` for sections like cast and genre lists. These are the building blocks that developers use to display and structure the information on the page, and thus are the targets when we want to extract data with code.
4. Tools like Beautiful Soup and Requests are very popular for web scraping because they are easy to use and are good at parsing website elements. Beautiful Soup is particularly adept at taking the raw HTML and turning it into a format that's easier for Python to handle. Requests on the other hand, makes it simple to retrieve the HTML code itself from the IMDb site.
5. To acquire metadata, a program can send a request for the IMDb page, then utilize libraries like Beautiful Soup to parse the HTML code. The parsing logic essentially "reads" the HTML structure, looks for specific tags (like `
` for the title) and extracts the text they contain, thus grabbing the metadata we desire.
6. It's important to be aware of IMDb's terms of service when extracting information from their website. Their policies likely exist to prevent overloading their servers and ensure that users have a positive experience. Violating these rules could lead to IP blocks or other limitations on your ability to access the site.
7. If IMDb has a well-defined API, using it to access information can be simpler than parsing HTML. This is because APIs offer data in a ready-made, organized format, such as JSON. APIs often have their own constraints, including rate limits, but when available, they are generally preferred to scraping.
8. When sending HTTP requests to websites with code, it's a good practice to include a "user-agent" header. This header helps to mimic the behavior of a real web browser, which can improve your chances of not being blocked or flagged as a bot.
9. If you want to extract a lot of data, you might have to deal with pagination. Sites like IMDb often display search results in chunks, and you'll need to code to automatically navigate to the next page of results to grab everything you're interested in.
10. When extracting data, it's a good idea to include error handling in your Python script. This is because websites often change their structure, or sometimes, information may be missing entirely. A robust script should account for potential errors and either skip problematic pages or gracefully handle missing information. This is important, since any script accessing a web page is susceptible to unintended alterations in how the pages are structured, and anticipating such disruptions is essential for preventing failures.
How to Extract Video Metadata from IMDb Using Python A Technical Guide for Content Creators - Parsing Video Information Using BeautifulSoup4 and Requests
This section focuses on the core techniques of "Parsing Video Information Using BeautifulSoup4 and Requests." These Python libraries are fundamental for web scraping, enabling us to retrieve and analyze HTML content from websites. To gather video metadata from IMDb, the process involves employing the `requests` library to fetch the desired web page. Once retrieved, the `BeautifulSoup` library is used to dissect the HTML structure and isolate relevant information like video titles, descriptions, and upload dates. The effectiveness of this approach rests on carefully identifying the HTML tags that contain the desired data, along with implementing robust error handling to account for potential changes in website structure. This adaptability is essential for creating scripts that can endure over time without becoming obsolete. Moreover, libraries like `pandas` can be employed to structure and organize the extracted data, enabling more in-depth analysis and management.
1. BeautifulSoup4 isn't just about grabbing text; it can also extract details like image URLs or links embedded within the HTML. This allows us to delve deeper into video-related elements, like understanding the source of images used in video descriptions or linked articles. It gives us a richer picture than simply the textual information.
2. The Requests library isn't limited to simple `GET` requests. It supports other HTTP methods like `POST` or `DELETE`, which open up possibilities for more involved interactions with websites. You could theoretically submit forms or try to modify data on a website. While not relevant for common IMDb scraping scenarios, it showcases the wider potential of the library.
3. `BeautifulSoup` uses a tree-like structure to represent the HTML. This tree-like structure makes it easier to navigate the often-complex page layouts of websites. Imagine finding specific cast lists or user reviews within a complex page, it’s much easier to maneuver within that structure versus having to search through a continuous stream of text.
4. IMDb's HTML is typically quite dense with many elements that aren't of interest. Extracting just the critical information demands a careful approach using CSS selectors to sift out what's useful and eliminate the noise. This type of selective parsing needs precision and a good understanding of how websites are structured.
5. Python, with libraries like `asyncio` or `aiohttp`, can handle concurrent requests. This is incredibly valuable when dealing with lots of pages or large amounts of data, as it can dramatically speed up the scraping process compared to requesting pages one after another. It's a powerful way to make our web scraping tasks much more efficient, especially for datasets spread across many pages.
6. One of BeautifulSoup's strengths is its ability to deal with HTML that's not perfectly structured. This is beneficial because sometimes a website's code has errors or isn't perfectly formatted. Even with somewhat messy HTML, we can still often retrieve the desired data, highlighting the robustness of BeautifulSoup in real-world web scraping scenarios.
7. Extracted data frequently needs cleaning and standardization for analysis. We might need libraries like `pandas` to reorganize data that comes in various, inconsistent formats. This demonstrates that data extraction is only the first part of the process; there's a considerable post-processing step involved in getting the data ready for analysis.
8. While `BeautifulSoup` is great for standard HTML, it struggles when websites heavily rely on JavaScript to dynamically generate content. For those cases, you might need to use a tool like Selenium to properly handle the JavaScript elements to acquire the required information. This is an important limitation to keep in mind when encountering more complex web structures.
9. When scraping, it's vital to carefully consider the ethical implications. We need to be mindful of how our scraping actions might affect both the website's server and other users. Websites like IMDb likely have data access policies, and it's crucial to adhere to them. Excessive scraping can have negative consequences, from overloading servers to creating a poor user experience for legitimate visitors.
10. `Requests` and `BeautifulSoup` are popular due to their simplicity and ease of use. However, it's essential to respect website restrictions like request limits. Going beyond those limits can lead to your IP address being blocked. A less-common technique is to space out your requests to try to mimic human behavior and make it less obvious that you are a bot automating scraping. It's a more subtle, though possibly more effective, approach in the face of sophisticated website security.
How to Extract Video Metadata from IMDb Using Python A Technical Guide for Content Creators - Creating Functions to Extract Title Release Date and Runtime Data
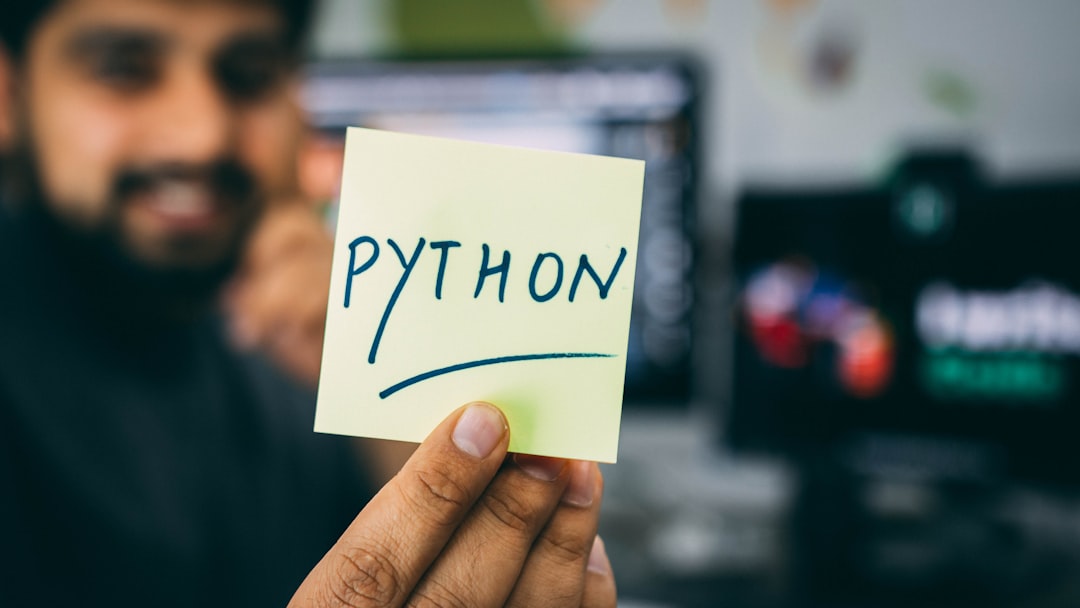
This part focuses on building functions specifically designed to extract key movie details like title, release date, and runtime. We'll see how Python, along with tools like the IMDbPY library and the `requests` library, can be used to create these functions. These functions will send requests to IMDb's API, handle the incoming JSON data, and pull out the information we need.
It's crucial to understand how to deal with potential problems that might crop up during the data extraction process, such as network timeouts or unexpected data structures. We'll discuss how to design functions that are robust and can handle these situations effectively.
A major advantage of creating functions for this purpose is the ability to automate data gathering. This allows you to gather metadata for multiple movies systematically, which can be incredibly useful for creators who work with large datasets or need to keep their metadata updated frequently. By automating this process, developers can significantly boost the speed and reliability of their content management workflows.
1. When creating functions to pull out the release date and runtime from IMDb, you often encounter different date formats, like "July 16, 2023" or "2024-10-24". This means you need to carefully choose how you parse the data to make sure all dates are in a consistent format for later use.
2. The way IMDb's pages are laid out can change a lot depending on whether you're looking at a movie, a TV show, or a documentary. This means that functions written to get data from one type of page might not work on another. This emphasizes the need to be able to adapt your parsing techniques.
3. Runtime information is usually given in minutes, but sometimes you'll see it in odd ways, like "1h 50m". This can make extraction difficult. The functions you write must not only correctly find the runtime but also turn it into a uniform number for easier analysis.
4. It's not unusual for functions to break when a website like IMDb changes its design. This highlights the need for ongoing adjustments to keep the extraction working. It's also really important to have good error and exception handling in the code so it can handle unexpected situations and keep going.
5. IMDb uses different languages in its pages, depending on things like the user's preferences or the country where the content originally came from. This makes things a little more complex. Your extraction functions need to be ready to handle this multilingual aspect when getting things like the title or release date.
6. The time zone a movie is released in can affect the way the release date is perceived, especially for titles released in different countries. So, when extracting this data, it's a good idea to think about how you'll make sure dates are shown in a way that avoids confusion globally.
7. Different parts of the world have their own ways of writing runtimes and release dates. Understanding these variations can make your extraction functions more accurate. This means your code might need extra logic to handle this diversity in formatting.
8. How quickly data is extracted can depend on the method used to parse the HTML. `BeautifulSoup` is easy to use, but other tools like `lxml` can be much faster when parsing, which is useful for dealing with lots of data.
9. The functions you write need to ensure that the data they collect is not only correct but also follows IMDb's API rules. If you don't follow the rules, they could limit your access to data, which could have a negative impact on your project.
10. Sometimes, IMDb uses JavaScript to add content dynamically to pages, which can complicate data extraction. The functions you build should be prepared to handle these situations. This may involve integrating tools like Selenium if the information you need isn't available in the initial HTML that's loaded.
How to Extract Video Metadata from IMDb Using Python A Technical Guide for Content Creators - Handling Rate Limits and Error Messages During Web Scraping
Web scraping, especially when targeting video metadata on sites like IMDb, often involves encountering rate limits and error messages. Websites implement rate limits to safeguard their servers from being overwhelmed by excessive requests from a single user within a specific timeframe. When your scraping encounters an HTTP status code like 429, signifying "Too Many Requests," you need to adjust your approach. This usually involves introducing delays between your requests. You can use the `time.sleep()` function within Python to create these pauses, or explore libraries that offer built-in mechanisms for managing retries and adjusting the delay ("backoff") between attempts.
Furthermore, if the website offers an API for accessing data, utilizing it is often a preferable approach. APIs provide a structured method for retrieving data and often help avoid errors. It's also critical to abide by the website's rules for scraping, such as following the instructions outlined in their `robots.txt` file. Implementing effective error handling in your code is also crucial, particularly when dealing with websites that frequently update their structure and content. Properly handled errors allow your scraper to continue functioning more reliably and adapt to inevitable changes in the website's design. This holistic approach ensures that you not only comply with website restrictions but also create a robust and sustainable scraping process for gathering the desired metadata.
1. Websites often impose rate limits to prevent excessive requests from overwhelming their servers and to discourage abuse. When scraping, particularly from a site like IMDb, understanding and adhering to these limits is crucial to avoid being blocked. Exceeding limits can lead to temporary or even permanent IP address blocks, hindering data extraction efforts.
2. Error messages are a crucial part of the scraping process. They can help identify issues like a page not being found (404 error), forbidden access (403 error), or other problems that might arise. Understanding the meaning of different error codes is essential for debugging and refining your scraping strategy.
3. Some websites communicate their rate limits through response headers, such as "RateLimit-Remaining" or "Retry-After". Paying attention to these headers can help you adjust your code dynamically, ensuring you stay within the allowed request limits. It’s like the website is giving you a subtle hint to slow down.
4. One approach to dealing with rate limits is to employ an exponential backoff strategy. This involves increasing the delay between requests after encountering an error, effectively adapting to server responses and slowing down if you're being flagged. It's a way to let the server "breathe" and prevent further blocks.
5. HTTP status codes can provide insights beyond just errors. A 200 status code means your request was successful, whereas a 500 code suggests a server-side issue that's unrelated to your scraping. Understanding the various status codes can give you a much better understanding of what your scrapers are seeing.
6. Error messages are more than just warnings – they're often valuable insights into a server's expectations and restrictions. Examining the error content allows you to adjust your scraping strategy, leading to more stable and compliant code over time. It's like the server is giving you a clue about how to behave.
7. Pinpointing the root cause of errors can sometimes be challenging. It often requires meticulous logging of failed requests and their associated responses. This type of detailed error handling is essential to troubleshoot problems effectively, enhancing the overall stability and robustness of the scraping operation. You basically need to be able to understand what went wrong before you can fix it.
8. Rotating the user-agent in your requests can minimize the chances of being detected as a bot. Each request will appear as coming from a different browser, making it more difficult for websites to pinpoint and block your scraping activities. It's an interesting way to "blend in" and avoid being noticed.
9. Introducing intentional delays between requests is an intuitive way to respect rate limits. These delays can align your scraping activities with normal browsing behaviour and help you stay within acceptable limits. It's a simple but effective strategy to avoid running afoul of rate limiters.
10. Many scraping libraries include built-in functionalities for handling common errors, making your code easier to write. For example, using features like retry mechanisms can reduce the need for custom error handling, simplifying the process and ensuring a more robust scraping setup. This is a big advantage for researchers and others who don't want to reinvent the wheel with their code.
How to Extract Video Metadata from IMDb Using Python A Technical Guide for Content Creators - Storing Extracted Metadata in JSON and CSV Formats
When extracting video metadata from IMDb, storing it in JSON or CSV formats provides versatile options for content creators to manage their information. JSON's hierarchical structure is ideal for handling intricate data, like detailed cast information, where data can be nested within each other. On the other hand, CSV presents a simpler, table-like format, making it very compatible with tools like spreadsheets and databases. Python, with libraries such as Pandas, smoothly integrates with both formats, making it easy to work with and transform the data. It's crucial to remember that each format has its strengths and weaknesses, and choosing the right one depends on the type of data being extracted and how you plan to use it. To make the stored metadata more reliable, especially if IMDb's website structure or data changes, implementing solid error handling during the metadata extraction phase is critical. This ensures the stability of your stored information.
Storing the extracted metadata in JSON and CSV formats presents distinct advantages and drawbacks. JSON, with its hierarchical structure, is well-suited for storing complex video metadata, such as cast lists and genre information. This allows for more intricate data queries and analysis without losing the relationships between different data points. While CSV is simple to read and edit in a text editor due to its tabular nature, this very simplicity can be a problem when dealing with intricate data relationships. JSON's ability to handle nested data leads to smaller file sizes in many cases, which can improve performance for large datasets when using Python.
However, CSV has limitations when dealing with special characters, often requiring extra processing steps to prevent data corruption if the movie title or other metadata contains unusual characters, commas, or quotations. Furthermore, CSV treats all data as strings, requiring explicit type conversions in Python. This potential for errors can be a source of frustration, especially when trying to use numeric or Boolean data directly.
JSON, with its inherent data typing capability, lets us work directly with strings, numbers, and booleans when scripting, streamlining the analysis process. This inherent structure also makes it easier to document the data format since JSON provides metadata within the structure itself. These details make it easier to understand the data and keep things maintainable, a feature CSV lacks.
The modern web leans heavily on JSON for data exchange through APIs. This makes JSON a more versatile format if you want to integrate data retrieval with external services or your own databases. Writing a script to extract metadata into JSON is often easier and more concise thanks to Python libraries like `json`. This keeps the code clean and reduces the chances of errors compared to manually formatting the same data into CSV.
In more practical applications, JSON’s flexibility allows for data conversions into formats like XML or direct database entries, giving us greater control over data storage. However, CSV is typically stuck with simple tabular layouts. JSON can effectively store repeating metadata, like multiple directors or genres for a single film, through the use of arrays. While you can imitate this in CSV, it makes the data structure more complex and leads to a greater risk of errors when manipulating it.
In conclusion, choosing between JSON and CSV hinges on the specific needs of your project. If the video metadata is complex and involves nested elements, JSON's hierarchical structure and flexibility make it a clear choice. Its capacity for handling special characters and data typing makes it more robust for various analysis methods. However, if you only need a simple tabular format for basic reporting, CSV is certainly a valid alternative. But if your data has some complexity, or if you want to use external APIs, JSON will most likely be a better choice in the long run for robustness.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: