Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024 - Setting Up Python ElementTree for Video XML Processing
To effectively work with video metadata in XML format, Python's ElementTree library is invaluable. You begin by importing the library using `import xml.etree.ElementTree as ET`. This opens the door to a wide range of tools for XML manipulation, including parsing, traversing, and modifying XML documents. You can handle XML data sourced from files or directly from strings, giving you flexibility. The tree-like organization of XML makes it intuitive to navigate and change the content, particularly with nested elements. However, robust code needs to anticipate potential problems during XML processing. Building in error handling helps maintain the stability of your video metadata management code, which is vital for smooth development.
1. Python's ElementTree (ET) is a built-in module, meaning you don't need any external libraries just to get started working with XML. It's a convenient choice when you need to get going quickly, for example, when handling video metadata files.
2. To start using ElementTree, just import `xml.etree.ElementTree` as `ET`. Pretty straightforward. It exposes functions that handle the core operations involved in reading and writing XML.
3. ElementTree provides a set of functions to work with XML data including basic parsing operations (reading XML from files or strings), navigating the hierarchical structure of an XML document, getting specific data, modifying the XML tree, and managing namespaces.
4. When you need to load data from an XML file, you can use the `ET.parse()` function. If you have XML data in a string, `ET.fromstring()` will help you parse it directly.
5. The `tree.getroot()` method will retrieve the top-level element of the XML tree. This allows you to jump directly into the root, making starting point for exploration clear.
6. Adding child elements to the XML tree and then setting their text content or attributes is very simple with ElementTree.
7. XML files can sometimes be a bit unpredictable. Ensuring that your code handles potential errors and doesn't crash is important. Consider the ways things can go wrong and create robust error handlers in your code.
8. The hierarchical tree-like structure of XML is the core concept of ElementTree. It reflects how XML organizes data. This allows for very easy way to navigate and change nodes in the XML.
9. XML is flexible in what data it can hold. It can manage diverse information, making ElementTree a versatile option for lots of processing tasks. You can use it for things like processing audio metadata, subtitle information or even video caption data.
10. You might use ElementTree to read information from an XML file, output specific elements, or construct whole new XML documents with a predefined structure. The possibilities are dependent on your needs.
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024 - Creating Basic Video Metadata Structure with Element Objects
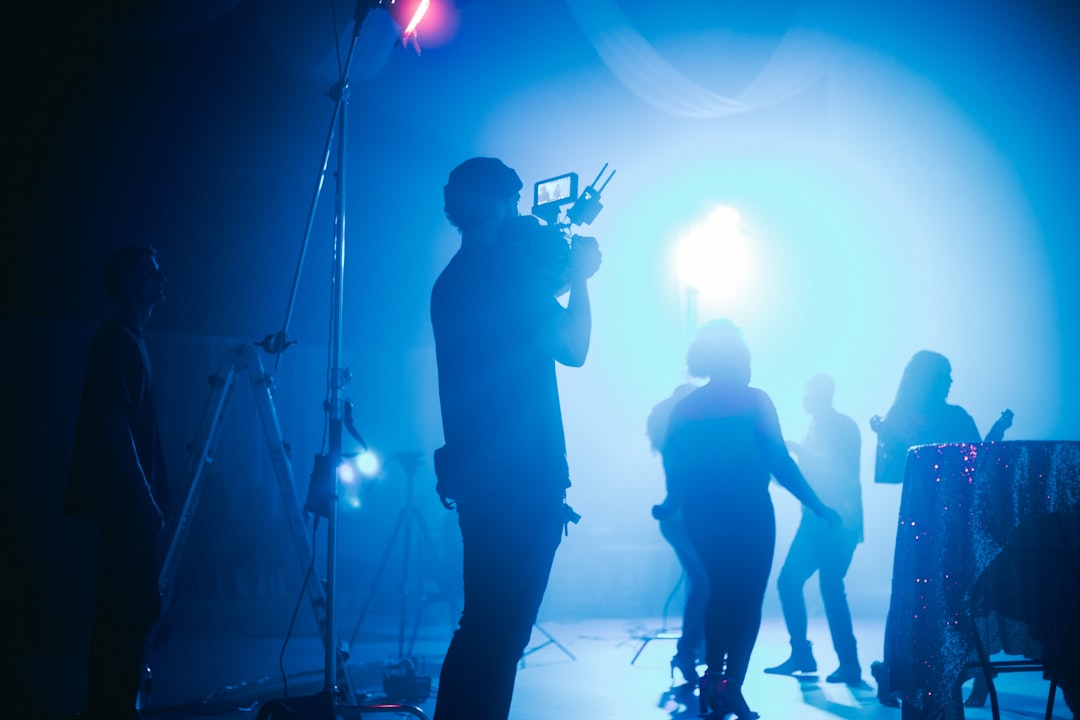
Building a fundamental video metadata structure using Element objects within Python's ElementTree library entails defining the core attributes that enable efficient management and identification of video files. Key elements like creation date, the person who created the video, location of recording, upload date, and camera ID form the foundation of this structure. This ensures that video files are well-organized and readily accessible. ElementTree's capabilities allow you to easily create and interpret XML files, embedding the essential video details directly into your Python code. This approach makes metadata management more manageable, which is extremely important for improving video usability and supporting strong data documentation. The adaptability of XML is definitely a plus, but users should always be aware of the possibility of inconsistent data. It's a good idea to include robust error handling to safeguard data quality.
1. Python's ElementTree library lets you build XML files using objects, treating each piece of your metadata structure as a distinct entity. This object-oriented approach makes handling complex metadata less daunting compared to directly wrestling with raw XML syntax.
2. Storing extra information about an element in XML without adding extra child nodes is done via XML attributes. It's a useful tool for keeping XML organized, especially when dealing with extensive metadata structures.
3. ElementTree's `find()` and `findall()` methods act like XPath queries within your metadata. You can pinpoint and extract specific pieces of information within a complex metadata tree, efficiently targeting specific elements without laborious looping through every single node.
4. When working with larger XML files, the `iter()` function can be used to stream through the data, enhancing performance. Instead of loading the whole file into memory, you can retrieve nodes one by one, thus avoiding the overhead that can sometimes come from handling larger datasets.
5. When you have metadata coming from different sources or standards, namespaces become critical. ElementTree helps manage these by providing ways to define and use namespaces, which is key when building or reading metadata from complex sources.
6. The ability to convert your ElementTree structures back to a string or write to a file offers a smooth path for changes. This is invaluable if your video metadata is dynamic and requires frequent modifications or updates.
7. Nested XML structures can seem intricate, but ElementTree's design makes navigating and changing these hierarchies relatively straightforward. It helps remove some of the initial intimidation that comes with working with complex XML.
8. For projects with metadata that is consistently changing, ElementTree's simple nature lends itself well to fast processing. If you are working with evolving video projects that require frequent metadata adjustments, this kind of efficiency is important.
9. A useful feature in ElementTree is the ability to insert comments directly into your XML. Though it might not seem important, this makes collaboration easier by enabling developers to add notes within the XML file for future maintenance and understanding.
10. ElementTree's ease of use can be a bit deceiving. While it simplifies some tasks, this convenience can potentially lead to overlooking the more intricate aspects of XML handling. Situations where complex error handling or data validation are needed might require more consideration than a novice might assume. It's a reminder that simplicity doesn't automatically equate to robustness in all situations.
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024 - Adding Custom Video Tags and Duration Properties
When managing video files, the ability to add custom tags and define the duration property within the metadata becomes crucial for effective organization and retrieval. Python's ElementTree library, coupled with tools like FFmpeg, provides ways to integrate extra information into the video file itself, which can greatly aid in search and sorting. By adding your own custom tags you can cater the metadata specifically to your needs, while accurately capturing the video's duration in the metadata helps maintain a correct representation of the content. While there are benefits, it's important to be aware of any limits that might be imposed by existing metadata standards. These limitations can sometimes interfere with how users are able to arrange and label their video collection. Understanding and working with these features leads to more accurate metadata and helps stay aligned with advancements in video processing technologies.
1. Adding custom video tags to the XML metadata can be a powerful way to improve how easily a video is found. If you're dealing with a situation where viewers might search for videos using specific terms or categories, having relevant custom tags improves the odds that your video will show up in search results. This can be especially important if you're uploading to platforms where there are many other videos competing for attention.
2. Knowing the duration of a video, encoded within the metadata, can be quite useful for understanding how people interact with it. For example, if you're analyzing viewership data, the duration property can tell you about average watch times. This can be valuable information for figuring out the best length for videos in a particular series or when dealing with different target audiences.
3. When dealing with automated systems like AI-based content recommendation engines or other services that process a large number of videos, custom tags can be a huge help in classification and filtering. By attaching custom tags that are meaningful, these AI systems can be guided to properly categorize content. This can lead to better recommendations for users and a more satisfying experience when navigating a video library.
4. While duration properties seem simple, a mistake in how they're defined or included in the metadata can cause issues. If there's a mismatch between what the XML metadata says about the video's length and the video itself, it can lead to problems like playback stuttering, or the video playing at an unexpected speed. These kinds of issues can significantly degrade the user experience.
5. The way you create the custom tags themselves impacts how readable and maintainable the XML file is. If you develop a systematic approach, you can reduce redundancy and make the metadata structure much clearer. This becomes especially important if several people are working on the metadata or if there's a need to modify the XML over time.
6. It's important to be aware of any constraints or limitations that might be imposed by the video platforms or services you're using. Some systems might have limits on how many characters are allowed in a tag. This can force you to come up with clever ways to encode all the important information you need, without exceeding these limits.
7. A more advanced application of custom tags is the inclusion of temporal markers. These markers can pinpoint specific points within a video. For instance, you could flag scene changes or important dialogues using this technique. This kind of granularity can provide viewers with a richer experience by enabling more refined video navigation or by serving as a guide through particularly complex videos.
8. It's a good idea to utilize schema validation if you're working with XML that's meant to be integrated into other systems. Using a schema helps to ensure that the custom tags you're defining follow a specific format and syntax. This validation process can prevent errors from propagating further down the pipeline.
9. Understanding when viewers choose to stop watching videos can be quite insightful. By segmenting videos based on duration properties, you can conduct analytics to figure out the points where viewers tend to lose interest. This can then be used to identify areas that might need refinement in future videos.
10. When working with a large collection of videos, it's essential to establish consistency in how custom tags are used across all videos. Otherwise, the whole system of metadata becomes a confusing mess. Maintaining uniformity is vital, both for organization and for improving the usability of the metadata when it's searched and utilized.
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024 - Implementing Video Search Indexing Attributes
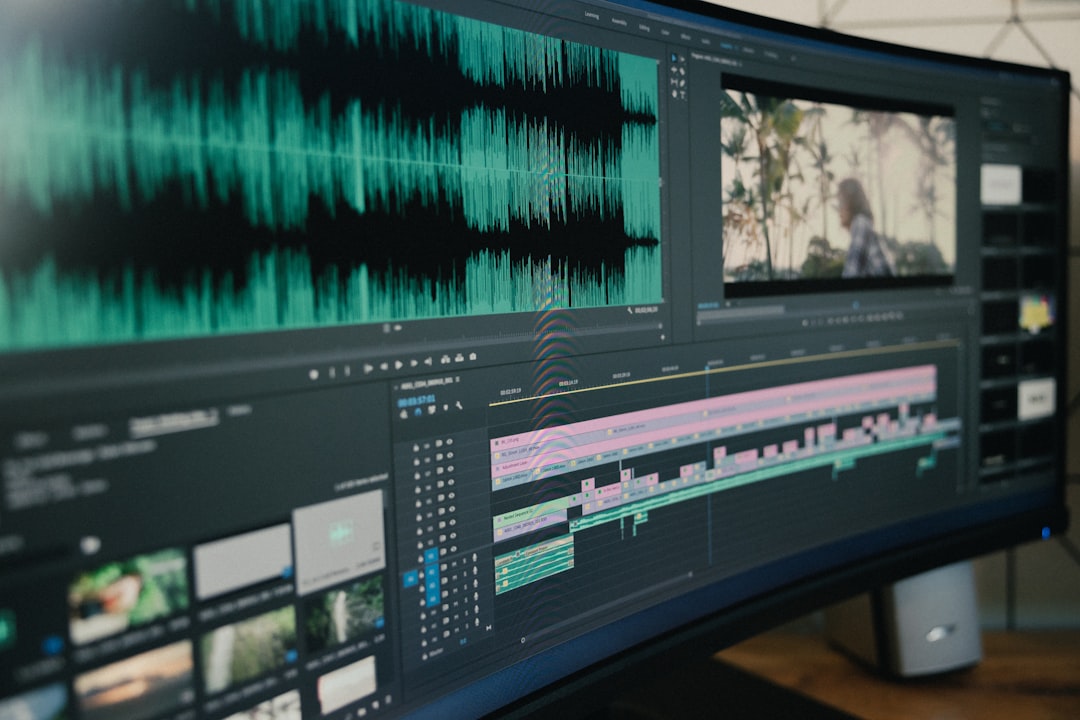
Implementing video search indexing attributes is about making your videos easier to find and use. It's all about helping search engines understand your videos so they can show them to the right people at the right time. This involves using tools like crawlers and sitemaps to help discover and process video content. Then, structuring the video's data (metadata) in a way that search engines easily understand. We can also use advanced techniques like AI-powered similarity search (e.g., using OpenAI's CLIP) to make search results more accurate based on what the user is looking for in the video. Additionally, since videos often feature complex scenes, good indexing relies on thoughtful design to extract meaningful details for better organization and context. Doing all this effectively leads to better search results and improved access to video content.
Implementing video search indexing attributes is a key strategy to boost a video's visibility. By including metadata that reflects common search terms, you can align your video with what people are looking for, potentially driving more views and engagement.
It's interesting to note that research suggests videos with well-structured metadata, including indexing attributes, can see a significant boost in visibility – up to 70% in some cases. This really highlights how crucial it is to optimize video metadata for search engines.
However, getting it right involves understanding the specific attributes that each platform prioritizes. Different platforms (YouTube, Vimeo, etc.) may value categories, tags, and descriptions differently. Ignoring these nuances could mean your video doesn't perform well across the board.
The timing of metadata updates, especially for indexing attributes, is also a factor in a video's online performance. Updating tags or descriptions based on trending topics after a video has been released can lead to increased traffic, but getting the timing wrong might not have the desired impact.
Beyond search, video duration embedded in metadata isn't just a technical detail. Platforms often use duration information to recommend videos based on viewers' habits. Having a duration property that is appropriate and accurate can influence how a video is presented in recommendations.
The whole process of improving indexing with metadata is an ongoing cycle of refinement. You continually monitor how people interact with the videos and adapt the indexing attributes to reflect that engagement. This iterative approach ensures the metadata evolves with changing audience interests.
Custom tagging not only helps viewers find videos, but can also greatly benefit organizations with large video libraries. Having a structured tagging system can make internal searches and automated content management much more effective.
While the benefits of indexing attributes are substantial, there's a risk of miscategorization or tagging errors. If the metadata doesn't accurately reflect the content, search algorithms might get confused, potentially decreasing rather than increasing a video's discoverability.
Further, video metadata needs to be compliant with various digital rights management (DRM) systems. Neglecting these rules can lead to a video being flagged or restricted, emphasizing the need to understand these limitations when defining metadata.
Finally, a standardized set of indexing attributes, established early on, can prevent a chaotic jumble of metadata. Building this framework early on helps maintain clarity and consistency throughout a project. This is really important for preventing a mess that would be challenging to fix later.
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024 - Writing Parseable Video XML Files for whatsinmy.video
Creating usable video metadata for whatsinmy.video requires building well-structured XML files. Python's ElementTree library offers a convenient way to generate these files, allowing users to incorporate crucial details like creation dates, the length of the video, and custom tags relevant to their specific needs. XML's flexibility helps manage complicated metadata, which is important for ensuring accuracy and consistency when using video processing services. However, it's important to be aware of potential inconsistencies or limitations in the way video metadata standards are applied across platforms. This can sometimes affect how videos are categorized and found in large collections. To manage video metadata efficiently, it's vital to include thorough error handling and adhere to good practices for structuring metadata. This combination helps to provide a more reliable way to manage video metadata.
When constructing XML files specifically for whatsinmy.video, a few key aspects warrant attention. Parsing large XML files can be resource-intensive, so employing efficient parsing methods within ElementTree is important for keeping things running smoothly. There are tools that can automatically build XML schemas based on your video data, which can help reduce errors and ensure that your files adhere to standards. It's also worth noting that XML permits you to specify data types for attributes, preventing inconsistencies that can lead to errors.
Furthermore, XML can be used to track the history of modifications made to video files, which is critical in scenarios where maintaining a history is essential, such as in production pipelines or archives. The hierarchical nature of XML lets you layer different categories of metadata in a structured way, allowing for more sophisticated organization schemes. It's worth exploring how XML can connect with Semantic Web technologies like RDF, which opens possibilities for richer data interoperability beyond whatsinmy.video's immediate needs.
You can also use checksums in your XML to safeguard your video files from corruption, especially in environments where files might be moved or stored in multiple locations. It's also a good idea to consider how you will handle different versions of your metadata files, allowing you to go back to earlier versions if necessary. Encoding choices matter too, as an improperly selected encoding can result in display issues on some platforms. Lastly, keeping a flexible XML schema that can evolve with your needs is a good practice, as it allows for future updates and expansions of metadata without needing to completely overhaul your structure.
While the platform whatsinmy.video likely supports common standards for XML parsing, there could be specific optimizations necessary depending on their internal infrastructure. This is where a researcher would explore options such as optimizing the XML structure for faster processing, integrating any specialized components they have, and ensuring the encoding is consistent with their preferences. The more research done on their underlying technology, the better the optimization of the XML files could become.
Quick Guide Building Video Metadata XML Files Using Python ElementTree in 2024 - Validating XML Output Against Video Platform Standards
Ensuring your XML video metadata aligns with the standards of video platforms is essential for smooth integration and data reliability. Python's ElementTree library, alongside packages like xmlschema, empowers you to validate your output against XML Schemas (XSDs). This verification step is crucial for catching errors early in your development process, which can prevent issues with platform compatibility and maintain data integrity. Following established metadata standards, like those proposed by initiatives such as the Video Metadata Hub, is also beneficial because it promotes consistency across different systems. This can improve video discovery and compatibility with a wide range of video processing platforms. However, inconsistencies in how these standards are interpreted across different platforms can exist, and it's wise to remain mindful of these potential variations. Failing to validate can lead to challenges in how your videos are discovered and used within various platforms and can introduce compliance-related problems. It's crucial to pay close attention to the validation process to ensure that your metadata fulfills these requirements and operates as intended.
1. The consistency of XML standards across different video platforms can be a bit of a challenge when trying to ensure that your metadata is presented uniformly. Each platform might have its own set of rules, so you need to be very careful to understand these standards. Otherwise, you run the risk of errors that could actually make it harder for people to find your video content.
2. Because of XML's hierarchical nature, a simple mistake in the structure (like putting an element in the wrong place) can cause errors to ripple through the rest of your metadata. This can result in big problems with video playback or search, making it harder to access your video files.
3. Sophisticated validation techniques include the use of XML Schema Definition (XSD) files, which let you explicitly define the structure and the types of data that each part of your XML can hold. This approach helps ensure that your metadata complies with the rules set by video platforms, reducing the chances of data-related problems before they ever happen.
4. While XML is designed to be quite flexible, some platforms impose limitations on the length of data you can include in specific attributes. If your metadata file exceeds these limitations, the information might be cut off without any warning, resulting in incomplete details that could impact the quality of the user experience.
5. Checking your XML output against platform standards can help you pinpoint performance problems. For example, having too many nested elements can cause the parsing process to slow down. This can affect things like buffering or streaming, which is particularly noticeable during busy times.
6. The use of namespaces in XML can complicate validation. If not carefully defined, this can create confusion and lead to miscommunication between different video services. This can ultimately affect the ability to share your data seamlessly across platforms.
7. Because video platform standards are frequently updated, what might be valid today could change tomorrow. This means it's important to continuously check that your metadata is still valid. This practice is often overlooked, but it's crucial for ensuring that your metadata performs as expected.
8. Automated XML validation tools are really useful for simplifying the process. However, they might not always catch subtle semantic errors. These are mistakes that don't violate the rules of the schema but could still misrepresent the context of your video.
9. The overall quality of the end-user experience relies on properly validated XML files. Errors in the metadata can result in things like incorrect thumbnails or inaccurate video descriptions. This can lead to confusion and potentially decrease user engagement.
10. Identifying and fixing validation errors early in the development process can save a lot of time and frustration. Platforms often provide ways to correct errors in metadata, but understanding the root cause of recurring problems is very helpful for ensuring long-term reliability.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: