Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures
Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures - Understanding NoneType Objects in Python Video Processing
When processing videos with Python, understanding the nature of `NoneType` objects is essential. `None` essentially signifies the absence of a value, which can trip up your code if not handled properly. Frequently, you'll see errors like "NoneType object is not subscriptable" or "NoneType object is not iterable." These crop up when functions, particularly those designed to handle video data, inadvertently return `None` instead of the expected iterable or indexed structure.
This is particularly problematic in video processing as functions may return `None` if no data or results are found. It's easy for such situations to cause a crash in your code. The core solution is diligent error handling. You need to validate function outputs to make sure they're not `None` before you try any operations that assume a valid object. This means adding checks to your code to ensure that you don't try to use `None` as if it were something else. By proactively addressing these potential error scenarios, you'll significantly enhance the stability and reliability of your video processing applications. It's about preventing your code from unexpectedly stumbling when it encounters the absence of data.
1. In the realm of Python video processing, NoneType objects act as placeholders for the absence of a value, often appearing unexpectedly when things like frame loading or data streams encounter hiccups. These 'empty' values can subtly impact the processing flow.
2. Video processing libraries frequently utilize None as a signal for various failures, such as invalid file paths or codec mismatches. This reliance on None emphasizes the importance of meticulous error handling, as ignoring these signals could lead to unexpected program crashes.
3. NoneType objects can stealthily infiltrate data structures. For instance, if a tuple includes a None value and you attempt to unpack it, a TypeError will abruptly halt your video processing pipeline. It's essential to be prepared for these unforeseen disruptions.
4. Pinpointing the source of a NoneType error can be a detective's game. Tracing the function call that initially returns None often involves painstaking debugging, especially in complex architectures with multiple layers of abstraction. This process can be more time consuming than one would hope.
5. During the batch processing of videos, NoneType errors have a nasty habit of snowballing. A single corrupted file can cascade errors through the different stages of your processing, negatively impacting the overall quality and output of your project.
6. Python's dynamic typing can be both a blessing and a curse in this context. Functions can accidentally return None instead of the anticipated data type, necessitating diligent type checking and robust logging to avoid cryptic program failures.
7. While potentially inconvenient, NoneType can provide valuable clues for developers. When encountered during video frame analysis, it can point to missing data or a condition that requires further attention and code adjustment.
8. Chaining method calls should always be undertaken with a consideration of potential NoneTypes. If a method in the chain returns None, the subsequent actions will be thwarted, particularly problematic when processing sequential video frames.
9. When working with video data, it's good practice to explicitly check for the possibility of NoneType values before proceeding. This approach, which leads to more robust and bug-resistant code, is especially crucial in environments where unexpected edge cases are more common.
10. Employing NoneType as default values in function parameters is a common practice that can optimize certain video processing operations. However, this convenience comes with a caveat—if you don't carefully consider how to handle a None value, it can create subtle, difficult-to-diagnose errors that undermine the processing outcome.
Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures - How Failed Video Processing Returns None Values for Stream Data
When processing videos using OpenCV, a frequent source of trouble stems from the `cv2.VideoCapture` function failing to deliver frame data. Instead of the expected video frames, it can return `None`, which, if not anticipated, causes `NoneType` errors. These errors usually happen when you try to access data from a `None` return value as if it held a valid object or sequence of objects. Often, these issues arise from problems accessing the input source like when a file isn't found or camera settings are incorrect. There are also potential issues within the complexities of streaming protocols, particularly with RTSP streams. Developers need to incorporate strong error handling measures to address these situations. This includes explicitly checking that the video capture process has indeed successfully acquired data and confirming the validity of all input source configurations before attempting any further processing. This careful approach helps to minimize disruptions and program crashes due to unexpected failures in the video pipeline, thus making the processing more dependable. It's about ensuring your application can gracefully handle the absence of expected video data without completely failing.
1. When working with video processing, a `None` value can pop up due to a variety of reasons, such as hardware limitations or incompatibility between codecs used by the video and your processing system. Figuring out the exact cause often requires some digging into system settings and the resources your program uses.
2. It's interesting that many common libraries for video processing, like OpenCV and moviepy, will readily return `None` when things go wrong. This design choice emphasizes the need to have robust error-checking and handling procedures within your code to keep everything running smoothly.
3. Somewhat counterintuitively, a `NoneType` error can actually occur even when the input data is fine. For example, if a video feed gets briefly interrupted, but the function expects a constant stream, it might return `None` instead of signaling a clear error.
4. Things get a bit more complicated when you start using asynchronous video processing, because `None` return values can cause problems in callback functions. If not managed carefully, this can cause delays and race conditions which can harm your application's performance.
5. Debugging a `NoneType` error within a multi-threaded system can feel like trying to untangle a complex knot. Finding exactly when a `None` value gets introduced can involve poring over shared resources and synchronization points in the code.
6. Even things like frame rates and resolutions can be affected by `None` values being returned, leading to potentially misleading results when trying to track how well a system is performing. This can lead to wrong conclusions about the system's efficiency.
7. When dealing with real-time video streams, `None` values can cause temporary slowdowns or dropped frames, creating a choppy or inconsistent viewing experience if not properly handled. Poorly written code in this area can lead to a frustrating user experience.
8. `NoneType` isn't only tied to the output of functions. Functions may sometimes use `None` as default values for their inputs. If these defaults aren't carefully managed, they can create faulty assumptions about the validity of your input data, causing problems down the road.
9. In fast-paced development environments, teams can often overlook the importance of `NoneType` errors, seeing them as minor issues. However, a single ignored `None` can lead to overall system reliability issues and result in larger, unforeseen failures if not addressed.
10. If you look into how `None` interacts with Python's exception handling, you can uncover even deeper insights. By carefully handling `None` returns, you can avoid a lot of unnecessary log messages and create cleaner, more maintainable code.
Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures - Debugging Missing Return Values in Video Frame Extraction
When extracting frames from a video, a common challenge is encountering missing return values, resulting in pesky `NoneType` errors. These usually stem from issues within the `cv2.VideoCapture` function or similar methods, where the expected frame data isn't delivered. This can occur due to problems with the video source (like a file not being found), incorrect configurations, or even complications with streaming. A key strategy for handling these situations is to build in strong error checking. Developers should always verify the function outputs before moving on to other processing steps to avoid problems caused by encountering a `None` value. Taking this proactive approach not only boosts the dependability of your video processing but also leads to a more polished, user-friendly experience. It's essentially about creating applications that can handle situations where video data isn't available without breaking completely.
1. Extracting frames from videos is a delicate process, where even small issues like mismatched codecs or frame rates can result in functions returning `None`. This can lead to large chunks of video being unretrievable, highlighting the sensitivity of frame extraction.
2. It's intriguing that using a `try-except` block might not always catch `NoneType` errors, particularly if it's not properly designed. A function that silently fails and returns `None` can go unnoticed until later, during the unpacking stage, making debugging considerably more challenging.
3. Video processing libraries frequently utilize `None` as an indicator that the end of a file has been reached. If checks aren't in place for this scenario, processing can halt prematurely, and developers may be misled into searching for issues elsewhere in their code.
4. Some video processing workflows may implicitly ignore `None` returns when stringing together methods. This oversight can propagate errors through the whole processing pipeline, potentially leading to silent data corruption without any immediate warnings.
5. The nature of the video source—local files or streaming data—plays a role in how often `None` values appear. Streams, especially those from unreliable networks, are more prone to random `None` returns compared to the more predictable local file processing.
6. In high-performance video applications, `None` can be a hidden performance bottleneck. If not diligently checked and managed, it can slow down frame processing and affect overall responsiveness, creating lag in real-time scenarios.
7. Developers often make the assumption that video files are error-free, but corruption can happen at any point in their lifecycle. This unforeseen situation can lead to functions quietly returning `None` without any explicit warning, making it harder to troubleshoot.
8. One of the trickiest aspects of `None` returns lies in multi-threaded video applications. Race conditions triggered by `None` values can lead to inconsistent application states, emphasizing the importance of robust synchronization methods.
9. The underlying operating system and platform can also influence the frequency of `None` returns during video frame extraction. For instance, differences in how hardware acceleration is implemented across various operating systems can affect frame retrieval success rates.
10. When employing libraries like OpenCV, carefully reading the function documentation is crucial. Overlooking how a function handles errors can create unrealistic expectations about its stability and complexity, hindering the debugging process when things go wrong.
Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures - Memory Allocation Issues Leading to NoneType Errors
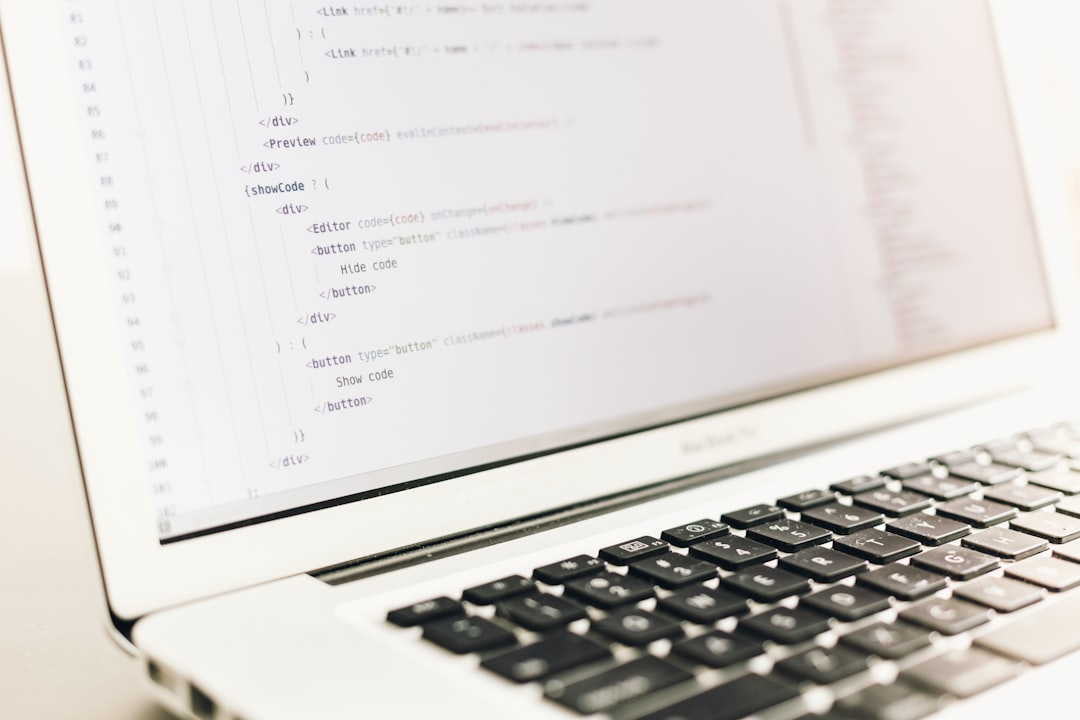
Memory allocation problems in Python can trigger `NoneType` errors, especially when a program runs out of the memory it's been assigned. If the available memory is tight, operations might accidentally return `None` when trying to create objects or access resources that can't be properly formed due to the memory limitations. This situation frequently arises in video processing, where the sheer size of data and numerous actions running simultaneously can increase memory use considerably. As a result, functions might return `None` instead of the intended objects. It's vital for programmers to be cautious about initializing variables and thoroughly checking function results, ensuring they can deal with potential `None` outputs smoothly to stop errors from spreading throughout the processing flow. Managing memory effectively and anticipating `NoneType` situations can make video processing applications significantly more resilient.
1. When dealing with video data, functions can unexpectedly return `None` for seemingly innocuous reasons like a momentary network hiccup. This highlights the sensitivity of video processing to subtle shifts in data availability.
2. The specific video codec employed can play a significant role in `None` values popping up. If there's a mismatch between the codec used in the video and the system's capabilities, frame extraction might fail, leading to unexpected halts in the processing sequence. Properly managing the choice of codecs is important.
3. It's not unusual for libraries to leverage `None` as a default return when a function doesn't yield valid data. However, this double duty of `None` can confuse things if not checked explicitly, potentially creating subtle errors within your logic.
4. Asynchronous programming styles bring with them a whole new set of challenges related to `None` returns. Timing-related issues can quickly lead to race conditions, situations where the order of execution causes unpredictable outcomes that disrupt the intended flow of your video processing tasks.
5. The possibility of video file corruption is always lurking, whether during downloads, storage, or processing. Corrupted files can often manifest as a `None` value, highlighting the importance of checking file integrity before launching any operations.
6. Instead of viewing them as purely negative, we can treat `None` values as signals that something isn't quite right. They could be flags for hardware problems, faulty configurations, or various other issues, helping guide developers in their troubleshooting.
7. Multi-threaded video processing brings the added complexity of potential inconsistencies between threads due to `None` values. Without adequate synchronization and state management, a `None` in one thread can lead to unpredictable side effects elsewhere.
8. In performance-critical applications, carelessly handling `None` can introduce hidden bottlenecks that negatively impact frame processing speed, especially when rapid frame rates are essential. Carefully implemented checks before operations can mitigate such delays and ensure smooth real-time processing.
9. Like a domino effect, a single `None` can trigger a chain reaction of failures throughout your video processing pipeline. The origin of the problem can be difficult to identify because it's not always obvious how this initial `None` impacted the downstream processes, leading to a need for intricate debugging.
10. The specific environment the code is running on can alter the probability and nature of `None` returns. Different operating systems and hardware can handle video processing with varying degrees of reliability, leading to platform-specific challenges. This reinforces the need for flexibility and adaptability in our code design.
Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures - Common API Response Failures in Video Processing Libraries
Video processing libraries often rely on APIs to fetch and manipulate video data. Unfortunately, these APIs are prone to various failures that can significantly disrupt your workflow. Issues such as incorrect configurations, network slowdowns, and unexpected responses from external services can lead to API calls returning invalid or even `None` values, causing headaches for developers. This can happen even with robust libraries like OpenCV or specialized tools such as VidGear.
Without proper error handling in place, a function like `getFrame` might return `None` unexpectedly, and this can cascade through your code, creating a chain of unintended consequences. Developers need to be mindful of potential API response failures and incorporate strong error checking into their code. Moreover, clear API documentation and ongoing monitoring are essential to minimize disruptions caused by these failures. A good understanding of how to anticipate and manage API-related issues is crucial for building reliable and robust video processing applications. Otherwise, subtle errors can have an outsized negative effect on the end result of your projects.
1. A significant portion of `NoneType` errors in video processing seem to stem from improperly configured environment variables, which can subtly impact how libraries like OpenCV interact with system resources. This hidden factor can make debugging more complex.
2. It's intriguing that even inconsistencies in frame rates can unexpectedly lead to `None` values during video processing. If the anticipated frame rate is exceeded, the library might drop frames and return `None`, interfering with the flow of data.
3. Video processing libraries often work with asynchronous operations, making the management of `None` even more critical. A thread attempting to retrieve a frame might be interrupted, causing it to return `None` and leading to intricate timing issues within the application.
4. The relationship between hardware capabilities and video codecs can reveal weaknesses in performance. Specific codecs require particular hardware resources, and a mismatch can result in widespread `NoneType` errors during processing.
5. Intricate data processing workflows can inadvertently propagate `None` values. A function early in the process returning `None` might cascade through later stages, affecting the final output without clearly indicating the source of the error.
6. Surprisingly, not all `None` returns represent major errors. A library might return `None` to signal the end of a data stream, highlighting the importance of having thorough checks to differentiate between various states.
7. The use of `None` as an error signal can lead to ambiguous bugs. Functions may return `None` for perfectly legitimate reasons, such as successfully completing execution without generating data. This makes it crucial to clearly define what return types to expect.
8. Advanced video editing and processing workflows often accumulate `NoneType` errors, as they involve interactions between multiple libraries. Each library has its own failure handling approach, so integrating APIs can exacerbate debugging challenges.
9. In environments with high processing loads, the memory demands of video processing can strain system resources. Under these conditions, `None` returns can rise considerably if memory management isn't rigorously controlled, underlining the need for effective resource allocation strategies.
10. Finally, the differences in programming styles across libraries (object-oriented versus procedural, for example) can influence how `None` errors are handled. This variability can lead to unpredictable behavior when integrating various libraries, making it challenging to maintain consistent error handling across the codebase.
Understanding Python's NoneType Unpacking Error A Developer's Guide to Fixing Common Video Processing Failures - Testing Strategies to Prevent NoneType Unpacking Errors
When working with Python, especially in the realm of video processing, it's crucial to prevent the dreaded `NoneType` unpacking errors that can bring your code to a screeching halt. A key aspect of preventing these errors is to thoroughly test your code. This involves proactively checking for `None` values before attempting to unpack any data structures. By doing this, you're essentially safeguarding your code against unexpected situations where a function returns `None` instead of a proper value.
It's important to develop a habit of verifying the outputs of functions. Make sure the data returned is indeed the data type you expect and not `None`, which can act as a signal of various issues. You can design checks to see if the return value is valid before passing it on to further processing. This can be achieved through various techniques, including conditional statements or validation checks. Additionally, you need to have a good grasp of how the specific functions you're using can lead to `None` values being returned. The more you understand how your functions behave in different situations, the better prepared you are to design robust code that effectively avoids these unpacking errors.
These proactive approaches not only help prevent bugs but also enhance the overall user experience, leading to more efficient and reliable video processing applications. A little effort during the development phase can lead to significant improvements in the overall quality of your video processing workflows.
1. **Conditional checks** are your first line of defense. Before you unpack any value, it's wise to confirm it isn't `None`. Using `if variable is not None:` not only prevents crashes but also makes your code easier to understand.
2. **Assertions** can act as early warning systems. If you suspect a variable might be `None` at a certain point, insert an assertion like `assert variable is not None`. This catches potential issues during testing instead of runtime, making debugging smoother.
3. **Context managers** prove helpful when dealing with resources that could return `None`. They ensure that resources are properly managed, stopping `None` from trickling into other parts of the code. This is especially important for dealing with things like file handles or network connections that might fail.
4. **Thorough logging** is a critical tool. Whenever there's a chance a function might give back `None`, add logging messages around that operation. If errors do pop up, this creates a trail of breadcrumbs that can quickly guide you to the origin of the problem, significantly simplifying debugging efforts.
5. **Default parameters** can be a good way to minimize the effect of `None`. By providing sensible defaults within function arguments, you can guarantee that even if a function receives unexpected inputs, it will return valid values.
6. **Functional programming paradigms**, like `map` and `filter`, can help proactively weed out `None` values from your data structures before unpacking. This approach streamlines the data processing step by reducing the chance of `NoneType` problems.
7. **Standardized return value patterns** can make your life easier. Instead of relying on `None` to represent both successful and unsuccessful operations, think about creating more structured returns, like using tuples with flags. This creates explicit signals for success or failure, improving error management.
8. **Edge case testing** is vital. During development, it's crucial to deliberately test the limits of your code. Include specific unit tests focused on scenarios where `None` might be generated to ensure that the code can manage unexpected situations.
9. **Graceful degradation** can save the user from a frustrating experience. Design your application to degrade gracefully when `None` appears. For instance, you might provide a fallback mechanism so that the application doesn't crash or freeze if it encounters a `None` result.
10. **Iterative development** is key. As you refine your code, it's helpful to continually assess how `None` is handled. Regularly refactor code that might potentially encounter `NoneType` errors. This promotes code quality and stability, especially for complex and long-running projects.
More Posts from whatsinmy.video: