Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide - Setting up the Python Environment for CNN Development
Building a CNN for video analysis starts with establishing a robust Python environment. Key to this is utilizing libraries like TensorFlow, Keras, and PyTorch—they form the backbone of CNN development, providing the tools for constructing and training these complex models. Alongside these core frameworks, you'll find libraries like NumPy for data manipulation and Matplotlib for visualization indispensable for understanding your data and model behavior. While you could set up your own environment, platforms like Google Colab offer a user-friendly path, pre-loaded with essential libraries and access to powerful GPUs, making development more efficient, particularly when handling the large datasets common in video analysis. It's recommended that you start with simpler CNN structures, potentially even using pre-built architectures found in numerous online tutorials. As your comfort with the tools and the nature of CNNs grows, you can then explore more sophisticated models tailored to the specific challenges of your video analysis project. While starting with simpler examples is wise, keep in mind that for some truly sophisticated applications, simple CNNs might be insufficient for the task.
To effectively develop CNNs in Python, you'll need to carefully manage your environment. Dealing with multiple library versions, especially for frameworks like TensorFlow or PyTorch, can be tricky due to their specific requirements. Isolating your projects with virtual environments becomes critical to avoid potential conflicts arising from different package versions used across projects on the same machine.
The operating system you use also matters. Certain libraries might be a bit more challenging to install on Windows compared to Linux. For managing intricate dependencies common in machine learning, Conda often proves superior to pip because it handles binary dependencies and compatibility seamlessly, thereby accelerating the setup process.
If you intend to utilize GPUs for accelerating your CNN training, configuring CUDA and cuDNN is mandatory. Getting this aspect right can dramatically reduce the time it takes to train your models. It's helpful to keep in mind the architecture of the GPU you're using as well. GPUs with a higher number of CUDA cores tend to be faster for deep learning because they can handle parallel computations more efficiently.
Jupyter Notebooks, while convenient for combining code, visualization, and documentation, might require some extra setup steps for compatibility with certain libraries. Another aspect to consider is Docker. Docker containers are gaining traction in development environments because they offer a consistent setup across different machines, facilitating deployment and scaling in production environments.
Tools like Poetry can be valuable for managing dependencies more systematically than traditional approaches involving requirements.txt files. But, often overlooked is the importance of establishing comprehensive logging and error tracking. Developing robust logging mechanisms within your environment can significantly improve debugging and overall development workflow, especially when dealing with intricate CNN models.
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide - Data Preprocessing Techniques for Video Frame Extraction
Preparing video data for analysis by a CNN involves several preprocessing steps. One crucial aspect is extracting individual frames from the video files, a task typically handled by libraries like OpenCV. Sometimes, a basic normalization step—subtracting the mean from each frame—can be beneficial, but it's not always necessary, and using the raw frames might be sufficient in certain scenarios.
Since video data can be quite large, efficient processing techniques are important. One approach, though naive, is to treat each frame as an independent image, run it through a CNN, and then select the most likely label based on the resulting probabilities. This avoids loading the entire video into memory at once, allowing for the analysis of even very long videos.
Frameworks like TensorFlow provide helpful utilities for managing the data pipeline, including preprocessing. The FrameGenerator class is an example of such a utility, offering streamlined ways to handle video data within the TensorFlow environment. It's also important to consider the varying length of video datasets. A common preprocessing step is to reduce videos to a standard number of frames. This ensures consistency across the data, and by carefully choosing the reduction method, it's possible to maintain most of the important information within each video, thereby improving the ability of the model to generalize from training to unseen examples.
When working with video data, we can leverage the inherent redundancy across frames. Often, consecutive frames only differ slightly, making techniques like extracting keyframes a reasonable approach to reduce the overall data size without significant information loss. This is especially relevant since, for many videos, a large portion of the frames are often quite similar.
More sophisticated techniques look at optical flow, essentially capturing the motion between frames. This approach can reveal dynamic aspects of the video that simply extracting individual frames might miss. While technically more complex, understanding the movement within the scene can be very important.
When dealing with large amounts of data, especially in real-time scenarios, downsampling is a good way to reduce the computational load. Reducing a video to a single frame per second, for instance, still provides valuable information about the content. It's a good compromise when you need to process many frames and are not as concerned with minor differences between frames.
To improve the visibility of features in frames, techniques such as histogram equalization can be used during preprocessing. This is especially important if the video was captured under poor lighting conditions. It enhances the contrast of frames, making features more distinguishable and easier for the CNN to identify.
We can effectively increase the variety of training data without collecting a whole new set by using data augmentation methods. Operations like frame rotations or flips can help reduce overfitting, creating diverse training examples that can help generalize to unseen data.
Videos with text can benefit from OCR during preprocessing. Incorporating OCR in this way enables the extraction of important textual data directly from the frames, expanding the scope of information we can use to analyze the video.
The choice of color space during the preprocessing phase, like switching from RGB to YUV, can make a big difference in CNN performance. Each color space represents information in a different way, making certain features stand out depending on the analysis. Understanding this relationship can lead to better performance.
Temporal sampling, like choosing every nth frame, is a clever way to cut down on redundant data while still retaining a sense of time within the video. It's a useful strategy for managing large video datasets and avoiding potential bottlenecks related to the high volume of data being processed.
Using multi-resolution techniques is a valuable approach for preprocessing video frames. Here, we process frames at various resolutions, enabling the CNN to identify features at different levels of detail. This approach can enhance the accuracy of the model by allowing it to identify a broader range of features.
Removing noise, such as with Gaussian blurring, during the preprocessing stage can improve the quality of the frames presented to the CNN. The aim is to filter out noise and distracting artifacts from the video frames, enabling the network to better focus on more significant information for classification. This is especially useful when videos may be captured in situations where there is inherent noise.
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide - Designing the CNN Architecture for Frame Analysis
Designing the CNN architecture for analyzing video frames is crucial for achieving good results. A typical CNN architecture is built from convolutional layers (to extract visual features), pooling layers (to reduce the amount of data), and fully connected layers (to make predictions). However, videos present unique challenges compared to static images because frames can change dramatically over time. As a result, we need a flexible design process for the CNN. We should start with a relatively simple CNN structure and then add more complex elements as needed to better capture the dynamics of videos. Furthermore, we need to pay close attention to how we prepare our video data and understand what kind of input our chosen CNN structure requires for it to learn effectively. This careful preparation of the data and design of the CNN are key for training the CNN to successfully understand patterns of movement and actions within videos.
Designing the architecture of a CNN specifically for analyzing video frames presents a unique set of challenges and opportunities. While CNNs are well-established for image recognition, applying them to sequences of frames requires careful consideration of temporal information.
One intriguing avenue is to incorporate specialized layers that can explicitly handle the temporal dimension. For instance, 3D convolutional layers can effectively learn patterns across multiple frames, capturing the dynamics of motion and changes within a video. It's a fascinating approach, although it's worth remembering that this added complexity also increases the number of parameters, which can lead to issues during training if not handled carefully.
Another key design aspect is feature extraction. Deepening a CNN typically improves its ability to extract more complex features. However, a trade-off emerges as we go deeper. We can sometimes encounter a decline in performance if we're not mindful of overfitting and the potential for diminishing returns. This highlights a need for techniques like regularization and careful validation to optimize the network's depth for the specific analysis task at hand.
Pooling layers play a vital role in how features are summarized and hierarchically represented within a CNN. Max pooling, for example, often focuses on the strongest features within a region, while average pooling offers a more holistic summary. The choice of pooling strategy can have a profound impact on the type of features captured, ultimately influencing a model's accuracy in classification.
Batch normalization is an often-overlooked but crucial component, especially in more complex networks. It helps stabilize the learning process by reducing the internal covariate shift that can occur during training. This technique essentially ensures that the distribution of input data remains relatively consistent across layers, which often accelerates the convergence of the training process.
Leveraging pre-trained models offers an interesting shortcut. For example, models like those trained on the massive ImageNet dataset can offer a head start in feature extraction for frame analysis. These models, having learned generic visual features, can then be adapted or fine-tuned for the specifics of our video analysis problem.
A clever strategy to further improve performance is to adjust only the output layers of a pre-trained model. Replacing the last few layers with a structure tailored for the specific number of classes we need to distinguish significantly improves the model's ability to focus on the relevant aspects of the data. This technique avoids the need to retrain the entire model from scratch, which can be computationally intensive.
More advanced architectures such as ResNet and Inception have emerged as strong candidates for challenging video analysis tasks. These designs incorporate clever techniques like skip connections and multi-scale feature extraction. This helps alleviate issues related to vanishing gradients and allows for deeper networks that maintain strong performance, all while reducing computational complexity compared to naively increasing depth.
The selection of activation functions plays a significant role in the overall training dynamics. ReLU and its variants (like Leaky ReLU or Parametric ReLU) often show faster convergence and improved performance compared to older activation functions like sigmoid or tanh.
Even the initial size and aspect ratio of input frames can significantly influence the model's performance. Changes in the input size affect how the CNN scales features, ultimately affecting its ability to generalize to different video inputs. Understanding how different input sizes affect the learned features is vital for ensuring that the CNN's capacity matches the nature of the video data.
Finally, ensemble methods can dramatically improve accuracy in many scenarios. These methods involve combining predictions from several CNN models. The concept is that different models may capture different aspects of the data, leading to more robust and accurate results compared to a single network. This approach shows that the potential of CNNs can be pushed even further through clever combinations and careful architectural designs.
In conclusion, designing a CNN for video frame analysis involves considering several facets that go beyond typical image classification tasks. Understanding the nuances of temporal information, applying advanced architectural techniques, and utilizing pre-trained models can pave the path to building robust and accurate models capable of capturing the rich information encoded within video sequences. While the field is rapidly evolving, a thorough grasp of these concepts is crucial for achieving successful results.
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide - Training the Custom CNN Model on Video Datasets
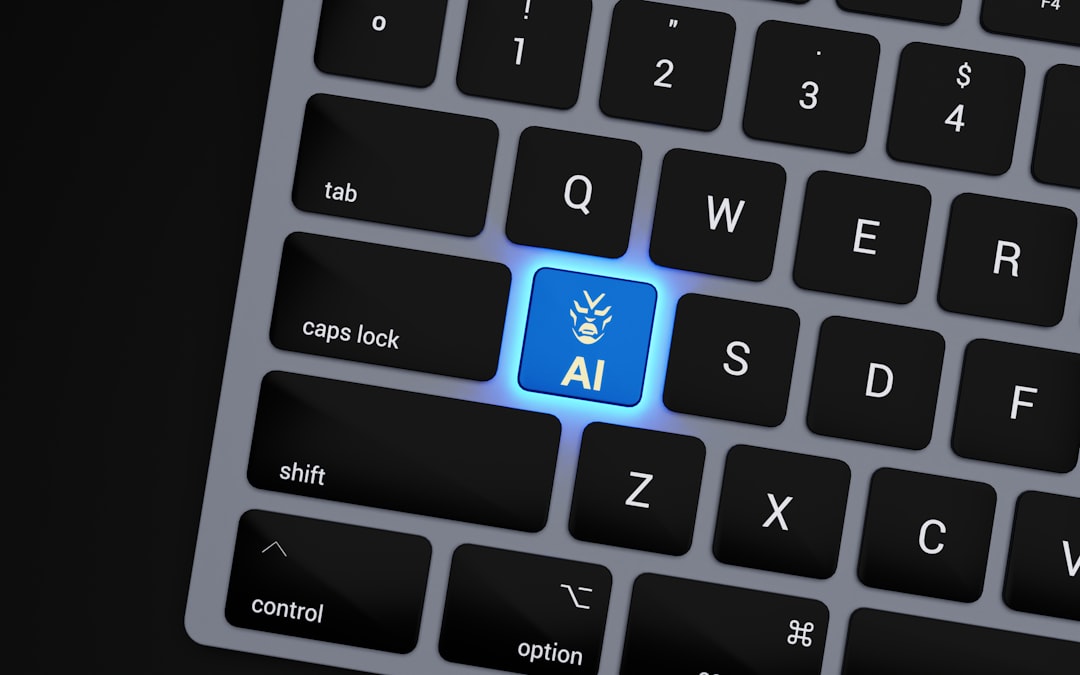
Training a custom CNN model on video data introduces unique challenges and opportunities compared to traditional image analysis. To handle the sequential nature of video, we often employ 3D convolutional networks, allowing the model to learn patterns across multiple frames and effectively capture the temporal aspects of movement and change within the video. A common strategy is transfer learning, where we take a pre-trained CNN model (often trained on a large dataset like UCF101, which is popular for action recognition) and fine-tune it on our specific video dataset. This approach leverages the model's prior knowledge to improve performance.
Before training, we must carefully prepare our video data. This includes tasks like resizing frames, standardizing pixel values, and ensuring all the input frames have consistent dimensions. These preprocessing steps are critical for consistency and efficient training. Training CNNs on video datasets typically requires harnessing the power of GPUs for faster computation, especially when working with large datasets. Careful selection of the model's architecture, combined with optimization of parameters (like batch size and learning rate), is essential to balance the need for high accuracy with practical considerations of computational resources and training time. The goal is to create a model that both effectively learns the patterns in the videos and trains reasonably quickly.
Training a custom CNN model for video analysis involves several unique considerations compared to standard image processing. One key challenge is capturing the **temporal dynamics** inherent in video sequences. Leveraging 3D convolutional layers allows the network to simultaneously process both the spatial information within each frame and the temporal information across a sequence of frames. This captures how features evolve over time, though it comes with the increased complexity of training a model with a greater number of parameters.
Another aspect is addressing the often-redundant nature of video data. Since consecutive frames are frequently very similar, techniques like **keyframe extraction** or **optical flow analysis** can significantly reduce the training data size without losing much information. This reduces the computational burden during training and can also potentially improve performance.
When training CNNs on video data, it's helpful to utilize **data augmentation** in ways that incorporate the temporal aspect. Techniques such as randomly shifting frames within a video clip or strategically cropping video sequences can help the model generalize better to diverse video content. This is especially useful in combating overfitting since the resulting augmented versions of videos contain more variance.
The choice of **pooling method** within the CNN is also particularly impactful when working with videos. Max pooling often focuses on the most important features in a frame, whereas average pooling provides a broader view of the features. The choice of pooling strategy affects the resulting feature representations and ultimately the overall model accuracy. Understanding this relationship between the choice of pooling and the model's performance is vital.
**Batch normalization** often provides greater stability in deeper CNN structures, especially those built for video analysis. By normalizing the input to each layer within the network, it effectively mitigates the phenomenon known as internal covariate shift. This stabilizes the learning process, making the training process more robust and efficient.
In some cases, utilizing **pre-trained models** can be beneficial. Models trained on massive datasets like ImageNet already have a wide range of features learned, making them potentially good starting points for video analysis tasks if they are properly fine-tuned. Adapting them to a video analysis task can significantly accelerate the training process.
It's frequently more efficient to only alter the **output layers** of a pre-trained model rather than retraining the whole network. This method preserves the pre-learned knowledge and allows you to retrain just the last few layers to better suit a particular video analysis task. This is often far more computationally efficient.
The specific **activation function** used within a CNN can also affect the training process. Newer functions like ReLU and its variants tend to produce faster convergence and generally better performance than older options such as sigmoid or tanh. This can be significant as the learning process for more complex models like those used for video analysis can take a very long time.
The **input dimensions** of video frames also play a role in model performance. Different input sizes, along with the associated aspect ratios, can significantly affect how the CNN extracts features from the video. For optimal performance, consistent preprocessing is recommended to maintain consistent input dimensions across the dataset.
For more complex or noisy video datasets, applying **ensemble methods** can be very useful. Here, you train several CNN models separately and then combine their predictions. This approach leverages the individual strengths of each model to produce more accurate and robust classifications.
In essence, training a CNN for video analysis requires a careful consideration of the temporal aspects of video data. Applying techniques like those mentioned above is critical for creating models that accurately identify actions, features, or other patterns that might be present within complex video data. The field is constantly evolving, and keeping abreast of advancements in CNN architectures, training methodologies, and the unique characteristics of video datasets is vital to developing successful applications.
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide - Implementing Real-time Video Frame Classification
Implementing real-time video frame classification pushes the boundaries of CNNs for video analysis. The challenge is to capture both the visual elements within each frame and the sequential nature of the video itself, enabling us to accurately recognize actions and extract meaningful features. This often involves the use of 3D convolutional layers within the CNN architecture, allowing the network to learn from multiple frames simultaneously. We also see the use of hybrid CNN-RNN architectures, where CNNs focus on spatial features and recurrent neural networks (RNNs) handle the temporal dimension.
Effective preprocessing plays a critical role. Video data needs careful preparation to highlight key features and ensure consistent inputs to the CNN. If not carefully done, the CNN may fail to generalize from the training data. This could lead to poor performance. Preprocessing might include resizing frames, normalizing pixel values, or highlighting specific areas within frames to ensure consistency and optimize the CNN's performance.
Moving forward, it's expected that the development of even more efficient algorithms and refined preprocessing techniques will be essential for the future of real-time video analysis. Maintaining robustness and accuracy in these systems, especially across diverse scenarios, will be a significant hurdle that will have to be overcome.
Implementing real-time video frame classification presents a unique set of challenges due to the need for extremely fast processing. To achieve smooth video playback at 30 frames per second, the processing time for each frame ideally needs to be under 33 milliseconds. This requires careful optimization of algorithms and leveraging hardware acceleration to the fullest.
Recognizing that video frames are inherently sequential and hold crucial temporal information has led to the increasing use of methods like 3D convolutions within deep learning frameworks. These 3D convolutions prove to be quite powerful in distinguishing between different actions within videos as they capture the dynamics of movement across multiple frames, offering a substantial improvement over just analyzing each frame individually.
One of the interesting features of video data is the significant redundancy that exists between consecutive frames. A video can be composed of many very similar frames. This is a powerful observation that has led to a variety of approaches like keyframe extraction. Keyframe extraction can dramatically reduce the amount of data that needs to be processed without a significant loss of important information. The consequence of using this type of approach is that it significantly reduces the computational load, which is a major issue in many real-world applications.
During the training process, using techniques called temporal data augmentation can result in models that generalize better. These techniques modify the temporal structure of video clips. Techniques like randomly shifting frames within a clip or carefully cropping sequential segments of the videos can both contribute to increased model performance and help to reduce the risk of overfitting. By introducing additional variation into the training data, the model has a chance to learn broader patterns which allows it to perform better when given new data.
Within CNN architectures, the specific pooling method that's used (whether max pooling or average pooling) can have a surprisingly large impact on model accuracy. While max pooling can highlight the most significant features in a frame which might be useful when classifying distinct actions, average pooling offers a more comprehensive perspective of the frame's features. The choice depends heavily on the problem and how one chooses to represent the information that's important within the dataset.
Batch normalization often helps stabilize the training process by mitigating a phenomenon known as internal covariate shift. This technique basically helps to ensure that the input to each layer of a CNN has a consistent distribution. In CNNs that are particularly complex and especially those that have been built for video analysis, using batch normalization can be critical for achieving fast and reliable training.
One of the most common techniques when working with complex CNN models is transfer learning. It's extremely beneficial in video classification. In this approach, pre-trained models that have been developed using very large datasets (e.g., ImageNet) can be used as the starting point for a new model. Using a pre-trained model can provide a foundation of existing, well-learned features. If the final output layers are then fine-tuned using a dataset more appropriate to a specific video analysis task, it can dramatically accelerate the training process.
The resolution, and even the aspect ratios of input frames, can have a large impact on feature extraction by a CNN. When input frames are consistently resized in a standardized manner, the models perform better because they are able to use features that have a more consistent representation of the visual information across the whole dataset.
One interesting way to improve model performance is to use ensemble methods. In this approach, we train multiple CNN models separately and then combine their results. These multiple models can often capture different facets of the data. The hope is that this will improve the overall accuracy of the classifier. These models that have been trained with different perspectives often generalize better when presented with very diverse video inputs.
The choice of activation function can greatly impact the model training process, particularly in the context of very deep CNNs that are needed for many video processing tasks. While many contemporary activation functions like ReLU often result in faster convergence speeds and better performance, some older functions might lead to unexpected difficulties during training. This highlights the importance of carefully choosing the right components of a CNN for a specific task.
In summary, creating a CNN that accurately classifies video frames in real-time involves considering numerous factors that are beyond simply analyzing static images. A deep understanding of the temporal aspects of video, application of advanced architectural techniques, and smart use of pre-trained models are all critical for building high-performing models. The field is constantly in flux, so staying current on advancements within CNN architectures, new training techniques, and the unique qualities of video datasets is crucial to building successful video analysis systems.
Implementing a Custom CNN in Python for Video Frame Analysis A Step-by-Step Guide - Optimizing CNN Performance and Handling Edge Cases
When implementing a CNN for video frame analysis, optimizing its performance and addressing unusual or unexpected situations (edge cases) are paramount for building a reliable system. Improving CNN speed can involve using specialized hardware, such as FPGAs, which excel at parallel computing, or even leveraging custom instruction sets within processors. Finding the right balance between the model's complexity and available computational power is vital, especially when running the CNN on devices with limited resources (edge computing). Dealing effectively with edge cases is essential for ensuring that the model maintains accuracy and reliability across varied and possibly unpredictable video inputs. As the field continues to advance, constantly reevaluating and adapting optimization and edge case handling strategies will be key to achieving even better performance from CNNs.
Optimizing CNN performance for video analysis involves understanding the unique challenges posed by the temporal nature of video data. For example, 3D convolutions are often employed to capture both spatial and temporal information in each frame, letting the network learn from a sequence of frames rather than just a single frame. This helps models better understand actions and changes across time.
A lot of video data contains a lot of redundant information. Many frames are very similar, so a technique called keyframe extraction can be beneficial. It minimizes computational cost and improves efficiency by only selecting frames that provide unique information, effectively reducing the total data size without losing critical details.
Data augmentation strategies in video analysis often involve manipulating the temporal characteristics of videos. Randomly shifting frames within a video clip or carefully cropping sections can help the model learn patterns across a wider variety of data and reduce overfitting, improving accuracy in a diverse range of conditions.
The choice of pooling method (max pooling or average pooling) significantly affects how information is processed within the CNN, especially in video analysis. Max pooling focuses on the most prominent features, which can be important in distinguishing between different actions in a video. Average pooling, however, presents a broader picture of the features within a frame. The right choice often depends on the specific tasks at hand and how the model needs to represent the data.
Batch normalization can be a key technique in improving performance during the training phase. It stabilizes training and can significantly accelerate convergence, especially with deeper network structures. This is important in video analysis, where training can be computationally intensive.
Interestingly, the size and aspect ratio of the input frames also impacts CNN performance. Keeping input sizes standardized ensures that the visual information is represented consistently across the entire dataset, improving overall feature extraction and model generalization.
Using ensemble methods, where several CNNs are trained separately and then combined, can be a way to boost model performance and build robustness. This approach aims to leverage the unique strengths of each individual network, leading to a more comprehensive understanding of the video data.
The choice of activation functions within the CNN can impact training. Newer activation functions like ReLU often lead to faster convergence rates and better overall performance compared to older alternatives. This is especially important for computationally intensive tasks like video analysis.
Transfer learning can be extremely helpful in accelerating CNN training, particularly with complex video classification. Models pre-trained on very large datasets like ImageNet offer a significant head start by providing a foundation of already learned visual features. These can then be tailored to specific tasks, making the whole training process faster and more efficient.
Real-time video classification presents some particular challenges, particularly given the need to process each frame incredibly fast. To achieve smooth video playback, models often need to process each frame in under 33 milliseconds for a typical video format, which is demanding and requires advanced algorithmic and hardware optimization.
In conclusion, optimizing CNNs for video analysis requires considering a variety of factors, all of which stem from the intrinsic temporal nature of video data. Understanding how to effectively model these temporal aspects using architectural changes, applying clever data augmentation and preprocessing methods, and taking advantage of techniques like transfer learning, can lead to more robust and accurate video analysis systems. The field continues to evolve, and keeping up-to-date on the latest research and trends in CNN architectures and video analysis is crucial for continuing to advance this research.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: