How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues
How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues - MoviePy NoneType Error Blocks Frame Processing at 24 FPS
When working with MoviePy, a common issue arises where frame processing grinds to a halt due to a `NoneType` error, often linked to a 24 FPS setting. This stems from MoviePy's reliance on FFmpeg, which demands a precise numerical value for the frames per second (fps). If the fps parameter isn't explicitly set and ends up as `None`, FFmpeg encounters an error, halting the process. This situation is frequently observed in constrained computational setups like Google Colab and can be compounded by older MoviePy or FFmpeg versions.
The solution often lies in manually specifying the fps value within the relevant MoviePy function calls. For instance, if your script doesn't establish the fps, assigning it to 24 might resolve the error. Beyond that, examining how arguments are unpacked in your script is crucial. Issues related to argument unpacking can sometimes lead to the fps inadvertently being set to `None`, which can disrupt the pipeline. Carefully managing these unpacking processes can make a significant difference in the robustness of your video processing routines, ensuring a more predictable and error-free workflow.
1. MoviePy's reliance on FFmpeg for video output can be tripped up by a `NoneType` error when the frames-per-second (fps) value isn't properly defined. This often manifests as a `TypeError` related to needing a number but receiving `None`. Essentially, if the fps isn't explicitly set, FFmpeg gets confused.
2. The issue with `None` fps stems from FFmpeg's inability to interpret it as a valid input. This leads to errors during video writing, hindering MoviePy's ability to produce the expected output.
3. Interestingly, older MoviePy versions or incompatibility with tools like 'ffmpegimageio' seem to contribute to this `NoneType` problem. This suggests the interaction between MoviePy and its underlying libraries can be delicate.
4. A pragmatic solution often involves directly setting the `fps` argument within MoviePy functions. If you encounter a `None` value for `fps`, simply setting it to 24, a common standard, can fix the issue. It highlights the need for careful attention to argument management.
5. This issue has been discussed in several online communities, with reports of errors related to video conversion and fps management specifically within MoviePy, demonstrating that it's a recurring concern among users.
6. Environments with resource constraints, such as Google Colab, seem to be more prone to this `None` fps error. This could indicate that MoviePy's resource management interacts in unexpected ways with different computing setups.
7. MoviePy's `write_videofile` function, if used incorrectly, can accidentally lead to the fps being incorrectly interpreted as `None`. This hints at how subtle mistakes in argument handling can propagate into larger issues.
8. Modifying code to use keyword arguments in MoviePy can sometimes resolve this error. By specifically defining `fps` during function calls, one can ensure that MoviePy receives the correct value. This emphasizes the importance of argument order and definition.
9. While the `NoneType` error can pop up in multiple Python versions, the effectiveness of various solutions appears to be version or environment-specific, which underscores that the problem is not a simple fix across all setups.
10. Proactively inspecting the input video for crucial information such as codec and fps can help anticipate potential issues. This type of pre-processing check could help prevent `NoneType` errors from derailing the entire MoviePy pipeline.
How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues - FFmpeg Version 1 Creates Silent None Returns in Video Buffer
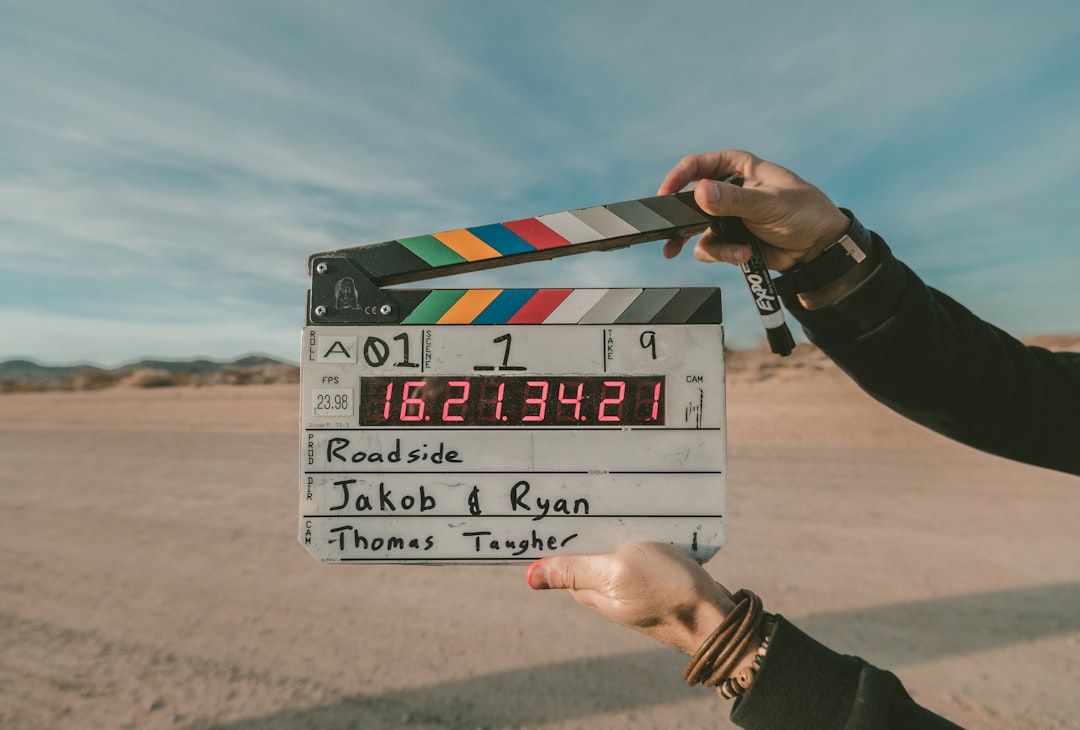
When using FFmpeg version 1, a peculiar behavior can arise where video buffers sometimes silently return `None`. This often occurs when certain operations are performed, such as removing the audio track from a video file using a command like `ffmpeg -i input.mp4 -an output.mp4`. This creates a video without sound. While seemingly innocuous, this can lead to complications downstream, particularly when integrating with Python-based video processing pipelines. The issue is further amplified when functionalities like `anullsrc`, designed for adding silent audio streams, are used. The problem arises when Python code expects specific data values from the video buffer, and instead encounters `None`. This can easily break the processing flow as code that assumes it'll be working with valid video/audio data is now presented with unexpected null values. These situations highlight how important it is to write robust Python scripts that handle unexpected null values properly when interacting with external libraries like FFmpeg. Otherwise, your scripts are prone to silent failures in a way that can be difficult to debug. Essentially, a `None` in an unexpected location can create a significant problem for the entire video processing chain, thus underscoring the necessity of attentive error handling in Python, particularly when integrating with FFmpeg.
1. FFmpeg's version history reveals that older versions may struggle with handling `None` values gracefully, potentially leading to silent audio drops or corrupted video output without any clear warning. This hidden behavior can be a real head-scratcher for users, as the problem isn't readily apparent until the output is examined.
2. The internal workings of FFmpeg's video encoding and decoding pipeline are complex, making it susceptible to issues when it encounters unexpected input types like `None`. This complexity highlights the importance of understanding how various parameters interact within FFmpeg, especially when dealing with potential error states.
3. It's quite interesting that FFmpeg, in contrast to Python's handling of `None`, doesn't have any inherent mechanism to treat `None` as a default or null value. This can create problems, particularly in situations where data types might change unexpectedly, leading to unexpected behaviour.
4. When using video filters within FFmpeg, if a filter receives `None` as an input it might inadvertently lead to the loss of the audio track without throwing a readily noticeable error. This underscores the importance of proper initialization of filter parameters and the potential for subtle errors to manifest in unexpected ways.
5. When `None` values sneak into video buffers, they can cascade through the processing pipeline, causing unexpected problems later on. This brings up a key point—rigorous validation of data types is essential to maintaining data integrity in any video processing system.
6. The fact that these silent failures can wreak havoc on automated processing workflows is intriguing. If FFmpeg silently skips processing steps because of `None` values, developers might find themselves with incomplete or broken video files without knowing the root cause. It's a scenario ripe for hidden bugs.
7. Debugging an FFmpeg processing pipeline can be really difficult when `NoneType` errors occur, as FFmpeg's error reporting isn't always very informative. This highlights the need for a thorough understanding of how data flows through the different stages of the pipeline. Many errors might remain hidden until the final output stage.
8. The interplay between FFmpeg and various video formats can introduce unexpected `NoneType` issues, especially when dealing with unusual codecs or metadata. This raises concerns about the robustness of format handling within different FFmpeg versions and implementations.
9. It's rather unfortunate that there's no built-in or universally agreed-upon way to capture `NoneType` errors within FFmpeg. As a consequence, developers frequently need to devise their own pre-processing checks and validations. This leads to increased complexity when designing and building video processing workflows.
10. Finally, a major takeaway is that continuous integration systems for video processing applications should include thorough tests using different FFmpeg versions. Otherwise, regression issues could potentially go unnoticed until they surface in production, making diligent version management crucial.
How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues - Python String Concatenation Fails With None Objects in Frame Labels
Within Python's string manipulation capabilities, a common pitfall arises when encountering `None` objects during concatenation. This often manifests as a `TypeError`, hindering the intended string joining process. This issue stems from the fact that `None` isn't a string type and thus cannot be directly combined with strings using the concatenation operator. This is particularly problematic when dealing with functions or variables that might produce `None` as a result.
The problem becomes more pronounced in applications like video processing where data flows through various stages. If a `None` value sneaks into a frame label or any intermediate data structure, it can lead to unexpected errors as subsequent operations try to process this null value. This emphasizes the importance of implementing mechanisms to deal with these `None` values before concatenation.
One approach is to carefully validate the inputs and ensure that `None` is either replaced with a suitable alternative, like an empty string, or completely filtered out if not needed. This can be achieved through conditional checks or by using tools like the `join` method along with filtering to avoid including any `None` items. Using Python's built-in string formatting features like `str.format` and f-strings also provides more flexibility and helps handle `None` cases elegantly.
By addressing these `NoneType` issues, developers can enhance the stability and reliability of their video processing pipelines. Being proactive in handling potential `None` values, especially in data-intensive workflows, is essential for ensuring a smooth and error-free user experience.
1. Python's string concatenation operation doesn't play nicely with `None` objects, resulting in a `TypeError`. This strictness underscores how easily a program can stumble if it doesn't anticipate and handle potential `None` values from user input or function outputs. It's a stark reminder that anticipating these scenarios is vital for avoiding abrupt operational hiccups.
2. Finding `None` unexpectedly during string concatenation usually implies a lack of careful input validation, which can be a red flag for potential problems further upstream in your data processing steps. It's a scenario where engineers might miss subtle issues, creating a more complex debugging experience later.
3. Python's dynamic nature, while flexible, allows `None` to sneak into lists or other data structures during list comprehensions or iterative processing without raising obvious errors. This highlights the importance of validating data types before operations, preventing headaches down the line.
4. Python's string formatting methods, such as `str.format()`, are designed to be more robust than basic string concatenation with '+', but they still don't tolerate `None` values. This highlights that seemingly minor differences in method implementation can drastically affect type handling behavior and therefore have unexpected outcomes.
5. A frequent tactic to handle `None` in concatenation is to conditionally replace it with an empty string. This solution, though functional, emphasizes that adopting good programming practices – namely, being aware of potential `None` issues – is critical for preventing them from becoming recurring problems.
6. The Python documentation emphasizes that `None` appearing during string concatenation is often a symptom of logical errors elsewhere. This reinforces the value of robust unit testing to pinpoint and resolve such inconsistencies before they impact a larger system.
7. The significance of meticulously managing variables becomes especially apparent when we consider that libraries handle `NoneType` in different ways. If you encounter an unnoticed `None` in a multimedia library, for instance, it can crash your video processing pipeline, causing a chain reaction of errors.
8. The coexistence of various data types, especially in the presence of `None`, can lead to inconsistent behavior and a range of challenging-to-debug bugs across different environments. This underscores the need for code that can anticipate type diversity and adjust accordingly.
9. The effect of `None` in applications that rely heavily on data is often overlooked, especially in logging or reporting. These systems are vulnerable because logs with `None` references can make troubleshooting unnecessarily difficult or lead to incorrect conclusions.
10. In collaborative coding projects where developers might have different styles or standards, the introduction of unexpected `None` values can disrupt the entire process. This underscores the need for stringent coding standards and thorough documentation to ensure that all contributors have a shared understanding of expected data types.
How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues - Threading Lock Issues With None Values in OpenCV 8 Pipeline
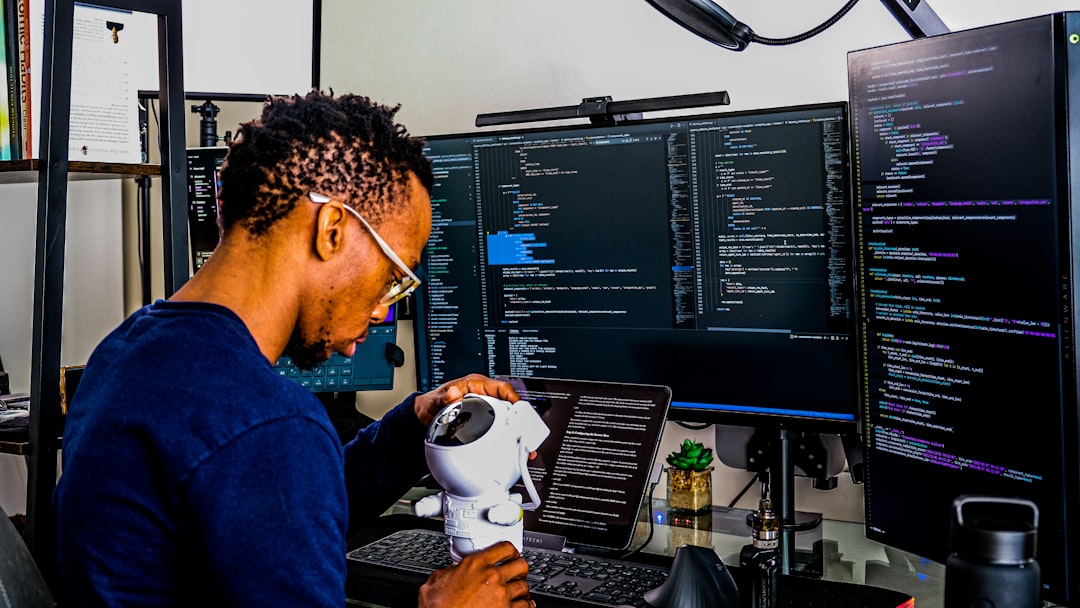
OpenCV's pipeline, especially when using the multithreading features in version 8, can become vulnerable to complications when `None` values enter the fray. These `None` values can cause `TypeError` exceptions during unpacking, particularly when multiple threads try to use shared elements like video frames. This is a classic example of a race condition where threads clash trying to access data, leading to a messy situation. The importance of properly handling threading locks becomes incredibly apparent. If something goes wrong with how threads synchronize access to shared resources, you'll see error messages like "fctx->asynclock failed". These lock errors can significantly impact performance, slowing down your pipeline and even causing erratic behavior. When `None` values aren't dealt with promptly, they can ripple through the processing, possibly jeopardizing the reliability of your video processing tasks, especially in real-time applications. To create a stable video processing system in OpenCV, you must create code that can handle these unexpected `None` situations. Incorporating strong error handling and validation in your OpenCV video pipelines is vital to ensure everything runs smoothly and prevents the cascading effects of these threading errors.
1. When using OpenCV's multithreading features, `None` values in the pipeline can cause significant headaches due to the potential for race conditions. Multiple threads trying to access and manipulate shared resources like video frames can lead to `TypeError`s if a thread encounters `None` where it expects valid data.
2. Imagine a thread in your OpenCV pipeline trying to process a frame, only to find a `None` where it was expecting a proper image. If thread synchronization isn't meticulously managed, this can lead to deadlocks or outright crashes, making debugging a real challenge.
3. The problems with `None` become especially evident in pipelines designed for high-speed processing. If these null values aren't handled, they can pile up, causing latent errors that appear seemingly at random—only manifesting during particular operations. It's like a ticking time bomb that eventually goes off in an unexpected way.
4. It's interesting to note that how OpenCV manages uninitialized variables or resources can change drastically between single-threaded and multithreaded environments. A piece of code might work fine when a pipeline is running in one thread but behaves erratically in multithreaded mode due to `None` values appearing unpredictably.
5. When using multithreading in OpenCV with Python, it's surprisingly easy for `None` values to sneak through the pipeline undetected. Since exception handling is typically limited in scope, you often have to write very explicit checks for `None` to avoid cascading failures and unraveling your processing pipeline.
6. In real-time applications like surveillance or live streaming, encountering `None` during frame operations like resizing or color conversion can cause problems. If a function expects a proper frame but gets `None`, it can silently fail, negatively impacting the user experience.
7. OpenCV's threading model is crafted for speed, but without careful validation, `None` can cause unexpected performance issues. It can lead to a dramatic slow-down or cause the pipeline to terminate early, a rather undesirable outcome for computationally-intensive video processing tasks.
8. A particularly sneaky aspect is that when one thread encounters a `None` and fails silently, other threads might continue blissfully under the illusion that everything is okay. This compounding of failures can create an even bigger mess than an immediate error.
9. Debugging these kinds of issues in multithreaded OpenCV pipelines can be a real puzzle. `None` values don't always lead to immediate, clear-cut exceptions. They can cause a build-up of unhandled internal states, making it difficult to pinpoint the exact source of the problem.
10. As engineers working on these pipelines, a smart approach is to introduce logging specifically designed to catch instances of `None`. This can help trace back the origin of data irregularities in the pipeline, particularly crucial when multiple threads are at play. This will allow you to pinpoint where the data is becoming corrupted, so that you can then attempt to rectify the issues in code.
How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues - Memory Leaks From Unhandled None Values in Video Stream Cache
When dealing with video stream caches, a common issue leading to performance problems is memory leaks caused by unmanaged `None` values. This often occurs when video processing libraries, like OpenCV, interact with systems like GStreamer in ways that don't properly release memory. It also arises in situations where video pipelines are repeatedly started and stopped without explicitly releasing the allocated memory. The problem worsens over time because each instance of this poor handling adds to the memory footprint. Eventually, it can lead to noticeable performance degradation as the system's resources become strained.
Another layer of complexity comes from Python's garbage collection. While it typically cleans up unused memory, it might fail to identify and release objects associated with these lingering `None` values, especially in the context of video processing. This necessitates explicit checks for and management of these `None` states.
To build reliable video processing systems, incorporating rigorous error handling and validation is critical. Failure to address these `None`-related issues can lead to unpredictable and potentially severe memory leaks, ultimately hindering the performance and reliability of the whole video pipeline. Careful attention to potential `None` values is thus vital for building robust and high-performance video processing solutions.
1. Memory leaks in video processing pipelines can stem from unmanaged `None` values within video stream caches, where the garbage collector might fail to release memory associated with objects that are essentially unusable due to null conditions in the code. This gradual memory build-up can noticeably hurt performance over time.
2. The presence of `None` values in a video stream cache can lead to an accumulation of unused objects in memory, potentially culminating in an application crash due to memory exhaustion. This is particularly critical for applications that run continuously, a common trait in video processing.
3. Due to Python's dynamic typing system, a `None` value can easily propagate throughout a video processing pipeline, increasing the risk of memory leaks. This flexibility, while beneficial in other scenarios, can also create silent errors, which can be hard to identify and fix, making a case for better type-awareness.
4. Data structures like Python lists and dictionaries, if populated with `None` values, might inadvertently cause inefficiencies and increased memory usage. It's a good practice to regularly clean up lists by removing `None` elements.
5. Memory profiling tools sometimes show `None` values in caches as seemingly valid references, making it challenging to pinpoint memory leaks. To diagnose these subtle memory problems, it's crucial to have a grasp of Python's reference counting and garbage collection mechanisms.
6. In multi-threaded video processing, `None` values can introduce unpredictability into memory management since threads might not synchronize access to resources correctly. This can cause threads to hold on to memory for longer than needed, hindering the garbage collector's ability to efficiently free it.
7. New features and updates to video processing libraries, like OpenCV, might unknowingly introduce code paths that don't handle `None` values well, potentially leading to memory leaks. Developers need to review their code periodically in light of library updates to avoid these problems.
8. Debugging memory leaks associated with `None` values requires logging cache states and memory usage over time. Anomalies in these logs can point to the root cause of leaks, even when they are difficult to find.
9. Video processing applications involving streaming might benefit from customized cache management. By actively controlling the lifecycle of cached frames and preventing `None` values from lingering, developers can improve performance and resource usage.
10. The consequences of unhandled `None` values go beyond just slowdowns. Video processing pipelines can generate unreliable results like dropped frames or corrupted data due to improper caching. This further emphasizes the need for meticulous error handling in video pipelines.
How Python's None Type Can Break Your Video Processing Pipeline A Deep Dive into TypeError Unpacking Issues - Deep Learning Model Output Returns None Instead of Tensor Shape
When working with deep learning models, particularly in frameworks like Keras, a situation can arise where the model's output is `None` instead of a tensor with a defined shape. This can be problematic because it disrupts the expected flow of data and can cause errors later in your processing pipeline.
Usually, `None` in an output shape signals that the batch size is variable, a common feature in Keras. However, encountering `None` can also be a sign that something is amiss in your model. Perhaps your model's architecture isn't properly configured, or maybe there's a problem with how you preprocessed the data before feeding it into the model. For example, if your model doesn't have the right reshape layers, it may not generate a proper tensor shape.
The issue with `None` isn't just that it lacks a shape—it can also trigger `TypeError`s if your code attempts operations that assume the output will be a valid tensor. This reinforces how critical error handling is in any video processing chain. You need to have checks in your code that can catch these unexpected `None` results and gracefully handle them, preventing the pipeline from crashing.
To get around this, you'll want to pay close attention to the design of your deep learning model and make sure the layers and data are compatible. Verifying your data carefully before you train your model can help prevent these `NoneType` issues in the first place. It's a good practice to ensure the model architecture is correctly configured and that the output dimensions are as expected. This proactive approach will increase the stability of your deep learning components.
1. It's a bit surprising that deep learning models sometimes return `None` instead of a tensor with a defined shape. This often happens in frameworks like TensorFlow or PyTorch if there aren't sufficient input checks. It can make debugging a headache because you're expecting a specific data type and get nothing instead.
2. Sometimes, a model outputting `None` might be a clue that there's a bug in how the model is trained, like a problem with how gradients are calculated. This shows how all the different pieces of a deep learning project are connected and how important each one is.
3. Relying on certain data types can backfire. If you design a model to return a tensor, but it ends up returning `None` because of some condition in the code not being met, it can cause problems in the following steps of your process. This emphasizes how delicate machine learning pipelines can be.
4. It's easy to forget to check for `None` immediately after running a model. If you don't validate the output before using it in your code, it can lead to errors in other parts of your program, like when analyzing or visualizing data. A good practice is to prevent these cascading errors with more careful coding.
5. In systems where models are used in real time, getting `None` as an output can stop the whole request. This shows just how important it is to be prepared to deal with `None` values. These situations can cause big performance slowdowns and affect user experience, especially in production environments.
6. Debugging models that return `None` can be difficult. Many deep learning frameworks don't give you very helpful error messages when things go wrong. It's important to create your own custom error handling, especially if you want production-ready code.
7. It's interesting that trying to improve model performance by changing hyperparameters can sometimes lead to it returning `None`. This can be caused by modifications that disrupt how data flows or the expected data structure. This is another reason why careful checking at each stage of experimentation is needed.
8. `None` in outputs can happen with complex model architectures like nested networks. This can occur if latent variables in hidden layers don't always make it to the output. This shows how important it is to keep your model designs clear and easy to understand, paying attention to the flow of data through the layers.
9. It's worth noting that frameworks can handle `None` differently. For example, TensorFlow might give you an error if it sees `None`, but PyTorch might just continue without a warning. This makes it hard to have consistent code across libraries.
10. The effects of not validating model outputs aren't limited to your code. In systems that combine machine learning with things like web services or IoT devices, getting `None` can cause major problems and stop the system from working as expected. Therefore, it's crucial to add thorough checks to your code before it's deployed.
More Posts from whatsinmy.video: