Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Missing OpenCV Video Frame Symbol Resolution In x86 Libraries
When working with video processing on x64 systems, developers often encounter difficulties stemming from "Missing OpenCV Video Frame Symbol Resolution" within x86 libraries. This usually presents as an "Undefined symbols for architecture x86_64" error message. The root cause often lies in using 32-bit OpenCV libraries within a 64-bit system. This mismatch between library and system architecture creates a crucial need to ensure project settings are correctly aligned with the target environment.
Furthermore, the connection between the build process and library linkage is pivotal. Without proper linking, the compiler might fail to locate essential components. This is particularly true when using OpenCV libraries or functions related to video capture. We need to ensure that all necessary libraries are available and correctly referenced during the build procedure to prevent the 'Undefined symbol' error.
And it's not just architecture. The versions of OpenCV and Python can also play a part in causing these symbol resolution issues. The version of each tool must match. If, for instance, Python and OpenCV are mismatched, issues like frame capturing may fail, due to inconsistent expectations on how libraries interact between them. Keeping careful track of all tool versions becomes paramount to avoid these problems.
Debugging can be significantly aided by using visual debugging tools, allowing developers to gain a better understanding of the flow of the code and the relationships between the involved libraries and functions, ultimately helping them pin down exactly where the missing symbol resides and what steps are required to ensure successful linking.
1. The "Missing OpenCV Video Frame Symbol" problem in x86 libraries often arises from inconsistencies in the library versions used. This can create headaches during the linking phase, particularly when different parts of your application depend on different versions of OpenCV. It's a common issue that highlights the need for meticulous dependency management.
2. When building your OpenCV projects, using incorrect architecture flags during compilation can create misleading debug information. This can make it surprisingly difficult to pinpoint missing symbols, which is especially problematic in video processing applications where even small errors can lead to unexpected behavior.
3. Sometimes, symbol resolution failures can be attributed to corrupted or incomplete library files. This underscores the importance of thoroughly checking the integrity of your OpenCV dependencies before running any video processing code. You don't want to waste time troubleshooting when a simple file check might reveal the issue.
4. In dynamically linked environments, the order in which libraries are loaded can have a significant effect on symbol resolution. If a needed library is loaded before the symbols it requires are available, it will lead to a “missing symbol” error. This is especially true in more complex projects with intertwined dependencies.
5. Using different compilers, or even different versions of the same compiler, can cause discrepancies in how the final executable is built. This can manifest as "mangled" symbol names, especially in C++ libraries like OpenCV. So if you're doing cross-compilation, keep this potential problem in mind.
6. Some debugging tools are capable of automatically resolving missing symbols by linking to the correct libraries. However, many developers are unaware of these tools, leading to a potentially frustrating and drawn-out debugging process. It might be a good idea to explore some of these tools to see if they can streamline your workflow.
7. CMake configurations play a critical role in making sure your compilation process is successful. If not configured correctly, you'll end up with unresolved symbols in x86 architectures. Pay attention to the linking flags and dependencies within your CMake files to prevent this kind of error from happening in the first place.
8. OpenCV supports different backends for video capture and processing. If you're using an unsupported or mismatched backend, this could potentially be the cause of your missing symbols. It's something to watch out for, especially if you're working in a multi-backend environment.
9. It's important to consider ABI (Application Binary Interface) compatibility, especially in large projects. Even if your libraries are linked correctly, incompatible ABIs can lead to unexpected symbol resolution problems. This is something that can easily be overlooked when integrating libraries from different sources.
10. How C++ functions are declared as visible or hidden can cause problems with unresolved symbols if not handled correctly. Using the appropriate export mechanisms, particularly when building shared libraries, can help to avoid these types of issues in OpenCV video processing applications.
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Global Variable Declaration Conflicts Between C and C++ Code Bases
When integrating C and C++ code, conflicts related to global variable declarations can arise, especially during the linking phase of compilation. This is a common problem that stems from the differences in how each language handles global variables. A key issue is that the linker might encounter multiple definitions of the same global variable, a violation of both C and C++ standards. This can happen if a variable is declared in multiple source files without proper care being taken. The problem is further complicated when integrating older code bases as there can be naming inconsistencies across different modules or libraries. While the use of global variables does simplify sharing data between different parts of a program, this benefit must be carefully weighed against their impact on code clarity and maintenance. Tracking modifications becomes more difficult when global variables are used frequently. Failing to properly manage these declarations across the code base is a significant source of headaches when dealing with symbol resolution errors.
1. **Name Clashes in Hybrid Code**: When combining C and C++ code, global variables can cause problems because C doesn't have name mangling like C++. This means if you use the same name for a global variable in both, the linker might get confused, leading to hard-to-find errors during runtime.
2. **Linking Subtleties**: Usually, C++ uses "extern C" to link with C code, helping prevent symbol conflicts. But if developers forget to use it correctly for all the necessary functions, you'll see "unresolved reference" errors when connecting C and C++ parts of your program.
3. **Initialization Sequence**: The order in which global variables are initialized can be quite different between C and C++. This can create situations where a variable in one part of the code isn't ready when another part tries to access it, causing unpredictable behavior, especially in video processing where timing matters a lot.
4. **Static vs. Global**: By default, C++ makes variables declared with `static` only accessible within their file. In C, though, the default is external linkage, meaning they're available everywhere. A misunderstanding of this difference can lead to unexpected limitations on how variables are shared across your project.
5. **Implicit vs. Explicit**: C allows functions to be used without being explicitly declared, but C++ requires declarations. This difference can cause hidden errors if you use a global variable in C without declaring it—it compiles, but when you switch to C++, it'll cause a compiler error, highlighting the importance of consistently declaring variables across different code styles.
6. **Header File Issues**: Using C header files in C++ can be confusing due to different rules about linkage and visibility. This can lead to multiple definitions of the same variable or unexpected variable visibility, making debugging a challenge.
7. **Compiler Settings**: Different compiler options for C and C++ can change how global variables are treated. For instance, aggressive optimization might inline functions or remove what the compiler thinks are unused global variables. This can cause strange symbol resolution issues that are challenging to understand.
8. **Compile-Time Constants**: The `constexpr` keyword in C++ lets you create compile-time constants, which isn't available in C. Not understanding this difference during compilation can cause discrepancies when accessing global states in a program, particularly when doing parallel processing for video which requires precise control.
9. **Namespaces**: Using namespaces in C++ can help avoid global variable clashes, but if not done properly, it can make calling C++ code from C harder. It's a matter of careful attention to how C++ code is exposed to the rest of the project.
10. **Debugging Tool Limitations**: Certain debugging tools might not provide much help when you're dealing with conflicts between C and C++ global variables because they're often geared towards C++ features. Developers might need to adopt more advanced debugging techniques to fully understand how these conflicts affect the code.
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Undefined FFMPEG Reference Causing Static Linking Problems
Static linking with FFmpeg can lead to frustrating "undefined reference" errors during the compilation process. These errors often arise when libraries are linked in the wrong order. This means a library might be referenced before the required symbols are available within the executable. Adding to this problem is the GNU linker's habit of removing unused symbols, which can inadvertently lead to undefined references if the link order is not carefully controlled. Proper use of linker flags is paramount: `-L` to set the library search path and `-l` to indicate the libraries to be linked. Further, it's essential to manage the linker's search path to prevent clashes with other libraries and ensure that all necessary FFmpeg dependencies are found correctly during the linking phase. Issues related to conflicting libraries can further complicate the process, necessitating diligent care in how they are integrated into the project. In essence, mastering the linkage process is essential to avoid symbol resolution failures and ensure a successful build of any FFmpeg project.
1. When using FFmpeg with static linking, you might run into "undefined reference" errors because of mismatched library versions. This is common when you mix different FFmpeg builds, causing incompatibility issues that surface during the linking phase, making debugging trickier.
2. The Application Binary Interface (ABI) is super important for how the linker connects to FFmpeg symbols. If your libraries were compiled with different ABIs, it can lead to those "missing symbol" errors, reminding us how important it is to keep your compilation settings consistent across your whole project.
3. Static linking problems can be quite different depending on the operating system you're working with. You might get it to work on Linux but see those unresolved references on Windows, simply due to differences in how binaries are formatted and how linking works. It makes cross-platform video processing a bit more of a challenge.
4. When you're linking to a bunch of libraries, the order you list them in matters for how those symbols are resolved. Some symbols won't be found if their dependencies aren't loaded in the right order. It's a strange thing that often complicates integrating FFmpeg into a project.
5. The way C and C++ handle names (name mangling) can also lead to unresolved symbols when using FFmpeg features. It can cause confusion for the linker, especially when FFmpeg exposes C++ APIs that might have the same names as C declarations.
6. If you build FFmpeg with certain compile-time flags enabled or disabled, it can change the symbols that are available. This can make it look like there are undefined references if your code relies on features that weren't included in the library build.
7. Many systems have multiple FFmpeg installations, and you can accidentally link against the wrong version without realizing it. This leads to unresolved symbols, which are annoying to track down, especially if you haven't set your library path correctly.
8. If your CMake configuration for FFmpeg is incorrect or missing pieces, you'll likely get static linking errors. You have to be careful to specify the correct paths and flags to avoid "undefined reference" issues because CMake doesn't magically do the right linking for you.
9. A lot of developers don't think about using dependency resolution tools, which could make linking FFmpeg much simpler. These tools can automatically resolve symbols and dependencies, but they're often overlooked, leading to people spending way too much time debugging these kinds of issues.
10. When you're doing static builds of programs that use FFmpeg, it's important to test them across different environments. Sometimes those integration issues won't show up until you run the executable in a different context, so you really need to test thoroughly.
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Memory Alignment Issues With ARM64 Video Buffer Processing
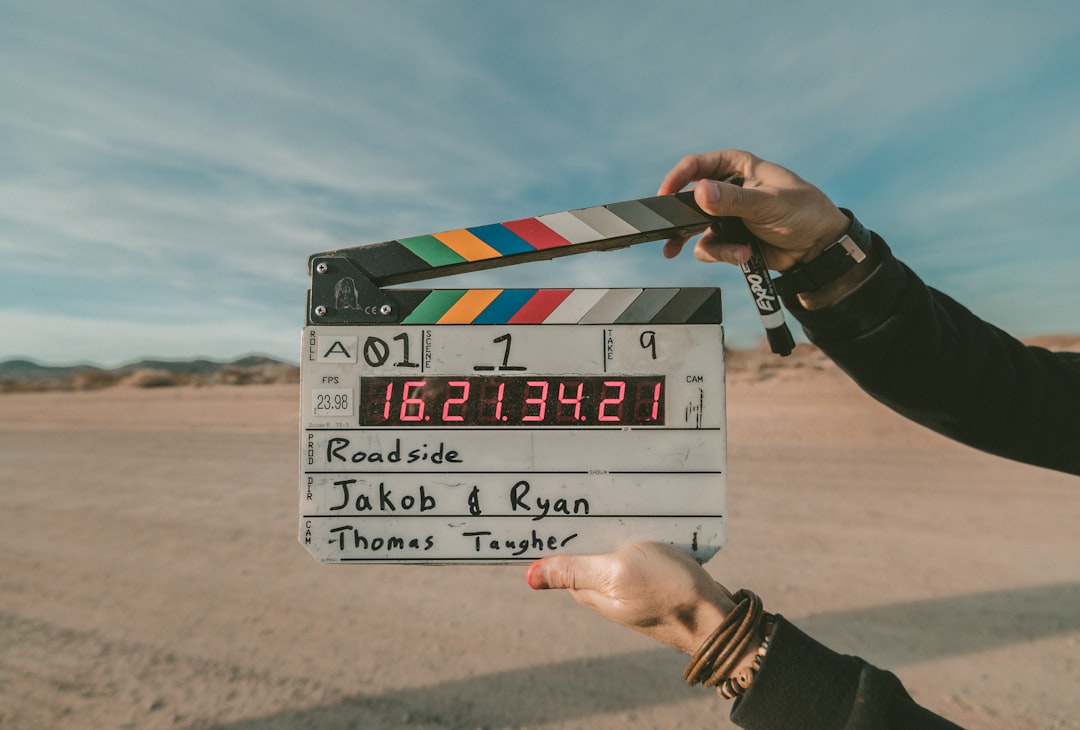
When processing video on ARM64 processors, memory alignment can be a major source of problems, especially when dealing with instructions that work with double words. These instructions need the memory address to be aligned correctly, or they trigger an alignment exception. When this happens, the kernel often steps in and handles the memory access, which can cause a big performance hit. The way to avoid this usually involves using alignment directives in your code (like `balign`) to ensure your memory structures are laid out correctly. Debugging tools like UBSAN can help find these problems and let you fix them by restructuring your data or using things like `memcpy` to copy data around in a way that satisfies alignment needs. It's really important to remember that the ARM64 architecture has strict alignment rules, and if you don't follow them, it can cause your video processing to run slower or even crash. Designing your code with memory alignment in mind is crucial for getting the best possible performance from ARM64.
### Memory Alignment Issues With ARM64 Video Buffer Processing
1. ARM64 processors are quite particular about how data is accessed in memory. They expect specific data types to be located at certain addresses, like 4-byte integers needing to start at an address that's a multiple of 4. If you don't follow these rules, you might get some unexpected performance issues or even crashes, especially when you're handling large amounts of video data.
2. The way your processor manages its cache can be affected by misaligned memory accesses. If you try to access data that isn't aligned correctly, it might have to load more data from memory than it needs, which can add a lot of extra time to the process. This becomes a problem in video processing, where you need things to happen quickly to avoid choppy playback.
3. If your code isn't carefully written to avoid unaligned memory access, it can end up overwhelming the processor's memory bus. Imagine a crowded highway where everyone's trying to get on at the same time. This leads to slower processing, which can translate to dropped frames or lag in your video.
4. Often, developers have to work around memory alignment issues by copying data to different parts of memory before they can process it. This creates extra steps that take up processing power. For high-speed video, this added work can really slow things down and prevent your app from processing in real-time.
5. How the compiler creates instructions for your program can also be influenced by alignment assumptions. If your data isn't properly aligned, the compiler might not be able to generate the most efficient code, leading to slower performance. This is something video processing developers need to be aware of to ensure their programs are as optimized as possible.
6. Unfortunately, the way ARM64 processors handle misaligned memory access isn't always consistent. Some devices might generate an error, and others might just silently deal with it, leading to some unpredictable behaviors. This inconsistency makes thorough testing across different platforms essential for video processing.
7. ARM64 also offers special instructions for performing the same operations on multiple pieces of data at once, called SIMD instructions. However, these instructions are very sensitive to memory alignment. If your video buffers aren't set up correctly, you might not get the performance gains you expect, which is crucial for dealing with high-resolution videos.
8. Video data often needs to be shared between different parts of your program or different software systems. If you haven't kept alignment in mind when designing the data structures, it can cause unexpected problems or corruption of video frames. It makes integrating video processing libraries into a larger project more complicated.
9. It's often difficult to pinpoint the exact cause of problems with memory alignment, especially if the software doesn't generate errors. You might not know about it until runtime, and debugging these kinds of issues in video processing can be tricky, particularly for real-time applications that require quick feedback and adjustments.
10. Many common debugging tools might not provide the necessary information about memory alignment. It makes it difficult to see the impact of memory access patterns on performance. For complex video processing tasks, you might need to use more specialized tools or analyze performance metrics to fully understand the effects of misalignments.
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Compiler Level Mismatch Between GCC 9 and Legacy Code
When you integrate older code with newer GCC versions, like GCC 9, you might run into compatibility issues. This is because the way GCC 9 handles code might differ from older compilers, such as GCC 7. One common sign of this incompatibility is getting "undefined reference" errors during compilation. These happen when the linker can't find the necessary code parts between the old and new code. This highlights the importance of making sure your code is compatible between compiler versions. If not, you might end up with unresolved symbols and broken connections between parts of your application. To help prevent these problems, you might need to make changes to your legacy code to match how the new compiler works, making use of GCC's debugging features to track down issues, and being aware of how the compiler versions affect linking. Keeping track of these compiler changes is increasingly important in software development. It can help avoid nasty surprises when you're trying to update or improve old code.
### Compiler Level Mismatch Between GCC 9 and Legacy Code
1. GCC 9's changes to the Application Binary Interface (ABI) can create compatibility problems with code compiled using older GCC versions. This can manifest as "undefined reference" errors or other strange behavior when parts of the code were built with different GCC versions. It can be particularly problematic in projects using a mix of old and new code.
2. GCC 9's stricter adherence to the C++ standards can expose hidden issues in legacy code that previously worked under older compilers. This can mean compilation errors that weren't problems before, so updating to GCC 9 requires a careful look at the existing code.
3. The optimization methods used in GCC 9 can differ from earlier versions, leading to varying code behavior under different optimization levels. This difference can make debugging more difficult since code that worked before might not behave as expected under the new compiler's optimizations.
4. GCC 9's approach to template instantiation can produce duplicate symbols or missing template definitions, leading to linker problems. If your legacy code uses templates frequently, you might need to modify it to work correctly with the newer compiler.
5. Certain older features and functions may have been deprecated in GCC 9, resulting in warnings or errors not seen with older compilers. This necessitates checking the legacy code to ensure no deprecated functions are being used and update them as needed.
6. GCC 9 gives more warnings about undefined behavior than previous versions, potentially highlighting issues in legacy code that were previously ignored. While this can help find bugs, it can also be frustrating as older code might have accumulated many such issues.
7. The way GCC 9 handles name mangling in C++ is different than older versions, possibly resulting in unresolved symbols during linking with libraries compiled with an earlier GCC. It's important to pay close attention to compiler version consistency when working with libraries and external components.
8. GCC 9 improved thread safety support, which might reveal threading problems (race conditions, etc.) in legacy code that previously worked. This can require code refactoring to be compliant with modern threading practices.
9. GCC 9 has better static analysis features, which might uncover hidden bugs in legacy code. While this can improve code quality, it can also be a source of annoyance if the code has many issues that previously went unnoticed.
10. The process of linking libraries with GCC 9 can differ from older versions, especially if the libraries were built with those older versions. Compatibility needs to be carefully managed to prevent symbol resolution errors when linking.
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Double Symbol Definition From Circular Dependencies in Video Filters
Circular dependencies within video filters can introduce a significant hurdle in the development process, often leading to the frustrating problem of double symbol definitions. This typically arises when the same symbol is defined in multiple parts of your code, which can cause issues during the linker stage. You might encounter error messages like "undefined reference," indicating the linker couldn't locate a symbol that was declared but not properly defined. To mitigate these issues, careful attention should be paid to how source files are managed during the compilation process. It's crucial that all relevant files are explicitly linked together to prevent these types of conflicts.
The situation becomes even more complex when working with dynamic libraries. Dynamic libraries introduce their own unique considerations for symbol management, often creating discrepancies when compared to linking individual object files directly into a single executable. Also, without the necessary debugging information (or if the debugging sections are incomplete), linker errors can become more obscure, making it harder to understand what's going wrong. It's vital for developers to understand how to avoid creating circular dependencies in their video filter designs and to be mindful of the complexities of dynamic linking to ensure cleaner builds and smoother program execution. Simply put, being aware of the potential for these issues and using thoughtful code organization can help streamline the process of developing stable video processing applications.
### Double Symbol Definition From Circular Dependencies in Video Filters
1. Circular dependencies can create a complex situation where the same symbol is defined in multiple places, often stemming from interconnected modules that rely on each other. This can lead to the linker getting confused about how to resolve symbols, especially when dealing with the many components found in video processing systems. It's a fairly common problem and requires careful consideration of module design to avoid.
2. Understanding how the linker handles symbols is crucial. In cases of circular dependencies, the linker might not always realize a symbol is truly "missing". Instead, the linker may hit a loop while trying to resolve the dependencies, before all required symbols are fully set in stone, which can lead to an undefined symbol error. This illustrates the intricacies of the linking process.
3. Using namespaces can offer a level of protection against these double symbol definition problems. By grouping related symbols, you help the linker understand the scope of each symbol better. This helps to avoid ambiguity and prevent the linker from mistakenly trying to use the wrong definition of a symbol in a circular dependency. It's a useful approach when you're dealing with a lot of code and potential conflict points.
4. Whether you link your libraries statically or dynamically impacts how circular dependencies are treated. When statically linking, you tend to uncover these issues during compilation. With dynamic linking, they can hide until runtime, making them harder to find and fix when the app is already running. This means your debugging strategy needs to be aware of how your application is being linked.
5. Modern compilers, especially newer versions, are often better at spotting the potential for circular dependencies. If you ignore those warnings, it's likely you'll run into the double symbol definition problem later when things get complicated—particularly when linking larger systems that need to assemble many smaller pieces together. This underlines the importance of taking warnings seriously, especially in cases like this.
6. The sequence of header file inclusion in your code can have a big effect on whether or not you encounter double definitions. If the code that actually defines a symbol is included after the code that uses it, the linker might throw an error as it's trying to resolve the symbol during compilation. This order of inclusion needs to be understood and correctly controlled for optimal compilation.
7. When working with C++ templates, there's a risk of unintentionally creating circular dependencies if not managed carefully, especially within video processing libraries. This is because templates are frequently used to optimize performance, making it important to be aware of this potential for creating issues when using them.
8. The choice of your build system can significantly influence how it handles these circular dependencies. Some build systems, such as Makefiles, might require you to write specific commands or use flags to manage those dependencies. On the other hand, tools like CMake can sometimes detect and manage them for you. Choosing the right build system is key to smooth compilation and to reducing the risks associated with symbol definition issues. It's worth understanding what your system does or doesn't provide for dependency management.
9. When a linker encounters a duplicate symbol, it needs to make decisions during runtime. This can potentially lead to performance slowdowns in your video processing software because the execution needs to go through extra steps to decide which definition to use. This can add latency to your processing, which can be a major drawback for applications where timely processing of video is essential. It's another reason to try to avoid these problems.
10. Testing should incorporate checks for these types of problems. You can do this by setting up continuous integration (CI) so that your system automatically performs checks for duplicate symbols. Doing this early in the development process helps avoid unexpected errors in complex systems where resolving symbol issues becomes a substantial effort. This testing can highlight problems before they become major roadblocks.
Debugging LD Exit Status 1 7 Common Symbol Resolution Failures in Video Processing Code - Missing Export Table Entry For Hardware Accelerated Functions
When dealing with video processing code, you might encounter the error "Missing Export Table Entry for Hardware Accelerated Functions." This error usually surfaces during linking, and it signals that the linker can't find the specific pieces of code needed to make hardware acceleration work. This could happen due to incorrect build setups or if crucial files (called shared objects) are missing.
It's important to get this right because hardware acceleration is crucial for processing videos smoothly. If these functions aren't linked correctly, you lose out on potential performance gains. Tracking down this type of error involves carefully checking the project's build settings and ensuring that all the required libraries are linked correctly. Developers need to make sure the build process includes all the necessary paths and settings to find the required symbols, and they should focus on understanding how linking parameters affect the final outcome. The success of hardware acceleration hinges on resolving these symbol errors during the compilation stage.
### Missing Export Table Entry for Hardware Accelerated Functions
1. The issue of missing export table entries for hardware-accelerated functions in video processing is often tied to how well the system is able to provide GPU resources. If there aren't enough resources, the features meant to speed up the process may not be available, which can cause these export table problems and break the intended flow of the code. This is an issue to be mindful of, especially if your video processing tasks are particularly demanding.
2. The way the graphics drivers interact with the system plays a significant role in whether these acceleration features are functional. A missing entry usually hints at a mismatch between what the driver can offer and what the application requires. This can lead to confusion as the system tries to figure out what's available to use. This mismatch creates issues with resource availability that we need to take into account.
3. If different libraries have varying requirements regarding how they exchange data (Application Binary Interface, or ABI), it can lead to missing entries. If they're built in ways that are incompatible, you might lose some functionalities. This is especially true with hardware acceleration where it's critical that the information exchanged is structured exactly correctly. It's a good idea to understand the implications of using libraries with different ABIs, especially in the context of video processing.
4. If we're targeting a specific hardware architecture (x86 or ARM, for example), differences in how export tables are set up across these different architectures can cause some problems. It might not always be obvious what's causing the problems, and the resulting issues could vary from just undefined behavior to outright missing functions. It's a good practice to make sure that any code we write is compatible across all relevant architectures, if needed, and this problem highlights the need to be thorough.
5. Interestingly, some developers don't emphasize the importance of writing detailed documentation about the hardware-accelerated functions. Libraries and frameworks are constantly evolving, and some functions may become outdated, or new ones may replace them. Without careful tracking of these changes in documentation, the issue of missing entries might pop up, creating headaches down the line. This underlines the value of proper documentation when managing hardware acceleration functions.
6. It's interesting how the sequence in which we link libraries during a build can affect whether the necessary functions are accessible. It seems that an incorrect sequence can lead to missing entries, generating errors that make troubleshooting even more challenging. In more complex video processing pipelines, understanding how the libraries relate to one another and the order they need to be linked can be quite important.
7. The choice of static or dynamic linking can have distinct effects on how visible the hardware-accelerated functions are. In static linking, all the code is included when the program is compiled, but dynamic linking involves loading libraries at runtime. There's a chance that missing entries might appear in dynamic linking if the loaded libraries don't match up with the executable's expectations. It's important to consider this potential behavior when choosing how libraries are linked to an executable.
8. It seems that the use of compiler flags can have an impact on the creation of export table entries. Inaccurate or inappropriate flags might block the creation of these necessary entries, which might be problematic when we really need hardware acceleration to improve performance. We should be cautious in our use of compiler flags, particularly with regard to hardware acceleration functions.
9. Environment settings can subtly affect whether the hardware-accelerated functions are available. For example, misconfigured paths could lead the program to the wrong library, which might lack the required export table entries. Environmental issues can cause a whole range of unforeseen difficulties, particularly within the context of video processing.
10. Cross-platform development adds another layer of complexity related to export tables. Different operating systems may not handle them the same way, so some missing entries might not appear until we try to run the program in a different environment. It's a good idea to make sure the code can function reliably across all the targeted platforms.
More Posts from whatsinmy.video: