Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications
Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications - Memory Footprint Analysis Comparing Raw Pointers vs shared_ptr in Frame Processing
When dealing with frame processing, understanding how raw pointers and `std::shared_ptr` impact memory usage is vital. `std::shared_ptr`, though beneficial for managing memory automatically and preventing leaks, carries a memory overhead due to its internal reference counting. This can impact performance, especially in time-sensitive video applications, as the added complexity of tracking shared ownership can slow things down. In scenarios where memory ownership is simple and clearly defined, using raw pointers may be a better option for performance optimization. However, if ownership semantics are more complex or there's a higher risk of dangling pointers, `std::shared_ptr` remains the safer choice, despite the added overhead. Ultimately, choosing the correct approach necessitates a careful consideration of the specific memory management needs within the frame processing pipeline.
In our exploration of memory usage within frame processing, we've encountered a notable difference between using raw pointers and `std::shared_ptr`. The latter's reference counting mechanism necessitates extra storage for tracking the number of pointers sharing ownership of a resource, potentially bloating memory usage, especially in situations where memory is a limited resource.
However, in multi-threaded contexts, `std::shared_ptr` proves invaluable by automatically managing the memory lifecycle, effectively shielding against dangling pointers, a significant safety benefit compared to raw pointers where careful management is required.
The act of object creation using `shared_ptr` can introduce a performance cost. This stems from the need to initialize the reference count at allocation time, potentially involving dynamic memory allocation for the control block.
This performance penalty becomes more pronounced in frame processing applications, where real-time performance is paramount. The latency introduced by reference counting, while possibly small, can impact the processing time for each frame, potentially resulting in missed deadlines for video processing in real-time scenarios.
It's intriguing to note that `std::weak_ptr`, which serves as a companion to `std::shared_ptr`, comes with a smaller memory footprint. This is because it does not increment the reference count itself. Instead, it offers a way to access the managed object without affecting the shared ownership, providing a nuanced approach to memory management in specific use cases.
Raw pointers, by their very nature, are more economical with memory. They are particularly suited for tightly-packed loops involving frequent allocation and deallocation, where the simplicity of their usage avoids the overhead of reference counting.
The actual amount of extra memory consumed by `shared_ptr` can be significantly influenced by the particular standard library implementation. Some libraries introduce extra overhead to ensure thread safety, which simpler implementations may not provide.
Situations where pointers are copied frequently can become performance bottlenecks when using `shared_ptr`. In these instances, the increased frequency of reference count updates requires synchronization across threads, which can become a bottleneck under heavy load.
Fortunately, profiling tools can provide valuable insights into the runtime overhead associated with both raw and smart pointers. By examining the impact of different memory management approaches on performance metrics like frame rates and latency, we can optimize the approach to memory management within the specific contexts of our applications.
While `shared_ptr` offers compelling benefits in various application domains, we must acknowledge the memory implications associated with its usage. In our video processing applications, it's critical to carefully consider whether the added memory usage aligns with the constraints imposed by the hardware and desired performance of the system. It's a balancing act between robust resource management and performance considerations.
Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications - Impact of Atomic Reference Counting on Video Decoder Performance
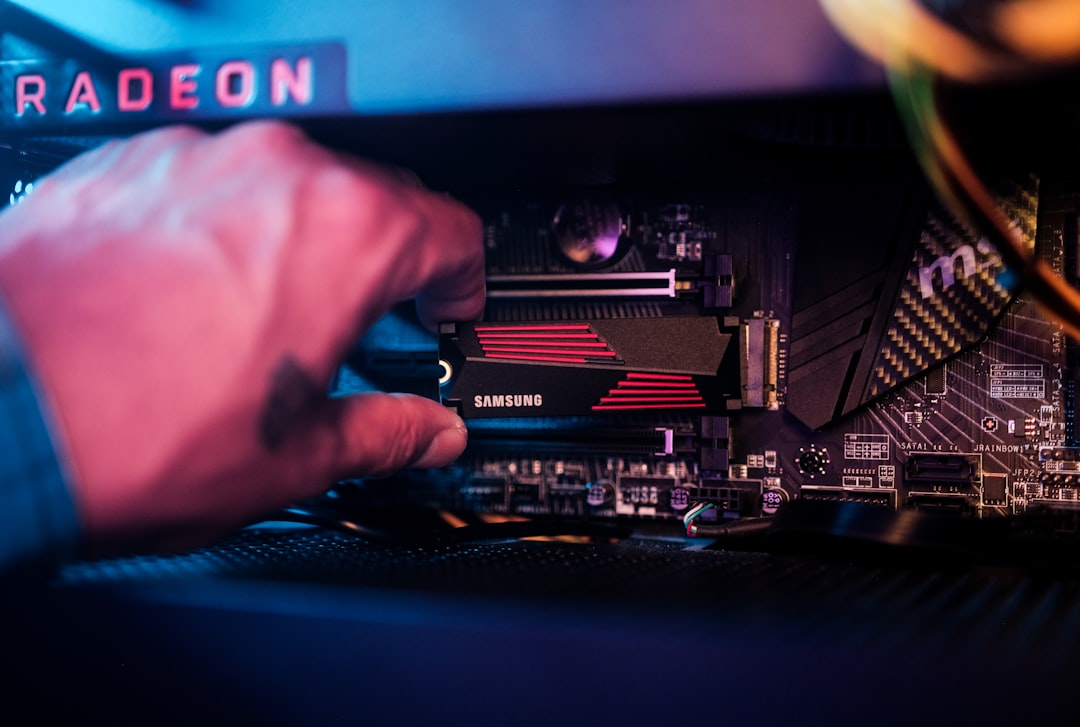
The use of Atomic Reference Counting (ARC) within video decoders can have a significant impact on performance. ARC automates memory management, tracking object lifetimes to ensure resources are released when no longer needed. This automated approach offers advantages in multi-threaded scenarios, preventing race conditions and resource leaks that can plague traditional reference counting methods. Moreover, the inherent thread safety of ARC contributes to a more stable and predictable memory environment within video decoders.
However, this enhanced safety and stability comes with a cost. The frequent updates to the reference count, necessary for accurate tracking, can introduce overhead that can impact the speed of video decoding. In real-time video applications, where performance is paramount, even small delays caused by ARC's operations could lead to frame drops or other undesirable outcomes. It's a balancing act: ARC promotes safety and reduces the likelihood of errors, but it can potentially impede the speed of the decoder.
Developers need to carefully consider the performance implications of ARC in their video decoding implementations. The benefits of automated memory management must be weighed against the potential performance bottlenecks that can arise from the added overhead of reference counting. Choosing the most appropriate memory management strategy ultimately depends on the specific needs and performance goals of the video processing application. There's no one-size-fits-all solution; the decision depends on balancing the need for safety against the need for speed in the context of a particular video processing task.
Atomic reference counting, relying on atomic operations, can potentially improve performance in multi-threaded video decoding by minimizing synchronization bottlenecks. However, the impact isn't always straightforward. Cache coherence, for instance, can introduce delays when multiple threads modify the reference count, impacting overall speed.
In contrast to traditional reference counting which might halt threads during count updates, atomic approaches allow for non-blocking updates, potentially increasing throughput, especially where low latency is crucial for video decoders. The overhead of this mechanism though, can be unpredictable. It varies across different processors and compilers, making profiling a necessity for understanding its impact on a specific video decoder implementation.
Compiler optimizations, another factor, can change how efficiently atomic reference counting gets executed, influencing a decoder's effectiveness. Along the same lines, increased CPU activity due to atomic operations can lead to higher power consumption, a critical consideration when dealing with battery-powered video processing hardware.
The story becomes even more complex in high-resolution video applications. What might appear to be minor latency from atomic updates can add up, noticeably slowing decoding during real-time playback. Further, if atomic reference counts are placed in frequently accessed structures, we might run into false sharing, where cache line invalidation degrades performance.
Even with the control block used by `std::shared_ptr` for resource management, it might lead to fragmentation in memory, especially for short-lived video frames. In these cases, simpler memory management approaches could be more effective.
In light of these potential drawbacks, it's valuable to remember that atomic reference counting is just one approach to memory management. We might discover that techniques like thread-local storage or specialized memory pools perform better in certain video decoding contexts, achieving a better balance between performance and resource use. Researchers and engineers can potentially fine-tune performance through careful exploration of these alternatives.
Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications - Thread Safety Costs During Multi-Stream Video Operations
When dealing with multiple video streams concurrently, ensuring thread safety becomes a crucial aspect that can significantly affect performance. Certain data structures, like `MemoryStream`, might not be inherently thread-safe, necessitating careful control to prevent corruption from simultaneous access by multiple threads. This underscores the importance of understanding which elements within a video processing pipeline are thread-safe and managing access accordingly.
While thread safety prevents race conditions and data corruption, the mechanisms employed to achieve it, including locks and atomic operations, can introduce overhead. These mechanisms can lead to performance bottlenecks through increased latency, or in more severe cases, deadlocks. The added complexity of managing concurrent access to shared resources can impact the fluidity of video processing, especially when dealing with real-time requirements.
For optimal performance in video processing applications, understanding and managing the tradeoffs associated with thread safety is paramount. Careful consideration of how threads interact with shared resources, implementing strategies to minimize contention, and choosing the right synchronization primitives are crucial to optimize the video processing pipeline for speed and efficiency. Striking a balance between ensuring data integrity and avoiding performance penalties is a key challenge in any multi-stream video application.
In multi-stream video processing, the performance impact of thread safety measures, particularly those associated with atomic reference counting used in `std::shared_ptr`, is a major area of concern. We're finding that the frequent updates to reference counts, necessary for maintaining the integrity of shared resources, can add considerable latency, potentially slowing down frame delivery rates. This is because these updates, although seemingly simple, contribute to a higher rate of cache misses as multiple threads compete to access the shared counters.
The increased competition for access to the reference count in high-thread-count scenarios can create bottlenecks during peak processing. This is particularly true when many threads are simultaneously attempting to increment or decrement the count, which can lead to delays that are noticeable, especially in demanding video processing. Memory allocation decisions for atomic reference counting present a tricky tradeoff. While the atomic operations offered by such mechanisms undeniably lead to safer memory management, they require additional memory for control blocks, which can complicate memory usage in resource-intensive applications.
The performance of thread-safe reference counting is also quite sensitive to the underlying CPU architecture. Some CPU designs inherently handle atomic operations more efficiently than others, leading to variable performance characteristics when using `std::shared_ptr` in a multithreaded video application. Furthermore, the temporal locality of reference counts in the video processing pipeline is critical. If updates to these counts consistently miss the cache due to frequent updates, access times slow down, affecting overall latency and potentially impacting frame rates.
False sharing, where multiple threads modify reference counts that share the same cache line, can lead to performance issues due to the constant cache line invalidations. This can significantly impede processing performance, especially in high-throughput video applications. Accurate optimization in such scenarios demands thorough profiling, as the effects of atomic reference counting can vary widely depending on the specific implementation details, including the threading patterns and nature of the processed video data.
Although atomic reference counting minimizes some synchronization needs, it doesn't completely eliminate them. Therefore, careful attention needs to be paid to how thread scheduling can affect performance, because unpredictable delays can derail the smooth operation of real-time video pipelines. And in the complexity of managing threads and shared resources through `std::shared_ptr`, we also must recognize the increased risk of circular dependencies, which can lead to deadlocks that grind the video processing to a halt. Given the potential for deadlocks and the inherent performance challenges related to thread safety measures in these applications, researchers must thoroughly explore the tradeoffs between safety, speed, and efficiency in the ever-evolving landscape of video processing.
Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications - Memory Deallocation Patterns Under Heavy Video Processing Load
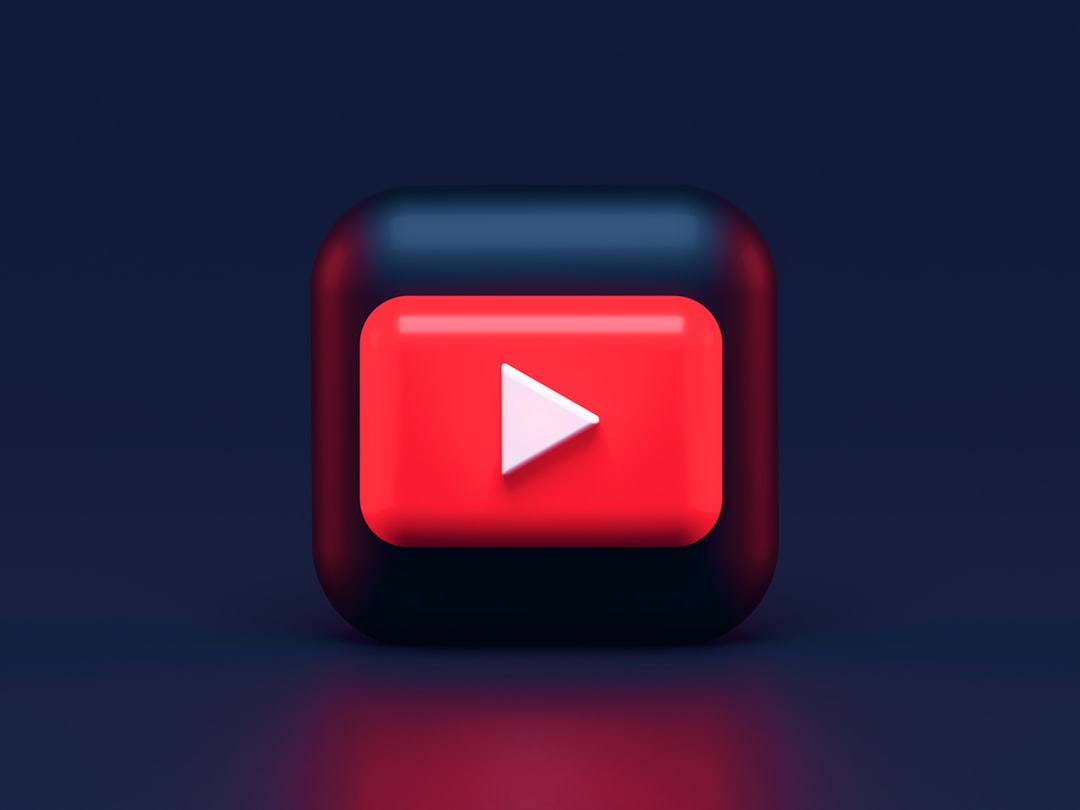
When video processing demands are high, the way memory is deallocated becomes crucial for performance. The allocation and deallocation of memory, especially when using smart pointers like `std::shared_ptr`, introduces overhead from reference counting. This overhead can lead to performance slowdowns, especially when dealing with frame rates and processing speeds. The situation gets worse in multi-threaded applications because of the increased frequency of updates to reference counts. Additionally, the need for thread safety while mitigating performance hits from synchronization creates a difficult balancing act between safe memory handling and speed. To design effective memory management solutions for video processing, a deep understanding of these complexities is essential for meeting the performance challenges inherent in such applications.
Video processing, especially under heavy loads, demands rapid memory deallocation to sustain a smooth flow of frames. How memory is released can significantly impact the speed and efficiency of the entire system. In high-pressure situations, poor deallocation techniques can lead to a scattered mess in memory, causing performance to deteriorate over time.
Under heavy video processing loads, the traditional ways of releasing memory, like using free lists, can struggle when multiple threads try to access them at the same time. This leads to delays. Utilizing custom object pools can ease this issue by minimizing the number of times memory is allocated and deallocated, potentially improving the responsiveness of the system.
The way memory gets released shows a distinct pattern depending on the intricacy of each frame and the processing happening. Frames with lots of detail, such as complex scenes, might need more frequent allocation and deallocation compared to simpler ones, illustrating the need for flexible memory management strategies.
Intriguingly, video decoders can optimize their performance by occasionally grouping together deallocation requests. This reduces the number of costly memory management operations that take place. This approach allows the system to reclaim memory in bigger chunks, resulting in better cache coherence and less overhead.
The design of the underlying hardware plays a critical role in how efficiently memory is deallocated. CPUs with multiple cores and caches benefit from approaches where several threads can safely release memory resources simultaneously without creating significant delays due to contention.
In applications where real-time video processing is essential, delays in memory deallocation can result in dropped frames. This compels researchers to carefully analyze and find the optimal balance between the overhead associated with memory management and maintaining optimal processing speeds to prevent performance degradation.
Using reference counting with automatic deallocation can be beneficial, as the system automatically releases memory when it's no longer needed. However, if reference counts aren't optimized properly, they can create bottlenecks that slow down frame processing rates because of frequent access and changes to the reference count.
A frequently overlooked aspect of memory management under heavy load is the pattern of how memory is released. When deallocation is concentrated, it can lead to significantly better cache performance, resulting in faster memory access times for subsequent allocations.
Using profiling tools to analyze memory allocation and deallocation can reveal unexpected memory usage patterns, providing opportunities to improve performance. These tools can pinpoint bottlenecks in memory management, guiding engineers to refine their strategies and increase application performance.
In applications that heavily rely on `std::shared_ptr`, keeping an eye on the reference count during heavy processing is essential. If you notice spikes in reference count updates and performance simultaneously, it might indicate that simplistic shared ownership models may not scale well under load without further adjustments.
Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications - Compiler Optimization Effects on Reference Count Management
Compiler optimizations can significantly impact how efficiently reference counting manages memory, especially in demanding applications like video processing. Compilers employ techniques like inlining and loop unrolling to reduce the overhead of updating reference counts, leading to potentially faster execution. However, this isn't always a straightforward win. Optimizations can sometimes create issues like heightened cache contention or false sharing, potentially negating the initial performance gains, especially in real-time environments where latency and throughput are crucial. This means that while compilers can improve reference count handling, developers need to carefully monitor and adjust their code using profiling tools to balance the benefits of optimization against potential drawbacks within the unique constraints of each video processing workflow. It's a delicate dance between leveraging compiler enhancements and ensuring that these optimizations don't introduce unexpected performance regressions.
Reference counting's performance can be heavily influenced by compiler optimization techniques. For instance, clever optimizations like function inlining and loop unrolling can reduce the overhead from updating reference counts. However, the degree of effectiveness varies significantly between different compilers, highlighting the need for thorough testing in specific environments.
While reference count updates might seem insignificant, they can actually contribute quite a bit to latency in video processing, especially when handling high frame rates. The consistent incrementing or decrementing of the count for each frame can accumulate and become a bottleneck.
When multiple threads are at work, the high contention for the reference count can lead to more cache misses. Each thread's attempts to adjust the count can inadvertently stall other threads, ultimately slowing down the entire process.
The memory usage of `std::shared_ptr` is impacted not only by the reference count itself but also by the control block that manages it. This control block can have a different size depending on the library, affecting how memory-efficient your video applications are.
False sharing can be a problem when using reference counting in multi-threaded scenarios. When updates to different reference counts end up sharing a cache line, this leads to needless data invalidations that hinder performance.
Using atomic operations for reference counting can increase the overall power consumption due to the higher CPU activity. This is a concern for devices that rely on batteries, like mobile video processors.
Understanding the performance impact of optimizations in reference counting needs a lot of context, and profiling tools are invaluable here. These tools can help uncover hidden bottlenecks and guide you toward using raw pointers or smart pointers in the most effective way for your specific application.
The timing of releasing memory during video processing tasks can differ significantly, particularly when complex frames require more time before resources can be freed. We need to build dynamic memory management approaches to keep up with these variable patterns.
The constant updates to the reference count can lead to memory fragmentation, especially when dealing with lots of short-lived objects in video applications. Using customized allocators might be beneficial in managing this fragmentation and enhancing memory access.
The choice between using atomic or non-atomic reference counts comes down to balancing safety and speed. Atomic reference counting generally provides a more stable environment, especially with high thread contention, at the expense of a bit more latency. In scenarios with low contention, non-atomic counting might offer a slight edge, but this can come at the cost of stability when there are many competing threads.
Benchmarking Memory Management A Deep Dive into stdshared_ptr's Reference Counting Performance in Video Processing Applications - Cache Line Implications of Control Block Memory Layout
Within video processing, the way memory is organized for `std::shared_ptr`'s control blocks directly impacts how effectively the CPU cache is used, influencing overall performance. Since CPU cache lines usually hold 64 bytes of data, arranging memory in a way that keeps related data close together can improve data access speed and reduce wasted cache lookups. If the memory is not laid out well, it can lead to a problem called false sharing. This occurs when different threads modify data that happens to be within the same cache line, creating needless slowdowns. Therefore, creating a memory layout for control blocks that minimizes fragmentation and ensures that related pieces of data are close together is crucial for optimizing the performance of reference counting, especially when dealing with video processing where speed is critical. Striking a balance between the added memory needed by `std::shared_ptr` and maintaining the speed needed for video processing requires carefully considering these factors.
The way we arrange control blocks in memory can significantly impact how efficiently the CPU's cache works, especially when dealing with things like `std::shared_ptr`'s reference counting in video processing. Cache lines, typically 64 bytes in size, are the units of data movement between main memory and the cache. If the related pieces of data like reference counts aren't laid out well within these cache lines, we can end up with a lot of cache misses. This can slow things down considerably during those frequent reference count updates that are common in video processing.
Ideally, we want data that's used together to be physically close to each other in memory. This helps maximize what's called spatial locality. If we can arrange our control blocks so the reference count and other related information are close together, we're more likely to get cache hits, speeding up reference count adjustments. This is really important for video processing where frame rates are crucial.
However, in multi-threaded settings, there's a potential problem called false sharing. This can really impact performance. If multiple threads are updating reference counts that happen to reside within the same cache line, we end up with a lot of cache line invalidations. This can slow down the whole process and impact throughput, especially in heavy video processing where high speed is a priority.
Another issue is memory fragmentation. Depending on how we lay out the control blocks, especially when working with lots of short-lived objects like video frames, we can create fragmented memory. This makes it more difficult for the system to find contiguous blocks of memory for future allocations, potentially leading to a slow-down over time.
Choosing between atomic and non-atomic reference counting introduces a trade-off. Atomic reference counting can improve thread safety, but the extra complexity can result in a performance hit due to the extra work involved in atomic operations. On the other hand, non-atomic reference counting is simpler, but we have to be much more careful about handling race conditions, which can lead to errors. The best choice depends on the specific needs of the application.
The way caches work and memory access patterns can differ across different types of CPUs. An arrangement of control blocks that works great on one type of CPU might not be as efficient on another. This means we might need to adjust our layout and test things out on each type of hardware to make sure we're getting the best results.
Compiler optimizations can sometimes have unexpected side effects on control block performance. Techniques like inlining, for example, can change how frequently reference counts are updated, potentially leading to performance issues if not carefully controlled.
The impact of delays caused by inefficient cache usage or synchronization within the control block management can be especially noticeable in real-time video processing. Even a small delay can cause us to drop frames, negatively impacting the user's experience.
Profiling can be very helpful to figure out where the bottlenecks are within the video processing workflow. We often find that reference count updates become a 'hot path' in these applications. If we can reduce the frequency of these updates, perhaps through some kind of batching technique or reducing how often resources are shared, we might see a significant performance improvement.
One interesting area of investigation could be the use of a custom memory allocator designed specifically for control blocks. These custom allocators might be able to reduce memory fragmentation and optimize memory allocation, potentially leading to gains in performance compared to using the standard memory allocation mechanisms. It’s worth exploring this as a potential performance optimization.
More Posts from whatsinmy.video: