trade-off to be mindful of – maintaining readability and clarity while also keeping an eye on efficiency, especially when dealing with extensive metadata collections. Finding the right balance is crucial for ensuring both clarity and speed in our video metadata handling systems.
Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling - Merging video metadata dictionaries using Python 5+ techniques
Merging video metadata dictionaries in Python, especially with version 5 and beyond, is a vital aspect of managing video data efficiently. Python offers several ways to achieve this, including the `update` method, a straightforward way to combine dictionaries and modify the target dictionary directly. This approach systematically combines key-value pairs, updating existing keys when they are encountered. For more compact code, dictionary unpacking allows for merging within a single expression like `z = {**x, **y}`, generating a new dictionary incorporating both input dictionaries. Another technique, the `ChainMap` found in the `collections` module, offers a convenient way to treat multiple dictionaries as a single unit without explicitly managing the underlying data structures.
While these methods are helpful, you should always consider how merging influences the integrity of your data. When dictionaries have the same keys, the merging process will typically overwrite data from one dictionary with the other. This is a key factor when dealing with large amounts of video data, where the implications for performance and data accuracy can be substantial. Understanding these dynamics, and their effects on performance, especially in resource-intensive video metadata applications, is crucial for robust data management.
1. Combining video metadata dictionaries can lead to memory savings by eliminating duplicate key-value pairs. This can be especially beneficial when dealing with large amounts of video data, where even minor optimizations in memory usage can have a significant impact on performance. However, the deduplication process itself can be computationally intensive, especially with very large dictionaries.
2. The sequence in which dictionaries are merged can affect performance. For example, merging a smaller dictionary into a larger one might be more efficient as it reduces the need for potentially complex rehashing steps, especially if there are many key collisions. This is due to how hash tables are structured and how they handle collisions.
3. Python's built-in dictionary methods, like `update()`, while convenient, can sometimes be less efficient for massive datasets. Using techniques like list comprehensions or generator expressions in conjunction with the `dict` constructor can offer better performance by avoiding some of the internal resizing operations associated with `update()`. But this approach can sometimes make the code more complicated.
4. When merging dictionaries with nested structures, it's essential to carefully handle cases where keys are shared across different levels. This complexity necessitates well-designed logic to ensure keys are resolved correctly without losing valuable information during the merge. This can be particularly challenging with deeply nested structures.
5. Using parallel or multi-threaded approaches for merging can substantially improve the speed for large datasets. By leveraging multiple processor cores, we can distribute the merge workload, significantly reducing the overall merge time. This strategy is helpful, but adds complexity to the code and may not always be a practical option, especially if the merging logic is itself complex.
6. Merging dictionaries with immutable keys, such as strings or tuples, can offer advantages in terms of consistency and error prevention. Since immutable keys cannot be modified, this approach reduces potential conflicts when merging dictionaries in multiple stages. However, generating new immutable keys for each merge operation can lead to unwanted overhead if not carefully managed.
7. Exploring specialized third-party libraries designed for efficient dictionary operations can often be a good idea. These libraries may utilize advanced algorithms and data structures not readily available within standard Python, thereby enabling better performance with larger datasets. However, introducing an external dependency could increase the maintenance burden.
8. While we often focus on optimizing merge performance during the process, improving the structure of the merged dictionary after the operation can offer long-term benefits. This includes things like rebalancing hash tables to ensure efficient lookups in subsequent operations. This can be crucial for large dictionaries where frequent data lookups are expected, though it adds a layer of post-processing that may increase overall run-time.
9. The efficiency of merging can depend on the architecture of the computer system, specifically the CPU cache. Optimizing merge operations to take advantage of cache locality can reduce the number of cache misses, resulting in improved performance. However, this type of optimization requires a deep understanding of the underlying hardware and how data is stored and retrieved, and it can be time consuming to implement.
10. Thoroughly analyzing the time complexity of different merging approaches through profiling can yield valuable insights. Sometimes, an O(N) merging algorithm can provide better overall performance than an O(1) lookup algorithm if the access patterns after the merge are predictable. This underscores the need for a holistic evaluation of the complete system, not just isolated aspects, when optimizing for performance. However, the effort to profile different techniques and develop comprehensive performance models can be considerable.
Efficient String Representation of Python Dictionaries Techniques for Video Metadata Handling - Efficient iteration methods for processing video metadata key-value pairs
Efficiently processing video metadata stored as key-value pairs within Python dictionaries is becoming increasingly important as video datasets grow larger. Optimizing how we iterate through and access these key-value pairs directly impacts the speed and efficiency of video applications. The goal is to minimize the time spent searching for and using metadata, whether it's for analysis, display, or updating.
Techniques for doing this include ensuring that dictionary keys, which are used for lookups, are immutable data types like strings or numbers. This helps keep the hash tables, which underpin Python dictionaries, working smoothly by providing consistent hash values. Further, strategies for reducing the likelihood of hash collisions, where multiple keys hash to the same location, are important. This can involve smart key selection, and possibly using data structures optimized for handling a larger variety of keys.
Beyond basic iteration, advanced techniques like parallel processing and optimized caching can significantly boost performance, particularly when dealing with massive video metadata collections. This is especially critical when systems need to rapidly retrieve and process large amounts of video metadata, such as when generating video summaries, managing large video libraries, or building automated video analysis pipelines. As the amount of video data continues to increase, finding innovative methods for efficient iteration will be crucial for maintaining application performance. There is also the question of whether other data structures, beyond simple Python dictionaries, might be better suited for handling certain metadata tasks.
1. While Python's hash tables promise, on average, O(1) lookup times for dictionary keys, performance can surprisingly dip depending on the hash function and the keys themselves. If the hashing isn't well-designed and leads to frequent collisions, lookup time can degrade to O(n), especially when the dataset is vast.
2. Research indicates that dictionary comprehensions, when used to construct complex, nested dictionaries—particularly those based on metadata attributes—can result in major performance gains. This benefit shines through when retrieving information from intricate datasets, where traditional loops might prove less efficient.
3. Using more advanced hashing techniques like cuckoo hashing alongside Python's standard dictionaries can significantly boost average-case lookup performance. This approach effectively reduces the probability of hash collisions, making it especially useful when working with extensive video metadata collections.
4. String interning, while beneficial for memory usage, needs careful management of string references. For applications handling millions of metadata entries, the optimization gains from interning can lead to huge reductions in both lookup time and the overall memory footprint.
5. Managing diverse metadata often requires a compromise between processing speed and data structure clarity. Flat dictionaries can be faster for lookups, but they lose the readability benefits of nested structures, which are often better for representing intricate video attribute relationships.
6. Exploring techniques like lazy loading for metadata offers the potential for substantial performance improvements by only initializing necessary parts of a large dataset when needed. This can minimize the burden of loading the whole dataset into memory all at once.
7. Python's built-in `ChainMap` tool can simplify working with multiple dictionaries, but there's a performance trade-off because of the overhead associated with managing several views of the underlying data. Researchers need to consider this trade-off carefully for specific applications.
8. Utilizing parallel processing with Python's `multiprocessing` module can provide significant performance boosts when merging large dictionaries. This lets the workload be distributed across multiple cores, but carefully planning the merge process is essential to prevent data corruption.
9. A critical, often-overlooked point is that adding more keys to a dictionary can, eventually, increase the average lookup time. Dictionaries often require resizing as they expand, which can lead to brief but noticeable performance dips while keys are rehashed and reorganized.
10. Benchmarking different data structures against Python dictionaries can reveal surprising results. For instance, specialized structures like tries or segment trees might have slightly slower lookups, but they can offer significant advantages in memory efficiency and faster updates—characteristics that are very desirable when dealing with video metadata that changes frequently.
More Posts from whatsinmy.video: