Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation
Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation - Understanding the Kalman Filter Fundamentals for Video Motion Estimation
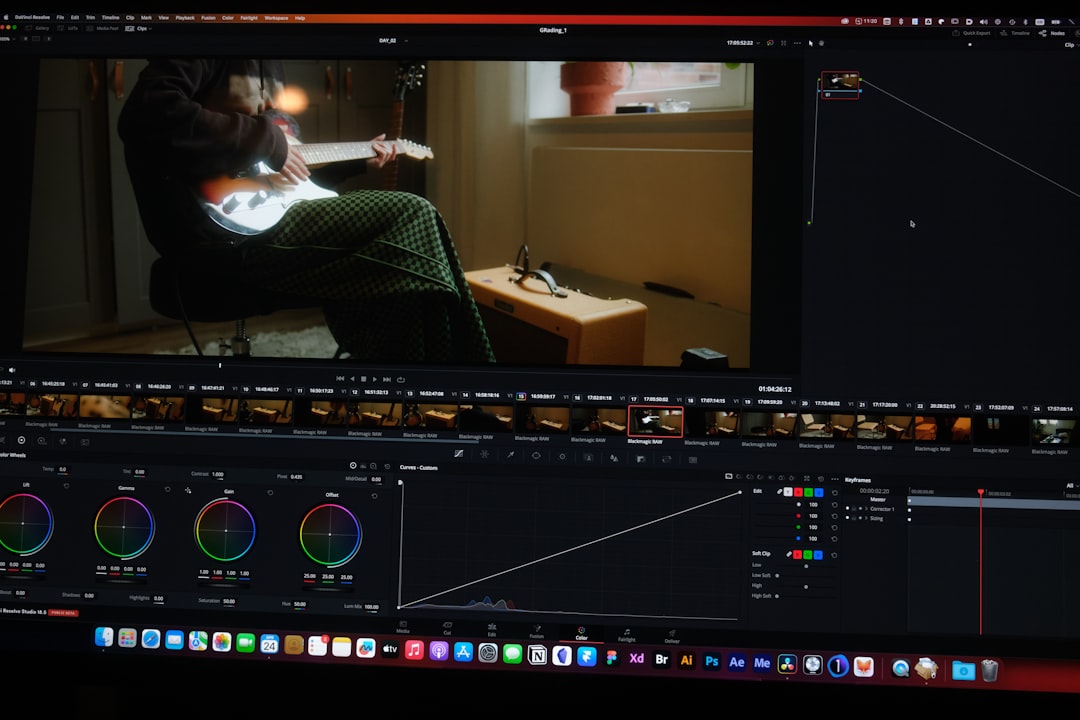
The Kalman filter's core principle revolves around continuously refining estimates of a system's state by blending predictions with incoming measurements. This iterative process proves particularly valuable when dealing with dynamic systems prone to noise and uncertainty, which are inherent characteristics of video sequences. Essentially, it operates by fusing a mathematical representation of the movement with the actual data acquired from sensors. This fusion allows for the precise tracking of object motion and extraction of important features within video frames. Achieving reliable results relies on careful consideration of starting conditions, such as a well-defined initial system state, and a thorough understanding of the inherent noise impacting the measurements. This aspect highlights the importance of carefully characterizing the system's inherent randomness or uncertainties. The filter's predictive capabilities extend beyond basic motion tracking into more advanced computer vision tasks, making it a versatile tool. Furthermore, its implementation doesn't typically require overwhelming computational resources, thus enabling its practical use in various applications.
The Kalman filter, initially conceived for aerospace applications, has proven remarkably adaptable, finding its way into diverse fields including video processing. Its recursive structure enables it to efficiently combine measurements over time, making it a good fit for real-time video tasks. However, video motion, often non-linear, presents challenges for the standard Kalman filter. Variants like the Unscented Kalman Filter are frequently utilized to address this intricacy.
The core of the Kalman filter lies in linear algebra and probability theory, surprisingly leading to robust results even when presented with noisy or incomplete visual data. Beyond motion tracking in video, its applications extend to robotics, economics, and beyond, demonstrating its broad utility. A key assumption of the basic Kalman filter, Gaussian noise, can introduce inaccuracies in scenarios like video where real-world noise distributions often deviate from this ideal.
Parameter tuning in Kalman filters is critical, particularly in selecting the covariance matrices for process and measurement noise, which heavily influence performance. Extensive experimentation is frequently necessary to optimize these settings. The simplicity of the filter equations can obscure the underlying complexity of the optimization procedure. Ensuring filter convergence to the true state over time, especially in dynamic conditions, requires careful tuning and adjustments.
Integrating Kalman filtering with machine learning holds promise for enhancing robustness against abrupt motion changes or occlusions, highlighting a confluence of classical and contemporary approaches. While powerful, the Kalman filter isn't without vulnerabilities. Filter divergence, for instance, can occur due to poorly chosen initial conditions or noise parameters, underscoring the need for careful implementation and a discerning approach.
Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation - Setting Up the C++ Environment for Kalman Filter Implementation
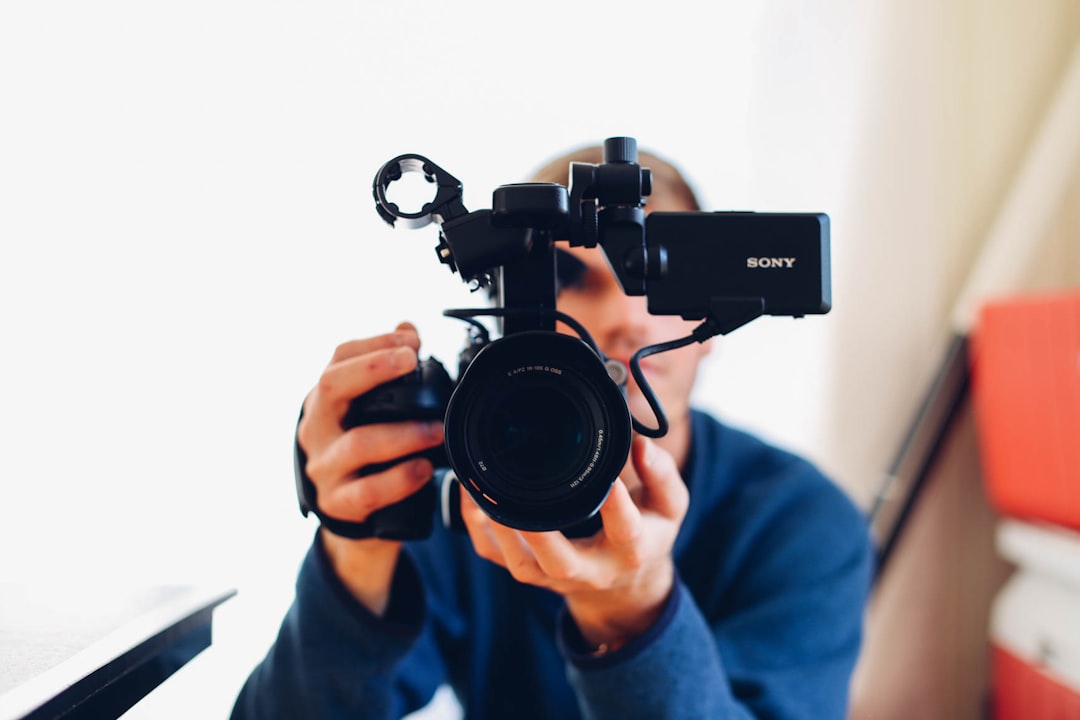
To effectively implement a Kalman filter in C++ for video motion estimation, we need to establish a proper development environment. This involves setting up the necessary libraries and tools for compiling and running the C++ code that will realize the Kalman filter's functionality. A fundamental aspect of this setup is defining the filter's initial state. This often involves initializing a state vector and a covariance matrix, using functions tailored to this task. Correctly defining these starting conditions is vital for filter performance.
Furthermore, the implementation necessitates defining the mathematical functions that represent the system's behavior and how it relates to measurements. These functions, along with their corresponding Jacobians, form the backbone of the filter's update process. Understanding the impact of measurement noise, in particular, its variability, is critical for ensuring that the filter effectively estimates the true state of the system from the sensor data.
While implementing a Kalman filter from scratch can be challenging, we can utilize existing implementations and examples found online. This provides a practical starting point and allows one to explore the core mechanics of the filter, its core equations, and its structure. This understanding can then be further leveraged for more complex scenarios such as implementing the Extended Kalman Filter when dealing with nonlinear systems. A proper understanding of the underlying mechanics is crucial for successful implementation and practical application to the intricacies of video motion. While starting with basic implementations provides a firm footing, it is crucial to delve into the nuances and subtleties of Kalman filter theory to apply it correctly for practical tasks such as motion estimation in videos.
Setting up a C++ environment for Kalman filter implementation involves several crucial considerations. The choice of compiler, like GCC or Clang, can significantly impact performance, particularly when dealing with high-frame-rate video data. Optimizing compiler settings can be crucial to achieving real-time processing.
Libraries like Eigen can simplify development by handling linear algebra operations, a cornerstone of the Kalman filter. Using established libraries can reduce errors and improve code maintainability, especially in complex computations. It's worth exploring the possibilities of parallelization, as the structure of the filter, particularly during measurement updates, may lend itself to parallelization techniques. This could lead to significant performance gains, especially when processing large amounts of data from video streams.
Accurately modeling noise is a critical aspect that often gets overlooked. While Gaussian noise is a common assumption, it might not reflect the true noise distribution in many video applications. Developing methods to characterize the actual noise in your data could be beneficial in refining filter accuracy. Real-time video processing necessitates a strong awareness of memory management. C++ allows fine-grained control over memory allocation, which helps to minimize latency and maintain the real-time aspect of the application, otherwise known as lag.
Selecting the appropriate floating-point precision can influence the numerical stability of the filter, particularly when tracking motion over extended periods. Understanding the difference between single and double precision in C++ is vital for achieving reliable results. Robust debugging tools, like Valgrind and GDB, play a key role in uncovering memory leaks or performance bottlenecks. A well-structured testing process is vital for a robust implementation.
Having a well-defined initial state can assist in faster convergence and mitigate the risk of divergence. Implementing methods for dynamically estimating the initial state from previous data or employing heuristics can be beneficial to overall filter robustness. C++ template metaprogramming offers flexibility in crafting reusable filter components while preserving performance and type safety. This can be advantageous in applications where you require a tailored filter.
Finally, integrating the Kalman filter with external systems like sensors and data streams presents a significant challenge. Synchronizing data acquisition and processing is crucial for ensuring data integrity and filter performance, vital in sensitive applications where reliability is paramount. In conclusion, successfully implementing a Kalman filter in C++ demands a blend of meticulous programming practices and deep understanding of the filter's behavior within the context of specific application challenges, like those found in video motion estimation.
Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation - Designing the State and Measurement Models for Video Processing
Designing the state and measurement models is a pivotal step when using a Kalman filter for video processing. These models essentially act as the filter's framework, enabling it to accurately track and estimate object motion within video sequences. The state model outlines the inherent dynamics of the system being tracked, essentially defining how it moves and changes over time. The measurement model, on the other hand, acts as a bridge between the real-world sensor data and the state model. It dictates how the filter interprets and uses sensor readings to refine its motion estimates.
However, the real world is far from perfect. Video data often contains noise and imperfections which deviate from the ideal, simplifying assumptions that often underpin standard Kalman filters. This deviation can affect accuracy in tracking movement. Therefore, ensuring these models can properly handle these uncertainties and adapt to noisy data is crucial for the filter's effectiveness. Furthermore, a good state and measurement model can significantly impact the filter's suitability in different domains, ranging from autonomous robot navigation to detailed video analysis. Without a thoughtful approach to these models, the Kalman filter can be prone to estimation errors and ultimately unreliable. Successfully applying the Kalman filter for video motion estimation rests on a solid understanding of how these models interact and how they can be tuned for a particular video task.
The Kalman filter, initially developed for aerospace applications like navigation and guidance, demonstrates its origins in demanding, high-precision contexts. This highlights its mathematical sophistication built upon linear algebra and statistical theory, making it well-suited for handling complex, noisy, and dynamic systems common in many real-world scenarios, especially when dealing with things like video data.
When designing the state vector within the Kalman filter, a researcher or engineer must carefully consider the system's dynamics and measurement characteristics. An ill-defined state can lead to flawed interpretations, underscoring the importance of modeling states accurately. In contrast to some filters that require perfectly synchronized measurements, Kalman filters can effectively process asynchronous data, a particularly useful characteristic when processing video streams from multiple, potentially unsynchronized sources.
However, the conventional Kalman filter assumes linearity, which can be a major limitation in video processing due to the often non-linear nature of motion. To address this, variants like the Extended Kalman Filter (EKF) or the Unscented Kalman Filter (UKF) are typically employed, showing the need for adaptation in real-world application. Covariance matrices within the Kalman filter, representing the uncertainty in the system and measurements, play a vital role in influencing the filter's response to noise. Their careful definition is crucial for optimal performance, particularly in real-time video processing where responsiveness is key.
Despite its seemingly simple equations, optimizing the Kalman filter's behavior is a complex task. Minor adjustments to the covariance matrices can result in vastly different filter performance and convergence characteristics. This illustrates the intricate relationship between parameter selection and desired filter behavior. Furthermore, effectively integrating the Kalman filter with various sensors or data streams requires careful synchronization and robust error handling. This interconnectedness is crucial to ensure that the filter receives accurate and timely data, enabling accurate state estimations.
The selection of floating-point precision (single vs. double) is another important consideration, influencing numerical stability. Subtle errors in floating-point operations can accumulate, leading to substantial drifts in long-duration tracking tasks, which can be especially important in video applications where processing continues over extended periods. Interestingly, the structure of the Kalman filter's update equations potentially allows for parallelization. This offers exciting possibilities for increasing performance, particularly crucial in real-time video applications where speed is vital. However, parallelized implementations add another layer of complexity to the design. All of these details suggest that using Kalman filters can be tricky even if the core math is relatively simple.
Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation - Implementing the Prediction Step in C++ for Motion Forecasting
Within the framework of a Kalman filter designed for video motion estimation, the prediction step takes center stage in forecasting future states. This prediction relies on a predefined dynamic model, often expressed using differential equations, to describe the system's movement patterns. A key aspect is that, during this stage, the inherent noise associated with the system's dynamics is momentarily ignored. This approach facilitates the generation of smoother, more refined predictions.
However, attaining optimal performance during the prediction step demands meticulous tuning of parameters like the covariance matrices. These matrices significantly influence how the filter reacts to any discrepancies between the predicted states and the actual measurements acquired from the video stream. Video motion itself can be quite challenging, exhibiting frequent non-linear behaviors that can easily throw off simpler tracking methods. It's within this context that the prediction step assumes paramount importance for maintaining reliable and accurate tracking.
The true power of the Kalman filter shines through in its ability to harmonize these predictions with the stream of real-time sensor data. This integration allows for a more refined and accurate forecasting of motion within video sequences, particularly valuable when dealing with situations involving continuous change and uncertainty. It's through this delicate balance of prediction and measurement that the Kalman filter proves its worth for video motion analysis.
Implementing the prediction step within a Kalman filter in C++ for motion forecasting involves a nuanced understanding of the filter's behavior within the context of real-world video data. While the core concepts are relatively straightforward, the practical implementation presents several interesting challenges.
For instance, the mathematical model we use to represent the system's dynamics might not perfectly capture the intricate movements found in video sequences. These discrepancies can result in tracking inaccuracies. It becomes essential to refine the model over time, adapting it to the specific motion characteristics observed in the video stream.
Moreover, the influence of noise covariance matrices, frequently overlooked, can significantly impact the filter's performance. Even minor adjustments to these matrices can lead to dramatic changes in how the filter converges, affecting the accuracy of our motion estimates. Finding the right balance in their tuning is crucial.
Adapting the Kalman filter through techniques like adaptive filtering can improve its predictive power. This approach enables the filter to autonomously adjust its parameters based on the observed data, making it more resilient to unexpected shifts in motion patterns, such as rapid object accelerations. This ability is highly beneficial in real-time applications where motion can be erratic and unpredictable.
One noteworthy characteristic of the Kalman filter is its capacity to handle asynchronous measurements. This means that it can readily integrate data from multiple camera feeds that are not perfectly synchronized, which is essential when tracking movement across a complex scene. This capability allows for more robust and comprehensive motion estimation by leveraging information from various perspectives.
The selection of floating-point precision (single or double) during the implementation can have a subtle yet crucial impact on estimation accuracy over extended periods. Small numerical errors inherent in single-precision calculations can accumulate, potentially leading to significant deviations in the long run, especially in extended tracking tasks common in video analysis.
Although the Kalman filter's equations are relatively simple, the implementation details can become surprisingly complex. Fine-tuning a multitude of parameters and seamlessly integrating the filter with other parts of a video processing system can introduce unexpected challenges.
Interestingly, blending the Kalman filter with modern machine learning approaches offers a pathway toward more robust motion forecasting. Combining classical filter techniques with machine learning can help to overcome some limitations of the basic Kalman filter, especially in the face of non-linear motion and sudden changes in the video scene. This fusion of older and newer methods holds great promise.
The structure of the Kalman filter, particularly during measurement updates, offers opportunities for parallelization. This has the potential to dramatically improve performance, making the approach more suitable for high-frame-rate videos. However, introducing parallelism adds complexity to the implementation, with new issues like synchronization needing to be handled carefully.
In many real-world scenarios, we encounter noise that doesn't follow the idealized Gaussian distribution assumed by the standard Kalman filter. This departure from the ideal necessitates adaptations. The Unscented Kalman Filter, for instance, can handle more complex noise distributions, offering improved estimations in challenging situations.
Finally, achieving optimal performance from a Kalman filter depends heavily on the accuracy of the initial state estimates. Poorly initialized filters can either converge very slowly or, worse, diverge completely. Ensuring proper initialization strategies is critical for reliable and efficient motion forecasting in real-world video analysis tasks.
In summary, successfully implementing the prediction step in a C++ Kalman filter for video motion forecasting requires a careful balancing act between the inherent simplicity of the filter's core math and the intricate complexities of real-world applications. The seemingly straightforward filter can become quite complicated, highlighting the need for a thoughtful and pragmatic approach.
Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation - Coding the Update Step to Incorporate New Measurements
The update step in the Kalman filter is where we integrate new measurements into the system's state estimation. This is done by using a measurement matrix that essentially translates the raw sensor data (like from a camera in video processing) into a form that can be compared with the filter's predicted state. The key to this process is the Kalman gain, a value that decides how much emphasis to give to the prediction versus the new measurement. Finding the right balance between these two sources of information is critical for accurate state estimation.
One issue that can pop up, particularly in video analysis, is the often non-linear nature of object motion. The Kalman filter in its basic form assumes linear systems, which can lead to problems if the real-world motion doesn't perfectly match this assumption. This highlights the need for careful implementation and, potentially, modifications to the update step to address the specific behavior of video motion. Getting this step correct is essential because a well-implemented update step contributes significantly to the filter's ability to accurately track and estimate object motion within video frames, boosting both reliability and robustness.
The Kalman filter's update step is where new measurements are integrated, allowing the filter to adapt and refine its state estimates in response to changes in the system's behavior. This dynamic adaptation is especially important for video motion estimation, where motion patterns can change rapidly.
The update process isn't about simply discarding predictions; rather, it involves a careful weighting of predictions against actual measurements, a principle derived from Bayesian probability. This intelligent combination of predictions and measurements results in robust estimates, even when measurements are noisy or uncertain.
However, a significant challenge arises from designing the measurement model. It's through this model that sensor data impacts the state estimates, and a poorly designed one can introduce substantial errors into the filtering process. It's critical to validate these models against real-world data to minimize this risk.
A fascinating aspect of the update step is its reliance on linear algebra, where the Kalman gain plays a central role. The Kalman gain dictates how much weight is given to new measurements relative to predictions, directly impacting the filter's performance, especially in dynamic video environments. This gain is where we see the "fusion" in action.
The update step's behavior can be very sensitive to the choice of covariance matrices, particularly those associated with measurement noise. Finding the optimal values often requires extensive experimentation, a time-consuming but essential step for reliable filtering. We're essentially trying to match a mathematical model to our video data.
In fact, assuming a constant measurement noise matrix might not always be realistic. Noise characteristics can vary within real-world video applications, so adaptive approaches where the noise covariance can change based on the situation can prove crucial in handling unpredictable scenarios.
The update mechanism has the capability to process multiple measurements at once, enhancing robustness when tracking objects in diverse conditions. This is particularly beneficial in video tracking where sudden motion changes or occlusions can disrupt tracking.
While the Kalman filter typically assumes Gaussian noise, a common simplification, real video sequences often have noise that deviates from this ideal. This necessitates investigating alternative filter formulations like the Unscented Kalman Filter (UKF), which can accommodate more complex noise patterns.
While the Kalman filter's update equations are conceptually simple, practically implementing them in a real-time system can be tricky. We need to focus on optimizing performance and managing computational resources, especially when dealing with high-resolution video data, where the sheer volume of data adds pressure.
Lastly, monitoring the filter's performance after the update step using techniques such as residual analysis can provide valuable insight into the system's behavior, allowing for swift adjustments to the filter model if necessary. This proactive approach helps ensure that the Kalman filter remains effective even as conditions change, maintaining accuracy over time.
Implementing a Kalman Filter in C++ A Practical Approach for Video Motion Estimation - Optimizing Performance and Handling Real-Time Video Data
When dealing with video data in real-time, optimizing performance and efficiently managing the stream of information are paramount for accurately estimating motion. The Kalman filter, with its ability to adapt to noisy and unpredictable situations, offers a powerful tool for tackling this challenge. Optimizing performance often involves leveraging predictive modeling to anticipate future movements and incorporating adaptive filtering methods that allow the filter to dynamically adjust to changing motion patterns within the video. Furthermore, computational techniques like parallelization can greatly accelerate the processing, making it feasible to handle high-frame-rate video streams that generate significant data. However, maintaining a balance between the filter's prediction steps and the incorporation of new measurements, particularly when video motion isn't perfectly linear, remains a delicate challenge. This necessitates careful tuning of noise models and the covariance matrices to ensure that the filter accurately tracks movement and provides reliable results. Successfully navigating these complexities is vital for the effective application of the Kalman filter in real-time video motion estimation.
When we delve into optimizing the performance of Kalman filters for real-time video data, we uncover several interesting facets that can significantly impact our results. Firstly, the Kalman filter's inherent computational efficiency, which scales linearly with the number of measurements, is a significant advantage for real-time processing of high-resolution video. However, relying on its core assumption of Gaussian noise can create challenges. In many real-world video settings, the noise distributions deviate considerably from this idealized Gaussian, leading to potential inaccuracies in motion tracking. This underscores the importance of considering alternative filtering methods, such as the Unscented Kalman Filter, that can accommodate more complex noise characteristics.
Further complicating the picture is the dynamic nature of video motion. Often, objects accelerate and decelerate, requiring models that are more flexible than simple constant state transition models. Adapting our models to better reflect observed motion dynamics is vital for reducing lags and estimation errors. Interestingly, the structure of the Kalman filter suggests avenues for performance enhancement through parallel processing. The ability to independently update the state for each measurement creates opportunities to use multi-core processors, offering potential for substantial speed increases, especially when handling high-frame-rate video.
However, it's crucial to be aware that the Kalman filter is very sensitive to initial state estimates. Poor initialization can lead to slow convergence or even filter divergence, rendering it unreliable. More advanced initialization strategies, perhaps leveraging prior data or even heuristics based on machine learning, could greatly improve the reliability of our results. In situations with irregular or missing measurement data, implementing techniques like "measurement filling," where multiple measurements are utilized concurrently, can maintain accuracy and robustness in object tracking.
Another important consideration is the dynamic nature of the covariance matrices. Adapting these matrices in real-time allows the Kalman filter to adjust to changing noise characteristics, ultimately boosting performance in a more realistic video environment. The video frame rate, itself, can dramatically influence how the Kalman filter performs. High frame rates, while enhancing visual fidelity, demand more frequent updates, leading to an increased computational load. This can push us toward exploring optimizations or even hardware acceleration.
Importantly, any errors introduced during the prediction step propagate through subsequent estimates. This means keeping a close eye on prediction accuracy is essential. Methods like residual analysis can help us determine if our assumptions about the video data and the underlying motion are valid and offer opportunities to adjust the model if they stray from reality.
Finally, a very promising avenue for future exploration is the integration of machine learning with the traditional Kalman filter. Combining these methods can help us adapt to abrupt changes or occlusions in video, adding layers of robustness to the estimation process. The resulting blended approach offers a powerful way to overcome some of the limitations of the standard Kalman filter for complex motion estimation tasks. In essence, while the core Kalman filter equations may be relatively simple, successful implementation in real-world video scenarios demands a careful and adaptive approach to address a multitude of factors, from noise and motion to computational resources and integrating with other methods.
More Posts from whatsinmy.video: