Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20
Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20 - Setting Up TensorFlow 20 and Required Libraries for Image Recognition
To get started with image recognition using TensorFlow 2.0, you'll need to ensure your Python environment is correctly configured. Fortunately, TensorFlow 2.0 supports both Python 2 and 3, offering flexibility in your choice. Building CNNs with TensorFlow is simplified with the Keras Sequential API, and it's this approach we'll be focusing on. To do this, you'll need to import a few essential libraries: TensorFlow itself, NumPy for numerical operations, and Matplotlib for visualizations.
A popular dataset for practicing image recognition is CIFAR-10. It's a collection of 60,000 small color images across 10 different categories, making it an ideal starting point for experimenting with CNNs. A common suggestion is to approach building these networks iteratively, implementing one part at a time and testing its output. This 'learn by doing' approach strengthens comprehension and allows you to quickly pinpoint potential issues.
Beyond the basics, you can explore techniques like Transfer Learning. It involves leveraging pre-trained models, allowing you to build upon existing knowledge for more complex image recognition tasks without starting from scratch. This can significantly speed up development and improve results in certain scenarios.
TensorFlow 2.0, with its shift to eager execution, makes the development process more interactive and straightforward compared to prior versions, enabling you to observe operations as they occur. This can be quite helpful when trying to track down the source of errors. But, ensuring compatibility across libraries like NumPy, Pandas, and Matplotlib can be a hurdle. Version mismatches can lead to issues in the CNN's functionality, especially during the initial stages of model building.
Keras, now integrated into TensorFlow 2.0, offers a convenient interface for designing and training neural networks. However, the ease of use should not overshadow the value of understanding how to customize the learning process with callbacks and create your own layers for more advanced scenarios.
It's recommended to leverage virtual environments with `pip` when installing TensorFlow to minimize potential conflicts with existing Python libraries, which is a common issue in machine learning setups. When utilizing a GPU, the CUDA and cuDNN libraries are essential. However, compatibility across versions can be challenging; discrepancies can result in performance issues, or in the worst-case scenario, prevent the software from working correctly.
One advantage of TensorFlow 2.0 is its versatility: it can run on various platforms, from mobile devices to web applications. Therefore, after designing your CNN, you can readily deploy it in different environments without significant changes. However, understanding pre-processing techniques, like normalization and augmentation, is crucial for enhancing your CNN's accuracy and robustness, especially in avoiding the issue of overfitting.
TensorBoard, a built-in tool, provides a robust visual overview of your model's training progress and aids in the optimization of hyperparameters through its interactive environment. TensorFlow Hub simplifies integrating pre-trained models by offering readily available architectures trained on large datasets, which can significantly speed up development and save resources. This is particularly useful if you do not have a large amount of labelled data.
Lastly, leveraging `tf.function` can improve TensorFlow's performance while preserving the flexibility of dynamic execution. This hybrid approach is a useful debugging tool, helping to trace problems in complex models.
Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20 - Understanding CIFAR10 Dataset Structure and Image Preprocessing Steps
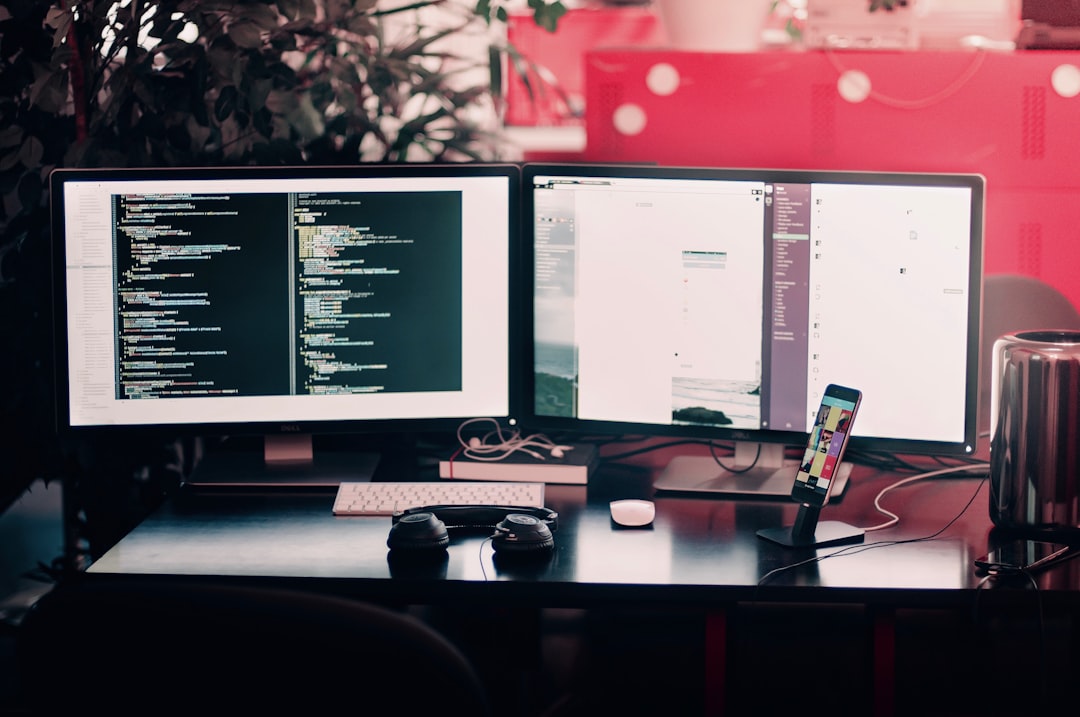
The CIFAR-10 dataset is a valuable resource for learning about and building CNNs for image recognition. It's composed of 60,000 32x32 pixel color images, categorized into 10 distinct classes. This structure is beneficial as it standardizes the input data for the model, streamlining the training process. Each image is represented with three color channels (red, green, blue), making it a straightforward dataset for initial experimentation.
However, simply feeding raw image data to a CNN is usually not optimal. Preprocessing is a crucial step. Techniques like normalization are commonly used to bring the pixel values within a specific range, which can often lead to faster and more stable model training. These steps ensure that the model doesn't prioritize one pixel intensity range over another and leads to better overall performance. While not as complex as some other image datasets, CIFAR-10's size and structure make it a great starting point for understanding CNN fundamentals. Furthermore, dealing with this simpler dataset helps prepare you for dealing with more complicated image data in the future, teaching valuable lessons that are applicable to larger, more difficult tasks. The insights you gain from working with CIFAR-10 will help you tackle more intricate challenges as you progress in your understanding of image recognition with CNNs.
The CIFAR-10 dataset is made up of 60,000 images, each a small 32x32 pixel color image, divided into 10 categories, like airplanes or dogs. It's a pretty useful tool for testing out various image recognition ideas, even though the dataset itself is relatively small.
One of the challenges with CIFAR-10 is that the images are quite low-resolution at 32x32 pixels. This means that getting meaningful features from them can be tricky for convolutional neural networks (CNNs), and it really stresses the importance of using good data augmentation and preprocessing methods.
Interestingly, it's a balanced dataset, with each of the 10 classes having 6,000 images. This means that we don't have to worry as much about issues with some classes having significantly more images than others. However, it's still important to make sure our models aren't biased towards any particular category during training.
Before using the data with our CNN, we'll need to normalize the images. This usually involves scaling the pixel values to a range between 0 and 1 or standardizing them using the mean and standard deviation. Normalizing helps with gradient stability and faster training.
It's important to use data augmentation techniques on CIFAR-10, given the small size and resolution of the images. We can do this by applying things like random cropping, flipping, or rotating the images. This essentially creates more training data, which can improve a model's ability to generalize to new, unseen images. It helps reduce overfitting, a common issue with small datasets.
If we train a CNN using CIFAR-10, we can further improve the process by using batch processing. This allows us to efficiently train using GPUs and often speeds up the convergence of the training process.
In some cases, we might get great results by using pre-trained models with the CIFAR-10 dataset. Transfer learning is a technique where we leverage models trained on a larger dataset. Features learned on bigger datasets can improve performance with CIFAR-10, which can be really beneficial, especially if we don't have a lot of time or resources.
Although CIFAR-10 is relatively simple, we still need to be aware of overfitting. Since the dataset is small, it's easy for our CNNs to become overly specific to the training data and not generalize well to new images. Dropout layers and regularization methods can help to tackle this.
To assess how well our CNNs are performing, we need to use evaluation metrics. Accuracy is one of the basic metrics we can use, and we also need to examine the confusion matrix. This can give us valuable information about which classes are being confused with each other, and it's often a good starting point for finding ways to improve our models.
Lastly, experimenting with different CNN architectures is crucial to achieving good performance on CIFAR-10. We can explore different combinations of convolutional layers, pooling layers, and the network depth. It is through such explorations that we uncover potential paths to more accurate and robust CNNs. Each tweak can yield significantly different results, emphasizing the need for experimentation and careful consideration of network design choices.
Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20 - Building Basic CNN Architecture with Convolutional and Pooling Layers
The core of a basic CNN architecture lies in the coordinated effort of convolutional and pooling layers, forming the foundation for feature extraction within image data. Convolutional layers function by applying filters to the input image, extracting key features defined by parameters like the filter size and the stride. These layers are followed by activation functions, such as the Rectified Linear Unit (ReLU), to add non-linearity to the learned representations. Pooling layers, particularly the common max pooling method, play a significant role in reducing the spatial dimensions of feature maps, a process that helps to prevent overfitting while preserving important feature information. This layered design, where convolutional and pooling layers are systematically stacked, allows the CNN to efficiently process and learn from image data. Grasping these fundamental elements is crucial for anyone aiming to create efficient image recognition models, especially when employing frameworks like TensorFlow or PyTorch. It's through this understanding of how these layers work together that we can truly begin to develop robust and useful image recognition tools. While convolutional layers are responsible for learning features, pooling layers offer a powerful way to manage the complexity of the features, reducing the risk of overfitting and leading to models that generalize better. There's an inherent trade-off between the reduction in spatial dimension and the information retained by pooling layers, but this process of careful dimensionality reduction is what empowers CNNs to achieve high accuracy on image recognition tasks.
The foundation of a Convolutional Neural Network (CNN) rests on a few core building blocks: convolutional layers, pooling layers, and, eventually, fully connected layers. Convolutional layers work by applying multiple filters across an image. Each filter learns to recognize specific patterns, such as edges or textures. It's interesting how this mirrors the way biological neural networks in our brains process visual information.
Pooling layers typically follow convolutional layers, and their main function is to reduce the dimensions of the extracted features. This lowers the model's complexity and improves computational efficiency. By focusing on the most crucial aspects of the image, it acts as a feature extraction method and discards less important data.
CNNs offer an advantage in the number of parameters needed compared to fully connected networks with a similar number of layers. This stems from the sharing of weights within convolutional layers. Fewer parameters means faster training times and a lower likelihood of overfitting, a significant problem when training AI models.
Within convolutional layers, there are a couple of important concepts to consider: stride and dilation. The stride dictates how many pixels a filter moves at each step during the operation. Dilation allows the filter to cover a wider area without requiring more parameters. Adjusting these aspects allows you to control how a CNN recognizes image features.
The design of a CNN is layered, with each layer building upon the features identified in the previous layer. Early layers might learn basic elements like edges, while later layers combine them to detect more complex shapes. It's a process that creates hierarchical feature extraction.
When convolutional layers are applied, it's usually followed by an activation function. These play an important role in defining how the CNN learns. ReLU (Rectified Linear Unit), a common choice, can speed up learning, but it can sometimes lead to problems where neurons become inactive during training—known as the "dying ReLU" issue.
Another valuable component of a CNN is the use of batch normalization layers. These help make training more stable and fast. They work by normalizing the input to each layer, preventing internal covariate shift, a condition that can impede learning in deeper networks.
To avoid overfitting—where a model performs well on training data but poorly on new, unseen data—CNNs often include dropout. This technique randomly removes some neurons from the network during training. By forcing the model to rely on redundant representations, the CNN's performance becomes more stable and generalizes well across datasets.
Compared to traditional image processing methods, CNNs have proven more powerful for image recognition. They excel because they can automatically learn hierarchies of features from the data itself. This eliminates the need for extensive manual feature engineering, which can sometimes overlook complex patterns, especially in high-dimensional data like images.
However, despite their advantages, CNNs can often be like a "black box." It's not always easy to tell how they're making a prediction. Luckily, new techniques like Grad-CAM (Gradient-weighted Class Activation Mapping) can give us a peek into which parts of the image have the biggest impact on the final output. These efforts move us towards more interpretable forms of artificial intelligence, a significant need as AI systems become increasingly prevalent.
Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20 - Adding Dense Layers and Configuring Model Parameters for Pattern Detection
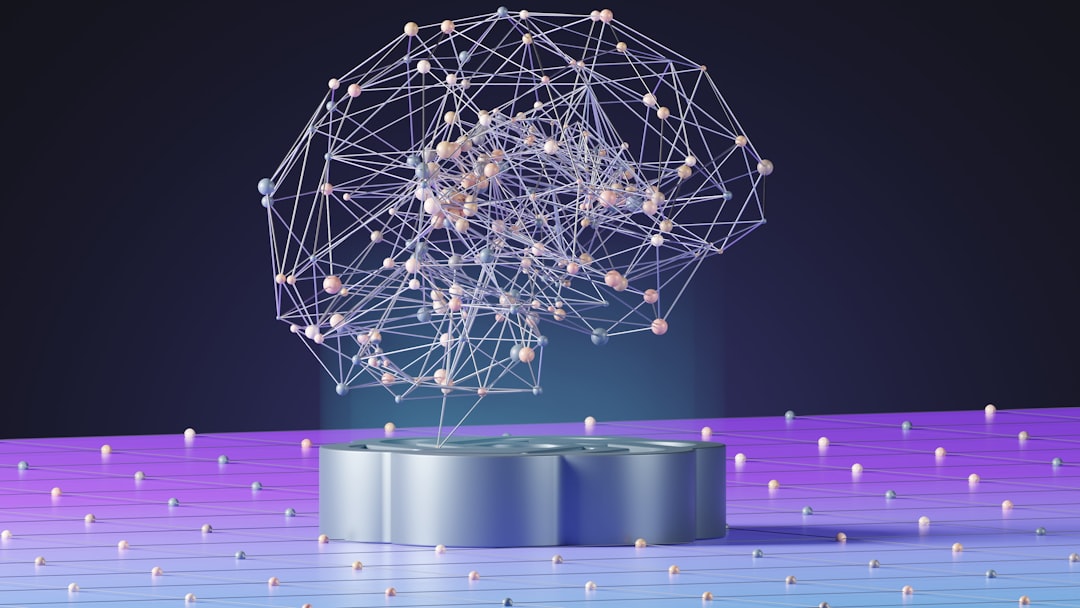
After building the foundation of our CNN with convolutional and pooling layers, we now introduce a critical component: dense layers. These layers serve as the final stage of our network, where the features extracted by the convolutional and pooling layers are combined to make a decision. You can think of them as a way to link all the learned features together for a final prediction.
Dense layers are fully connected, meaning every neuron in one layer is connected to every neuron in the next layer. This allows the network to learn complex relationships between the extracted features. In our image classification task, these connections are fundamental for enabling the CNN to classify images into the different categories (e.g., airplane, automobile, bird, etc.) in CIFAR-10.
However, getting the best performance from these dense layers isn't just about adding them in. It requires careful configuration of several parameters. This includes the number of neurons in each dense layer, as well as broader choices about the overall architecture of the CNN. The size of batches we use during training, the number of training cycles (epochs), and even strategies like dropout can all impact the final result.
One important detail is to flatten the output from the convolutional layers before feeding it into the dense layers. This essentially reshapes the multi-dimensional output of the convolutional layers into a single, one-dimensional vector. This is needed because dense layers only work with 1D arrays, preparing the data for the final classification task.
If we don't pay careful attention, CNNs can sometimes suffer from overfitting, where they perform very well on the training data but struggle with new images. Overfitting happens when a model learns the training data too well, including the noise or quirks that aren't general features of the broader class of images. To address this, techniques like dropout can help improve a model's ability to generalize. Dropout randomly deactivates some neurons during training, forcing the network to distribute its learning across multiple neurons, leading to more robust and less overfit models.
By carefully adding and configuring dense layers, adjusting network architecture, and considering techniques like dropout, we can create a CNN that effectively recognizes complex patterns in image data. This process will be further refined as we move into the next section of training and evaluation, allowing us to build a highly functional image recognition tool.
Okay, let's rephrase that section on adding dense layers and configuring model parameters in a way that's suitable for a curious researcher or engineer in late 2024, keeping the original style and length in mind, and avoiding repetition from the earlier parts of the article.
Dense layers in a CNN are where the real magic of feature interpretation happens. After convolutional layers extract features from images, these fully connected layers take over, weaving together these features into a coherent understanding. The model essentially learns to associate these extracted features with specific patterns, transforming hierarchical spatial data into a form that's ready for the final classification decision. It's at this stage that the CNN begins to make sense of what it has learned in the earlier layers.
However, introducing dense layers comes with a cost—the number of parameters can increase very rapidly. It's like expanding a network rapidly, adding more interconnectedness between neurons. This increase in parameters is a double-edged sword. Each one allows for learning, but at the same time, also increases the chances of our model becoming too complex and potentially overfitting to the training data. It's a delicate balance, and careful planning is needed.
One way to tackle this potential overfitting is by adding dropout to dense layers. This is a regularization technique that randomly disables some neurons during training. Think of it as introducing a bit of controlled chaos to the training process. It forces the network to develop more robust and less interconnected learning pathways. The benefit is that this leads to a model that is less susceptible to overfitting and able to better handle unseen data.
The activation function within these layers has a huge impact on the model. We have options like ReLU, which can make training quite fast but also has a known issue – the 'dying ReLU' problem. If the weights get too large or too small, neurons can become unresponsive and effectively drop out of the network. This can affect model performance and requires careful monitoring.
Using batch normalization can be helpful within dense layers. It works by normalizing the inputs of each layer. This reduces 'internal covariate shift'—a fancy way of saying that the distribution of input data to each layer changes over time. Batch normalization helps prevent these changes, ultimately contributing to a more stable learning process. It also helps in controlling gradient issues that can arise in deep networks, preventing the gradients from either exploding or vanishing, thus assisting in a smoother convergence during training.
When building your network, it's wise to add dense layers incrementally. This allows you to observe the effect of each layer on accuracy. It's a practical approach, especially in debugging and fine-tuning the model. You can analyze exactly how each layer contributes to your classification accuracy and pinpoint potential trouble spots in the model. It's a step-by-step method of experimentation and discovery.
Fully connected layers, because of the high number of connections, can be prone to overfitting, even more so than convolutional layers. Overfitting can cause the model to perform very well on training data but fail on new, never-before-seen images. Regularization techniques like L1 and L2 can be used to prevent this. These techniques essentially add a penalty to the loss function for having larger weights, which in turn helps create a more generalized and robust network.
Another strategy we can use to tackle overfitting in dense layers is called global average pooling. Instead of fully connected layers, we can average the feature maps for each class. It's a more direct and simpler approach and it helps reduce the model complexity by eliminating a lot of the parameters, reducing the potential for overfitting.
When you choose a loss function, you're deciding how the model learns to associate the outputs with the features. For multi-class classification, like CIFAR-10, the categorical cross-entropy loss function is a popular choice. It's very effective in measuring the difference between the predicted probabilities and the true class label. It pushes the network to make sharp and clear predictions for each class.
Ultimately, it's important to experiment with the size and number of neurons in your dense layers. This is another area where trial-and-error often yields the best results. You might find that a smaller layer with fewer connections can prevent overfitting in certain cases. But, for other tasks, larger layers might be needed to effectively capture the subtle intricacies of the data. The best approach is to explore and analyze which configuration offers the highest accuracy for the specific image recognition problem you're tackling. It's a dance between model complexity and desired performance.
I hope this is more in line with what you're looking for. Let me know if you'd like further revisions or if there's anything else you need!
Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20 - Training Your Model Using Google Colab GPU Resources
Leveraging Google Colab's GPU resources presents significant benefits for training CNNs, especially for image pattern recognition. Colab offers free access to powerful GPUs, dramatically accelerating the computationally intensive training process compared to using CPUs alone. This makes it a practical choice for experimenting with complex CNN architectures.
The Keras Sequential API, readily available within Colab, significantly simplifies building and modifying CNNs. You can create and train sophisticated models with just a few lines of code, which is particularly helpful for those just starting out. Moreover, Google Colab provides a complete development environment without the need for intricate local setup, making it incredibly accessible.
Further streamlining the process is TensorFlow 2.0's emphasis on eager execution. Eager execution allows you to see the results of your code immediately, which can be invaluable for debugging and understanding how a model is learning. This feature makes the learning process much more interactive and facilitates a deeper understanding of CNNs during training. Overall, Google Colab offers a robust, flexible, and accessible platform for CNN training, particularly valuable for exploring and implementing image pattern recognition solutions.
Google Colaboratory, or Colab, offers a compelling platform for training convolutional neural networks (CNNs) by providing free access to GPU resources. This eliminates the need for expensive hardware, making deep learning more accessible to a wider audience, especially researchers and hobbyists. However, the free access comes with the caveat of shared resources, potentially leading to temporary performance bottlenecks during peak usage times. While useful for initial experiments and learning, relying solely on Colab for lengthy or computationally intensive training might not be ideal.
Setting up TensorFlow and leveraging GPU acceleration in Colab is surprisingly smooth. The environment automatically handles the intricate configurations of CUDA and cuDNN, a process that can be quite tedious and error-prone on local machines. This simplicity enables researchers and engineers to focus on designing and experimenting with their models, rather than grappling with environment-specific complexities.
The integration of Google Drive into Colab is another highlight, facilitating easy access to datasets and streamlined model storage and management. No more convoluted data transfer processes or struggling to find where you saved the latest version of your model. It's a feature that greatly improves the overall workflow.
However, this seamless integration also comes at the cost of reduced customization options. While Colab offers a user-friendly environment, the control over the underlying hardware and software components is limited. Those looking for fine-grained control over specific GPU models or other components might find the rigid environment a constraint. For instance, it's not always clear what type of GPU you will get in each session.
Another important factor to consider is the nature of Colab sessions. They have a tendency to expire after 12 hours of inactivity or continuous usage, presenting a risk of losing valuable training progress. Users need to adopt strategies like frequent checkpointing to save their models at intervals to avoid this potential interruption. This can be a little frustrating when dealing with exceptionally long training runs.
Colab also offers useful features for examining the training process. Interactive visualization tools integrated into notebooks, like Matplotlib and TensorBoard, help monitor training progress in a seamless manner. Analyzing performance using these tools is straightforward and can significantly aid in debugging and model refinement.
For those with more demanding projects, Colab Pro and Pro+ options are available. These paid tiers provide faster GPUs, along with extended runtime limits, offering a middle ground between the free tier and fully dedicated hardware. However, these options are not intended to be a replacement for dedicated servers, and still can be limited compared to robust local setups.
Interestingly, Colab provides built-in tracking of execution times and GPU utilization. This provides valuable insights for code optimization. Identifying bottlenecks and fine-tuning code for improved performance becomes more streamlined with access to these metrics.
Furthermore, the vibrant Colab community provides a vast collection of shared notebooks and resources, proving incredibly helpful for learning and getting started with projects. The wealth of public projects can be a boon to beginners and experienced engineers alike, offering starting points and templates for various tasks. This collaborative aspect greatly reduces the hurdles in embarking on complex projects.
In essence, while Colab's free GPU access offers a remarkable opportunity for exploring the world of deep learning, it's vital to acknowledge its inherent limitations. Shared resources, session expiration, and restricted customization are considerations that need to be taken into account when deciding if Colab is the best fit for specific machine learning projects. While not a replacement for robust local systems, it's undoubtedly a fantastic starting point for educational purposes and quick explorations into CNNs and other deep learning techniques.
Building Your First CNN for Image Pattern Recognition in Python A Step-by-Step Tutorial with TensorFlow 20 - Testing and Evaluating CNN Performance with Custom Images
After training your CNN, it's time to see how well it performs with images it hasn't encountered before. This section focuses on testing your CNN using custom image datasets. We'll examine key metrics like accuracy and loss to gauge how well the model generalizes to new data, which is crucial for real-world application. Understanding these metrics provides valuable insights into the model's strengths and weaknesses.
For a more detailed look at where the model might be struggling, we can use a confusion matrix. This helps pinpoint specific areas where the model tends to misclassify images, such as confusing a cat with a dog. These evaluations are critical to refining and improving your model. Based on the results, you can adjust your CNN's architecture, hyperparameters, or even try out different training strategies to improve its accuracy and robustness. This iterative process of testing, evaluating, and refining is fundamental for creating a CNN that performs well on a diverse range of custom images.
When evaluating a CNN's performance using custom images, we encounter a unique set of challenges and opportunities compared to established benchmark datasets like CIFAR-10. The inherent variability in custom image data, like differing lighting conditions, object orientations, and diverse backgrounds, can significantly influence how well our models perform. This highlights the crucial need for training datasets that capture the full range of expected variations in real-world scenarios.
One effective approach to address this variability and potentially enhance performance is to leverage transfer learning. By starting with a model pre-trained on a massive dataset—often encompassing a wide variety of image types—we can capitalize on its learned feature extraction capabilities. This pre-existing knowledge often provides a strong foundation for recognizing patterns within our custom images, even if those images are quite different from the original training data. We might see a significant improvement in accuracy simply by adapting a model previously trained on a large, diverse image collection.
Furthermore, the limitations of a potentially smaller custom dataset can be mitigated through data augmentation. By artificially expanding the dataset with techniques like random cropping, flipping, or applying color adjustments, we introduce a broader range of training examples. This can significantly reduce the risk of overfitting, a common concern when training with limited data. It essentially teaches the model to be more flexible and recognize the essence of a particular object regardless of minor variations in the images.
While accuracy is often the primary performance metric, it's important to consider others when evaluating models trained with custom images. In scenarios where classes are not evenly represented—a common occurrence in custom datasets—accuracy alone can be deceptive. Metrics like precision, recall, and the F1-score provide a more nuanced view of model performance across various classes. These metrics give a more holistic picture of the model's abilities, revealing its strengths and weaknesses in handling diverse image types.
Analyzing a confusion matrix after testing with custom images can offer deep insights into where our model is struggling. It helps identify which classes are often misclassified, which is incredibly useful in pinpointing specific areas for improvement. By understanding these patterns of error, we can tailor the model's architecture or further refine the training dataset to address these weaknesses.
The resolution of our custom images is another important factor influencing CNN performance. Images with overly low resolution might lack sufficient detail for accurate feature extraction, potentially leading to poor classification. Conversely, very high-resolution images can impose an unnecessary computational burden without significantly improving accuracy. Finding the right balance between resolution and information content is crucial for optimal results.
When working with custom datasets, implementing k-fold cross-validation for model evaluation provides a more reliable assessment. This technique involves dividing the dataset into multiple folds, using each fold in turn as a validation set while training the model on the remaining data. The resulting average performance across all folds offers a more robust measure of a model's generalization capabilities, minimizing bias that can arise from a single train/validation split.
Another consideration is the impact of training duration on performance. Inadequate training can lead to underfitting, where the model hasn't learned enough from the data to generalize effectively. Conversely, even with techniques like dropout, excessive training can still result in overfitting to specific characteristics within the custom dataset. Careful monitoring of validation loss throughout the training process is crucial for identifying the optimal balance between learning and generalization.
Preprocessing steps extend beyond simple normalization. Techniques like histogram equalization or contrast stretching can enhance specific image features, potentially improving accuracy for custom images. By carefully manipulating the images to emphasize key characteristics, we can improve the CNN's ability to distinguish relevant patterns within the data.
Lastly, adapting the learning rate dynamically can greatly benefit CNN performance with custom image data. Using techniques like learning rate schedules or incorporating adaptive optimizers, like Adam, enables us to adjust the learning process throughout training. This can facilitate smoother convergence and improved performance when the model is presented with a variety of image inputs. By tailoring the training process to the specifics of our custom dataset, we increase the likelihood of achieving optimal performance.
In conclusion, evaluating CNN performance with custom images necessitates a careful consideration of factors beyond simply measuring accuracy. The insights gained through techniques like transfer learning, data augmentation, and detailed performance analysis are crucial for building robust models that perform well in the intended real-world applications. Each project presents unique challenges, emphasizing the importance of experimentation and careful evaluation for optimal results.
More Posts from whatsinmy.video: