Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Python Regex Mastery 7 Essential Patterns for Video Content Analysis
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Extracting timestamps from video subtitles using \d{2} -\d{2} -\d{2}
When dealing with video subtitles, you often encounter timestamps embedded within the text. A common timestamp format is `\d{2}-\d{2}-\d{2}`, representing two digits for hours, minutes, and seconds separated by hyphens. This particular regex pattern allows us to effectively target these timestamps and isolate them from the surrounding text. Python's `re` module offers the tools to implement this regex pattern, enabling the extraction of these time indicators from subtitle files.
The `\d{2}` part of the pattern specifically targets two-digit numbers, making it well-suited for representing time components like hours, minutes, and seconds. The hyphen (`-`) acts as a delimiter, ensuring that the pattern only matches timestamps with this specific structure. This approach simplifies the process of locating and extracting relevant information within subtitle files, a crucial step for many video analysis workflows.
Essentially, the ability to utilize regex patterns like `\d{2}-\d{2}-\d{2}` allows developers to refine their data manipulation techniques, especially within contexts that involve analyzing video content. This kind of pattern matching is an invaluable tool, especially when dealing with large volumes of subtitle data where efficient processing and extraction are essential. It underlines the significance of incorporating regex skills into your programming toolkit.
1. Using the regular expression `\d{2}-\d{2}-\d{2}` for extracting timestamps from video subtitles can be a fast and effective way to isolate date-like patterns within the text. However, the simplicity of the pattern can be both a strength and weakness, depending on the data.
2. While the pattern matches a specific format, it doesn't inherently know if it's dealing with a genuine timestamp or just a sequence of numbers. Something like '99-99-99' would also match, underscoring the importance of having some sort of validation step after the initial regex match.
3. While timestamps in video subtitles often follow a relatively standard structure including hours, minutes, and seconds, content creators don't always play by the rules. Consequently, a robust regex approach has to account for the possibility of unusual timestamp formats and non-standard configurations.
4. When working with extracted timestamps, it's vital to remember that the encoding format of the video file can influence subtitle timing accuracy. The timestamp we extract might not line up with the video content correctly if there's a discrepancy between how the subtitles were encoded and how the video itself handles timing.
5. Python's `re` module is well-suited for regex operations on subtitle files, particularly large ones. In comparison to languages like JavaScript, Python offers advantages due to its memory management and optimized search features, which can be crucial when dealing with substantial datasets.
6. Robust error handling is crucial in this domain. Subtitle files can be prone to errors (corrupted data, formatting inconsistencies), and if we blindly rely on a simple regex approach, a poorly formatted timestamp can potentially break the whole process. Incorporating error checks and validation is essential for creating a more robust system.
7. Interestingly, different languages can influence timestamp formatting within subtitles. Certain regional conventions may differ from the expected `\d{2}-\d{2}-\d{2}` pattern, making a one-size-fits-all approach problematic. Regex strategies must be flexible enough to handle various subtitle formats and languages.
8. Subtitle files can sometimes employ nested formats where timestamps aren't just pure numbers but mixed with other text. This requires more complex regex approaches, involving multiple patterns or more intricate capturing groups to fully extract the information we're after.
9. Reliance on regex alone for timestamp extraction is not always perfect. It's easy to get false positives, especially when numeric strings appear within dialogue. Incorporating contextual information or using other parsing techniques can help reduce the number of incorrect matches.
10. Video editing software commonly relies on subtitle information to incorporate timestamp features. As a result, the accuracy of our timestamp extraction process is directly related to the usability of the software that uses it. If the extracted timestamps don't align with the video, it can lead to wasted time and potential frustration for users trying to edit or interact with the video. Without automation, manually verifying these timestamps can become very tedious.
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Identifying speaker names with \b[A-Z][a-z]+ [A-Z][a-z]+\b
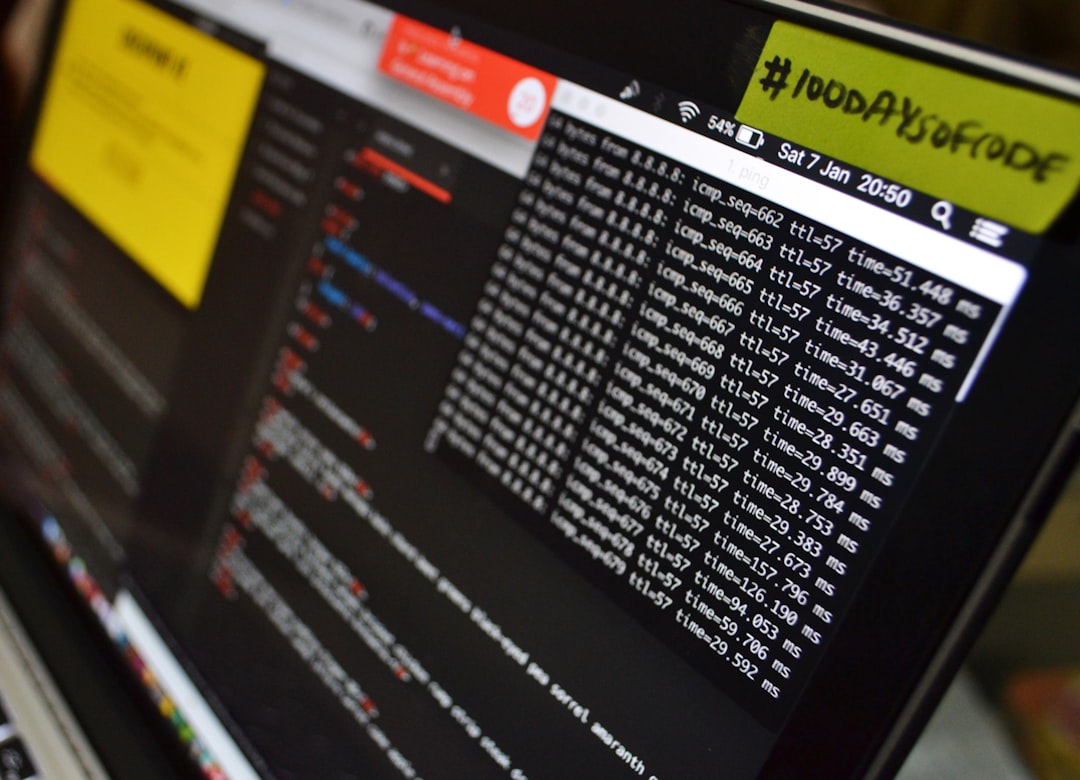
The regex pattern `\b[A-Z][a-z]+ [A-Z][a-z]+\b` is a useful tool for identifying speaker names in text. It specifically targets names formatted as two capitalized words, which is a common convention. This makes it particularly useful for analyzing video content, like transcripts or dialogues, where you want to quickly isolate who is speaking. However, it's important to recognize the limitations of this simple pattern. Real-world names are diverse, sometimes including special characters, multiple words, or even non-standard capitalization. For those cases, you might need to develop more elaborate regex expressions or combine the regex with additional data checks. Essentially, regex expertise provides a more robust approach to data extraction, allowing for more precise and sophisticated analyses of video content. By understanding these patterns and their limitations, you can gain a powerful edge in your video content analysis efforts.
1. **Focusing on Capitalized Words:** The regex `\b[A-Z][a-z]+ [A-Z][a-z]+\b` is designed to specifically find speaker names in text by looking for patterns of capitalized words, a common characteristic of names, particularly in Western contexts like English. This pattern is quite useful in situations where you're analyzing video subtitles where speaker names are typically written out.
2. **Not a Universal Solution:** While the pattern works well for many names, it's not without limitations when applied globally. If you're dealing with languages or cultural naming customs where names aren't structured as "First Last" with capitalization, this simple regex won't capture all the speaker names, highlighting the need for flexibility in our regex approaches.
3. **Brand Names vs. People**: This regex pattern, focused as it is on capitalized words, might also pick up on brand names or fictional character names, which can introduce errors in content analysis if we're not careful. We might end up mistakenly thinking a brand is a speaker in our data, so there's a need to be mindful of that potential problem.
4. **Case Sensitivity**: The pattern, like most regexes by default, is case sensitive. Meaning it won't correctly recognize names like "McDonald" or "O'Connor" without us adapting the pattern to accommodate these cases. It's important to keep this in mind and modify our regex pattern if we want a more robust solution to handle these situations.
5. **Scaling for Large Videos**: While regex is generally fast for finding things in text, if we start applying it to huge video libraries with massive subtitle files, performance can be affected. It's crucial to think about these kinds of performance issues as the datasets we're working with increase in size.
6. **Unintentional Matches:** Just like the timestamp issue, there's a chance of the regex picking out phrases that fit the pattern but aren't actually names, such as "A B" appearing in a descriptive phrase. It illustrates the value of trying to combine our regex techniques with some understanding of the text to reduce those types of mistakes.
7. **Dealing with Multiple Languages**: This particular regex is heavily biased towards names in English. As video content increasingly originates from all over the world, there's a real need for regex patterns that can handle names in different languages, which requires a more intricate approach.
8. **Limited Understanding of Context**: A core limitation of regex is its lack of understanding beyond simple pattern matching. Regex can't figure out the speaker's role in a conversation or their tone of voice, so it's not ideal when the goal is to analyze video content in a more sophisticated way.
9. **Collaborating with Other Tools**: We can make regex work even better by using tools designed for natural language processing, like spaCy or NLTK, which use machine learning models for name recognition. Combining regex and these kinds of AI approaches gives us a better chance of accurately finding speakers.
10. **Impact on User Interface**: When we're trying to make video content more accessible, like providing captions for hearing-impaired viewers, it's super important that we accurately identify who's speaking. If the names are wrong or messed up, users won't understand what's going on, reminding us how important correct regex usage is for user experience.
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Detecting scene changes through (?i)cut to|fade (in|out)
Scene changes in video are often marked by transitions like cuts and fades. Identifying these transitions is crucial for tasks like video analysis and editing. The regular expression `(?i)cut to|fade (in|out)` effectively targets these common transitions within text descriptions or subtitles. This regex, with its case-insensitive nature, allows us to quickly locate instances of "cut to" or "fade in/out".
While this regex provides a basic method for finding scene changes, tools like OpenCV or specialized libraries like PySceneDetect can provide more advanced functionalities. These libraries often leverage techniques such as frame-by-frame comparisons or threshold-based analysis to accurately detect fade effects or other more subtle transitions that regex alone might miss. In addition, video formats themselves can have diverse transition styles, demanding that scene detection methodologies be robust and adaptable. Ultimately, combining the power of regex for pattern recognition with the capabilities of specialized video analysis tools is an efficient approach to automate and improve the process of extracting scene change information, allowing for more insightful and efficient video content analysis.
1. **Capturing Scene Shifts**: The regular expression `(?i)cut to|fade (in|out)` aims to identify common cues used to signal scene changes within video scripts and subtitles. These cues, like "cut to" or "fade out," are frequently used to indicate shifts in a story or location, offering a potential pathway for efficiently analyzing video content.
2. **Language Diversity**: The way scene changes are described in scripts can vary wildly. While "cut to" and "fade" are standard, some writers may employ alternative phrases like "switch to" or "transition". Adapting the regex to accommodate this diversity in terminology would be crucial to make it more robust.
3. **Ignoring Case with `(?i)`**: The inclusion of `(?i)` within the regex allows for case-insensitive matching. This flexibility is critical when analyzing different types of scripts where capitalization can be inconsistent or even irrelevant.
4. **The Peril of False Positives**: We may encounter false positives with the regex because it might accidentally match unrelated phrases that contain those keywords, such as "don't cut to that". This underlines the importance of using more contextual methods that can help us refine the detection of actual scene transition indicators.
5. **Automating Video Editing**: Recognizing scene changes can be a boon for automated video editing software, where precise detection of these transitions is critical for editing efficiency. If the detection is inconsistent, it can lead to wasted editing time and the potential for creating an undesirable narrative flow.
6. **More Than Just Cuts and Fades**: The regex only catches a subset of possible transitions (cuts and fades), neglecting other methods like dissolves, wipes, or crossfades. To expand this capability, you'd need to refine the regex to include a more comprehensive range of transition markers found in video content.
7. **Computational Load**: While regex is generally efficient, it can impose a processing burden when handling extensive video scripts or subtitles. For real-time applications, we might need to optimize regex operations or incorporate alternative data processing methods to maintain a good level of performance.
8. **Cross-Cultural Considerations**: The way scenes transitions are written in scripts can be influenced by cultural and regional filmmaking customs. A globally applicable approach would need to handle these variations, requiring a more flexible regex pattern that goes beyond the standard vocabulary.
9. **Merging with Timestamp Data**: Identifying scene changes becomes even more powerful when combined with timestamp data, which offers a deeper view of the flow of a video. This can shed light on video pacing, allowing creators to fine-tune their editing practices and ultimately improve how viewers interact with the content.
10. **How it Affects the Viewer**: The way scene changes are managed during video production and editing can significantly affect the pace of the narrative and how engaged viewers are. Improper or missing transitions can lead to confusion or lost interest, highlighting the importance of accurately detecting scene changes using techniques like regex.
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Capturing hashtags and mentions using #\w+ and @\w+
Hashtags and mentions, common in social media, can be efficiently extracted using the regular expression patterns `#\w+` and `@\w+`, respectively. Hashtags, denoted by the '#' symbol followed by alphanumeric characters, help categorize content. Mentions, indicated by '@' followed by alphanumeric characters, tag users or accounts. Python's `re` module provides the means to apply these regex patterns, using functions like `findall` to locate all occurrences within a string or `search` to find the first instance. These tools enable the isolation of relevant information, such as who is being mentioned or what topics are being discussed.
While these patterns are generally effective, handling complex situations can be tricky. Variations in hashtag usage (e.g., including punctuation) or non-standard characters in usernames might necessitate adjustments to the regex patterns. Furthermore, considering the broader context of the text is important for avoiding incorrect matches. For example, `@\w+` could pick up on a randomly placed sequence of characters that happens to begin with an `@` symbol rather than an actual username. In the broader field of video content analysis, using regex effectively to capture mentions and hashtags, alongside other essential information, helps reveal insights about the content and viewer interactions with it. As the complexity of social media and online communication continues to grow, mastering these techniques becomes increasingly important for anyone working with text data and extracting meaning from it.
1. **Hashtag and Mention Recognition**: The regular expression patterns `#\w+` for hashtags and `@\w+` for mentions are frequently used to identify these elements within social media text. These patterns are quite efficient at recognizing sequences of alphanumeric characters following the hash and at symbols, reflecting how simple patterns can effectively represent important aspects of online communication like topic discussion or user engagement.
2. **Enhancing Text Understanding**: Extracting hashtags and mentions from text adds valuable context to the overall analysis. This information can be essential for understanding sentiment, identifying popular topics, or analyzing trends within online discussions. Researchers can then gain a better understanding of how users engage with specific content and topics over time.
3. **Adapting to Evolving Social Media**: Social media is incredibly dynamic, and the way hashtags and mentions are used can change rapidly. Because of this, regex patterns used for this type of analysis have to be adaptable. This underscores the interesting relationship between language, technology, and the constant need for improvements in how we use regex for online data.
4. **Regex Limitations in Complex Cases**: While regex is very powerful, there are situations where its effectiveness can be limited. For example, hashtags can include non-alphanumeric characters (like emojis), and mentions might include punctuation. These nuances mean that we might need to have more complex validation techniques to make sure we're getting correct information from the data, highlighting the value of carefully designing regex patterns.
5. **Performance Challenges in Large Datasets**: The need for speed is crucial when applying regex for extracting hashtags and mentions in large datasets. Social media archives are a perfect example. The speed at which the regex can perform the pattern matching has to be taken into account along with all the other computational tasks happening. This is particularly important when you're trying to analyze data from streaming sources in real-time.
6. **Global Variations in Usage**: The use of hashtags and mentions can differ across different platforms and cultures. Some cultures might use underscores differently, or certain platforms might have specific conventions for these elements. This diversity means that researchers need to be aware of these variations when they are designing their regex patterns to make sure the data is gathered from a range of contexts.
7. **Mistaken Identities**: It's possible that the patterns `#\w+` and `@\w+` can mistakenly identify something as a hashtag or mention when it's not. For example, text that just looks like a hashtag or a mention could trigger a false positive. This reinforces the idea that when analyzing social media data, we must pay careful attention to the context of the text in addition to using regex for pattern recognition.
8. **Synergy with NLP**: Combining regex-based hashtag and mention detection with more sophisticated natural language processing (NLP) tools can lead to significant improvements in accuracy and the quality of data extraction. NLP techniques can give us more contextual information and a better way of distinguishing between similar things, creating a richer understanding of the information gleaned from the simpler regex match.
9. **Real-Time Monitoring**: In a wide range of contexts, including marketing or event analysis, regex for hashtag and mention recognition is used to monitor user interactions and identify trends in real-time. This demonstrates the value of obtaining this information quickly for decision-making processes.
10. **Understanding User Behavior**: By carefully examining the frequency and context of hashtags and mentions, researchers can discover valuable information about how people use these elements. This data can help inform better ways to create content and promote engagement, illustrating regex's vital role in interpreting online user interactions.
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Recognizing file extensions with \.(mp4|avi|mov|wmv)$
When analyzing video content, identifying the file format is often a necessary first step. The regular expression `\.(mp4|avi|mov|wmv)$` serves as a practical tool for recognizing common video file extensions like MP4, AVI, MOV, and WMV within filenames. Each extension corresponds to a specific video format, with each format having different uses. MP4, for example, has become popular for web streaming as it balances quality with smaller file sizes. While knowing the file extension is helpful in managing video data and selecting appropriate tools for tasks like playback or editing, dealing with diverse video formats can be challenging. Ensuring compatibility with various formats often necessitates further checks within the larger video analysis workflow. In the end, employing regex patterns like this one increases the overall efficiency and reliability of video data processing, especially in situations where filtering and categorizing video files is crucial. This ability to swiftly recognize file types allows developers to quickly identify suitable data for analysis, streamlining the entire process.
1. **The Limitations of Simple File Extension Matching:** The regular expression `\.(mp4|avi|mov|wmv)$` effectively catches a common set of video file extensions. However, the world of video formats is constantly evolving, with newer formats like AV1 and HEVC appearing. This regex, due to its simplicity, might miss these new file types, suggesting a potential need for more flexible solutions to address future developments in the realm of video technology.
2. **The Importance of Positional Matching:** The dollar sign (`$`) in the regex ensures that the matched extensions are at the very end of the filename. This is helpful in a lot of cases. But it introduces a limitation—if file extensions aren't strictly at the end (maybe they are in a more complex filename), the regex won't match. This makes it clear that the structure of the input data is important for this kind of regex-based matching to work correctly.
3. **Case Sensitivity Issues:** Regular expressions, by default, are case-sensitive. This means that if someone names a file `video.MP4`, this regex won't catch it. While you can add flags to make it case-insensitive, this involves learning more about regex behavior, which can be tricky for those not yet familiar with these patterns.
4. **Cultural and Regional Differences in Naming**: File naming habits can be very different across various cultures. A regex designed for English-language contexts might not perform as well when dealing with files named in a way that doesn't conform to those patterns. It's easy to imagine that this could lead to regex not matching relevant files if there are characters or structures not anticipated in the original pattern.
5. **The Implications for Automated Processes**: If we rely on regular expressions like this for video analysis automation, we implicitly assume consistent file naming conventions. But if filenames aren't well-formed, or if they don't adhere to a standardized structure, these automated processes might break down. This poses a potential challenge to efficiently processing huge libraries of video content.
6. **The Significance of Escaping:** The need to escape the dot character (`\.`) in regex patterns is a basic yet important concept in this field. It underscores the intricacies of pattern matching, as even small mistakes in how the characters are handled can throw off the intended output. It's an excellent example of how meticulous attention to detail is needed when designing these patterns.
7. **Performance in the Face of Scale:** The performance of the regex depends significantly on how the data is stored and indexed. For very large datasets, the process of scanning and searching through each entry could be slow if the dataset isn't optimized. This aspect is often ignored because regex is simple and easy to use, but it's a very real constraint when we start working with truly large-scale applications.
8. **Incorrectly Labeled Files**: It's not always certain that a file with a `.mp4` extension actually contains video data. Maybe someone has mislabeled the file, or maybe it's a non-video file that just happens to have that extension. The regex alone isn't enough to ensure that a matched file actually has the expected content. This emphasizes the need for some kind of validation step to make sure the regex matches are valid.
9. **Metadata's Influence on Video Data:** Modern video formats often include a large amount of metadata beyond just what the file extension tells us. This means the filename could be misleading in certain cases. It may be necessary to use other tools or techniques to extract more detailed information than just what the regex offers.
10. **The Need for Forward-Looking Regex Design:** As technology changes, video file formats evolve as well. This underscores that our regex patterns might need to be adjusted over time to remain effective. It's important for developers and engineers to design tools and patterns that can adapt to changes without requiring a complete rewrite, which is a crucial aspect of building robust software that's meant to last.
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Parsing video resolution patterns like \d+x\d+
When examining video content, being able to understand resolution patterns like `\d+x\d+` becomes crucial. This regex pattern isolates sequences of digits separated by an "x," which typically represents video resolution (width x height). It's specifically designed to deal with common ways we express video dimensions, often reflecting the resolutions of screens and displays. Python's `re` module provides the tools to apply this pattern, functions like `findall` or `search` being particularly helpful for finding and extracting resolution values. This empowers analysts to gain insights into the visual characteristics of video content, which can be important in various scenarios.
While effective for common situations, the simplicity of this regex can create problems if the data isn't consistent. There's a risk of errors if the data doesn't follow the expected pattern (e.g., non-standard format). It emphasizes the need to be thoughtful when using such patterns to ensure accuracy. However, if used correctly, mastery of this regex pattern within a larger video analysis context significantly improves a developer's ability to understand video properties, especially when categorizing, organizing, or evaluating content. It's a powerful tool for working with the core visual information inherent to the content itself.
### Surprising Facts About Parsing Video Resolution Patterns with Regex
1. The `\d+x\d+` regex pattern is primarily designed for standard video resolutions like 1920x1080 (Full HD) or 1280x720 (HD). But the reality of video resolution is far more diverse, encompassing 3840x2160 (4K) and even 7680x4320 (8K), a testament to the ongoing pursuit of ever-higher video fidelity.
2. Video resolution directly affects the quality of a streaming experience. For example, the difference between 720p and 1080p impacts the amount of data needed for streaming, potentially affecting viewers with slower internet connections. It's interesting how such a simple aspect of a video file has such a tangible impact on how people consume it.
3. The `\d+x\d+` pattern is flexible enough to accommodate non-standard resolutions which have become more common, particularly in mobile content. For instance, 1080x1080 square resolutions are often used for vertical videos, hinting at the adaptability required of regex patterns to keep up with video's ever-evolving landscape.
4. While regex is incredibly useful for finding patterns, it's not a perfect solution on its own. For example, this pattern might match non-video related dimensions (like a photo's resolution) in a data set. To ensure accuracy, it's crucial to validate the regex matches, highlighting a common need to bridge the gap between automated tools and intelligent validation.
5. The origins of video resolution can be traced back to the dawn of television, where resolutions were considerably lower than what we see today. Back then, 480i (standard definition) was the norm. The journey to HD and 4K has been quite a remarkable evolution in the technology we rely on for visual entertainment.
6. Even for established resolution standards, inconsistencies arise due to varying aspect ratios. For example, 16:9 for widescreen and 4:3 for older TVs, are simply different approaches to how video is presented on screens. Consequently, consistent usage of patterns like `\d+x\d+` is a challenge when managing video libraries that include a variety of formats, underscoring the complexity of managing digital video assets.
7. The growing consumer preference for higher resolution video has shifted production practices. Content creators now prioritize high-quality resolution, often aiming for 4K or even 8K, recognizing that viewers have increasing expectations about visual fidelity in a way that the early days of video simply didn't face.
8. Algorithms that leverage `\d+x\d+` can automate video quality detection during file conversion or uploads. This automated capability allows platforms to adapt content delivery based on the viewer's internet speed, which is a critical aspect of creating a consistent viewing experience regardless of location or technology.
9. Tagging video files with their resolution, using regex, enhances database management and retrieval efficiency. This type of automation becomes invaluable in large media libraries that require rapid access to content based on resolution, especially if the library needs to quickly provide different versions of content based on the devices used to view the videos.
10. Resolution can have surprising legal implications. Higher-resolution content often holds more value for licensing purposes. Thus, accurately capturing and categorizing this information via regex can impact agreements between content creators and distributors, emphasizing how this seemingly technical detail can have real-world legal impact in the realm of media rights.
Python Regex Mastery 7 Essential Patterns for Video Content Analysis - Extracting YouTube video IDs with (?<=v=)[a-zA-Z0-9-]+(?=&)
The regular expression `(?<=v=)[a-zA-Z0-9-]+(?=&)` is a powerful tool for extracting YouTube video IDs from URLs. It's designed to find the string of alphanumeric characters and hyphens that comes right after the "v=" parameter, which is how YouTube typically identifies a specific video. This pattern isolates the core identifier for YouTube videos, making sure that the extracted ID follows the correct alphanumeric format. Since YouTube video IDs are generally 11 characters long, this regex approach is also helpful in validating the IDs we're extracting.
While generally quite effective, it's important to consider that YouTube URLs come in many different forms, and users might input URLs in various ways that don't follow the standard format. This means that there can be scenarios where the extraction process becomes more complicated. By using regex for extracting video IDs, we not only simplify the process, but we also create a base for doing more advanced analysis or automating different video workflows. This can be useful for video analytics and other applications that require accurate video ID information.
### Surprising Facts About Extracting YouTube Video IDs with `(?<=v=)[a-zA-Z0-9-]+(?=&)`
1. YouTube URLs aren't always straightforward. While the `?v=` followed by the ID is common, shortened URLs like `youtu.be` are also used. The `(?<=v=)[a-zA-Z0-9-]+(?=&)` regex specifically targets the `?v=` format, making us realize that different URL structures need different regex approaches.
2. YouTube video IDs aren't random. They are typically 11 or 12 characters long and can contain upper and lowercase letters, numbers, and hyphens. This diverse character set is important because we need to build regexes that precisely grab the IDs without grabbing something else by accident.
3. Often, URLs have lots of extra information. The regex extracts the video ID but can also manage other parameters that might be there. This shows how careful we have to be with regexes: we want to be very precise about what we extract from potentially messy URLs.
4. The `[a-zA-Z]` part of the regex shows us that it handles both upper and lowercase letters. URL encoding can change case, so this is a nice detail that shows how precise regexes need to be.
5. While the regex is a good start, it might not work if a YouTube URL is odd or not properly formed. This tells us we might need to add extra validation or error handling. It's a reminder that a simple regex can't solve everything.
6. How regexes are used depends on the language or environment. Python's `re` module is optimized for this sort of text matching. But other languages might not be as good, and how they implement regexes might influence how efficiently we can extract IDs.
7. Getting the video ID is often the first step of a much bigger process, like understanding who watches the video or looking for trends in video content. This shows how correctly extracting IDs can be really important for analysis.
8. For large video datasets or platforms that analyze videos, extracting IDs using regex can slow things down if it's not done efficiently. Understanding how regex operations impact performance is crucial for building quick and effective video analysis workflows.
9. Sometimes, URLs might have multiple video IDs (think playlists). The `(?<=v=)[a-zA-Z0-9-]+(?=&)` regex on its own won't be enough if we want to grab multiple IDs. We need to combine it with other logic to make sure we get all the IDs correctly from more complex cases.
10. YouTube will likely evolve and change its URLs in the future. To keep the regex useful, we need to make it flexible enough to adapt to those changes. It's a good reminder that the design of the regex has to be future-proofed if we want it to work reliably over time.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: