Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis - Understanding the basics of KMeans clustering algorithm
KMeans clustering is a fundamental unsupervised learning technique designed to organize data into distinct groups, or clusters. The algorithm's primary objective is to partition data into a predetermined number of clusters (k) by minimizing the overall distance between each data point and its corresponding cluster's centroid. This involves an iterative process where initial centroids are randomly selected, data points are assigned to the nearest centroid, and then centroids are recalculated based on the assigned data points. This process repeats until the algorithm stabilizes, achieving convergence.
The simplicity of KMeans makes it a favored choice for both beginners and experienced data scientists. Its strength lies in its ability to expose inherent patterns within datasets, providing valuable insights for various applications. These applications span a wide range, from understanding customer groups in market segmentation to identifying unusual data points for anomaly detection. However, users need to carefully consider the choice of 'k,' the number of clusters, as this parameter can significantly impact the clustering results and the effectiveness of the analysis. Choosing the wrong value can lead to suboptimal cluster assignments and potentially misleading insights.
KMeans clustering, while a foundational technique, relies on the idea of minimizing the spread of data points within each cluster. It typically uses Euclidean distance to measure this spread, which can lead to unexpected results when the clusters have non-spherical shapes. The algorithm's starting point, the initial centroid positions, can significantly impact the final clusters, and running it multiple times with different initializations often helps in getting a more stable outcome.
Choosing the right number of clusters (k) is critical, and the commonly used 'elbow method' can be tricky, especially with intricate datasets. The method is subjective and can result in varying interpretations of the optimal k. Another limitation is KMeans' struggle with clusters of differing sizes and densities. In these cases, alternative algorithms like DBSCAN or hierarchical clustering might offer better results.
Furthermore, the time it takes for KMeans to converge can vary considerably based on the dataset's characteristics. Working with large or high-dimensional datasets can lead to longer processing times. Though straightforward, KMeans can become computationally expensive, especially when you increase the number of clusters or the dimensions of the data. This can hinder real-world applications where processing speed is crucial.
Dealing with data that includes categorical features is challenging for KMeans. We need techniques like one-hot encoding to pre-process the data and make it suitable for KMeans. Also, the possibility of getting empty clusters during the clustering process is a frequent problem with KMeans, potentially leading to unpredictable behavior. It often necessitates adjustments to the algorithm or extra handling to manage this.
Applying dimensionality reduction techniques like Principal Component Analysis (PCA) before using KMeans can help improve the algorithm's performance by removing noise and making the underlying patterns in the data more apparent. As a bonus, KMeans can be used to build prototypes for use in machine learning, specifically in supervised learning, offering valuable insights into how different classes might be distributed within the data.
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis - Implementing KMeans in Python without external libraries
Implementing KMeans in Python without external libraries provides a unique opportunity to grasp the inner workings of this algorithm. By building the core components from scratch, you gain a deeper understanding of the iterative process: starting with centroid initialization, moving to assigning data points to the nearest centroid, and finally, recalculating centroids based on their assigned points. This approach emphasizes key concepts, including the need for scaling features to ensure fair contributions to distance calculations. The process of finding the optimal number of clusters, often through the Elbow Method, becomes more apparent.
While conceptually simple, challenges surface when dealing with clusters of varying sizes or irregular shapes. This highlights the need for careful consideration of whether KMeans is the appropriate choice for a particular dataset. Despite potential limitations, developing KMeans purely in Python reinforces crucial principles in both machine learning and data analysis. This practical approach can be highly valuable for solidifying your comprehension of this core clustering algorithm.
1. **The Complexity of Implementation**: Building KMeans from scratch offers a stark contrast between the algorithm's conceptual simplicity and the practical challenges of coding it. This is particularly true when attempting to optimize the algorithm's convergence speed and handling unusual situations like completely empty clusters or cases where the algorithm simply doesn't settle on a stable solution.
2. **The Impact of Initial Centroid Selection**: The initial placement of the centroids can have a huge impact on the resulting clusters. Running the same algorithm multiple times with different starting points can yield wildly different outcomes. This sensitivity raises questions about the development of better, more systematic initialization methods, perhaps moving beyond simply random choices.
3. **Beyond Euclidean Distance**: While KMeans typically uses Euclidean distance, we can alter this core metric. By experimenting with other measures, such as Manhattan distance or cosine similarity, we can potentially uncover distinct patterns within the data, especially when working with very high-dimensional datasets.
4. **The Curse of Dimensionality**: As data gets more complex and features increase, KMeans tends to struggle. This is often termed the curse of dimensionality, as the meaning of distances between points becomes less reliable in high-dimensional spaces. This implies that data pre-processing techniques, like dimensionality reduction, become crucial but can add another layer of intricacy to our work.
5. **Sensitivity to Noise and Outliers**: KMeans can be easily swayed by outliers in the data, as these points can heavily skew the centroid calculations. Incorporating robust methods or employing data filtering techniques to remove problematic data points might address this issue, but adds more layers of complexity to our code.
6. **The Assumption of Spherical Clusters**: KMeans fundamentally assumes that data clusters will be relatively spherical or compact. This assumption can lead to inaccurate results if the true data structure is more complex or elongated. It highlights the need to explore alternative clustering methods that are better equipped to handle more intricate shapes.
7. **Defining Convergence**: Choosing when to stop the iterative KMeans process can significantly impact the outcome. Implementing various criteria for halting the algorithm, based on things like changes in the position of centroids or the degree to which data points are shifting between clusters, can create subtly different outcomes.
8. **Analogy to Learning Rates**: While not typically described in those terms, considering the number of iterations we allow the algorithm to run before stopping can offer insights into how the algorithm 'learns' by iteratively refining centroid positions.
9. **The Challenges of Real-World Data**: Datasets encountered in practice aren't always neat and complete. They often have missing data or inconsistent entries. Implementing KMeans without libraries forces us to handle these imperfections with more explicit coding, potentially leading to pre-processing and data cleaning routines being integrated more directly into the KMeans algorithm.
10. **Bringing KMeans to Practical Applications**: Implementing KMeans from the ground up allows us to not just understand the algorithm but to apply its principles more flexibly. Areas like computer vision, where we might want to segment videos into meaningful parts, could greatly benefit from a deeper understanding of clustering principles, potentially improving the accuracy and effectiveness of analyses.
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis - Applying KMeans to video frame analysis
Applying KMeans to video frame analysis provides a valuable approach for organizing and understanding visual content within a video. We can treat each video frame as a distinct data point, allowing KMeans to group together frames that share similar visual traits, enabling applications like identifying scene changes or creating concise video summaries. This method offers the potential to unearth underlying patterns within the video that might not be easily discernible through simple observation, ultimately improving the efficiency of video processing.
However, practical application brings forth certain difficulties. Variable frame quality, noise inherent in video capture, and the possibility of generating clusters with no assigned frames can lead to less reliable results. Therefore, a strong grasp of the KMeans algorithm's inner workings and limitations, including the significance of pre-processing like reducing the number of characteristics in each frame, is critical to obtaining meaningful outcomes in video analysis. This careful application of KMeans to videos allows us to extract deeper, more useful information from the content.
Applying KMeans to video frame analysis introduces a unique set of considerations. We can treat each frame as a sequence of data points, allowing us to potentially capture how the scene changes over time. However, each frame can have hundreds of thousands of features, one for each pixel. This presents a significant hurdle for KMeans, especially concerning computational cost and the reliability of distance calculations.
The high dimensionality associated with video frames intensifies the curse of dimensionality, where distance-based clustering can become unreliable. Furthermore, interpreting the resulting clusters can be tricky as compared to traditional KMeans applications where clusters often represent easily understandable categories. Video frame clusters might be more abstract, possibly requiring human interpretation to connect them to meaningful segments in a video.
We must also consider the variability in how video frames are captured. Lighting, motion blur, and other factors can introduce noise or artefacts that can hinder the effectiveness of KMeans. We need to think about how to address these inconsistencies during pre-processing. It might be useful to combine KMeans with other machine learning techniques to extract more information from videos. For instance, integrating optical flow analysis or object detection algorithms could provide a deeper understanding of movement and objects within a frame, extending beyond simply grouping pixels.
Real-time video analysis poses a different challenge entirely. Applying KMeans in real-time requires fast convergence and readily interpretable results, which might not always be achievable with KMeans. We might have to consider alternative methods better suited to handling dynamic data.
While KMeans typically employs Euclidean distance, experimenting with other metrics could prove advantageous when dealing with motion-rich or sparse video segments. We might need a more refined measure of similarity for these specific types of data.
We might encounter situations where KMeans produces empty clusters in video segmentation tasks, potentially because certain frames lack features that align with existing groups. This presents a situation where we need to either re-initialize or merge clusters to ensure the segmentation process continues without gaps.
Perhaps one of the more interesting uses of KMeans within this context is prototype creation. We can potentially identify normal behavior or scenes by clustering representative frames. This can subsequently help us detect anomalies, like unusual events or security breaches. By creating a model of 'normal', we can then identify anything deviating from it.
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis - Optimizing cluster selection using the Elbow Method
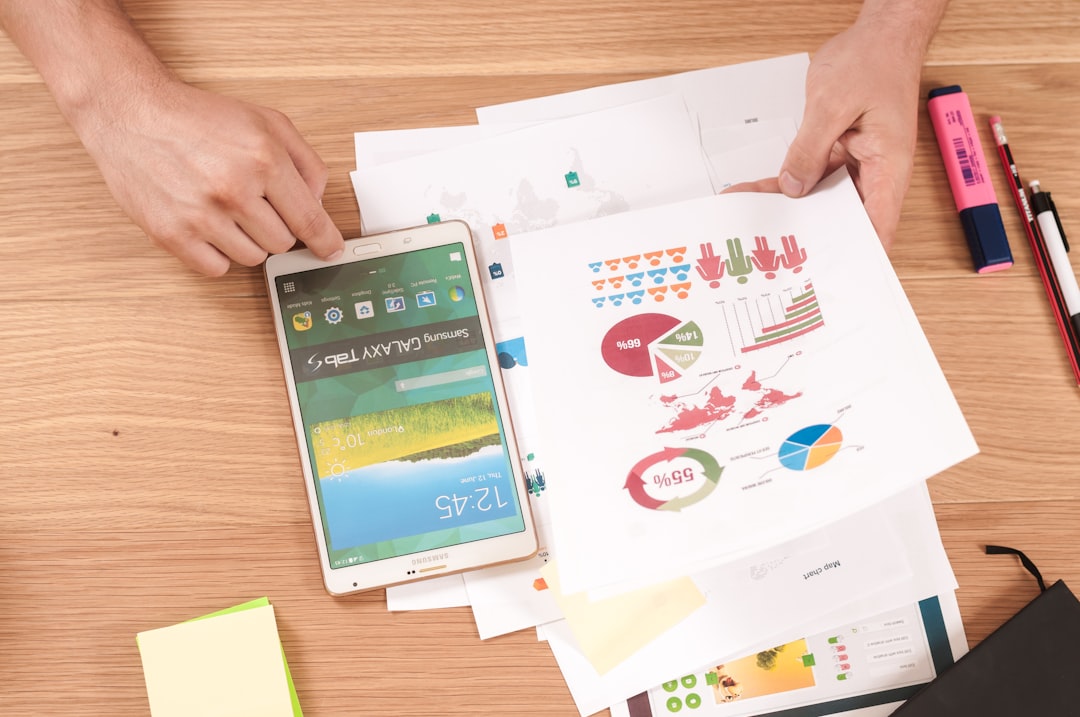
Finding the optimal number of clusters (k) is essential when using the KMeans algorithm, especially for applications like analyzing video content. The Elbow Method helps us achieve this by calculating the within-cluster sum of squares (WCSS) for different values of k. As we increase the number of clusters, the WCSS naturally decreases, suggesting tighter clusters. The Elbow Method visually depicts this relationship by plotting WCSS against the number of clusters. The "elbow" point on this plot, where the rate of decrease in WCSS changes, serves as a guide to the ideal k value. While the Elbow Method offers a simple way to estimate k, it's important to acknowledge that it's a somewhat subjective approach. The interpretation of the "elbow" can be influenced by the specific characteristics of the dataset. It's also beneficial to consider other techniques, like silhouette analysis, to further refine the selection of the optimal number of clusters. By carefully navigating these aspects of the Elbow Method, we can leverage the power of KMeans more effectively in a wider range of applications, including video analysis, ensuring that our clustering results are more robust and meaningful.
1. **Interpreting the Elbow's Bend**: The Elbow Method aims to pinpoint the optimal number of clusters (k) in KMeans by observing the point where the rate of decrease in within-cluster sum of squares (WCSS) slows down. However, finding this "elbow" can be tricky, as it often relies on visual inspection and can be subjective. What looks like a clear bend to one person might not be as evident to another, emphasizing the role of experience and intuition in this process.
2. **Dataset Influence**: The effectiveness of the Elbow Method depends heavily on the characteristics of your data. Some datasets have a very clear elbow point, making it straightforward to choose k. Others are more complex, perhaps with overlapping clusters or a more gradual decrease in WCSS, leading to less definitive elbow points.
3. **A Broader View**: While the Elbow Method primarily focuses on the total WCSS, using it in combination with other visual checks can improve our understanding of the clustering quality. For instance, plotting silhouette scores or Dunn indices alongside the WCSS plot can give a more nuanced view of the clusters and help us make a better-informed choice about k.
4. **Feature Scaling Matters**: The Elbow Method can be sensitive to the range of values in the features of our data. If features have very different scales, the distances used to calculate WCSS might be heavily skewed toward the features with larger values. This can lead to an inaccurate representation of the elbow point, underscoring the need for proper feature scaling before applying the Elbow Method.
5. **An Iterative Process**: The elbow point might not be set in stone. It can shift as the KMeans algorithm iterates, especially with datasets that change over time. It's a good practice to check the elbow point regularly during the algorithm's runs to ensure we are still selecting the most appropriate k for the current data.
6. **Automation of Elbow Detection**: Researchers have come up with automatic ways to find the elbow point, using methods like calculating second derivatives or using statistical tests. These approaches try to reduce the subjective aspect of identifying the best k value.
7. **Avoiding Overfitting**: If we choose too many clusters because we misread the elbow, we might end up overfitting the data. Overfitting means the model becomes too specific to the training data and doesn't generalize well to new data. This emphasizes the importance of carefully validating our clustering choices after applying the Elbow Method.
8. **Limited Applicability**: The Elbow Method isn't a one-size-fits-all solution. In high-dimensional or very complex datasets, there may not be a distinct elbow. This makes it necessary to explore other cluster validation methods to find the optimal k.
9. **Data's Role**: The way the data is organized has a huge impact on the outcome of the Elbow Method. For instance, if clusters overlap a lot or if the dataset is very imbalanced (with some clusters having significantly more data points than others), the elbow can be hard to interpret. We might need to combine the Elbow Method with other tools to make a sound decision about clustering.
10. **Beyond Simple Shapes**: The Elbow Method relies on the assumption that more clusters always lead to a better fit. However, for datasets with non-linear structures (clusters that aren't spherical), it might not capture the best configurations. In such cases, density-based clustering techniques or other approaches might provide more accurate results, suggesting the need for a variety of methods for a given task.
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis - Visualizing KMeans results for video content
Visualizing the outcome of KMeans when analyzing video content is key to making sense of the clusters it creates. We can use methods like scatter plots to represent the clustered video frames in a way that's easier to understand. Different colors on the plot usually represent different clusters, making it easier to see how the frames group together. But video frames have a lot of data (high-dimensionality), which makes visualizing the clusters directly difficult. To solve this, we often use techniques like Principal Component Analysis (PCA) or t-distributed Stochastic Neighbor Embedding (t-SNE) to reduce the amount of data, making the visualization more manageable. This process is vital because it helps us understand the relationships between frames and spot patterns that might be hidden otherwise. It's important to remember that these visualizations are tools, and like any tool, they can be misinterpreted if we're not careful. For instance, if the clusters aren't perfectly round or if there's noise in the video data, it might lead to a skewed interpretation of the clusters.
Visualizing KMeans results for video content presents unique challenges compared to standard applications. Each video frame can be seen as a high-dimensional data point, with the number of dimensions often reaching tens of thousands due to pixel color values. This high dimensionality can make clustering less reliable, requiring careful feature selection or methods like dimensionality reduction before using KMeans effectively. Additionally, video frames are sequential and related through time, which makes the analysis more intricate. We're not just grouping similar frames, but also trying to understand how clusters evolve as the video unfolds.
We might also observe cyclical patterns in video content where scenes repeat, and while KMeans may group similar frames together, it might struggle to separate distinct cycles from unique events. This can lead to mixed or unclear results. Preprocessing steps, like converting frames to grayscale, resizing them, or adjusting their histograms, are often needed to improve KMeans performance in this context, although this adds to the complexity of our implementation. KMeans, with its tendency to favor spherical clusters, can be easily affected by the visual noise that often comes with videos, such as changes in lighting or motion blur. Even small changes can cause frames to shift between clusters, highlighting the need for careful pre-processing to minimize this problem.
Interpreting the output of KMeans applied to video can be tricky as well. Clusters may not correspond to easily understandable segments of the video. Extra analytical steps might be needed to link the clusters back to video transitions or other meaningful elements. Another thing to consider is that KMeans' assumption of spherical clusters may not always hold with video data. Scenes with gradual shifts in color or spatial patterns can create fuzzy clusters. We might need to look into other clustering techniques better suited for capturing more complex structures.
Furthermore, running KMeans in real time is difficult due to its computational demands. The algorithm's need to process lots of data, especially with high-resolution frames, can cause delays, making it less useful for time-sensitive video processing applications. There's also the possibility of creating empty clusters, particularly if certain frames lack distinct features. This makes subsequent frame assignment a problem and requires methods for cluster maintenance and potentially re-initializing the process.
One potential application is identifying representative frames, also known as prototypes. KMeans can cluster frames based on visual characteristics to summarize a video or to help in anomaly detection. By developing a model of 'typical' frames, we can then easily spot frames that deviate from this pattern, which can be helpful in spotting unusual events or potentially highlighting issues in video content. This example showcases the unique potential of KMeans when working with video data.
Demystifying KMeans A Python Implementation from Scratch for Video Content Analysis - Practical challenges and solutions in KMeans implementation
When implementing KMeans, especially for video content analysis, several practical obstacles can arise that influence the algorithm's performance. One significant issue is KMeans' susceptibility to the initial positioning of centroids, which can drastically affect the final clustering results. Furthermore, the algorithm's reliance on Euclidean distance can become problematic in high-dimensional spaces. This is often referred to as the "curse of dimensionality" where the accuracy of distance calculations decreases with increased dimensions. Handling noisy data and outliers presents another hurdle, as these elements can skew the centroids. The possibility of creating empty clusters during the process adds further complexity, requiring adjustments to prevent erratic behavior. These challenges highlight the necessity of understanding KMeans' shortcomings and exploring alternative clustering methods when facing intricate data structures or situations where the inherent assumptions of KMeans may not be met.
1. **Convergence Challenges**: While KMeans is conceptually simple, its convergence can be a bit tricky. Things like how the centroids are initially placed or the way the data is distributed can cause the algorithm to bounce around or get stuck in suboptimal clustering solutions. This can lead to less-than-ideal results.
2. **Sensitivity to Feature Scaling**: KMeans is quite sensitive to how the data is scaled. If features aren't on the same scale, the ones with wider ranges can dominate the distance calculations, which can distort the clustering. Therefore, it's super important to properly scale features, like by normalizing or standardizing, to get accurate clustering.
3. **The Challenge of Finding the Right 'k'**: Deciding on the optimal number of clusters (k) is more of an art than a precise science. The Elbow Method, while helpful, can be a bit subjective. We need to explore other techniques like the Silhouette Method or Gap Statistics to get a more complete understanding of how k influences clustering performance.
4. **Struggles with Non-Round Clusters**: KMeans tends to stumble when clusters aren't perfectly spherical or have uneven densities. If the data's true structure doesn't fit the algorithm's assumptions, we might see better results using other clustering methods, such as Gaussian Mixture Models or Spectral Clustering.
5. **The Curse of High Dimensionality**: When data gets highly complex and has a lot of features, points can appear equally distant from each other. This is called the curse of dimensionality, and it can hinder KMeans because the meaning of distance becomes fuzzy. To address this, we might need to use techniques like PCA or t-SNE to reduce the number of dimensions before applying KMeans.
6. **The Influence of Outliers**: Outliers can really mess up KMeans because they can drastically shift the centroids, ruining the overall quality of the clusters. To mitigate this, we might need to use more robust clustering methods or clean up the data by removing the outliers.
7. **Dealing with Empty Clusters**: One issue that comes up with KMeans is empty clusters. This means a centroid might not have any data points assigned to it anymore. This can happen during the iterations and often requires strategies to reinitialize or merge clusters to keep the segmentation process going smoothly.
8. **Balancing Speed and Accuracy**: There's always a trade-off between how fast the algorithm runs and how accurate the results are, especially with large datasets. To optimize performance, we might need to implement some clever shortcuts or sampling techniques to reduce processing time without sacrificing accuracy.
9. **Interpreting the Results**: Interpreting the output of KMeans can be tough, especially with complex data. Clusters might not always line up neatly with pre-defined categories. So, we may need to do some post-hoc analysis or use different visualization techniques to get a better grasp of what the clusters represent.
10. **Adding Time to the Mix**: When using KMeans for video analysis, we face added complexity due to the sequential nature of video frames. How things change over time can influence cluster formation. Adapting the algorithm to incorporate time can help us create more meaningful clusters related to scene transitions or activities within the video.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: