Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts
Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts - Using Break Statement Inside Outer Loops in Python 11
Python's `break` statement, when used within outer loops, offers a way to exit a loop prematurely based on conditions encountered during execution. However, its behavior within nested loops can be somewhat limiting. While `break` readily exits the innermost loop where it's encountered, achieving termination of multiple loops simultaneously requires a workaround. This often involves employing a flag variable to signal loop termination from within the inner loop, impacting the outer loop's continuation.
Failing to use `break` within the proper context of a loop leads to predictable syntax errors. This underscores the need for careful consideration of its placement within loop structures. Using `break` effectively can streamline complex scripts by simplifying logical control flow and simultaneously helps steer clear of issues like indefinite loops that can stall programs. Understanding these aspects of `break` contributes to writing robust and efficient Python code.
1. Within nested loops, the `break` statement's behavior becomes more intricate. It typically exits only the innermost loop, but this can be leveraged to influence the outer loops as well, leading to potentially complex control flow. This aspect could be quite valuable when working with scripts involving complex video processing, enabling early termination of inner loops to save processing time.
2. Python's `break` statement is delightfully concise in its syntax. Unlike some languages that demand an accompanying condition, Python's `break` simply exits the loop without any additional keywords or complex logic. This simplicity facilitates quick exits from loops, maintaining clean and straightforward code.
3. It's worth noting that when a `break` statement executes, the program flow immediately jumps to the instruction after the loop. This transition can be a source of potential confusion if one assumes the loop's conditions continue to guide execution. Careful consideration of this behaviour is necessary.
4. When working on video processing, utilizing `break` efficiently can bring considerable performance improvements. If a script can exit the processing loop upon fulfilling specific criteria – perhaps when the needed data has been gathered – it avoids unnecessary computations on subsequent frames. This can be a big deal for processing speed.
5. When you have multiple conditions for exiting a loop, relying solely on `break` can lead to more compact and readable code compared to heavily nested conditional statements. This characteristic can be useful for video processing scripts as it maintains readability and reduces complexity for developers when debugging.
6. The `break` statement's versatility shines through in its compatibility with both `while` and `for` loops. This adaptability is essential for managing a variety of video processing tasks, each potentially requiring unique loop structures for optimal performance.
7. While `break` simplifies control flow, improper or poorly documented usage can lead to unforeseen logical errors. A developer might inadvertently introduce bugs into a video processing script, causing frames to be missed or data handling to be incomplete.
8. Python lacks support for labelled breaks, unlike some other languages. While this feature can be helpful for navigating complex control flow in deeply nested loops, its absence here means developers need to exercise more caution when using `break` to ensure they are targeting the intended loop.
9. The interaction between `break` and exception handling can sometimes be tricky. If an exception occurs after a `break` statement, it might mask the intended effect of the `break`. Therefore, developers need to be aware of this possible interaction to build reliable video processing systems.
10. While `break` is a powerful tool for controlling loop flow, overusing it can lead to code that is difficult to follow. Over-reliance on `break` in video processing scripts can lead to spaghetti code, making maintenance a nightmare. Establishing clear and well-defined exit criteria for your loops can help avoid this issue, fostering more maintainable software.
Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts - Common SyntaxErrors When Break Meets Function Arguments
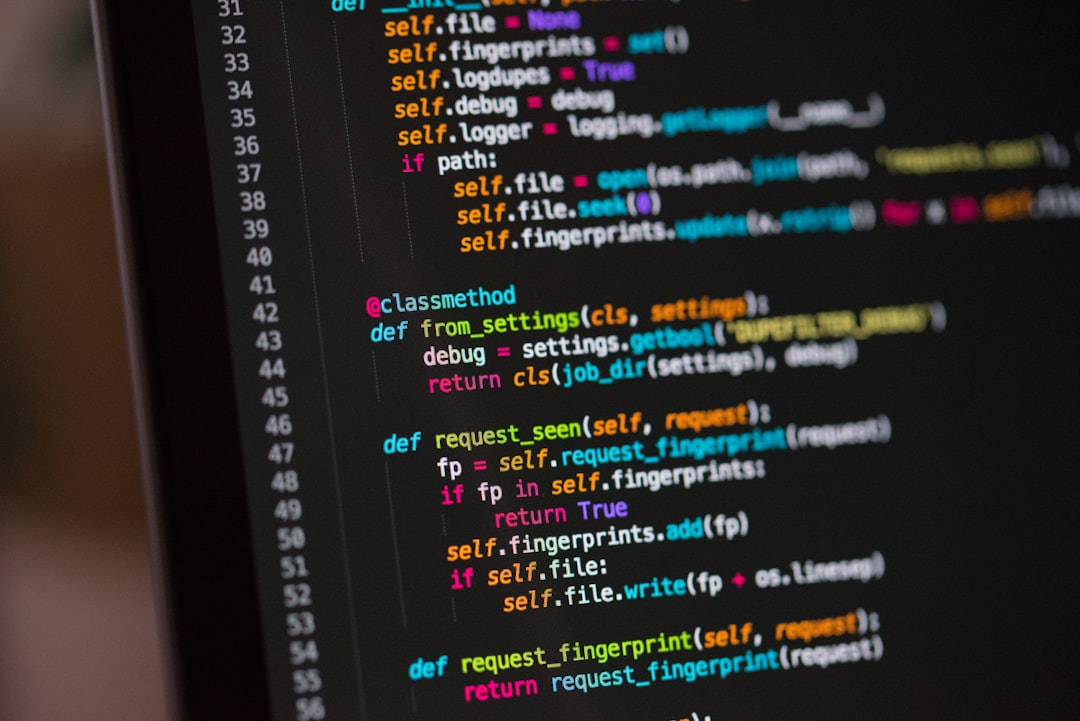
When crafting Python scripts, especially those involved in video processing, understanding how the `break` statement interacts with function arguments is vital for preventing common syntax errors. One frequent issue occurs when developers inadvertently place a `break` outside of a loop, leading to syntax errors that can halt script execution. Furthermore, when functions with loops are used and arguments are passed, improper use or placement of `break` can cause unexpected behavior and disrupt the intended logic flow. Recognizing these pitfalls is key for creating efficient and understandable code, particularly when building complex video processing tools. By being cautious about the use and placement of `break`, developers significantly enhance the robustness and performance of their Python scripts.
1. Python's strict syntax requires `break` to be inside a loop. If you put it elsewhere, you'll get a `SyntaxError`. This rule helps keep code organized and prevents accidental changes to how it runs.
2. When things go wrong with `break`, debugging can get tricky. It can abruptly change how the code flows, making it hard to track what parts of nested loops actually ran. This can add a layer of complexity during troubleshooting.
3. It's surprising how incorrectly using `break` can impact how long your script takes to run. If a loop stops early without careful planning, you might need to re-evaluate the assumed efficiency. Missing steps can lead to recalculations or issues with future frames or data.
4. Poorly documented code using `break` can become a major problem for collaborative projects. If multiple developers work on a video processing script, vague exit points can cause inconsistencies in how they understand the code, leading to less effective teamwork and potential integration difficulties.
5. While `break` simplifies loops, it can sometimes hide the original intention of the loop. This lack of clarity can lead to misunderstandings about the logic after the `break`, introducing potential deeper issues within the video processing code.
6. Think about the long-term maintenance of complex loops using `break`. Every time you come back to a function, figuring out why several `break` statements are there can take time and mental effort. This can impact productivity in large video processing projects.
7. The interplay between `break` and list comprehensions is often confusing. When creating a new list within a `for` loop, `break` abruptly ends the loop without completing the list. This results in incomplete datasets, which could negatively affect video analysis.
8. Many programmers aren't aware that `break` works well with generator functions. Using `break` to exit a generator early can save memory, particularly with large video streams. This can make code run more efficiently without compromising performance.
9. The impact of `break` on code readability is a frequent topic of discussion. It can reduce the length of flow control code, but overusing it in video processing scripts can create dense code sections that are challenging to understand for future developers when they're sifting through complex operations.
10. Python's indentation rules add another layer to `break`'s complexity. Incorrect indentation can cause unexpected program flow, leading `break` to exit the wrong loop. This can be catastrophic in video processing where accuracy is crucial.
Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts - Break Statement Memory Impact on Video Processing Tasks
When it comes to video processing, the `break` statement's influence on memory isn't always obvious but can be significant. While `break` offers a clean way to exit loops early, potentially improving processing speed, it can also affect how your program manages memory.
If a `break` halts a loop before its natural end, it might prevent the script from releasing resources or tidying up properly, a concern when dealing with large video files. This could be especially true in complicated loop structures or if multiple threads are involved, potentially causing memory leaks or overusing system resources.
The key takeaway is that understanding how `break` interacts with memory is crucial for writing efficient video processing code. If not used carefully, `break` can introduce memory-related problems that can negatively affect performance. Paying close attention to these potential impacts ensures your Python video processing tools are robust and perform as intended.
1. The `break` statement's influence on memory usage within video processing loops can be substantial, especially when dealing with large data structures like frames or pixel arrays. Early termination can free up resources immediately, potentially optimizing the overall memory footprint during processing.
2. In Python's video processing realm, mistimed `break` statements can unintentionally cause memory usage to increase. This can happen if data structures aren't designed to handle abrupt exits cleanly, leading to incomplete clean-up operations.
3. Strategically using `break` can help minimize memory fragmentation, especially in demanding video processing scenarios. Premature termination can prevent leftover references from cluttering memory, which would otherwise need to be managed by Python's garbage collector.
4. Employing `break` enables controlled exits from loops that might otherwise run indefinitely during video stream processing. This allows for prompt memory resource reclamation instead of holding onto unnecessary variables that could consume memory unnecessarily.
5. When handling massive datasets, leveraging `break` to exit a loop upon finding the desired outcome can drastically reduce memory overhead. This leads to more efficient memory management, particularly when processing high-definition video.
6. An intriguing aspect of `break` is its subtle influence on how Python manages garbage collection. Exiting loops that handle large objects can trigger their deletion sooner, altering the timing of memory recovery during intensive video tasks.
7. Careful application of `break` in nested loops can improve the clarity of memory management strategies. Developers can define explicit exit conditions that lead to more deliberate memory cleanup procedures.
8. Improper use of `break` might inadvertently cause memory leaks in long-running video processing applications. Specifically, if it interferes with the proper closure of resource-intensive objects that would normally be freed at the end of a loop.
9. The timing of `break` within loops directly affects memory efficiency in Python. When used effectively, it can minimize peak memory usage during video rendering by influencing how and when data is retained or discarded.
10. A less-discussed consequence of using `break` is related to how Python handles memory allocation. Prematurely exiting loops can, in some cases, result in less-than-ideal memory allocation patterns if not carefully managed, potentially slowing down subsequent processing stages.
Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts - Handling Missing Break Keywords in Video Frame Loops
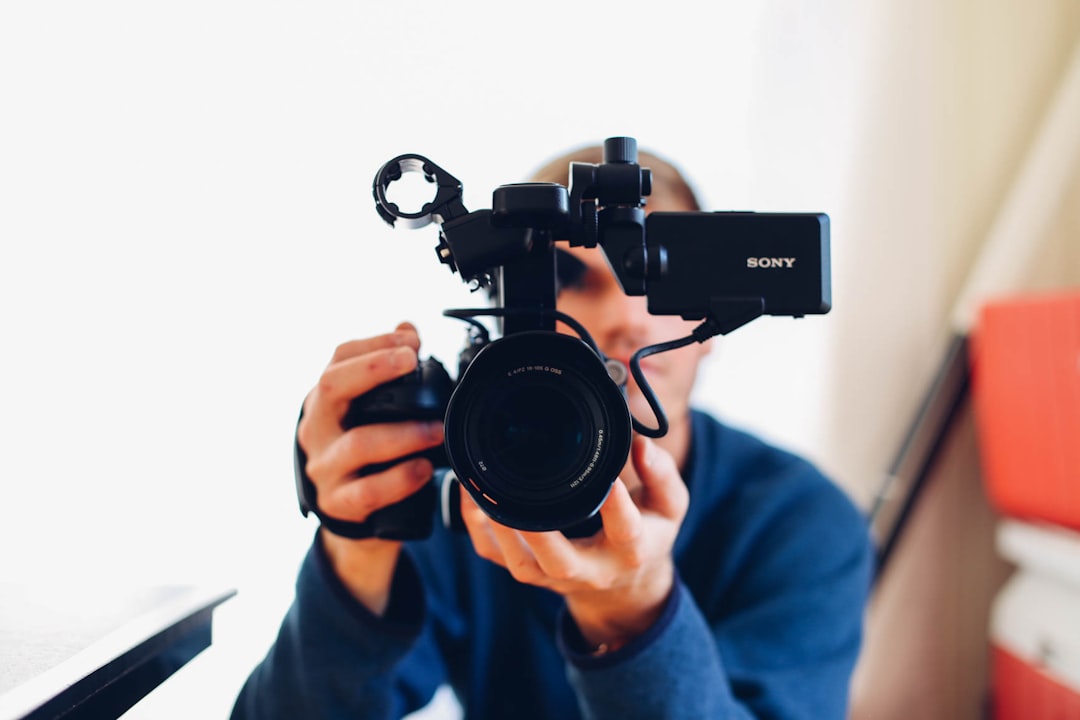
In Python video processing scripts, especially when working with frame loops, overlooking the `break` statement can cause issues. If a frame is missing or a condition is met that requires early loop termination, without a `break`, the loop might continue indefinitely, leading to wasted computing power. This often happens when processing video frames and a missing frame is encountered; standard loop structures might not be designed to handle this gracefully. The result could be an endlessly running script, or worse, errors due to data gaps. This highlights the importance of careful loop control. By including `break` statements at strategic points in the code, developers gain fine-grained control over the loop's execution, ensuring that it terminates as intended when missing frames occur. This leads to smoother, more efficient, and reliable video processing. Understanding how to integrate `break` appropriately is essential for tackling video processing tasks where missing frames or other unpredictable conditions could impact script behaviour.
1. When dealing with video frames, the sequential nature of data can make using `break` within loops a bit tricky. If a loop stops before it processes all the frames it should, it might miss crucial information, which can mess up any analysis you do later on.
2. When you use `break` to get out of a loop early, it could leave some operations unfinished, especially if you're changing pixels in a frame. If a loop modifying pixel values is cut short, it might lead to corrupted frames or incomplete renderings. This means that thorough testing is crucial when writing video processing code.
3. How you organize your data can have a surprising impact on how `break` works. For instance, using generators, which let you get data piece by piece as needed, makes `break` more efficient, because it can stop the processing after getting only the data you need. This can help you use memory more wisely.
4. In video processing where you have multiple threads running, using `break` can get tricky because of the timing issues that can come up. If one thread exits a loop with `break` while another thread is still working with the same data, it can lead to inconsistencies or problems managing resources.
5. The effects of `break` are especially important in real-time video processing, where you need instant feedback. Using `break` can make sure that the processing stops as soon as the needed frame is found, making your scripts more responsive and efficient.
6. Because `break` can cause loops to exit in different ways, it can create situations where errors don't show up until you run into a specific edge case in your video processing. If results from nested loops aren't handled carefully, unexpected outcomes can arise.
7. Debugging video processing scripts that have multiple `break` statements can be challenging. If the exit conditions aren't aligned correctly, it can make the intended flow of control hard to see, making it really important to document the purpose and conditions for each `break`.
8. If you're using external libraries to do video processing, the way `break` interacts with the library's functions can be unpredictable. Some libraries aren't designed to handle abrupt exits gracefully, which can cause problems like resource leaks or corrupted data.
9. Interestingly, resetting the state of your variables after a `break` can make your video processing tasks more stable. If you have some clean-up routines that run after the loop is finished, it ensures that resources are released, reducing memory pressure and making your system run better overall.
10. Using `break` with the `else` clause in loops allows for more precise control over your video processing. The `else` block only runs if the loop finishes naturally, providing a way to handle various states, especially when processing conditions change between frames.
Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts - Break Statement Conflicts With Asynchronous Video Operations
When working with asynchronous video operations in Python, the `break` statement can cause unexpected issues. For instance, in scenarios like real-time video processing, prematurely exiting a loop with `break` can interrupt the flow of frame processing, potentially causing frames to be missed or data inconsistencies. This is especially concerning when dealing with continuous video streams, as improper use of `break` can lead to unnecessary resource consumption. Developers need to be mindful of how `break` affects the asynchronous nature of video processing tasks. They need to consider how and where they use `break` within asynchronous structures to make sure that video data stays intact and performance doesn't suffer. A deep understanding of the interaction between `break` and asynchronous operations is crucial for creating robust and efficient video processing scripts that handle various video processing scenarios smoothly.
1. If you're not careful with how you use `break` in video processing scripts, you might accidentally end up with loops that never stop, especially if the conditions for exiting the loop aren't properly defined. This can cause programs to keep using up computer resources without actually getting anything done.
2. Forgetting to use `break` can cause a build-up of resources. When you're going through video frames in a loop, if you don't stop the loop early when you encounter a problem, you could keep processing bad frames. This would lead to a growing pile of data you can't handle easily.
3. The speed at which you can process video frames can be seriously affected. If `break` isn't used in a strategic way to get out of loops, frames might not be processed, causing delays and making the entire video analysis process slower.
4. When `break` is used improperly, debugging can get very complicated. Using it too much to exit loops can hide how the code actually works, making it tough to figure out where things went wrong. This is particularly true in scripts with multiple threads running at the same time.
5. In situations where video processing happens asynchronously, the timing of `break` becomes very important. If you exit a loop at the wrong moment, you could interrupt the way frames are being processed, possibly leading to video with broken parts or audio that doesn't sync with the visuals.
6. The way `break` works in nested loops can cause problems if it's not managed carefully. Depending on where you put `break`, it could affect the outer loops in a way you didn't intend. You might need those outer loops to keep going to finish processing the remaining data.
7. It's interesting that `break` can also affect how memory is managed by prematurely stopping the release of resources for unprocessed frames. This can cause the garbage collection process to be less efficient, leaving objects in memory for longer than needed because the loop didn't end properly.
8. Having clear `break` conditions not only lets you have more control over what's happening, it can also make your code easier to understand. This leads to code that's easier to maintain and allows other developers to quickly grasp what's going on. This is especially helpful when working with others on the same project.
9. The way `break` interacts with the libraries used for video codecs can be tricky if the libraries aren't set up to handle early exits properly. If this isn't handled correctly, it could cause video files to be corrupted or leave you with incomplete data after processing.
10. Understanding how `break` works with other control flow statements, like `continue`, allows you to have more precise control over loops. By using these statements together in the right way, you can create video processing systems that can react quickly to changes during the process, greatly improving how frames are handled.
Understanding Python's 'break' Statement Common SyntaxError Pitfalls in Video Processing Scripts - Side Effects of Break Statements on Video Frame Buffer Management
When managing video frame buffers using Python, the `break` statement can have unforeseen consequences. Its main purpose is to stop a loop prematurely based on specific conditions. However, misusing `break`, especially within complex loop structures, can cause problems like frame loss, incomplete data processing, and even memory-related issues. This becomes more complicated in nested loops where `break` only stops the innermost loop, possibly resulting in unintended behavior in the outer loops. For instance, outer loops might run longer than expected, using up more resources than intended. Developers must take care in how they use `break` to create clear exit points and ensure their video processing scripts are efficient and reliable. Ultimately, understanding `break`'s behavior in complex video processing scenarios is crucial for writing code that runs well and produces accurate results.
1. When working with multiple threads in video processing, `break` can introduce synchronization problems. If one thread exits a loop early using `break` while another thread is still using the same data, it can cause inconsistencies and potentially disrupt the entire processing process.
2. In complex video processing tasks involving large datasets, the way `break` is used can directly impact buffer management. If a buffer loop isn't terminated promptly with `break` when it should be, the buffer could overflow, potentially leading to delays, crashes, or errors.
3. The timing of `break` within video filtering routines significantly influences the overall performance. If `break` is used poorly, it could lead to incomplete analysis or inaccurate results, which is a particular concern in situations where subtle details are critical for the quality of the output video.
4. `break` can be especially helpful when used with Python's generator functions for video processing. By allowing early termination in the generation of frames, it helps keep memory usage low, which is particularly beneficial for video analysis processes that might run for long periods.
5. When `break` isn't used correctly, it can create "zombie" frames, where parts of a frame or data associated with that frame remain in memory even after the loop is exited. This can lead to issues with subsequent processing steps or unexpected behavior.
6. A less obvious pitfall of using `break` improperly is that it can potentially mask race conditions in asynchronous operations. If a loop exits unexpectedly using `break`, important synchronization steps within the asynchronous operations might be missed, which could lead to unreliable and unpredictable results in the final video.
7. `break` can significantly impact memory usage in your video processing scripts. Used well, it can free up resources quickly, but when mismanaged, it might prevent the system from releasing data that it no longer needs, making it more complex to manage memory overall.
8. Strategically embedding `break` within tightly controlled loops can help optimize CPU utilization when processing video. Exiting loops early when a desired resolution threshold is reached can conserve processing power, especially when working with high-resolution content.
9. When working with external video APIs, using `break` carelessly can disrupt the interaction with the API. An abrupt exit from a loop could cause errors in the data flow, potentially resulting in leaks of resources or inconsistent video output.
10. When processing video streams with variable frame rates, understanding how to utilize `break` effectively is crucial to maintaining frame synchronization. Proper implementation of `break` can ensure that the processing of video frames stays consistent with the actual video data, especially when dealing with live video applications.
More Posts from whatsinmy.video: