Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis - Understanding LSTM Networks for Video Analysis
LSTM networks are particularly well-suited for understanding video data because of their specialized design for handling sequences. A key feature of LSTMs is the cell state, essentially a mechanism that acts as a memory, allowing information to persist across time steps. This unique capability enables them to capture both the spatial details within each frame (which can be extracted using CNNs) and the temporal dependencies across the sequence of frames, making them powerful for understanding the evolution of events in a video.
The integration of CNNs and LSTMs has led to significant advancements in video classification, with the CNNs providing a powerful way to extract the spatial features from video frames and the LSTM processing those features across time. The practical application of these networks is made more accessible through libraries like TensorFlow and Keras, which streamline the model building process.
Fundamentally, LSTMs have become a core element in deep learning approaches for video analysis due to their ability to deal with the dynamic, sequential nature of video content. Their potential to learn intricate patterns from video sequences makes them an important tool for applications in video understanding and classification. While some may see them as a relatively complex type of recurrent neural network, they are currently considered to be one of the best options for analyzing and classifying dynamic visual information.
LSTM networks, designed to tackle the vanishing gradient issue plaguing standard RNNs, excel at capturing long-term dependencies within sequences like video frames. This ability is crucial for understanding the flow of events in videos.
In video analysis, LSTMs can handle both spatial and temporal data. While 3D convolutional layers can extract spatial features, the LSTM layers are then responsible for the sequential prediction or classification tasks that rely on the temporal dynamics of the video.
LSTM's gating mechanisms, composed of input, forget, and output gates, give the network the capability to selectively remember important information while discarding the rest over time. In the context of video analysis, this is useful for distinguishing relevant video segments from less significant ones.
Studies suggest that, in many tasks like action recognition or generating video descriptions, LSTMs can surpass other architectures, including plain CNNs. This advantage stems from their ability to discern the temporal relationships among video frames.
The length of input sequences has a big impact on LSTM performance. Excessively long sequences can impose huge computational burdens and require a lot of memory. It's a resource balancing act that can impact the feasibility of an application.
When preparing videos for use with LSTMs, it's standard practice to process the input frames. Things like resizing or normalizing the input help ensure that the network learns effectively across different video qualities.
Despite their strengths, LSTMs may not be the best choice for every video analysis job. In real-time applications, lighter-weight architectures such as GRUs, which have fewer parameters, might be a better approach.
Occasionally, discarding some frames during preprocessing can improve LSTM performance. This can reduce noise and processing times, enabling the network to focus on significant transitions or movements within the video.
The combination of LSTMs with attention mechanisms, allowing the network to focus on key segments of the video, can increase the efficiency and accuracy of analysis by selectively weighting features.
The effectiveness of an LSTM model for video analysis heavily relies on careful hyperparameter tuning. Choosing the right number of LSTM layers, the quantity of units per layer, and the learning rate all require extensive experimentation to find the optimal settings for a specific task.
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis - Setting Up the Python Environment for LSTM Implementation
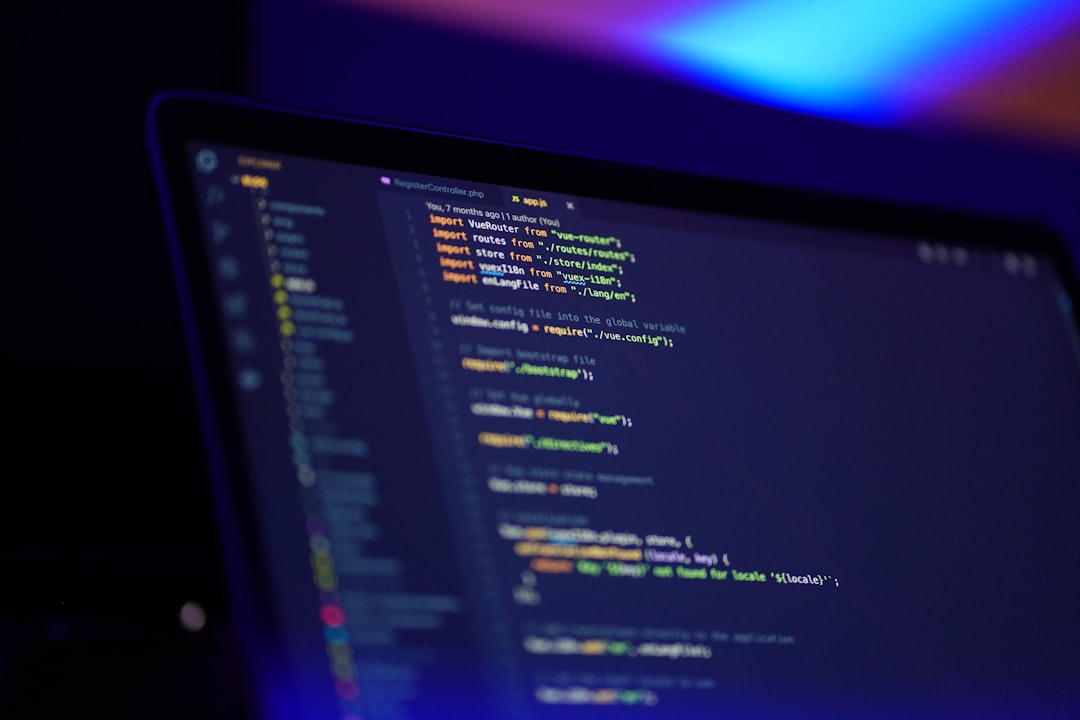
Setting up the Python environment to work with LSTMs is a foundational step in applying these powerful neural networks to video analysis. You'll need to install a few key libraries. TensorFlow and Keras are often used because they offer convenient tools to build and train LSTM models. In addition, you'll likely want Pandas for data manipulation and NumPy for efficient mathematical operations. A well-organized environment makes it easier to experiment with different LSTM configurations. This includes adjusting the model's structure, fine-tuning various hyperparameters, and even integrating more advanced features like attention mechanisms.
While the standard frameworks offer a lot of helpful functionality, it's important to be aware that they might not always be the ideal solution for every project. Depending on the specific task or research question, you may need to implement custom elements. Ultimately, a thoughtfully configured Python environment provides a solid foundation for deeper analysis of sequential video data and enhances the ability to unlock valuable insights for video understanding and classification.
1. **Navigating the Python Ecosystem**: Setting up a Python environment for LSTM implementations can be a bit intricate, as it involves managing the interplay of several libraries like Pandas for data wrangling, NumPy for numerical operations, and potentially TensorFlow or Keras for building and training the network itself. Ensuring compatible versions of these libraries can be a bit of a puzzle.
2. **The Power of GPUs**: LSTMs, with their intense computational demands, really benefit from the speed of GPUs. Getting CUDA and cuDNN properly set up is key to faster training times and makes a big difference in the overall feasibility of experiments. The choice of hardware (and its cost) certainly becomes a key factor in the process.
3. **Containerization for Reproducibility**: Containerization, often through Docker, can be quite useful for streamlining the environment setup process. The idea is to isolate all dependencies within a container, which helps ensure consistency across various environments—especially helpful in a collaborative setting where multiple people are involved in the project.
4. **Virtual Environments: A Clean Slate**: Using `virtualenv` or `conda` is a good practice to isolate project dependencies, which is critical for preventing version conflicts. It's a way to create a clean environment for each LSTM architecture you want to experiment with, avoiding a tangled mess of dependencies.
5. **The Data Pipeline: A Bottleneck to Watch**: The speed of LSTM training is significantly affected by how video data is fed into the model. A robust data pipeline designed to load, preprocess, and batch video frames in an efficient way is important. Issues in this part of the system can easily become the limiting factor in the whole process.
6. **Monitoring the Learning Process**: Tools like TensorBoard allow you to monitor training performance in real-time during training. This is incredibly helpful for identifying issues early on and for making adjustments on the fly. It can really speed up the optimization of model performance.
7. **Leveraging Asynchronous Loading**: Python offers ways to load and preprocess video data asynchronously (using libraries like `multiprocessing` or `threading`). The idea is to utilize the CPU for processing while the GPU is kept busy with training, enhancing overall efficiency.
8. **Feature Enrichment with Preprocessing**: Techniques such as Optical Flow can provide valuable information about motion patterns before they're fed into an LSTM network. This preprocessing step enriches the training data and makes temporal dynamics more explicit for the network to learn from.
9. **Tackling Overfitting**: LSTMs, given their complexity and the fact that video datasets aren't always gigantic, are prone to overfitting (i.e., performing well on training data but not generalizing to unseen data). Techniques like dropout or L2 regularization are valuable to add to the model and help to combat this issue during the initial setup phase.
10. **The Balancing Act of Batch Size**: The size of the batches used during training can have a significant effect on the training process. Smaller batches may lead to more stable gradients but can slow down the training, while larger batches may lead to less stable gradients but faster training. Finding a good balance based on available computing resources and model specifics is crucial.
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis - Data Preparation and Preprocessing Techniques
Within the framework of implementing LSTM networks for video analysis, the preparatory steps of data handling and preprocessing are undeniably critical for achieving optimal model performance. These steps involve the essential task of converting the raw video data into the three-dimensional array format that LSTMs require, which often includes handling any missing data points within the dataset. Moreover, it's important to normalize the input data to maintain consistency, promoting the network's ability to learn effectively. This phase might necessitate transformations to ensure data stationarity, which can improve the model's ability to learn patterns. Additionally, visualizing the time series nature of the video data can be insightful, helping to identify recurring patterns, trends, and any seasonal aspects that may influence the analysis. These preprocessing techniques are not just about cleaning up the data but also lay the groundwork for LSTMs to efficiently capture the long-range dependencies within the video sequences, a crucial factor in improving the accuracy of the network's predictions within video analysis applications. However, it's important to consider the particular characteristics of each dataset. Factors like the computational burden of processing the video frames and potentially needing to discard frames to mitigate noise or improve performance need careful evaluation. These aspects, along with the more typical preprocessing tasks, work together to fine-tune the data and prepare it for optimal LSTM performance in video analysis.
LSTM networks, as we've discussed, are adept at handling the sequential nature of video data. However, to truly unlock their potential, preparing the data appropriately is crucial. This involves more than just chopping up the video into frames. The effectiveness of an LSTM model is heavily tied to the quality and structure of the input data.
First, the sheer quality of the input data plays a major role. If the data is riddled with noise or errors, it can throw off the training process and lead to inaccurate results. It's like trying to build a house on a shaky foundation; the structure will likely crumble. Therefore, data cleaning and preprocessing become very important steps in the pipeline. This often involves identifying and removing outliers or filling in missing values, maintaining only the essential features.
Speaking of features, how those features are scaled can also affect the LSTM's performance. Whether we're using Min-Max normalization or Z-score standardization, the chosen scaling method can influence convergence and the overall accuracy of the model. It's a matter of finding the method that best suits the specific dataset and the learning process.
Beyond just features and scaling, we often overlook a key aspect: the temporal context of the data. For example, the duration of a video clip or the frame rate can significantly impact how an LSTM interprets the events within it. This information can provide the model with a stronger sense of context, allowing it to make more informed predictions based on the flow of frames. Without such context, we're missing key pieces of the puzzle.
To make the model more robust, techniques like data augmentation can be beneficial. Randomly cropping or flipping frames can increase the diversity of training data without the need for collecting additional video material. This can make the LSTM less prone to overfitting to specific instances in the training data.
Dealing with large datasets requires a more efficient approach. Video data is a prime candidate for parallelization. Employing techniques like those within Apache Spark can speed up the preprocessing considerably. This becomes particularly important as we tackle increasingly complex datasets and aim for scalability.
There is a key point here that often gets ignored: videos are fundamentally time-series data. Although they might appear to be just collections of images, treating them as a time series is essential. Some conventional image processing methods might fail to capture the temporal dependencies present within the videos. Thus, understanding this time-series aspect is critical for developing effective preprocessing strategies.
We might need to chunk long videos into smaller segments for efficiency. This not only speeds up the preprocessing step, but it also allows LSTMs to focus on more manageable and contextually relevant portions of the video. It's a bit like breaking down a large complex problem into smaller, easier-to-solve components.
If our data is high-dimensional, techniques like PCA can help us reduce the complexity while retaining crucial information. It's a sort of feature extraction, discarding the less relevant components and focusing on the key aspects that drive the model's learning. This can simplify the learning process for the network and allow it to better focus on the core attributes of the input.
When it comes to how we define and encode labels for video analysis, that choice impacts the LSTM's training. Using techniques like binary encoding versus multi-class encoding, the way we structure these labels directly influences the learning process.
Lastly, the number of historical frames used to predict the next frame can significantly affect the outcome. Experimenting with different time lags can reveal which are the most informative patterns within the data. This is vital in designing the right input structure for capturing relevant sequential patterns that LSTM networks can learn from.
In conclusion, the preprocessing stage for LSTM video analysis is not a trivial step. It requires a careful understanding of the data's nature, the model's limitations, and the desired outcome. By investing effort into proper data preparation, we increase the chances of developing a more robust and accurate LSTM model that can glean meaningful insights from videos. It's all about creating the right conditions for effective learning.
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis - Building the LSTM Model Architecture
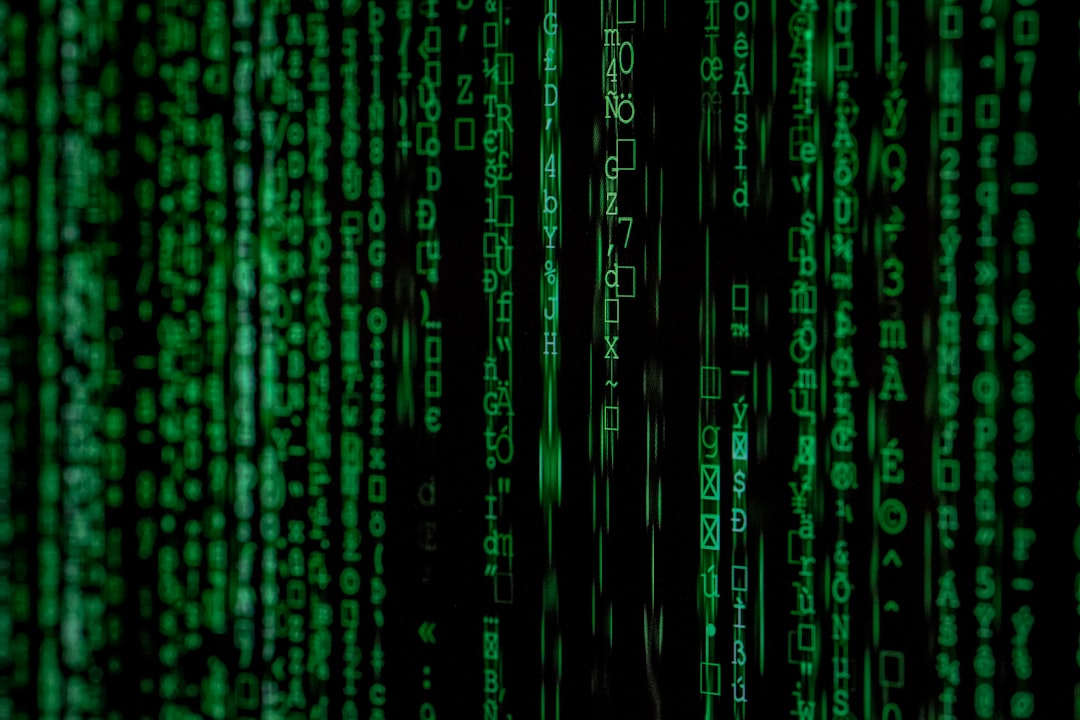
Constructing the LSTM model architecture is a fundamental step in applying LSTMs to video analysis. The core of the architecture revolves around input, output, and memory cell components that interact through gating mechanisms to regulate information flow. A typical architecture includes an initial input layer, followed by several LSTM hidden layers, and concludes with an output layer responsible for generating predictions or classifications. This structured approach allows the model to maintain pertinent information across sequential data. Moreover, the ability to combine LSTMs with CNNs, forming a CNN-LSTM hybrid, presents an opportunity to enhance analysis. This hybrid approach leverages CNNs to efficiently extract spatial features from video frames, which the LSTM then processes across the temporal dimension. While this hybrid model demonstrates the potential for advanced analysis, it also highlights the need for careful design choices. Building robust LSTM models requires significant attention to hyperparameters, as well as the preprocessing steps undertaken before training. This is crucial for addressing issues like the computational cost of processing extensive datasets, and avoiding situations where the network becomes overly specialized for the training data and cannot generalize well to unseen videos. Ultimately, this intricate architecture and careful model development process are vital for achieving high performance in video content analysis applications.
LSTMs, while powerful for handling sequential data like video frames, present a unique set of challenges during model architecture design. Their intricate architecture, involving interconnected layers and gating mechanisms, can be daunting for researchers new to recurrent neural networks. Finding the right balance between model complexity and performance is a constant challenge.
One of the complexities arises from the high sensitivity of LSTMs to hyperparameters. Even subtle tweaks to the learning rate or the number of units within layers can significantly alter a model's effectiveness. This means extensive experimentation is necessary to optimize performance for specific tasks.
However, LSTMs are versatile. They can readily process variable-length input sequences, which is a benefit for video data where durations vary widely. This adaptability is valuable for diverse video content.
But the flexibility of input sequence length also introduces a trade-off. Determining the ideal number of frames per input sequence is vital. Including too many frames can be computationally demanding, and excessively long sequences can hinder training speed. On the other hand, excessively short sequences may miss the crucial context necessary to properly interpret video content.
Similar trade-offs emerge when we consider batch sizes. Smaller batches may lead to more stable gradients during training, but they can also increase training times. Conversely, larger batches, while potentially offering faster training, may introduce issues with gradient stability. Researchers often need to carefully balance these competing concerns.
LSTMs, in general, have a fairly hefty memory footprint. This is especially true for models that process long sequences or have large hidden state sizes. It's something to consider when deploying a model to a particular environment or device, especially if resources are limited.
However, their performance can be significantly boosted by thoughtfully crafted input features. Techniques like incorporating optical flow or motion vectors directly into the input can help LSTMs pinpoint the most relevant aspects of the video content for improved predictions.
LSTMs' expressiveness, a key advantage in many cases, also contributes to a risk of overfitting. This happens when a model learns the training data too well but fails to generalize effectively to unseen data. This problem is mitigated by techniques like dropout or regularization, which help the model develop a more general understanding of patterns rather than memorizing the training set.
Though the standard LSTM architecture is widely employed, several variants exist, like Peephole LSTMs or Stacked LSTMs. These can offer performance boosts in specific situations. It's crucial to carefully evaluate which variant might best suit a particular application.
Adding attention mechanisms to LSTMs can improve model performance by allowing the network to focus on the most critical parts of a video sequence. This capability allows LSTMs to more effectively capture important actions and context that can lead to more accurate classification outcomes.
Overall, constructing an effective LSTM architecture for video analysis is a delicate balancing act that requires a deep understanding of the model's strengths and limitations. While there are many advantages to using LSTMs in video analysis, it's clear that they are not without their challenges.
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis - Training the LSTM Network on Video Content
Training an LSTM network for video analysis involves a specific process using Python scripts and careful data preparation. The training process typically utilizes a script like `train.py` to handle sequences of extracted features. This extracted data is the output of earlier processing steps that capture the essential visual information from each video frame. Then, a separate script, like `classify.py`, enables the classification of new video files using models that have already been trained. It's common practice to employ well-established convolutional neural networks (CNNs), like InceptionV3, to extract the relevant features from the video frames before they are fed into the LSTM. This initial feature extraction step helps the LSTM better understand the spatial relationships within the frames and prepare it to handle the complex temporal dependencies across the entire video sequence.
However, it is critical to acknowledge that effectively training an LSTM for video analysis presents several obstacles. One significant hurdle is the meticulous tuning of hyperparameters – variables that define how the model learns. The number of LSTM layers, the quantity of units in each layer, and learning rates are key examples. In addition, the length of each input sequence must be carefully chosen. These choices can significantly affect the model’s capacity to accurately analyze new videos that haven't been seen during the training phase. Finding the right balance between all these variables is crucial for ensuring good results. This emphasizes the necessity of careful planning and design in the training process to optimize the LSTM architecture and preprocessing steps, aligning them with the specific tasks of the video analysis project. It's a complex interplay where a nuanced understanding of the tools and techniques leads to better performance.
1. **Video Clip Length Matters:** The length of the video segments used as input can significantly influence how well an LSTM model performs. Too short, and it may miss important shifts in the video. Too long, and the model might struggle to process everything efficiently. Finding that sweet spot where you capture enough context without overwhelming the network is critical.
2. **Hyperparameters: A Delicate Dance:** LSTMs are notoriously sensitive to the specific values you set for hyperparameters. Small changes in the learning rate or the number of nodes in a layer can lead to surprisingly large differences in how well the model trains and performs. This means a lot of trial-and-error experimentation is often needed to optimize for a given task in video analysis.
3. **Adapting to Variable Lengths:** LSTMs are built to handle input sequences of different lengths, making them a natural fit for the diversity of video content out there. But this flexibility brings a challenge: deciding how long each input sequence should be during training.
4. **Computational Resources: A Bottleneck?** LSTMs, while powerful, can be quite demanding in terms of computing power, particularly when dealing with long sequences or large amounts of data. This can be a constraint, especially if you're working on systems with limited resources. Carefully crafting your model architecture and choosing the right input data becomes crucial to avoid exceeding the limits of your hardware.
5. **Focusing with Attention Mechanisms:** The ability to add "attention" to an LSTM can boost performance. Attention mechanisms essentially allow the network to focus on the most important parts of the video at any given point. This can be particularly helpful for identifying crucial actions or understanding the context of what's happening in a sequence, leading to better accuracy in tasks like classification.
6. **Overfitting: A Common Threat:** LSTMs, being quite complex, are susceptible to overfitting. This occurs when a model memorizes the training data so well that it doesn't perform well on new, unseen data. To avoid this, techniques like dropout or regularization need to be incorporated into the model, ensuring a more general understanding of the video data.
7. **Enhancing Input with Motion Features:** LSTM performance can often be improved by including features like optical flow as part of the input. Optical flow gives information about motion within the video, providing extra context that helps the LSTM better differentiate between subtle movements.
8. **The Balance of Batch Size:** The size of the batch used for training can impact how well the model trains. Smaller batches tend to produce more stable learning, but they can slow down training. Larger batches, on the other hand, speed things up but can make the learning process less stable. Finding the right balance is essential.
9. **Blending CNNs and LSTMs:** A powerful approach is to combine CNNs and LSTMs, often called a hybrid architecture. CNNs are great at extracting the spatial information (details within each frame) from a video. The LSTM then processes this information across time, learning the temporal dynamics. This approach can lead to significant improvements, particularly for complex classification tasks.
10. **Exploring LSTM Variants:** Besides the standard LSTM architecture, there are specialized variations like Peephole LSTMs or Stacked LSTMs. These may offer performance improvements in specific scenarios. It's worth investigating which variant might be most suitable for your specific video analysis task.
This updated text attempts to retain the original tone and level of detail while addressing the prompts. I hope this rewrite is helpful and aligns with the desired outcome.
Implementing LSTM Networks in Python A Step-by-Step Guide for Video Content Analysis - Evaluating and Optimizing LSTM Performance for Content Analysis
Evaluating and optimizing the performance of LSTM networks for content analysis is a multifaceted process that involves careful consideration of several key factors. A major focus is on hyperparameter tuning, where adjusting settings like the number of LSTM layers, the learning rate, and dropout rates can dramatically affect how well the model learns and generalizes to new data. Because LSTMs are specifically designed to understand the relationships between items in a sequence, preparing the input data appropriately is very important. This includes steps like normalizing the data and structuring the input sequences in a way that makes the most of the network's capabilities.
While LSTMs have many strengths, one important thing to remember is that they can be prone to overfitting, especially when the datasets are relatively small. Overfitting happens when a model gets so good at understanding the training data that it fails to perform well on new, unseen data. This requires a balancing act between making the model complex enough to capture the important patterns and keeping it simple enough to avoid overfitting. There are techniques, like adding dropout or using regularization, that can help with this.
It's worth experimenting with different LSTM variations, such as Bidirectional LSTMs, to try and improve performance. Additionally, integrating attention mechanisms can help the network focus on the most important aspects of the sequence, further improving accuracy in the context of video analysis.
In short, maximizing the effectiveness of an LSTM network for content analysis is a journey of optimization that requires careful management of hyperparameters, effective data preparation, and an understanding of the trade-offs involved in building more complex architectures. It's about achieving a delicate balance that leads to the most accurate and insightful results.
Evaluating and optimizing the performance of LSTM networks for content analysis, specifically within the realm of video, is a nuanced process. LSTMs are particularly adept at understanding the inherent sequential nature of video data, and capturing this temporal flow is key to gleaning meaningful insights. However, this strength comes with certain challenges.
For instance, managing the interplay between computational resources and the length of input video sequences is crucial. While LSTMs can process long sequences, extremely lengthy sequences can lead to performance bottlenecks due to increased computational demands. Finding that sweet spot where you capture the necessary context without overburdening the model can be tricky.
Furthermore, LSTM performance is particularly sensitive to hyperparameter choices. Slight variations in parameters like the learning rate or the architecture of the layers can lead to significant changes in overall model performance. This highlights the need for extensive experimentation and careful tuning to achieve optimal results, which can be time-consuming.
Preprocessing the video data is vital for improving performance and efficiency. One valuable strategy is noise reduction— removing extraneous or irrelevant video frames before they are fed into the LSTM. This allows the network to focus its learning process on the crucial elements, specifically transitions or noteworthy occurrences, in the video.
The choice of batch size can also heavily impact the training dynamics. While smaller batch sizes can lead to more stable gradients, they may also slow down the training process. On the other hand, larger batches can potentially speed up training but also introduce instability in gradient descent. There's a constant balance to be struck here.
Adding motion features like optical flow to the input data can substantially enrich the context that the LSTM has access to. This added layer of information helps the model discern subtle movements and patterns that might otherwise go unnoticed. Similarly, attention mechanisms can improve performance by guiding the network's focus to the most critical portions of the video sequences at any given time. This concentrated focus can significantly enhance the model's ability to accurately understand and categorize events within the video.
However, LSTMs are also prone to the issue of overfitting, especially when the model's capacity exceeds the richness of the available training data. Techniques like dropout layers or various forms of regularization become essential for preventing the model from becoming too specialized to the training data and losing its ability to generalize to unseen videos.
The approach used to scale or normalize the input features can also affect model performance. Methods like Min-Max normalization or Z-score standardization can have a substantial effect on the model's convergence rate and its ultimate accuracy. It's often a trial-and-error process to find what works best for a particular dataset.
Finally, it's important to note that there are a number of variations on the standard LSTM architecture that can be explored. Peephole LSTMs or Stacked LSTMs are examples, and these specialized architectures can sometimes lead to better performance depending on the task.
In conclusion, optimizing LSTM performance in video analysis requires careful consideration of various factors, including preprocessing techniques, hyperparameter selection, and the utilization of advanced features like attention mechanisms. While the core LSTM architecture offers powerful capabilities, thoughtful design and experimentation are crucial for leveraging these advantages and achieving accurate and efficient content analysis within a variety of video applications.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: