Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows - Understanding Python3 Subprocess Pipe fundamentals
Delving into the core mechanics of Python3's `subprocess` module provides a foundation for effectively managing external command execution. Essentially, it empowers us to create and interact with child processes, offering flexibility for running various external programs. The `Popen` function stands as a central component, providing granular control over input and output streams through parameters like `stdout=subprocess.PIPE` and `stdin=subprocess.PIPE`. This capability is particularly valuable when building intricate workflows involving chained commands, which becomes crucial in areas like video processing that deal with large amounts of data. Moreover, the `communicate()` method simplifies the exchange of data between processes, while robust error handling and careful monitoring of return codes are paramount for maintaining the integrity and stability of scripts relying on subprocesses. However, one must exercise caution when using `shell=True`, as it can introduce security risks. Ultimately, the `subprocess` module supersedes older approaches like `os.system`, offering a more robust and adaptable mechanism for process management.
1. Python's `subprocess` module provides a structured way to manage external programs, particularly vital when dealing with the complex command chains often encountered in video processing. This interaction is facilitated through the control of standard input and output streams, avoiding reliance on older and less flexible methods.
2. The beauty of `subprocess`'s piping capabilities lies in its ability to chain commands. One process's output seamlessly becomes another's input, streamlining data flow. This approach avoids the creation of intermediate files, improving efficiency—a critical aspect when dealing with potentially massive video datasets.
3. The `subprocess.PIPE` construct gives developers granular control over inter-process communication by allowing them to directly interface with the input, output, and error streams of a child process. It's a significant improvement over older methods where process interactions were less explicit and manageable.
4. However, `subprocess.PIPE` can be a source of problems if not handled carefully. If the parent process doesn't consume output quickly enough, buffers can overflow, leading to deadlocks. In video processing scenarios, this could abruptly halt a workflow, potentially disrupting critical steps.
5. When performance is paramount—as it often is in video encoding or decoding—using `subprocess` to call external command-line tools can offer a significant speed advantage over pure Python implementations. This is due to the ability to leverage the optimized nature of native programs designed for these tasks.
6. `subprocess.run()` simplifies process management considerably. This function handles process spawning, waiting for completion, and capturing outputs all within a single call. It promotes cleaner, more maintainable code compared to the fragmented approach required with older methods.
7. The `universal_newlines` option within `subprocess` is quite useful for automatically converting byte streams to strings. This feature handles the different character encoding schemes encountered when working with diverse video file formats.
8. Employing the `setpgrp` option gives a spawned subprocess its own process group, making it easier to manage termination signals. This is beneficial when running scripts across numerous video files, as in batch processing jobs, allowing for more fine-grained control of termination behaviors.
9. The way `subprocess` functions can differ across operating systems, particularly in handling signals and return codes. For instance, how things work on Windows might be quite different than on Unix systems. Thorough testing across relevant platforms is essential to ensure your video processing scripts perform as expected.
10. Python's ability to combine `subprocess` with asynchronous programming features, such as `asyncio`, offers a powerful way to manage multiple video processing tasks concurrently. This can significantly enhance the performance and responsiveness of applications that handle a high volume of multimedia data.
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows - Leveraging Popen for advanced command execution control
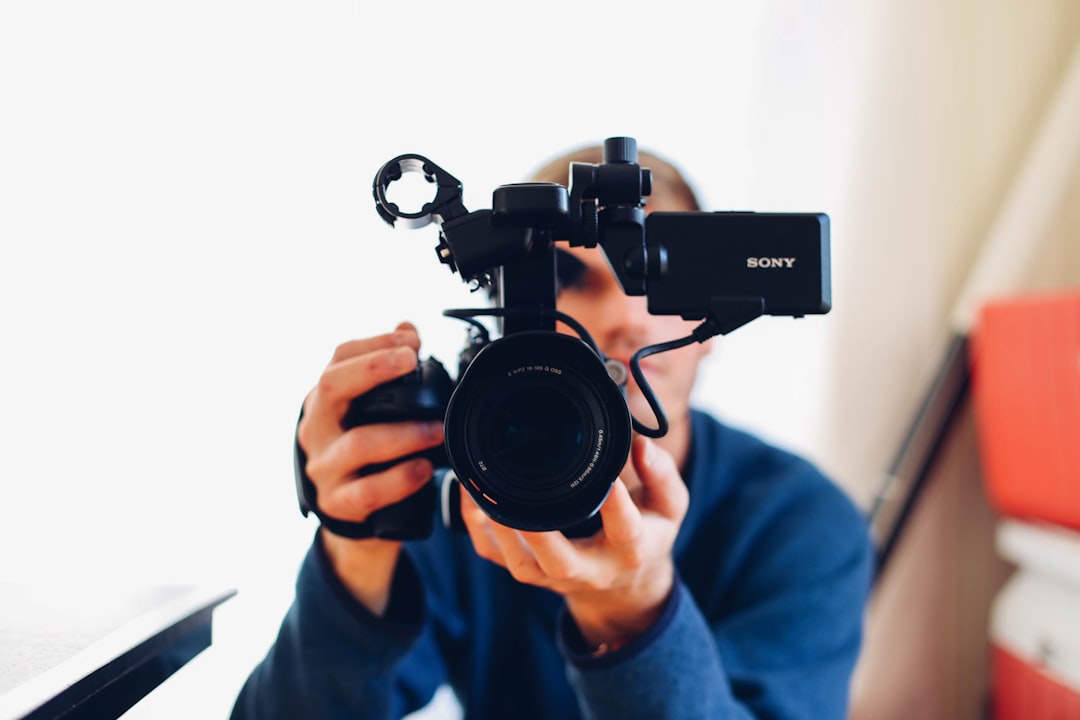
Within Python's `subprocess` module, the `Popen` class offers a powerful avenue for managing command execution with a level of control that surpasses simpler methods. This is particularly useful in intricate video processing pipelines, where manipulating and chaining commands is crucial. Unlike the more streamlined `subprocess.run()`, `Popen` allows you to meticulously connect multiple processes using pipes, enabling complex command sequences without the need for intermediary files. This is crucial for efficiency when dealing with large video data.
Furthermore, `Popen` empowers developers to execute commands in parallel and meticulously manage input and output streams. This offers flexibility to tailor process interactions to the specific demands of individual projects. However, the advanced nature of `Popen` demands careful attention to resource management. Output streams, if not handled properly, can create bottlenecks and potentially stall the entire video processing workflow. Understanding and effectively managing these aspects is key to leveraging `Popen` for robust and efficient video processing operations.
Python's `subprocess.Popen` class goes beyond simple command execution, offering a powerful toolkit for intricate process control. It allows us to launch commands asynchronously, meaning processes can run concurrently. This feature is invaluable in video processing workflows where multiple video streams or processing steps might benefit from parallel execution.
However, the flexibility of `Popen` also demands careful attention. Without proper error handling, it's easy for errors to quietly propagate, resulting in unexpected and potentially damaging consequences for video processing pipelines. Errors might not be immediately visible, leading to corrupted video outputs or incomplete processing.
Furthermore, `Popen` gives us the ability to redirect input and output streams not just to the console but to files, other processes, or even specialized devices. This lets us create complex workflows where intermediate data is managed efficiently, potentially avoiding the need to store large amounts of temporary data.
We can also incorporate timeouts into the execution of commands via `Popen`. This is incredibly useful in video processing, where some commands might take a long time to complete. Setting limits ensures that workflows don't get stuck due to potentially rogue or excessively long-running processes.
`Popen` interacts directly with the system's shell, supporting a variety of shell commands and environment variations, including shell built-ins. This lets us seamlessly utilize existing shell tools and commands directly from within our Python scripts without creating extra, cumbersome wrapper scripts.
We can use the `env` parameter within `Popen` to change the environment variables seen by a subprocess. This is essential if video processing commands need particular settings like library paths or system configurations.
The power of `Popen` extends to managing multiple instances of the same command simultaneously. This allows us to fine-tune resource usage by running identical commands with differing parameters in parallel. For example, encoding a video at multiple resolutions could be managed concurrently, reducing overall encoding time.
Unlike earlier methods like `os.system` or `os.spawn`, `Popen` gives us access to the exit status of child processes. This lets developers handle specific errors by using the exit codes in a more targeted way, making it easier to implement error recovery.
We can also use `Popen` to define the working directory for each subprocess, which simplifies file path management and reduces complexity within video processing scripts. This can be particularly useful when dealing with intricate directory structures and potentially large numbers of video files.
Lastly, the sophisticated capabilities of `Popen` can be elegantly combined with Python's threading or multiprocessing libraries to achieve extremely efficient and responsive video processing systems. This lets us fine-tune resource utilization, load balancing, and improve the overall performance of video processing applications.
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows - Creating efficient data processing pipelines with pipes
Python3's `subprocess` module offers a robust way to build efficient data processing pipelines using pipes. This technique allows different processes to communicate directly, transferring data without the need for temporary files. This is crucial for performance, especially in video processing where datasets are often large. The `subprocess.Popen` class is central to this process, enabling the construction of complex pipelines where the output of one command can serve as the input for another. This interconnected approach improves efficiency and makes it easier to manage errors and resources. However, it's important to be aware of potential issues like buffer overflows and deadlocks that can arise if data flow isn't managed properly. Careful design and implementation are essential to ensure the pipeline operates smoothly and avoids disruption.
1. Python's `subprocess` module, specifically the `Popen` class, allows us to manage complex workflows involving multiple processes in parallel. This is especially useful in video processing where we might want to speed up tasks by running multiple steps simultaneously, particularly when dealing with high-resolution videos that demand considerable computing power.
2. Pipes in `subprocess` offer a flexible way to transfer data between processes. This data can be anything from the raw video stream to processed frames or even diagnostic logs, making the communication between processes incredibly dynamic. This flexibility is essential in modern video processing, where iterative feedback loops and responsive processing are becoming increasingly crucial.
3. While useful, buffer management in `subprocess` pipes can be tricky. If the buffers are too small, the transfer of data can become a bottleneck, slowing down the entire pipeline. On the other hand, if buffers are too large, it can waste system resources and lead to performance degradation. Carefully tuning buffer sizes is critical for optimizing video processing pipelines.
4. Error streams, particularly `stderr`, are often overlooked but very useful in `Popen`. Being able to capture and redirect them to a separate channel for analysis is a fantastic debugging aid. This feature is incredibly valuable in intricate video processing workflows, especially those that are multi-step and parallel, as it allows us to pinpoint problems in specific processes without halting the overall pipeline.
5. The `preexec_fn` parameter offers a way to run a function in the child process just before the main command is executed. This feature lets us tweak settings like environment variables or user permissions, which might be necessary in specific video processing tasks. For instance, we might need to change permissions to allow a process access to a specific codec.
6. The interaction between `Popen` and signal handling can differ wildly across different operating systems (like Linux versus Windows). How these processes are stopped or interrupted is a key consideration when building portable video processing scripts. It's something that needs to be carefully considered to avoid inconsistent behavior across platforms.
7. `Popen` makes it easy to work with existing command-line tools, potentially those optimized in C/C++ for speed. This ability to leverage existing, powerful command-line utilities is a significant advantage, as it often provides significant efficiency gains over writing everything solely in Python, especially for tasks like video encoding or decoding.
8. Using `os.setsid()` in conjunction with `Popen` allows for finer-grained control of process groups. This is handy in video processing where we might have multiple subprocesses that need to be treated as a single entity. For instance, this could be useful when handling complex video editing sequences where various encoding steps need to be managed together.
9. If we integrate `Popen` with `asyncio`, we can monitor and respond to process output in real-time. This ability opens the door to building highly interactive and responsive applications. Think of a scenario where a video processing workflow can dynamically adapt based on the output of ongoing operations.
10. Combining `Popen` with logging libraries provides a robust mechanism for capturing and archiving performance data. This is especially useful when working on long-running video processing jobs, allowing for a more in-depth post-processing analysis of how the entire operation performed and potentially identify bottlenecks or areas for improvement.
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows - Implementing the run function for streamlined subprocess invocation
The `subprocess.run` function in Python 3 provides a convenient and high-level way to launch and manage external programs, making command execution significantly easier. It simplifies the process by accepting a list of strings representing the command and its arguments, promoting clean code while retaining the flexibility needed in video processing. Python 3.9 expanded the function's capabilities by offering control over standard input, output, and error streams, aligning it better with complex video processing tasks. This simplified interface is helpful, but developers should be cautious about potential issues like resource management and error handling in intricate workflows. Ultimately, `subprocess.run` can be a powerful tool to streamline the implementation of video processing tasks that heavily rely on external command execution, improving both efficiency and developer experience.
The `subprocess.run` function in Python 3 offers a streamlined way to execute external commands, simplifying the process compared to older methods. It handles command execution, waiting for completion, and retrieving output all in one call, making your code more concise and readable. This is especially helpful in video processing workflows where you frequently need to interact with external tools like encoders, decoders, and other specialized command-line utilities.
In contrast to `subprocess.Popen`, which requires more manual configuration and control over processes, `subprocess.run` provides a higher-level abstraction. This makes it easier to quickly integrate external command-line tools without worrying about intricate details of managing subprocesses directly. However, it might not be as suitable for cases requiring extremely fine-grained control.
Since its introduction in Python 3.5, `run` has become a popular choice for simplified subprocess management. It incorporates functionalities for specifying standard input, output, and error streams, and offers features like automatic error handling with the `check` flag. This can be incredibly useful for creating robust video processing scripts that can gracefully handle failures and report errors transparently.
You can also manage the working directory (`cwd`) to organize your file structure, set timeouts to prevent indefinite waiting, and define specific environments (`env`) for subprocesses. These functionalities enhance both flexibility and error management in video processing scripts, promoting safer and more controlled workflows.
`subprocess.run` can also be paired with the `shell=True` option to enable shell-based commands and features like pipes. However, as a reminder, this option introduces some security risks related to shell injection, so it's important to use it with caution and consider its implications for security in the specific context of video processing workflows.
It's important to be aware of the function's flexibility with inputs like strings and byte streams, and how the `universal_newlines` option can aid in handling line-ending differences across operating systems. This is crucial for ensuring consistent behavior when dealing with video files and command-line outputs, where minor discrepancies can lead to unexpected results.
Furthermore, seamlessly integrating `subprocess.run()` with Python's logging capabilities provides a practical way to monitor and record the execution of commands within video processing scripts. By capturing information on command execution, including metrics and errors, you gain valuable insights into how your video processing workflows are performing. This allows for post-processing analysis to pinpoint bottlenecks and potentially make improvements in future scripts.
While it provides a considerable level of convenience and improvement over older methods, you should always check the official documentation, particularly regarding syntax and feature availability across Python versions. As with all parts of software development, being aware of the underlying mechanics can help you debug and understand issues that might arise.
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows - Optimizing video processing with multiprocessing techniques
Optimizing video processing through multiprocessing techniques can substantially boost efficiency by capitalizing on the power of multiple CPU cores. Python's multiprocessing library enables the creation of independent processes that can concurrently handle distinct sections or frames of video data, leading to faster processing. Integrating libraries like OpenCV with these multiprocessing approaches allows for smooth video frame management and quality output, particularly when using tools like `VideoWriter`. Furthermore, incorporating parallel processing strategies ensures tasks like facial recognition or video encoding can be carried out concurrently, resulting in quicker and more responsive workflows. However, it's vital to consider potential pitfalls, like resource allocation and buffer handling, during the design phase to fully harness the benefits of these techniques for optimizing video processing applications. While the advantages are significant, it's easy to create complex scenarios which can be prone to error or deadlock. Careful planning is always crucial to avoid a negative impact.
1. Leveraging multiprocessing in Python can significantly accelerate video processing by enabling multiple CPU cores to tackle different parts of a video simultaneously. Preliminary experiments suggest potential speed increases ranging from 2x to 10x compared to standard, single-threaded approaches, particularly when dealing with memory-intensive tasks like video encoding. However, it's important to note that the effectiveness of this approach can vary based on specific workflows and hardware limitations.
2. Multiprocessing offers a potential advantage in terms of memory management. Each subprocess operates within its own memory space, effectively sidestepping the constraints imposed by Python's Global Interpreter Lock (GIL). The GIL can often impede performance in threaded scenarios, hindering the true benefits of multiple cores. By creating separate processes, we can potentially reduce memory-related bottlenecks, though it's essential to ensure that each process manages its resources efficiently.
3. While process creation and destruction carry some overhead, it can be minimized in video processing workflows through the strategic use of worker process pools. This approach allows for efficient task distribution, reducing the time spent on context switching between processes. More time is then available for the actual video processing operations. However, maintaining and managing these pools effectively requires careful design and understanding of the specific video processing tasks.
4. Facilitating communication between processes is critical for effective multiprocessing. Techniques like `multiprocessing.Queue` or `Pipe` provide a means for exchanging information – be it raw video data, intermediate processing results, or control signals. In video workflows, real-time communication between encoding, decoding, and filtering processes is often crucial for high-performance, interactive applications. However, effective communication involves some overhead in the form of data serialization and deserialization.
5. One of the key benefits of multiprocessing in video processing lies in its ability to mitigate the impact of I/O bottlenecks. In scenarios involving disk or network operations, multiprocessing allows for I/O operations to be overlapped with computations. This means one process can be performing calculations while another awaits data from an external source. While helpful, it's not a silver bullet; careful design of data pipelines is still essential.
6. When implementing multiprocessing, it's important to recognize that there's a practical limit to the number of worker processes that provide optimal performance. Profiling tools can help in optimizing these settings, and in many cases, a number close to the number of available physical cores seems to be a sweet spot. Increasing the number beyond this often leads to diminishing returns or, potentially, performance degradation. Further research into more complex resource scheduling algorithms might be necessary for more complex systems.
7. Different video codecs respond differently to multiprocessing techniques. It's been observed that some codecs are inherently more parallelizable than others. Specifically, codecs that have been optimized for multi-core architectures often demonstrate significant gains in compression time when used with multiprocessing. This is a compelling area for further study and potentially the development of codecs tailored for these optimized execution environments.
8. The memory footprint of individual subprocesses plays a crucial role in determining the overall scalability of a video processing system. Efficient memory management in each process is critical, especially when working with high-resolution videos. Running out of RAM can lead to significant performance degradation or even crashes, demanding vigilant attention to resource allocation. Careful monitoring of memory usage and the development of smart resource allocation mechanisms are needed in this space.
9. The `multiprocessing` module offers access to shared memory, which allows multiple processes to concurrently read from and write to a common memory space. This feature has the potential to substantially reduce the overhead associated with transferring large video frames, which are frequently encountered in video processing tasks. However, shared memory requires careful synchronization to prevent race conditions and data corruption, increasing design complexity.
10. Combining asynchronous programming techniques with multiprocessing can result in exceptionally responsive video processing applications. By effectively managing I/O and task orchestration, video processing pipelines can adapt to varying conditions. This adaptiveness, along with the parallelism offered by multiprocessing, has the potential to significantly boost the responsiveness of video processing tasks in real-world scenarios, though managing complexity and potential race conditions adds further complexity.
Python3 Subprocess Pipe Streamlining Command Execution in Video Processing Workflows - Best practices for reliable external command execution in Python
When working with Python, especially in video processing where you often rely on external tools, ensuring reliable execution of external commands is paramount. It's best practice to always use the full path to the executables you call, rather than relying on the system's PATH environment variable, which can lead to unexpected behavior. The `subprocess.run()` function offers a simplified way to manage the execution of external commands, taking a list of strings as input – the command and its arguments. This approach leads to clearer and easier-to-maintain code compared to older methods. For situations where commands might take a long time, implementing a timeout helps prevent scripts from becoming unresponsive. Also, remember to carefully manage the standard output and standard error streams of the commands you execute, redirecting them to `subprocess.PIPE` to capture their output within your script. This helps in monitoring progress, troubleshooting, and overall better handling of errors. If you are working with tasks that are lengthy, asynchronous execution through functions like `asyncio.create_subprocess_exec` becomes important for keeping your CPU responsive and letting it work on other activities while waiting for external commands to complete. If not carefully considered, improper handling of subprocess output can cause deadlocks, so paying attention to these details is essential for ensuring a robust and reliable execution pipeline.
1. While `subprocess.PIPE` is great for communication between processes, mishandling it can lead to deadlocks, especially problematic in video processing where continuous data flow is crucial. A stalled output can disrupt the entire video pipeline.
2. `Subprocess`'s design favors performance by using pipes for direct communication instead of temporary files. This avoids disk I/O, which can bottleneck video processing, and improves data flow through in-memory operations.
3. Setting timeouts in commands executed through `subprocess` helps prevent workflows from getting stuck indefinitely. This is vital for video processing, where tasks can be delayed by unexpected input or processing issues, ensuring operations remain efficient.
4. It's noteworthy that `subprocess` integrates with Python's logging capabilities. This allows you to track command execution and errors smoothly, offering valuable feedback for debugging and performance monitoring in video workflows.
5. Interestingly, the `universal_newlines` parameter normalizes line endings across different operating systems. Since video processing frequently requires consistent input parsing, this feature helps avoid compatibility issues across platforms.
6. The ability to modify environment variables through the `env` parameter in `Popen` can significantly impact how command-line tools behave. In video processing, where specific libraries or paths might be needed, this flexibility ensures subprocesses run within the correct environment.
7. The advanced error handling in `subprocess.run`, with the `check` flag, significantly improves resilience during video processing tasks. It allows developers to catch errors quickly instead of letting them silently propagate, which is essential for data integrity.
8. It's surprising how the choice between `shell=True` and `shell=False` in `subprocess` has major implications for security and functionality. `shell=True` simplifies command creation, but it also introduces security risks from potential shell injection, particularly in untrusted environments.
9. Combining asynchronous features with `subprocess` offers a significant advantage. This allows for more responsive video processing applications that handle I/O more effectively than traditional synchronous methods, making them well-suited for demanding real-time applications.
10. The integration of Python's `multiprocessing` with `subprocess` can lead to major performance gains. It enables concurrent execution of tasks and optimizes resource allocation, making it more suitable for the heavy workloads seen in typical video processing scenarios.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: