Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction
Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction - Leveraging Bash Variables for Efficient Metadata Parsing
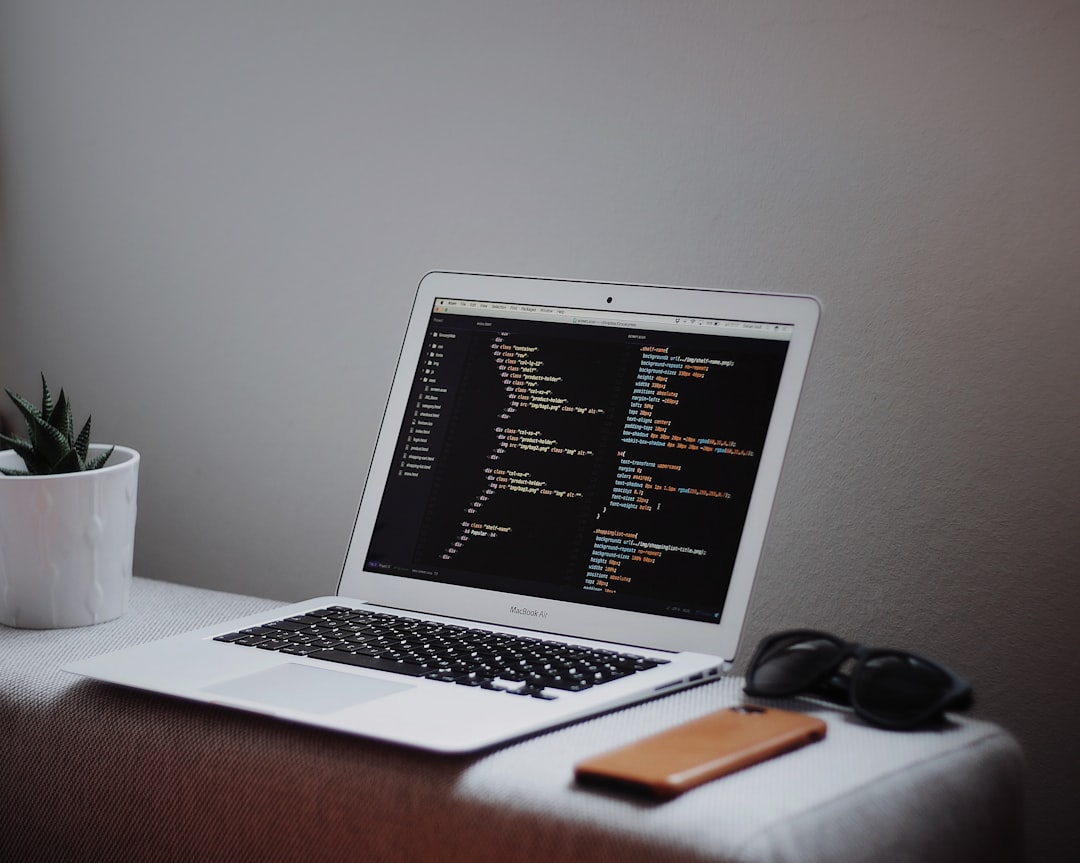
Bash variables can be used to store and manipulate metadata during video processing. This helps with efficient parsing and extraction of relevant information from various data formats, like CSV and JSON. Techniques like using `IFS` (Internal Field Separator) to split data, `read` to assign values to variables, and the `jq` and `awk` commands for processing JSON and CSV respectively, can streamline the entire process. Furthermore, good practices like defining local variables within functions and using descriptive names for variables enhance script readability and minimize the potential for unwanted side effects.
Bash, with its flexible variable handling, offers a surprisingly powerful toolset for parsing metadata. Beyond the basic assignment, Bash provides mechanisms for making our scripts more efficient. For instance, leveraging the `${variable:-default}` construct avoids the need for explicit conditional checks, simplifying code and improving execution speed. We can also exploit the `read` command's ability to populate multiple variables from a single line, which is invaluable when working with structured metadata formats. Parameter substitution, like trimming whitespace or replacing substrings, further enhances script readability while reducing code redundancy.
Associative arrays (available in Bash 4.0 and up) provide a powerful way to organize metadata key-value pairs, making it easier to access and manipulate different metadata attributes within video files. Conditional statements based on variable states, such as `[[ -n $variable ]]`, allow for cleaner control flow, potentially enabling quicker exits from loops or processes. Local variables, declared with the `local` keyword within functions, offer a way to control memory usage by limiting variable scope. This prevents unintended overwrites and improves script maintainability.
Command substitution, achieved using `$(command)`, offers a streamlined approach to capturing command output directly into variables, eliminating the need for temporary files and boosting performance. Bash's diverse set of string manipulation techniques, such as `${string#substring}` and `${string%substring}`, further reduce the need for external tools when parsing and processing metadata.
The distinction between global and local contexts is key when dealing with variables. While variables are global by default, functions provide a way to isolate specific variables, enhancing modularity and simplifying debugging. Lastly, altering the Internal Field Separator (IFS) can significantly impact how data is read and processed. By manipulating IFS, we can efficiently parse complex metadata formats without relying on extensive parsing routines.
While these techniques can be powerful, it's worth remembering that each comes with its own tradeoffs. Experimentation is vital to finding the most suitable approach for your specific metadata extraction task. It's tempting to think of Bash as a one-size-fits-all solution, but it's important to remain mindful of the underlying mechanisms to avoid inefficient practices.
Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction - Streamlining File Input with Command Substitution

The "Streamlining File Input with Command Substitution" subsection delves into the benefits of using command substitution in Bash scripts designed for video metadata extraction. By leveraging `$(command)`, we can directly capture the output of a command within a variable. This eliminates the need for temporary files and streamlines the process, resulting in cleaner, more efficient code. This approach also opens the door for dynamic scripting, enabling complex command nesting. Combined with Bash's array of string manipulation capabilities, command substitution simplifies complex metadata parsing. Ultimately, embracing this technique leads to scripts that are more readable, maintainable, and minimize file-handling complexities.
Command substitution in Bash, achieved using `$(command)`, seems like a straightforward way to capture command output directly into a variable, potentially bypassing the need for temporary files and boosting performance. This technique simplifies the syntax for capturing command output but, more importantly, avoids unnecessary disk I/O. Scripts become quicker and leaner, using less system resources compared to writing to and reading from temporary files.
This efficiency can be further enhanced by combining command substitution with process substitution, allowing a command's output to act like a file for another command. This streamlined approach can significantly improve processing operations on large datasets or batch tasks.
Surprisingly, command substitution handles multi-line outputs with ease. By simply using `$(command)`, the entire output gets assigned to a single variable, eliminating the need for complex parsing steps. This simplicity contributes to leaner, less intricate scripts.
Bash supports nested command substitutions, creating a platform for highly customized output processing. For example, `$(command1 $(command2))` can be used to perform actions that depend on the results of multiple commands, making scripts more versatile.
While powerful, command substitution can be tricky if used excessively or incorrectly. Handling very large outputs can introduce maintenance challenges and performance drops, highlighting the need to carefully consider the size and complexity of the data being manipulated.
One potential pitfall is neglecting whitespace or special characters in command outputs when employing command substitution. An improperly managed output can break scripts, leading to subtle errors not immediately apparent during initial testing.
Parameter expansion within command substitution provides a robust way to manipulate the output dynamically. Techniques like trimming or replacing substrings directly within the substitution expression can save time and reduce the need for additional commands to clean the data.
Command substitution, although powerful, is constrained by the maximum command line length on the system. Trying to process extremely long command outputs may fail, requiring alternative approaches like piping to other commands.
Using command substitution often results in cleaner, more concise code by reducing the boilerplate associated with temporary variables. Scripts become easier to understand, particularly when dealing with intricate data composition and parsing.
The ability to combine command substitution with process control commands like loops and conditionals opens up possibilities for enhancing script efficiency. For instance, using command substitution within `while read` loops can streamline data processing pipelines, creating both powerful and compact scripts.
Overall, command substitution is a valuable tool in the Bash arsenal, but it requires thoughtful implementation to maximize its benefits and avoid potential pitfalls. The key is to understand its strengths and limitations, and to use it strategically as part of a comprehensive approach to Bash scripting.
Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction - Utilizing Associative Arrays for Structured Metadata Storage
Associative arrays, available in Bash version 4.0 and higher, offer a powerful way to organize metadata key-value pairs within video files. This helps manage complex datasets more efficiently by allowing programmers to store and retrieve data using meaningful string keys, rather than just numeric indices. This makes it easier to access and manipulate various metadata attributes. Declaring an associative array using `declare -A` enables the creation of a dictionary-like structure, making the association of metadata keys with their corresponding values more intuitive. You can then populate this structure by reading information from a structured file, parsing each line, and assigning values to the corresponding keys. These arrays are particularly beneficial for tasks that rely on specific identifiers, which are common when dealing with video metadata extracted from diverse formats.
Associative arrays, a feature available in Bash 4.0 and above, offer a robust way to manage metadata within video processing scripts. Their ability to use strings as indices, allowing for key-value pair storage, greatly enhances organization and clarity compared to traditional arrays. Unlike traditional arrays, where indices are limited to integers, associative arrays embrace flexibility by accepting strings containing dots, underscores, or hyphens as keys, allowing for more meaningful and descriptive metadata labels.
One of the key advantages of associative arrays is their dynamic resizing capability. This avoids the need for manual memory management, proving beneficial when dealing with unpredictable amounts of metadata. However, it's crucial to acknowledge that their order is not guaranteed, which can be a drawback when metadata sequence is vital. Accessing values within associative arrays might also introduce a minor performance penalty compared to indexed arrays due to their hashing mechanism, a factor to consider when processing extensive metadata sets.
Despite this potential performance trade-off, associative arrays significantly improve code readability and maintainability. Their clear syntax for retrieving values makes scripts easier to understand, especially during debugging. They also simplify collaboration by facilitating the transfer of structured metadata between scripts.
However, not all tools and commands within the Bash ecosystem inherently support associative arrays, potentially leading to compatibility challenges when integrating with external scripts or tools designed for traditional arrays. Furthermore, their reliance on a specific Bash version adds a dependency to scripts, necessitating system compatibility checks to avoid encountering unexpected errors.
Ultimately, while associative arrays present a compelling solution for managing structured metadata in Bash scripts, they should be implemented thoughtfully, considering both their advantages and potential limitations. Balancing their convenience with potential performance implications, while being mindful of compatibility issues, is crucial for effective integration into video metadata extraction workflows.
Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction - Optimizing Performance with Process Substitution Techniques
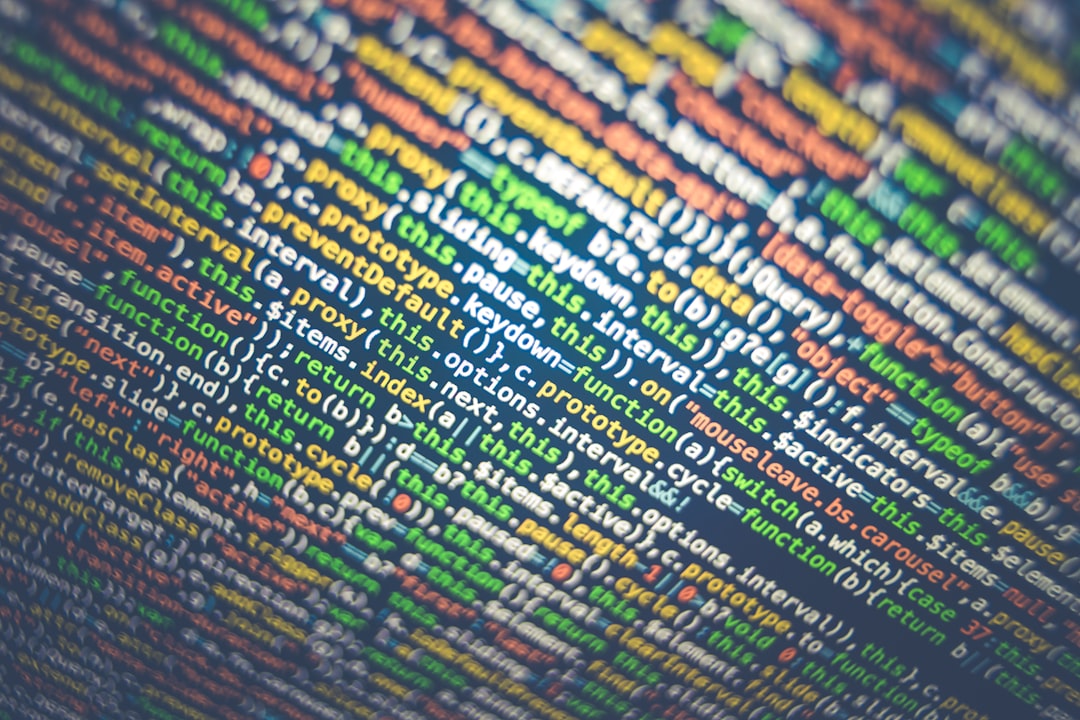
Optimizing performance in Bash through process substitution techniques offers a potent way to boost the efficiency of file-to-variable reading strategies, particularly within the context of video metadata extraction. Process substitution essentially allows you to treat the output of a command as if it were a file, making it a direct input for another command. This avoids the overhead of creating and using temporary files, leading to a noticeable performance improvement. Beyond that, process substitution also makes your scripts cleaner and easier to manage by simplifying the flow of data within them.
However, it's crucial to test and benchmark the performance of your scripts before implementing process substitution, as the benefits can vary depending on the specific task at hand. Also, bear in mind that process substitution works asynchronously, which can sometimes be a challenge to manage effectively, particularly when dealing with complex operations. Despite these potential hurdles, process substitution is a valuable tool that can be mastered to build robust and efficient Bash scripts for a wide range of metadata extraction tasks.
Process substitution in Bash offers a potentially valuable tool for optimizing script performance in video metadata extraction tasks. It effectively streamlines command chaining by treating the output of a command as a file, thereby eliminating the need for temporary files and simplifying the script syntax. This can lead to faster execution times, particularly when handling large datasets.
However, its advantages are not without caveats. One concern is that process substitution, being dependent on subprocesses, can sometimes introduce performance overheads, particularly in complex nested command scenarios. This potentially limits its usefulness when dealing with extremely intricate operations or environments with limited resources.
On the other hand, its resource efficiency can be a significant benefit. Because it leverages kernel features rather than creating separate files, it minimizes system resource consumption, particularly in scenarios where resources are constrained. This makes it a compelling choice when working with substantial datasets or environments with limited hardware.
Another advantage is its flexibility. Process substitution can be used for both input and output operations, which grants greater flexibility in data processing tasks like sorting, filtering, or manipulating live data streams. This offers a convenient alternative to file-based approaches.
However, integrating process substitution can add a layer of complexity to error handling, as it introduces additional nesting and interdependencies between commands. This can make diagnosing issues more challenging and requires a more robust error-checking strategy.
Further limitations exist with regards to compatibility. Older operating systems and shells may not support process substitution, necessitating compatibility checks and possibly limiting the reach of scripts that leverage this feature.
While potentially powerful, its syntax can be less intuitive for novice Bash users, leading to misunderstandings regarding how input and output are managed. This can result in unexpected behavior and necessitates comprehensive documentation within the script.
Finally, it's essential to understand the internal buffering behavior of process substitution. This can be influenced by factors such as the size of the data being processed and the interaction between commands, potentially leading to variations in performance.
In conclusion, process substitution offers several benefits for video metadata extraction tasks, but it's crucial to carefully consider its limitations, including potential performance overheads, increased error handling complexity, compatibility concerns, and potential confusion for users. When implementing this technique, it's essential to weigh these tradeoffs against its strengths in order to optimize its effectiveness for your specific needs.
Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction - Implementing Error Handling and Logging in Bash Scripts
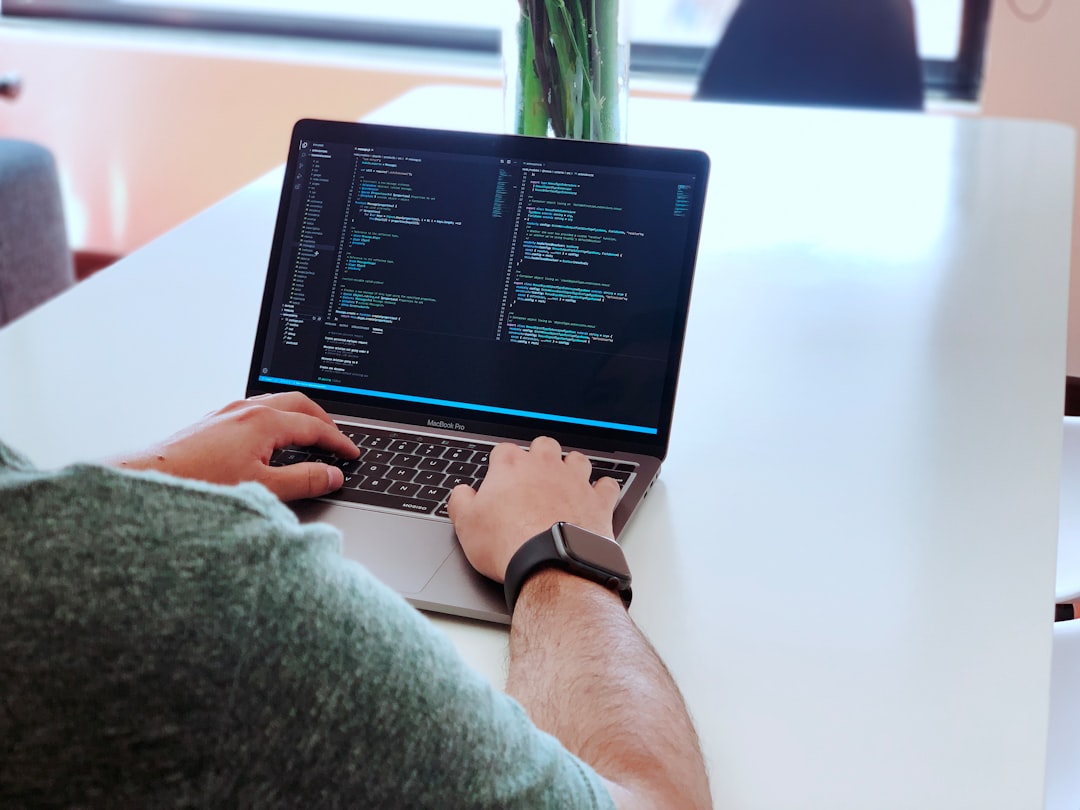
Implementing robust error handling and logging within your Bash scripts is crucial, especially when dealing with complex processes like video metadata extraction. You want to ensure that your scripts can handle unexpected issues gracefully and provide useful information to help you pinpoint the root cause of any problems.
First, you should familiarize yourself with the standard way Bash scripts indicate success or failure - by returning a numerical exit code. A zero exit code means the command executed successfully, while any other value indicates an error occurred. You can check the return code of a command using `$?`.
To proactively stop execution if an error occurs, you can incorporate the `set -e` directive within your script. This causes the script to exit immediately if any command returns a non-zero exit code. This is vital to prevent further erroneous commands from executing and compounding the problem.
The `trap` command provides a powerful mechanism for handling various signals, including errors. You can use it to define custom actions when specific events occur, allowing for graceful handling of unexpected situations like interruptions, invalid input, or resource exhaustion.
Effective logging practices are equally important. For debugging purposes, you can use the `bash -x` option to print each command as it executes, along with expanded variables. This helps in tracing the flow of your script and understanding the values involved at each step. You can also use output redirection to store script execution details in a log file for later review.
Finally, tools like ShellCheck can help you identify potential issues within your scripts, highlighting best practices and areas that might require attention. A structured approach to logging, such as writing logs to a centralized location like `syslog`, can improve script maintainability and ensure you don't lose crucial debugging information.
In conclusion, a solid understanding of error handling and logging techniques can greatly enhance the reliability and maintainability of your Bash scripts. While they may seem like secondary concerns at first, these techniques are crucial for creating scripts that can handle challenging situations, and provide valuable debugging information when errors arise.
Error handling and logging in Bash scripts are crucial for building robust and maintainable code, especially in the context of video metadata extraction. These tasks involve processing potentially large datasets, and encountering errors is inevitable. Efficiently handling these errors allows engineers to swiftly identify and address problems, leading to quicker development cycles.
The `set -e` option, for instance, halts script execution upon any command failure. While this helps catch errors early, it can also be detrimental if not carefully managed in complex workflows. It's a tool with potential for both good and bad outcomes. Similarly, using `set -x` for debugging provides invaluable insights into the script's execution path, showcasing each command and its execution. It's a vital tool for troubleshooting intricate variable manipulations involved in video metadata extraction.
Bash allows for redirecting errors to separate files using `2>`, helping to isolate errors from standard output for easier review. Additionally, `trap` allows for custom error messages based on exit status codes. This helps create informative feedback during debugging. These mechanisms allow for creating structured logs that comprehensively track script execution, including any errors. Such logs are essential for tracing issues and pinpointing their origins in complex metadata extraction scenarios. However, it's crucial to be mindful of the performance overhead that logging can introduce. Striking a balance between robust error monitoring and maintaining efficient performance is key.
While the `set -e` option can be useful, it can also interrupt execution unnecessarily. The use of conditional logging can help mitigate this by logging errors only when certain conditions are met, streamlining the log output and filtering out noise. This approach helps in focusing on relevant issues, particularly in scenarios with extensive metadata processing.
Error handling and logging techniques in Bash play a pivotal role in the successful implementation of video metadata extraction scripts. Each tool offers its advantages and drawbacks, demanding careful consideration and strategic implementation to achieve a balance between script robustness, performance, and maintainability.
Efficient File-to-Variable Reading Techniques in Bash for Video Metadata Extraction - Integrating External Tools like FFprobe for Enhanced Extraction
Integrating external tools like FFprobe into Bash scripts opens up a whole new level of capability for extracting video metadata. FFprobe, a powerful command-line tool, lets you delve into the details of multimedia files, pulling out metadata in formats like JSON, CSV, or XML. You can use FFprobe to target specific attributes like video duration, resolution, and bitrate, making your Bash scripts much more efficient.
The beauty of this integration lies in the ability to directly store extracted metadata into Bash variables, streamlining your extraction process and making your scripts much more user-friendly. But remember, relying on external tools introduces a layer of complexity. You'll need to fully grasp both Bash and FFprobe to optimize your extraction workflow and avoid performance issues. It's a balancing act, but a well-integrated FFprobe can significantly boost your video metadata extraction capabilities.
FFprobe is a handy command-line tool bundled with the FFmpeg suite. It excels at analyzing multimedia files and extracting metadata without needing to fully decode them. This makes it efficient for quickly getting video information.
One neat feature of FFprobe is its ability to output data in various formats like JSON and XML. This flexibility is great for integrating with other tools and scripts, including Bash scripts, making for smoother interoperability.
You can extract tons of information with FFprobe in a single command, including codec details, bitrates, frame rates, and even stream information. This is useful when you're working with large collections of video files and don't want to check them all manually.
The speed of FFprobe is impressive. It often returns metadata in seconds, even for large files. This is crucial in workflows where time is of the essence, like automated video processing pipelines.
Interestingly, FFprobe can be used to analyze real-time media streams, giving you immediate feedback on the data being processed. This means it can help diagnose issues with streaming media setups or during large batch processing.
FFprobe lets you customize the output by specifying which metadata fields you want to extract. This selective extraction feature lets you tailor your Bash scripts for efficiency and focus on the relevant information.
Another great thing about FFprobe is that it can handle both video and audio streams, making it a single point of reference for different media types. This simplifies the extraction process, especially in projects that deal with both video and sound.
FFprobe can also analyze a variety of file formats beyond just the typical video and audio containers, making it great for handling a range of files. This adds versatility to your media handling capabilities.
It can even detect corruption in media files, providing valuable information for troubleshooting efforts. This diagnostic feature adds a layer of reliability to metadata extraction processes.
Since FFprobe works independently of the input file’s codec or container format, your Bash scripts remain robust and versatile, capable of handling a wide range of multimedia files without needing to know their specifications beforehand.
More Posts from whatsinmy.video: