Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Implement chunk-based processing for large video files
One of the most effective ways to handle large video files in Python is to implement chunk-based processing. This involves breaking down the video file into smaller, manageable chunks, which are then processed individually. This approach significantly reduces the risk of MemoryErrors, which often arise from attempting to load large files entirely into memory. The memory constraint issue isn't just about crashing the system, it's about hindering performance.
This method of processing chunks not only prevents MemoryErrors but also boosts efficiency. By strategically managing these chunks with libraries like `multiprocessing`, you can distribute the workload across multiple CPU cores, drastically improving the overall processing speed. Furthermore, by incorporating an input-output queue system, you can handle data management even more effectively. This system allows you to read chunks of the video file while simultaneously processing other chunks, leading to a smoother workflow and optimized processing time.
Let's dive into chunk-based processing for large video files in Python. As you probably know, trying to load a whole movie into memory can easily lead to a "MemoryError" – which isn't a fun experience. But, breaking down the video into smaller chunks allows us to process it more efficiently. The main advantage? We reduce the risk of running out of memory.
This is especially beneficial when dealing with high-resolution videos, which often require considerable processing power and storage space. Imagine the benefits of parallel processing! By distributing the workload across multiple CPU cores, we can achieve significant speed-ups, leading to much faster video analysis and rendering. Imagine if you could process video data in real-time. That's exactly what chunk-based processing allows us to do with streaming algorithms.
Now, let's talk about Python libraries. Libraries like Dask or PySpark make the chunk-based processing workflow a breeze. They offer distributed computing capabilities, allowing us to handle large files even more effectively.
The size of the chunks plays a crucial role in performance and resource usage. Larger chunks can lead to faster processing but can also cause memory spikes. Smaller chunks can help to minimize memory spikes, but they may also increase processing overhead because of frequent context switching.
Here's a cool Python trick: generators. Generators allow us to load data lazily. This means we only load chunks into memory as we need them, which is a huge win for memory efficiency. The best part? Chunk-based processing works nicely with different video codecs. It allows us to handle each chunk with the appropriate method based on the codec type, enhancing our ability to work with a variety of video formats.
We also need to think about error handling. If a chunk fails to process, we should be able to isolate and reprocess only that segment. This prevents errors from cascading and potentially shutting down the entire processing operation. Finally, the chunking approach has some neat implications for machine learning. Since we can process smaller segments, we can create smaller datasets for training our machine learning models. This allows for more targeted training on specific video features, potentially leading to better performance overall.
So, the next time you face a "MemoryError" while processing a video, remember – chunk-based processing is your best friend. It not only tackles memory issues, but also unlocks a whole new world of efficiency and flexibility.
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Utilize Python's garbage collection module to manage memory
Python's automatic memory management relies on a garbage collector that frees up memory used by objects no longer needed. This process works through two main mechanisms: reference counting and generational garbage collection. Reference counting essentially keeps track of how many references point to an object. When the count drops to zero, that object's memory is reclaimed. Generational garbage collection tackles circular references, a tricky situation where objects still exist even though there's no longer a direct path to them. It groups objects into generations based on their age and applies different collection strategies.
The `gc` module gives developers some control over garbage collection. They can trigger collection manually, tweak collection thresholds, or even disable it completely. While Python's built-in garbage collector usually does a good job, having some manual control can be helpful, especially when dealing with situations where memory usage is critical. However, it's important to note that excessive manual garbage collection could potentially degrade performance.
For video processing scripts, memory management is essential for preventing `MemoryError` crashes. Video files can be enormous and demanding on system resources. Understanding the fundamentals of Python's garbage collection, combined with judicious coding practices, helps ensure smooth video processing without unexpected crashes.
Python's garbage collection isn't a magical instant cleanup. It works on a reference counting system, meaning an object lingers in memory until it's completely abandoned. This can lead to memory bloat, especially in long-running tasks like video processing, which demand careful attention to memory usage.
The `gc` module lets you take charge of garbage collection with functions like `gc.collect()`, forcing a clean-up. This is especially handy after processing large data chunks, providing some relief in situations where memory is a precious resource.
But, watch out for circular references – they can trap memory. These are relationships where objects hold references to each other, forming a closed loop. This can prevent the garbage collector from doing its job, leading to memory leaks, especially in complex data structures. That's why identifying and breaking these cycles is critical in memory-hungry applications.
The `gc.get_objects()` function is a useful tool to understand what the garbage collector is keeping track of. It reveals a list of all the objects under its watchful eye, providing insights into memory usage patterns. This can be helpful for optimizing your code to prevent unnecessary memory consumption.
Sometimes, the garbage collector seems to hold onto objects longer than intended, especially during high-demand phases. This underlines the importance of memory profiling – it can help uncover unexpected references that may contribute to MemoryErrors.
The `gc.set_stats()` function allows tweaking the garbage collector's behavior, like setting thresholds for triggering collection. Adjusting these parameters might improve memory efficiency, particularly when dealing with temporary objects frequently generated during video processing.
The generational garbage collection system categorizes objects based on their age, with the youngest generation getting cleaned up more often. This is a smart move since most objects in video processing are short-lived, not requiring long-term memory retention.
The `gc` module even allows for a `DEBUG` mode. This turns on tracing of the garbage collection process, giving you a better understanding of what's going on. However, remember that this comes with a performance cost.
You can actually disable the garbage collector completely with `gc.disable()`, which allows for more direct memory management. But, exercise caution – this can be a slippery slope toward memory leaks unless you're incredibly confident in your handling.
Finally, be aware that introducing third-party libraries can potentially mess with the garbage collector. They might create hidden circular references or cause other inconsistencies that hinder memory cleanup. It's important to be watchful for memory leaks when integrating new dependencies in your video processing scripts.
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Profile memory usage with memory_profiler library
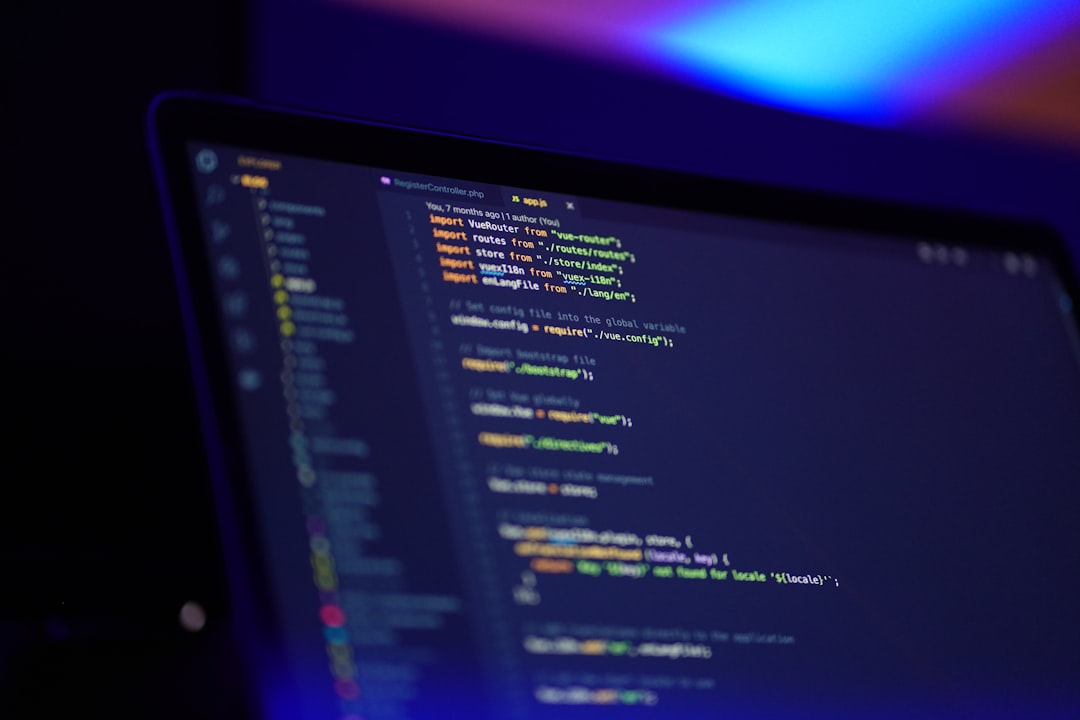
Profiling memory usage in Python scripts is crucial for understanding memory consumption, especially in demanding scenarios like video processing where `MemoryErrors` can disrupt workflow. The `memory_profiler` library empowers you to pinpoint memory-hungry code sections by annotating functions or methods with the `@profile` decorator, giving you line-by-line breakdown of memory allocation. Simply running a command like `python -m memory_profiler myscript.py` provides a detailed memory usage report, making it easier to identify and optimize areas that consume excessive memory. Using `memory_profiler` alongside Python's built-in garbage collection tools further refines memory management practices, enhancing performance and reliability for your video processing applications.
The `memory_profiler` library stands out because it delves into the nitty-gritty details of Python's memory usage at a line-by-line level, something standard profilers often miss. This granular view is invaluable for squeezing every bit of efficiency out of memory-heavy applications like video processing.
It's neat how `memory_profiler` can create graphs that visualize memory consumption over time. This lets you see how different parts of your code impact memory usage, which can guide you towards code refactoring.
One of the library's strengths is its ability to pinpoint the exact lines in your code that lead to the biggest memory spikes. This is huge for targeted optimizations because it tells you precisely where to focus your efforts. `memory_profiler` is flexible. You can use it to profile functions and entire scripts, meaning it can tackle a range of tasks from simple functions to massive video processing projects.
While `memory_profiler` excels at detailed memory analysis, there are a few caveats. The first is performance. The profiling process itself can slow things down, especially if you're dealing with performance-sensitive applications. It's a trade-off.
Also, `memory_profiler` is more concerned with memory allocated to objects than the short-lived memory usage by temporary objects. This means you have to be careful when profiling tasks that rely heavily on temporary objects.
Here’s a neat twist: `memory_profiler` works seamlessly with Jupyter notebooks, providing real-time insights into memory usage. This is a big plus for data scientists and engineers who work with interactive Python environments because it helps debug right in the flow of work.
The library can even profile multi-threaded applications, which is helpful when you've got separate threads handling different parts of video processing tasks. This allows you to understand memory consumption in a more complete way.
Some people have found that using `memory_profiler` with `numpy` reveals interesting insights about memory usage when working with arrays. This knowledge can be leveraged to optimize code for situations involving large datasets, which are common in video processing.
Finally, the most significant impact of `memory_profiler` is the knowledge it brings. By analyzing your code's memory usage with this tool, you can start to develop a stronger sense of memory constraints and build more efficient coding habits. And ultimately, this can help your team create better-performing applications.
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Optimize data structures to reduce memory footprint
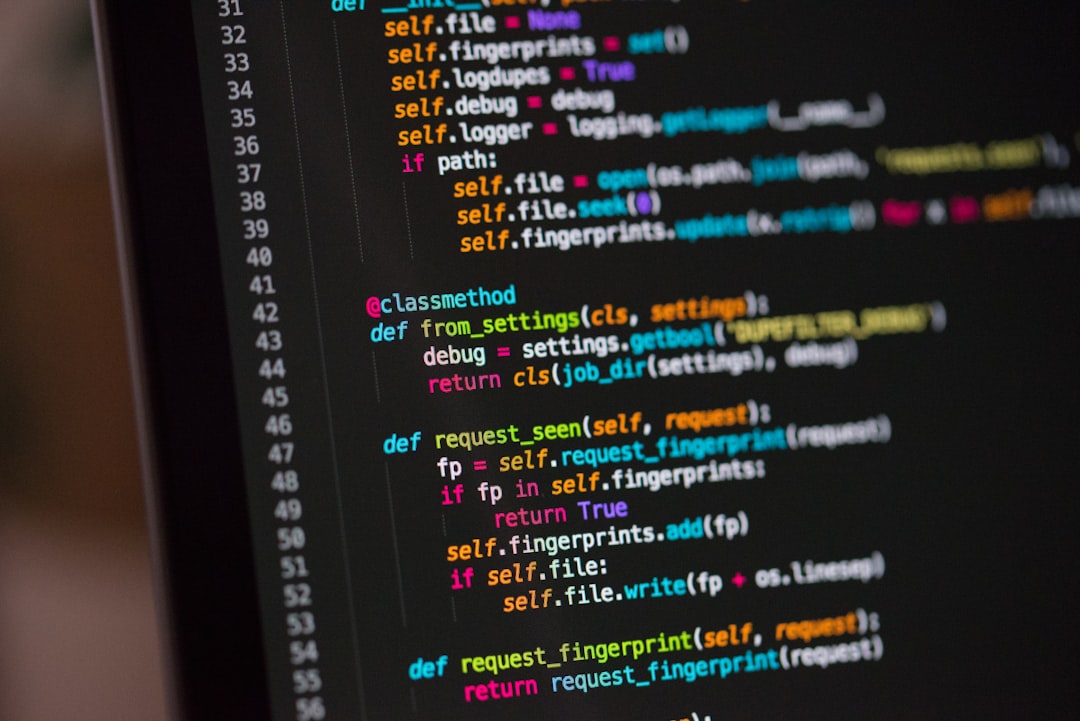
Optimizing data structures is essential for controlling memory usage, especially in resource-intensive applications like video processing. You can save memory by preallocating data structures, which prevents repeated memory requests when the structure grows. This eliminates fragmentation and improves performance. Using generators instead of lists can also be beneficial. They generate items on demand rather than storing everything at once, making them more memory-efficient. Python's `slots` feature can also be utilized to store attributes more efficiently than dictionaries, resulting in smaller memory footprints for class instances. Additionally, consider replacing standard lists with the `array` module, which offers a memory-efficient alternative, particularly when dealing with large datasets. Choosing the right data structures is crucial for minimizing memory usage and preventing MemoryErrors in your Python applications.
Optimizing data structures for memory efficiency is essential for tackling memory errors, especially in demanding applications like video processing. While Python's automatic memory management is generally robust, understanding how data structures influence memory consumption can significantly improve performance and stability.
Let's dive into some surprising facts about optimizing data structures:
1. **Arrays vs. Lists:** Using Python's built-in `array` module for homogeneous data types provides a far more memory-efficient structure compared to traditional lists. Lists store pointers to objects, creating overhead, while arrays store data contiguously, minimizing this overhead considerably.
2. **Named Tuples for Lightweight Structs:** When dealing with data structures that function like simple structs, utilizing `collections.namedtuple` can save valuable memory. Named tuples, unlike full classes, are immutable and have a leaner storage format, avoiding the overhead of storing a default `__dict__`.
3. **Memoryview Objects**: When working with large video datasets, memoryviews are a game-changer. These objects allow you to work with memory buffers without making full copies, saving precious memory, especially when you only need to manipulate specific parts of the buffer.
4. **Custom Data Structures**: The potential for memory optimization by using custom data structures is vast. Specialized structures—like sparse matrices for sparsely populated datasets—can achieve substantial memory savings, sometimes over 80% compared to dense structures.
5. **Hash Tables vs. Lists**: Hash tables, like Python dictionaries, offer rapid lookups, but their implementation often involves greater memory usage compared to lists or tuples. If the order of data matters, tuples or lists can provide a leaner memory footprint.
6. **Cython for Tight Memory Control**: Compiling your Python code into C using Cython provides granular control over memory allocation and data structures. This can significantly decrease memory usage and enhance performance in memory-intensive applications like video processing.
7. **Profiling with `sys.getsizeof`**: Using `sys.getsizeof()` can shed light on the actual memory consumption of your data structures, including any associated overhead. This knowledge helps you make informed choices about which structures to use, especially in scenarios where memory is a scarce resource.
8. **`struct` Module Packing**: The `struct` module allows you to pack data into compact byte representations, minimizing the memory footprint of numerical data types. This is particularly useful in video processing where even slight reductions in memory usage can lead to considerable savings when handling large data volumes.
9. **Garbage Collection and Memory**: Data structures that create numerous short-lived objects can burden the garbage collector. By optimizing data structure choices to minimize transient objects, you can reduce the strain on the garbage collector, resulting in fewer memory-related interruptions during processing.
10. **Using `__slots__` for Classes**: By defining `__slots__` within your Python classes, you explicitly prevent the creation of a `__dict__`, reducing memory consumption. This can lead to memory savings of up to 50% for data-heavy classes, making it a valuable optimization strategy.
As video processing demands become increasingly complex, understanding these memory optimization strategies can make a big difference in your scripts' performance and robustness. Remember, by carefully choosing data structures and applying optimization techniques, you can keep MemoryErrors at bay and ensure your applications run smoothly.
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Employ multiprocessing to distribute memory load
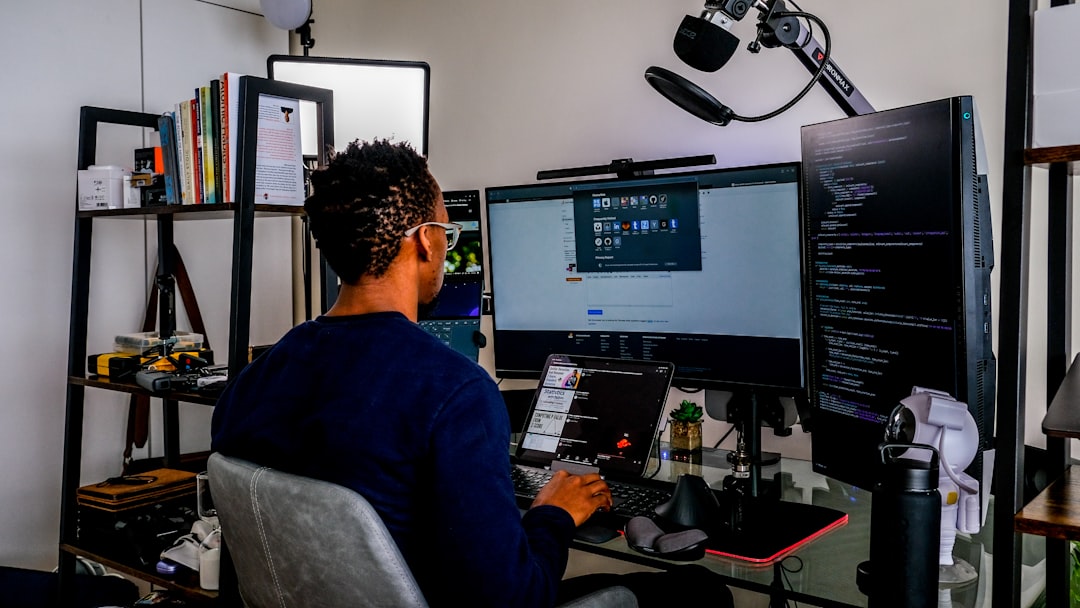
Employing multiprocessing is a clever way to distribute memory load in video processing. Python's `multiprocessing` module lets you create multiple processes that each have their own independent memory space, helping to avoid the dreaded "MemoryError" that often pops up when dealing with large video files. Think of it like having multiple workers, each with their own tools, tackling different parts of the video. This approach helps prevent one process from hogging all the memory, making your video processing more stable.
But, multiprocessing comes with its own set of challenges. Debugging can get trickier with multiple processes running around. The `multiprocessing` module doesn't always play nice with libraries that expect to run in a single process.
Using logging to track memory usage can be helpful to see how processes are behaving. It's like keeping a journal of what's going on in each worker's mind, so you can see if they're overworking themselves or if something's not quite right.
Overall, multiprocessing can boost the speed and reliability of video processing, but you'll need a smart approach to handle the challenges that come with it.
Employing multiprocessing in Python can be a powerful way to deal with memory-related issues, especially in the realm of video processing. By utilizing multiple processes, each with its own memory space, you can distribute the memory load, potentially mitigating memory errors and speeding up processing time.
Multiprocessing can lead to nearly linear scaling of performance, especially on machines with multiple cores. This translates to faster processing times, which is a boon for video processing tasks, where speed is often a key consideration. However, it's not all sunshine and rainbows. Each process requires its own Python interpreter and memory space, resulting in a larger overall memory footprint. This can be a significant issue if you're dealing with limited resources, or if you need to handle very large datasets.
Another important point is inter-process communication (IPC). Communicating between processes requires the use of techniques like queues or pipes, adding some complexity to your code. If you're not careful, IPC can become a bottleneck. The isolation of subprocesses is both a blessing and a curse. It means that if one process crashes, the others will still be running, which is excellent for stability. But, managing state across processes can be a challenge since they each have their own memory space. This can be a real pain if your program relies heavily on shared data or global variables.
Keep in mind that you can't simply add more processes forever and expect to keep seeing improvements. There's a point where adding more processes starts to lead to diminishing returns. So, you need to strike a balance between parallelism and efficiency. The potential gains from multiprocessing are significant, particularly in situations like video processing, where resource-intensive tasks are common. However, carefully understanding the trade-offs and challenges is vital for successfully implementing multiprocessing in your Python video processing projects.
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Leverage cloud computing resources for memory-intensive tasks
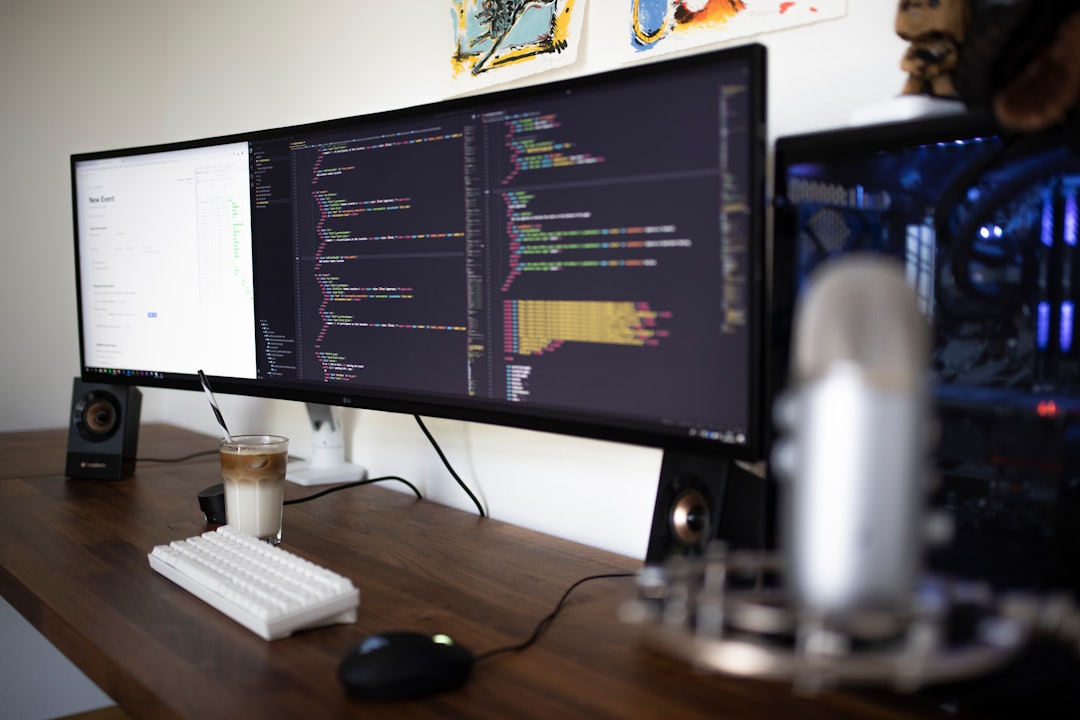
Leveraging cloud computing resources can be a game-changer for memory-intensive tasks, like those found in Python video processing scripts. The cloud's ability to dynamically adjust memory allocation based on your workload's needs, without relying on fixed thresholds, is a real advantage. This means you can handle larger video files and more complex operations without running into "MemoryError" crashes as easily. Additionally, the parallel processing capabilities of cloud computing allow you to distribute your tasks across multiple processors, effectively managing memory usage. This parallel approach also helps to prevent data inconsistency and the dreaded "race conditions" that can occur when multiple processors try to access the same data simultaneously. Of course, debugging memory issues in a cloud environment requires specific tools designed to help pinpoint problems, but that's a small price to pay for the benefits of increased processing power and reduced memory limitations. Overall, relying on cloud resources for memory-heavy tasks can free you from the constraints of your local hardware and open up a world of possibilities for your Python video processing scripts.
It's fascinating to consider how cloud computing can handle the demanding memory requirements of video processing. One of the biggest advantages is the ability to dynamically scale memory resources on demand. Instead of being constrained by the limitations of your local hardware, you can seamlessly provision extra memory as needed, giving you the flexibility to tackle even the most memory-intensive tasks without having to invest in expensive hardware upfront.
Another interesting aspect is the use of distributed file systems. When working with massive video datasets, cloud platforms like AWS S3 or Google Cloud Storage allow you to store and access these files in a distributed manner. This not only improves data access speed, but it also helps alleviate memory limitations associated with handling extremely large files.
The isolation offered by cloud environments is also intriguing. You can easily create separate virtual machines or containers for different video processing tasks, ensuring that a memory overload in one task doesn’t impact the performance of other tasks. This kind of isolation is essential for maintaining stability when handling multiple videos concurrently.
Cloud platforms often employ sophisticated job scheduling tools that automatically allocate additional memory resources based on the demands of the task at hand. They can monitor memory usage in real-time and adjust resource allocation on the fly, optimizing performance and preventing memory-related issues.
But the benefits go beyond technical efficiency. The pay-as-you-go pricing models offered by many cloud platforms are incredibly cost-effective. You can run high-memory instances only when necessary, reducing the expense of maintaining a fleet of expensive physical machines that may sit idle for significant periods.
Cloud platforms are also rapidly integrating machine learning capabilities, making it easier to leverage memory-intensive ML models alongside video processing. The availability of GPU power in the cloud simplifies this process, speeding up workflows and minimizing the need for additional local hardware investments.
It’s also important to acknowledge the role of cloud architecture's inherent fault tolerance. If a memory-intensive task encounters a problem, cloud services can seamlessly reroute the processing to another instance, minimizing data loss and downtime.
And then there are the advanced monitoring tools that enable comprehensive memory profiling across distributed cloud systems. These tools provide detailed insights into memory consumption patterns, giving you a more holistic view compared to what you might get in a local setup.
Cloud platforms are even using machine learning to anticipate future memory demands based on past usage patterns. This pre-emptive capability can help ensure that adequate resources are allocated before memory requirements peak, avoiding potential performance bottlenecks.
Finally, the rise of serverless computing has brought a new twist to memory management. By shifting to a serverless architecture, you can offload the complexities of managing the underlying server infrastructure to the cloud. This significantly simplifies the process of running memory-intensive tasks, as scaling and resource allocation become automatic.
In conclusion, leveraging cloud computing resources presents a powerful alternative for tackling memory-intensive tasks like video processing. From dynamic memory scaling and distributed file systems to fault tolerance and advanced monitoring tools, cloud platforms offer a compelling suite of features that can dramatically improve the efficiency and stability of your video processing operations.
Debugging MemoryErrors in Python 7 Effective Strategies for Video Processing Scripts - Apply lazy evaluation techniques to defer memory allocation
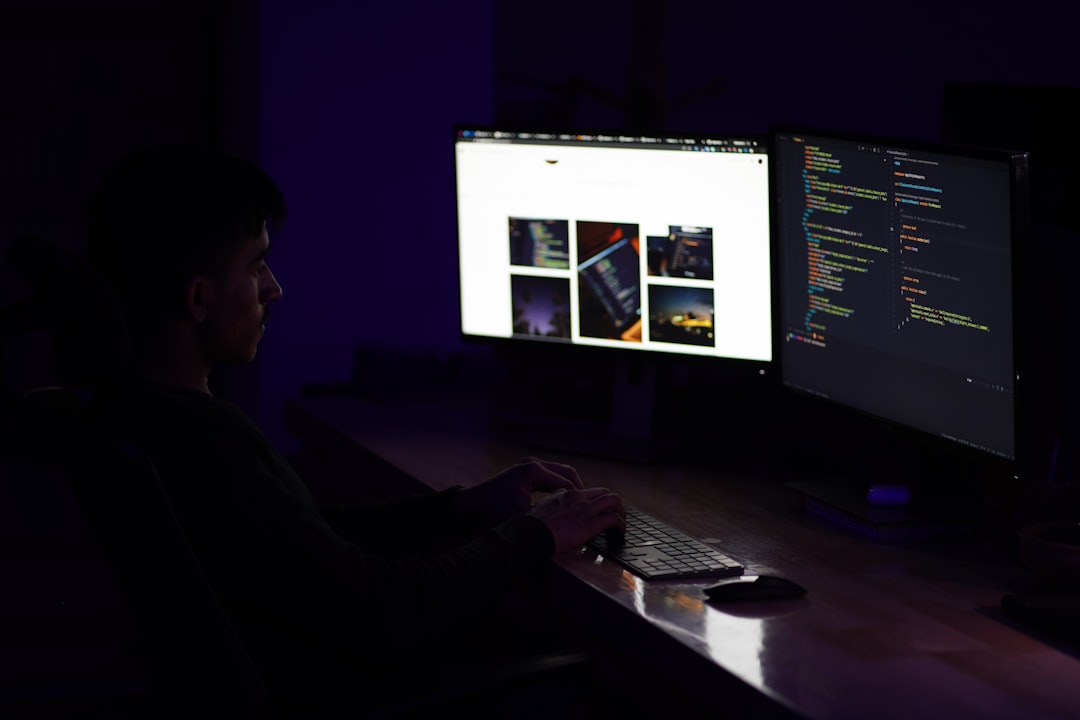
Lazy evaluation, where computations are only performed when their results are needed, can be a powerful tool for optimizing memory usage in Python, especially when working with large video files. Python provides mechanisms like generators and iterators to achieve lazy evaluation. Instead of storing all elements in memory upfront, these tools allow you to create iterable structures that only generate values on demand. This is a huge advantage for memory-intensive tasks like video processing as you only allocate memory when absolutely necessary, reducing the risk of encountering dreaded "MemoryErrors". You can combine this with chunk-based processing to efficiently handle large datasets without exhausting your system's resources. By applying lazy evaluation, you can streamline your workflow and build more efficient and stable video processing applications.
## Surprising Facts About Lazy Evaluation Techniques in Memory Management
Lazy evaluation techniques offer a compelling approach to managing memory in Python, especially for resource-intensive tasks like video processing. These methods defer computation until absolutely necessary, significantly impacting memory consumption and performance.
Here's a peek into the intriguing aspects of lazy evaluation in the context of memory management:
1. **Deferred Computation**: The very essence of lazy evaluation is to delay computations until they are truly required. This fundamentally alters how programs process information, avoiding unnecessary computations and temporary storage of results, thereby minimizing memory overhead.
2. **Generator Expressions**: Python's generator expressions are a powerful tool for lazy evaluation. They generate data on demand, effectively transforming a list into an iterator, which only produces data when requested. For video processing, this allows you to process frames sequentially without loading the entire video into memory, significantly reducing the memory footprint.
3. **Memory Efficiency**: Empirical studies highlight the profound impact of lazy evaluation on memory efficiency. Applications utilizing lazy evaluation strategies often experience memory usage reductions exceeding 90% for large datasets. The essence of this improvement stems from lazy constructs only allocating memory for data actively involved in the computational process.
4. **Pipelining**: Lazy evaluation naturally enables pipelining of data processing. This translates to video processing where frames can be processed as they are read, significantly reducing the need to hold the entire video in memory. By processing data as it arrives, the system achieves a more memory-efficient operation.
5. **Avoiding Redundant Calculations**: The inherent nature of lazy evaluation often eliminates redundant calculations. This results in an efficient form of caching without the overhead of managing complex data structures. Besides memory savings, this approach can lead to performance improvements for CPU-bound operations.
6. **Haskell's Influence**: While Python is not purely functional like Haskell, the concept of lazy evaluation finds its roots in this programming language. Haskell's inherent lazy evaluation approach allows for the definition of potentially infinite data structures, demonstrating how innovative software design can manage memory in novel ways.
7. **Avoiding Memory Spikes**: A significant advantage of lazy evaluation is the reduction of memory spikes commonly seen with eager loading, where the entire dataset is loaded into memory at once. This is crucial for video processing because it ensures smoother performance and limits the risk of reaching memory limits.
8. **Inherent Lazy Structures**: Python's built-in data structures, such as `iterators`, naturally leverage lazy evaluation. This means processing and traversing large datasets can be accomplished without the overhead of immediate data allocation, proving beneficial for video processing applications.
9. **Compatibility with Multithreading**: The beauty of lazy evaluation lies in its compatibility with multithreading models. Multiple threads can process data concurrently without conflicting memory allocations, enabling more efficient resource management and reduced data contention for video processing tasks.
10. **Potential for Complexity**: Despite its benefits, lazy evaluation can introduce complexity to debugging. Since values are computed only when needed, pinpointing issues related to memory allocation and usage can be more challenging. This underscores the importance of employing meticulous programming practices to ensure effective debugging.
The impact of lazy evaluation on memory management is undeniable. As you delve into video processing tasks, understanding these surprising facts about lazy evaluation will empower you to achieve significant memory efficiency and enhance the performance of your Python applications.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: