7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - List Comprehension for Quick Frame Analysis in Any 60fps Video Dataset
When analyzing videos captured at 60 frames per second, the sheer volume of data can overwhelm traditional methods. Python's list comprehension feature offers a solution by providing a compact way to manipulate and filter frame data. Imagine you're working with a large dataset like Inter4K, containing numerous high-resolution videos. List comprehension can quickly sift through frames, extract specific information, or prepare data for further analysis, potentially speeding up your analysis pipeline. This approach proves particularly helpful in situations where you need to efficiently process frame data, such as when combining data from multiple sources using Pandas. Essentially, list comprehension empowers video analysts to streamline their workflows when facing the challenges of large video datasets, ultimately leading to faster and more effective analysis. While handling long, untrimmed videos requires more sophisticated methods compared to shorter, focused studies, incorporating list comprehension into your toolkit provides a valuable advantage.
1. List comprehension provides a compact way to build new lists from existing data structures like video frames, streamlining the process within video analysis workflows. This approach can make your Python code more readable and easier to understand, particularly when dealing with frame-by-frame analysis.
2. The speed benefits of list comprehension are noticeable, particularly when working with large datasets or high frame rates like 60fps. While it's not a universal speedup, the reduction in runtime compared to traditional looping methods is often substantial, potentially resulting in a 4-5x improvement.
3. The concise structure of list comprehension can help prevent errors often associated with loop counters and indices. This is valuable in avoiding off-by-one errors, especially when manipulating frame sequences.
4. The compact and focused nature of list comprehension can greatly enhance code clarity and team collaboration. This improved readability leads to smoother debugging, maintenance, and modifications when working on complex video analytics tasks.
5. Filtering out specific frames based on certain criteria becomes straightforward with list comprehensions. You can apply conditions directly within the list creation process, allowing for the rapid extraction of relevant segments from the video, effectively acting as a targeted frame selection mechanism.
6. List comprehension seamlessly incorporates function calls, allowing for sophisticated frame-level operations during the list creation itself. This "on-the-fly" processing capability keeps your code more focused and reduces the need for separate processing stages.
7. The synergy between NumPy arrays and list comprehensions can lead to faster computations within video analytics. This is very helpful when dealing with numerical operations on video frames, especially in applications involving motion detection, object tracking, or other frame-by-frame calculations.
8. You can nest list comprehensions to process multidimensional structures. This is handy when analyzing features derived from multiple frames or when handling video data with multiple dimensions of features. However, understand that the complexity increases as you nest, and can make it difficult to follow the code.
9. List comprehension exemplifies the functional programming style in Python, encouraging a style of writing code that emphasizes immutability and avoids side effects. This functional approach tends to generate cleaner code, especially in the context of handling and processing frame sequences.
10. While powerful, list comprehension can create large lists in memory. When analyzing massive datasets, it's wise to consider the potential memory implications, particularly for resource-constrained setups. Generator expressions are a memory-friendly alternative to consider in those situations.
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - NumPy Broadcasting to Process 4K Resolution Matrices
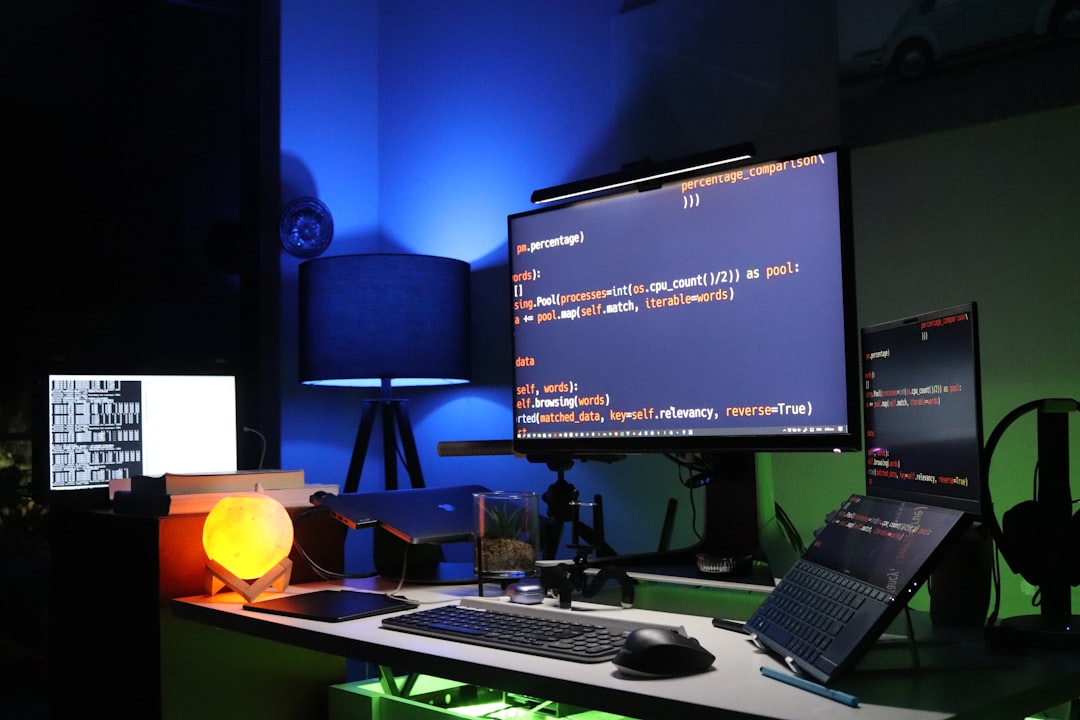
NumPy's broadcasting capability is a game-changer when dealing with the massive matrices found in 4K video resolutions. Essentially, it lets you perform arithmetic operations between arrays of different shapes, automatically expanding smaller arrays to match the larger ones. This avoids the need to manually replicate data, leading to cleaner and faster code. The core principle is straightforward: two dimensions are compatible if they're the same or if one is 1. Understanding and applying this compatibility rule is essential for utilizing broadcasting effectively.
Broadcasting is invaluable for video analysis due to the sheer volume of data involved in processing high-resolution videos. Mathematical operations on these datasets become significantly more efficient. It becomes especially relevant in the modern landscape where 4K and even higher resolutions are becoming increasingly common. For analysts aiming to extract insights quickly and accurately from these large datasets, mastering broadcasting is not just an advantage, but arguably a necessity. While powerful, like any tool, it's not a magic bullet, and one still has to understand how it functions under the hood to avoid errors.
### Surprising Facts About NumPy Broadcasting to Process 4K Resolution Matrices
1. **Element-wise Operations Made Easy**: NumPy's broadcasting feature enables us to perform calculations on arrays with differing shapes, which is quite handy when dealing with the massive pixel grids found in 4K videos (those with a 3840 x 2160 resolution). It allows us to bypass explicit for-loops, simplifying our code considerably.
2. **Memory Management**: Through broadcasting, NumPy can conduct operations without creating extra copies of large datasets. This is especially helpful for 4K video frame processing, leading to reduced memory consumption and the ability to handle computationally intensive tasks that might normally strain memory.
3. **Performance Gains**: Broadcasting can boost speed considerably, leveraging optimized C and Fortran code under the hood. When utilized with extensive video matrices, we might see performance improvements by several orders of magnitude compared to basic implementations that don't leverage broadcasting strategies.
4. **Rules of the Game**: Grasping broadcasting rules, particularly how NumPy aligns array shapes, is crucial for avoiding typical issues. For example, you can only broadcast two arrays together if their dimensions are compatible, with compatibility being dependent on the rightmost dimension of each array.
5. **Color Tweaking Made Simple**: In video analysis, broadcasting simplifies color manipulation of high-resolution images. For instance, adjusting brightness or contrast uniformly across all pixels in a 4K frame can be achieved easily by broadcasting a scalar or a smaller array to the entire image.
6. **Dimensionality Magic**: Broadcasting allows us to seamlessly expand the dimensions of smaller arrays to match the dimensions of our high-resolution matrices. This means we can readily apply operations using 1D arrays, or even scalar values, across 2D images which streamlines tasks like normalization or adjusting color hues.
7. **Powering Complex Math**: Broadcasting proves remarkably useful for executing complex mathematical operations on matrices such as Fourier transforms or convolutions without the need for manual loops. This is vital in video analysis where frame-by-frame transformations are needed either in real-time or close to it.
8. **Visualizing Changes in a Flash**: When modifying large pixel grids, broadcasting empowers us to see the changes across a video sequence rapidly. We can implement masks or overlays that apply directly to video frames without the added overhead of rearranging or duplicating data.
9. **Fewer Coding Errors**: Broadcasting reduces the chances of coding errors when working with large matrices. By eliminating nested loops and replacing them with straightforward operations, our code stays clean and maintainable, which is key for collaborative video analysis.
10. **Knowing the Limits**: Recognizing the limitations of broadcasting, especially when dealing with exceptionally high-dimensional datasets, is important. When applied to complex data structures, broadcasting might sometimes yield unexpected outputs if the array shapes don't match up as anticipated. Thus, we must always rigorously test array shapes before running operations.
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - Pandas DataFrame Batch Operations for Multiple Video Files
Pandas DataFrames are extremely useful for handling data from multiple video files because they allow for efficient batch operations. Video analysts can readily load data into a DataFrame using functions like `read_csv`, which is a common format for video data. Quickly examining the loaded data is possible with functions like `head()` and `describe()`, giving a preliminary sense of the data structure and statistical properties. When working with non-integer or non-consecutive indexes, `groupby` paired with `nparange()` enables efficient batch processing. Before deep analysis, the `info()` method is helpful for ensuring data quality by providing information on column data types and the presence of missing values. To accelerate processing for large datasets, techniques like using the `multiprocessing` module can distribute the workload across multiple processors, significantly speeding up calculations of summary statistics. This set of features offers a strong foundation for handling and analyzing data from multiple video sources. It's important to remember that data analysis always requires careful consideration, especially for video data, as often the results will depend on the assumptions one makes.
Pandas DataFrames offer a powerful way to handle multiple video files at once, especially when you're dealing with a lot of data. You can process multiple files in batches, which is generally much faster than processing them one by one, particularly if you're using vectorized operations that are built into Pandas.
One challenge with large video datasets is that they can use a lot of memory. Pandas offers chunking as a solution, where you can break up your data into smaller chunks and process those. This can be quite helpful for running analyses on machines with limited RAM.
Missing data is quite common in video analysis. Thankfully, Pandas is designed to handle that. You can easily fill in, remove, or even interpolate missing values without a lot of extra pre-processing steps, keeping your data cleaner and more reliable for the analysis stage.
When working with video timestamps in metadata, Pandas' tools for manipulating dates and times can be really useful. You can easily filter or aggregate data based on specific time frames, such as identifying events during certain intervals within a video.
Pandas DataFrames play well with other popular libraries, such as NumPy for numerical operations and Matplotlib for plotting. This seamless integration can streamline your entire video analysis workflow, from extraction of data to visualizing results.
The `groupby` method is extremely helpful for segmenting data based on things like different shots in a video or changes between scenes. This targeted analysis is very helpful for digging into specific parts of a video without a lot of clunky looping.
Pandas allows you to define and apply your own custom functions to the columns of a DataFrame using `apply()`. This customizability is valuable for tasks that require specialized video processing algorithms.
When you need to combine data from multiple videos – say, combining metadata or results from several analyses – Pandas provides efficient ways to merge DataFrames. This capability makes it straightforward to create a consolidated view of your dataset from multiple sources.
Pandas is designed with efficiency in mind, which makes it suitable for doing manipulations or analyses of video data in real-time. This can make applications built on it more responsive and suitable for tasks that need quick insights.
Pandas lets you plot directly from the DataFrame itself. This allows you to quickly generate visualizations and get a sense of the overall trends, distributions, and potential outliers in the video data without having to export the data first. This helps in the exploratory analysis phase.
While Pandas isn't a silver bullet, it provides a solid base for many video analysis tasks, especially when it comes to handling the complexities of multiple files and large datasets. For engineers or researchers working on video, Pandas offers a useful set of capabilities that can accelerate data exploration and improve the efficiency of their pipeline.
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - Matplotlib Time Series Plots for Frame Rate Analysis
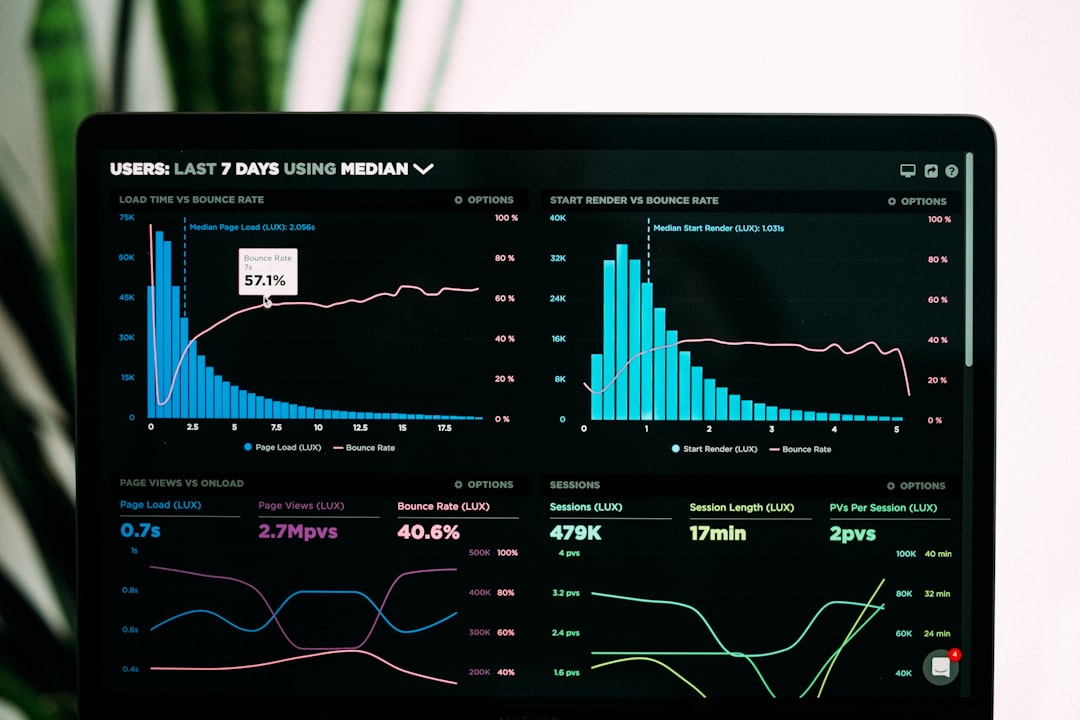
Matplotlib offers a powerful way to visualize frame rate data over time using time series plots. These plots are particularly useful for video analysts, as they allow for quick identification of trends, changes, and other patterns in frame rate data. The basic setup involves placing time on the horizontal axis (X-axis) and frame rate on the vertical axis (Y-axis). This simple visualization can be very revealing, especially when it comes to understanding how frame rates change throughout a video.
If you need to compare different frame rate data sets, Matplotlib's `plt.plot` function can easily overlay multiple datasets onto a single graph. This helps illustrate how things like encoding changes, different resolutions, or network conditions impact the frame rate. To make these plots more informative, it's valuable to customize them with features like titles, axis labels, and grid lines. These small additions help improve the clarity and interpretability of the visualizations.
Overall, Matplotlib provides an essential set of tools for creating informative time series visualizations. This capability is important for video analysts who need to understand how frame rate fluctuates or changes over time. This understanding is central to understanding the performance of video and ultimately forms the basis for many important decisions in the process of analyzing and working with video data. While simple to create, time series plots from Matplotlib offer a powerful way to extract useful insights from video data and support the use of video data to support conclusions based on evidence.
Matplotlib, a widely used Python plotting library, proves exceptionally useful for generating time series plots, a key technique for analyzing frame rates in video data. Time series plots, where time is represented on the horizontal axis and a measured value (in our case, frame rate) on the vertical, are essential for uncovering trends, repetitive patterns, and seasonal variations within data.
The `plt.plot` function, central to Matplotlib, enables us to create these graphs. We can easily visualize multiple frame rate datasets on a single plot by simply calling this function repeatedly, with each call using a different data source. Creating informative plots hinges on using functions such as `plt.title`, `plt.xlabel`, and `plt.ylabel` to clearly label the plot, its horizontal (time), and vertical (frame rate) axes. To further improve the readability and interpretation of the plots, it's crucial to customize them. This might involve adding grids for better orientation or markers to easily identify specific data points within the plot.
When working with frame rate data in Python, dataframes, using the `pd.date_range` function to denote specific time ranges, are a popular and very useful data structure. This allows us to more readily align frames with their respective capture timestamps. Basic time series plots are truly fundamental within the video analysis domain, and Matplotlib makes them surprisingly easy to generate. The code required is often concise, demonstrating that building strong visual representations of data can be very easy with the right tools.
Understanding how frame rate patterns change over time is crucial for anyone working with video data. This is especially true in today's environment where the expectation for high quality and consistent video is higher than ever. Matplotlib makes this level of analysis achievable, allowing you to create custom visualizations tailored to the specific insights you're seeking. While it's possible to generate these kinds of graphs with other tools, Matplotlib remains a very common tool, with a good track record.
It's worth noting that even with relatively high frame rates (like 60fps), Matplotlib effectively handles sub-second timestamps, allowing for detailed analysis of how frame delivery impacts video quality. This is crucial in situations like live streaming, where even minor delays can noticeably affect the user experience. In exploring video performance, you may encounter wide variability in frame rates. Matplotlib's capability to overlay numerous datasets on a single plot lets us identify overarching trends and differences across various video types or configurations.
Furthermore, you can integrate visual cues (markers) into these time series plots to associate dips in frame rate with specific actions within the video. This is important because it helps with debugging - where the cause of any frame rate problems are located in the video data or the pipeline processing it. Matplotlib also allows annotations, which gives us the chance to provide context to any frame rate fluctuations seen in the plots. These customizations can be very useful in making plots that are more informative to both technical and non-technical audiences.
Customizing the aesthetics of the plots using Matplotlib's versatile customization features helps to highlight critical trends and metrics, making these results easy to understand for both individuals and teams. It's possible to go even further and make these static plots interactive, using libraries like `%matplotlib notebook` or Plotly. This adds another level of detail because one can zoom in and explore specific sections of the video more readily.
When dealing with substantial quantities of high-resolution frame data, memory consumption can be a real concern. However, Matplotlib includes methods for optimizing rendering to help control memory usage, allowing analysts to continue analyzing datasets that might otherwise be too large to process. It's common practice for analysts to script their workflows so that time series plots are automatically generated and updated. This kind of automation proves useful in production settings for regularly monitoring frame rates.
The tools provided by Matplotlib for time series analysis are valuable for researchers and engineers working with video data. It provides a foundation for a deeper level of video performance analysis, which allows engineers to identify potential issues, evaluate different configurations, and optimize the overall video experience for viewers. While there are some limits to what it can do, Matplotlib remains a very robust tool that can help greatly with frame rate analysis.
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - Scikit-learn Model Training for Video Classification
Scikit-learn is a valuable tool for video analysts needing to apply machine learning techniques to classify videos. This open-source Python library streamlines the process of using various machine learning algorithms, both supervised and unsupervised, to train and evaluate classification models. Key aspects include using GridSearchCV for tuning model settings and robust evaluation metrics like accuracy, precision, and recall. Preprocessing video data and extracting useful features is crucial to creating reliable and accurate models. Combining Scikit-learn with other Python libraries like NumPy and Matplotlib improves the entire data science process, solidifying its importance in today's video analysis approaches. While it provides many useful tools, its usefulness will vary depending on the task, the size of the dataset, and the complexity of the video analysis. It is crucial to understand how Scikit-learn functions, especially if complex or very large datasets are used.
Scikit-learn, a widely-used Python library for machine learning, offers a robust framework for building and evaluating video classification models. It's known for its simplicity and supports a broad range of algorithms, including both supervised and unsupervised methods. The foundation of any model in Scikit-learn relies on training and test data, the choice of a model, and evaluation metrics to assess performance.
One popular classification approach within Scikit-learn is Random Forest, which excels in handling diverse datasets for classification tasks. However, effectively training any model hinges on careful feature selection and engineering. In video classification, it appears that motion-based features (like frame differences) often outperform simple pixel-based ones. This implies that incorporating temporal dynamics into the features can improve prediction.
Furthermore, Scikit-learn's ability to leverage pretrained models from Keras, a deep learning library, opens doors to transfer learning. This technique can speed up training and enhance accuracy by fine-tuning models on smaller, specific video datasets.
The high dimensionality of video data necessitates careful consideration of dimensionality reduction. Techniques like Principal Component Analysis (PCA) can be used to decrease complexity and reduce overfitting by reducing the number of dimensions in the data without losing critical information, which speeds up training.
It's crucial to implement proper cross-validation to assess how generalizable the resulting model is across different video clips. K-fold cross-validation can help address issues of overfitting, particularly important given the diverse nature of video data.
Ensemble methods like Random Forests and Gradient Boosting can produce significant gains in classification accuracy. They combine the predictions of several models to learn complex patterns within video data, often outperforming single models. However, finding the best configuration of the model's settings or hyperparameters can be a challenge. Scikit-learn's GridSearchCV simplifies hyperparameter tuning via cross-validation, which can boost the effectiveness of the trained model by a significant amount.
One of the limitations of Scikit-learn for video analysis is its traditional focus on batch processing, making it less suitable for real-time video classification tasks. If real-time applications are needed, then the pipeline design has to be thoughtfully constructed.
Beyond simple frame analysis, understanding and modeling the temporal nature of videos is also important. Using techniques like LSTMs, which are a type of recurrent neural network, within a Scikit-learn pipeline can provide a means to capture the dynamic nature of video and potentially unlock a deeper level of understanding.
Expanding the training dataset using data augmentation methods is another helpful technique. Randomly manipulating training data, like cropping or flipping frames, can help improve the robustness of the trained models. Although these manipulations aren't directly part of Scikit-learn, they can be incorporated into the workflow easily.
Finally, the choice of evaluation metrics is crucial. Using metrics like precision, recall, or F1-score instead of simply accuracy is often more helpful when assessing model performance, particularly with datasets that have an unequal distribution of classes, which is common in video data.
While Scikit-learn presents an accessible framework for building video classification models, researchers need to be aware of the complexities inherent to the data, including the importance of feature engineering, efficient model training, and the careful selection of evaluation metrics. Addressing these challenges effectively allows Scikit-learn to be used to unlock deeper insights into video data.
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - Apply Function for Custom Video Metadata Processing
Within the realm of video analysis, the ability to customize how metadata is processed is crucial. This is where the `apply()` function within Pandas comes into play. It allows you to efficiently modify or create new data columns in a DataFrame, letting you adapt to the specific quirks of different video metadata formats. Video metadata can be quite varied, and this approach makes it much easier to manipulate and analyze the data without writing a bunch of loops. However, keep in mind that performance can be a concern if you're working with very large datasets. It's worth thinking about the best way to avoid making the whole process slow. This is a prime example of the versatility of Python; it lets analysts use their own custom functions without much hassle. While generally very useful, it requires a bit of thought to use correctly if dealing with enormous datasets.
The `apply` function within Pandas provides a flexible way to perform custom operations on video metadata stored in DataFrames. This becomes particularly useful when you need to extract specific information from metadata, like timestamps or video durations, or when you need to perform unique calculations on metadata.
However, while versatile, `apply` can present performance challenges when working with very large datasets. Unlike vectorized operations within Pandas, which are often highly optimized, `apply` can be slower because it involves calling Python functions for each row or column, adding processing overhead.
One way to simplify custom operations within `apply` is to use lambda functions. These compact functions allow you to implement straightforward transformations in a single line, which can be convenient. But, it's easy to over-simplify with lambdas to the point where it can become difficult to follow what is happening.
Moreover, using `apply` can lead to increased memory consumption, especially if the functions being applied modify the DataFrame. This can become a problem with very large video files.
It's important to be mindful of data types when working with `apply`. Pandas often attempts to guess the data type of the output, and this can lead to unexpected results if the output isn't consistent across rows or columns.
Beyond row or column-wise transformations, `apply` also enables custom aggregations of metadata. For instance, you can calculate summary statistics (average, sum, etc.) for different video clips. However, as the logic inside your custom functions increases in complexity, so too does the potential for things to go wrong. To prevent disruptions, error handling within these custom functions is advisable.
The choice of whether to apply functions row-wise or column-wise is important for getting the right result. This gives you more control over how your custom function manipulates the data.
Results generated with `apply` can readily integrate with other libraries for further analysis and visualization, offering a streamlined process. For example, `apply` results can be merged with NumPy arrays or visualized with Matplotlib.
Keep in mind, however, that relying heavily on `apply` for large DataFrames can result in performance overhead compared to directly manipulating NumPy arrays. In scenarios involving extensive numerical operations, it might be more efficient to switch to using NumPy's features directly or alongside `apply` to improve the performance of your processing.
In summary, while the `apply` function can be valuable for custom metadata transformations in video analysis, engineers and researchers should carefully consider the potential trade-offs in terms of performance and memory usage, especially when handling massive datasets. This careful attention can help ensure your video analysis pipelines are both flexible and efficient.
7 Essential Python Data Science Functions That Every Video Analyst Should Know in 2024 - Image Array Manipulation with Built-in Math Functions
Within the realm of video analysis, the ability to directly manipulate image data represented as arrays is essential. This often involves applying built-in mathematical functions provided by libraries like NumPy and SciPy. These libraries equip video analysts with tools to perform a wide range of operations on image data, such as filtering, transformations, and various mathematical adjustments. These manipulations are especially important when dealing with the vast quantities of data found in video, making analysis both faster and potentially more accurate. Further, understanding and implementing different color spaces helps analysts finely control visual information, which is useful for tasks related to image quality and manipulation. While these libraries offer considerable power, it's critical to develop a robust understanding of their nuances and functionalities to avoid errors and achieve the desired outcomes, especially in more complex situations involving large datasets.
Python's built-in math functions, when used with image arrays, can be a real asset in video analysis. It's often overlooked, but they provide a way to perform operations on images without needing to write out explicit loops. This can be a significant performance boost and leads to simpler, more readable code.
It might seem counterintuitive, but even though NumPy is frequently associated with array broadcasting, the built-in math functions can also work on arrays, making it easier to manipulate image data without the need for writing out loops for each pixel. This feature can be very helpful for improving the speed of image processing, especially if you are doing complex operations.
Another advantage is that many of these functions enable in-place operations, which helps keep the memory footprint relatively small. This is useful for high-resolution images, where avoiding creating duplicates can be important for keeping things running smoothly.
Furthermore, many of the built-in functions offer element-wise calculations, making it easy to apply things like squaring pixel values or adjusting the brightness across the entire image at the same time. When you compare it to traditional iterative methods, this approach is much more efficient, potentially leading to substantial improvements in processing time.
Interestingly, Python's built-in functions aren't limited to simple addition, subtraction, multiplication, or division; they also offer more complex operations like logarithmic or trigonometric calculations. This opens up the possibility of using more advanced image processing techniques, like edge detection or contrast enhancement. In video analysis, these advanced techniques can be incredibly useful.
One important consideration is the built-in functions’ support for multidimensional arrays. This makes it easier to work with color images (since they're represented as 3D arrays), allowing for individual manipulations on each color channel. This can simplify things greatly when applying complex transformations.
Another key aspect is that they integrate well with other libraries, like OpenCV and PIL, making for a smoother image processing workflow. For video analysts who frequently manipulate image metadata and pixel values, this seamless integration is a major benefit.
The ability to avoid using explicit loops can also minimize the chances of coding errors that frequently pop up in image processing. Things like off-by-one errors, which can be very difficult to track down, become less of a concern, particularly in more complicated image manipulation tasks.
It's also worth noting that these functions can support images with a wide range of dimensions, which means they can readily be used for tasks like dynamically resizing images. This is a big plus for video analysis, as it can help streamline situations where you need to make sure frames have consistent resolution across datasets that have images with different resolution.
The built-in functions frequently take advantage of vectorization, which leads to significant speed enhancements when you're processing images in batches. Tasks like histogram equalization or pixel intensity adjustments are particularly well-suited for vectorized operations, potentially leading to real-time image processing of video frames.
Finally, one subtle but important feature is that the functions automatically handle type promotion. So, if you have integer and floating-point data types in your arrays, they'll intelligently handle them. This helps ensure you don't lose data unintentionally while performing your manipulations. This is helpful when working on tasks where maintaining image quality is a priority.
While there are limits to the built-in functions, they provide a very practical set of capabilities that can significantly improve the way one works with images in video analysis. For researchers and engineers, taking advantage of them can make your image processing tasks easier and faster, allowing you to focus on the aspects of your project that require deeper expertise.
More Posts from whatsinmy.video: