Using Built-in Functions in Python Video Processing Common Errors and Solutions
Using Built-in Functions in Python Video Processing Common Errors and Solutions - Frame Rate Error Prevention In OpenCV 9 Video Processing
Frame rate, or frames per second (FPS), is crucial in OpenCV's video processing, impacting how smoothly motion appears. Achieving consistent FPS can be a hurdle, often due to buffering problems and the need to retrieve and handle frames effectively. OpenCV's `cv2.VideoCapture` function is vital for accessing video data from different sources. It lets you read, process, and save video files. To improve processing speeds, multithreading is a helpful tool. It lets you dedicate one part of the program to grabbing frames while others deal with the processing and display. You can control the FPS by adding pauses in the frame capture loop using `cv2.waitKey()`, but this needs proper setup of capture properties. To make sure the FPS is what you want, ensure the `cv2.CV_CAP_PROP_FPS` property is correctly configured. Troubleshooting FPS problems often involves checking that video frames are available and the processing part of your code is running smoothly. There are various OpenCV functions for tasks like frame transformations, object detection, and editing which help with video processing, but optimizing processing loops and efficiently managing frame rate remain key.
Frame rate inconsistencies in OpenCV can lead to noticeable visual issues like jerky or uneven playback, ultimately impacting the perceived quality of the video processing output. The maximum achievable frame rate within OpenCV is constrained by both the system's hardware and the video's compression format, highlighting that minor parameter adjustments can have a significant influence on processing speed. While OpenCV offers `cv2.VideoCapture.get(cv2.CAP_PROP_FPS)` for retrieving the frame rate, relying solely on this function can be problematic when dealing with videos that have inconsistent frame timings.
A common pitfall is the assumption that the expected frame rate in OpenCV directly aligns with the actual output frame rate, which might not be the case. This mismatch can easily lead to timing discrepancies within processing loops. When handling multiple video streams or implementing filters in real-time scenarios, developers need to utilize `cv2.waitKey()` strategically to regulate the delay between frames. This is crucial for maintaining the desired frame rate throughout the process.
OpenCV includes tools for frame dropping or skipping, but improper use can cause missed frames and reduce overall video integrity, often going unnoticed until playback. Frame rate errors pose a particular challenge in applications like object detection and motion tracking, where accurate timing is paramount for maintaining tracking and detection performance. The concept of managing both constant and variable frame rates in OpenCV is interesting. Handling variable frame rates often necessitates custom logic to maintain smooth video playback.
Debugging frame rate errors can be complex because the source of the issue might not always be within OpenCV itself. Upstream stages like decoding or network latency in streaming applications can introduce errors that ultimately manifest as frame rate problems. It's crucial to evaluate the performance of your video processing in different environments. Factors like lighting variations and scene complexity can significantly influence the perceived frame rate and how effectively OpenCV's real-time processing functions perform. Understanding these influences is vital for optimizing performance and delivering a high-quality viewing experience.
Using Built-in Functions in Python Video Processing Common Errors and Solutions - Memory Management Solutions For Large Video Files
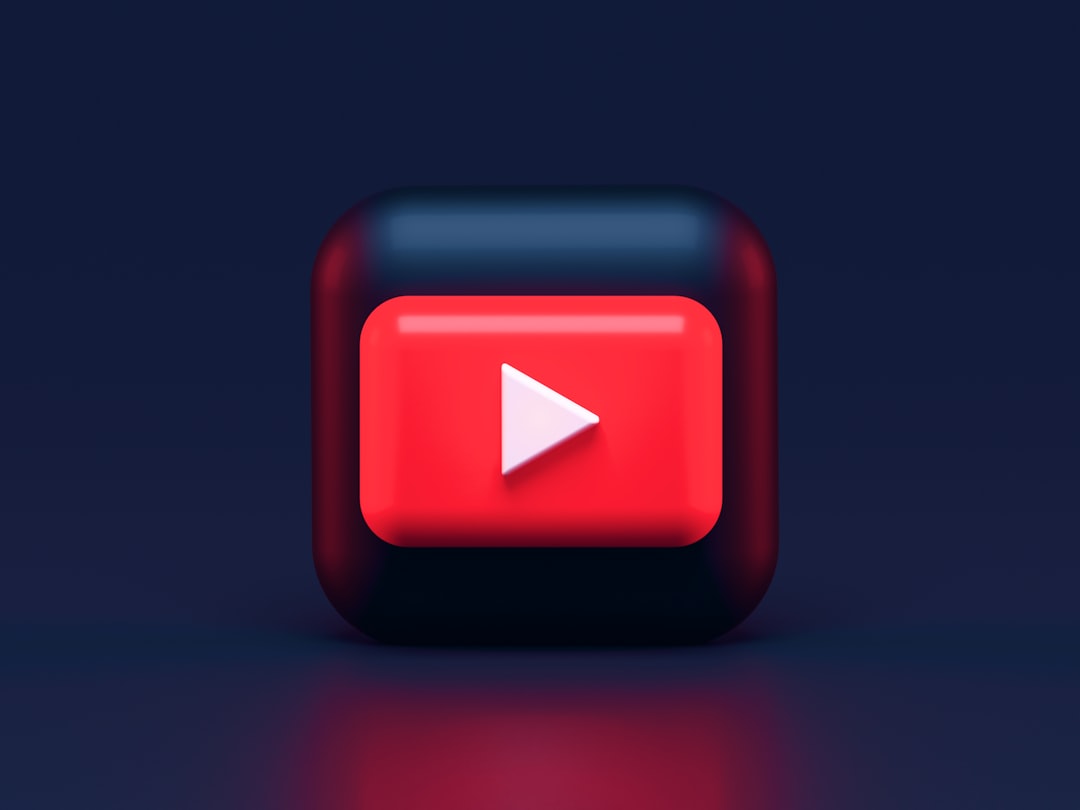
When dealing with large video files in Python, memory management becomes a crucial aspect for preventing errors and maximizing performance. Failing to manage memory effectively can cause your program to run out of memory or become sluggish. Memory mapping, through the `mmap` module, offers a way to work with files that are too big to fit entirely into RAM. This allows you to process parts of the file without loading the whole thing into memory at once, which saves memory. Another technique to reduce memory usage is leveraging Python generators. Instead of loading a massive video into memory all at once, generators process it frame by frame, saving a lot of space. Tools like `Numpy` can be useful for working with these techniques. They often abstract some of the technical details, making memory mapping easier to implement for large data sets. For anyone processing large video files in Python, a good grasp of these memory management techniques is a must if you want to ensure that your programs remain efficient and avoid crashing due to memory overload.
When dealing with extensive video files, often reaching sizes of tens of gigabytes, especially in high-definition or raw formats, efficient memory management is paramount. This becomes crucial due to the substantial system resources these files can consume during processing.
One common approach involves strategic memory buffering. Techniques like using circular buffers can be helpful, as they limit the number of frames held in memory simultaneously. This reduces overall memory usage while providing smooth video playback. For real-time video processing, it's often more efficient to rely on data streaming rather than loading whole files into memory. This approach allows processing of large videos without straining the system's RAM.
The choice of video compression format can significantly impact file size and processing speed. While lossless compression delivers high-quality output, resulting files can be several times larger than those compressed with lossy methods. This necessitates different memory management approaches for different compression formats.
Memory-mapped files offer an interesting solution for interacting with large video files stored on disk. This method treats the file as if it were in memory, enabling quick access while minimizing actual RAM usage. However, it is important to consider how much of an impact this may have on processing time due to how it interacts with the disk.
Another potential optimization lies in how threading is used. Traditionally threading has been useful in video processing for frame handling, but it can also be helpful to incorporate it into memory management. Each thread can handle various tasks, like reading data from the source file, processing frames, and storing the output.
Python's automatic garbage collection can be a double-edged sword. In long-running video processing tasks with a lot of memory usage, it can lead to unexpected results. Careful monitoring and possibly some fine-tuning may be necessary to prevent memory leaks and make sure that objects no longer needed are released efficiently.
The resolution of a video plays a large part in the memory requirements. Naturally, higher resolutions demand more processing power and RAM, emphasizing that the chosen resolution is a significant aspect of managing memory in video processing.
Leveraging profiling tools can provide engineers with a deep understanding of how their code interacts with memory. This information helps uncover bottlenecks and other performance issues and enables engineers to fine-tune their methods for better efficiency. This information then can lead to finding ways to manage memory use in the context of specific video processing workflows.
There's a consistent theme in programming which is that there's often trade-offs, and memory optimization in video processing is no exception. While reducing memory use is often desirable, some techniques can cause a decrease in processing speeds. Recognizing these trade-offs and balancing them with project goals is an important skill in computer science.
It's important to note that these are merely some of the potential techniques in use; many other approaches may be needed depending on the specifics of the situation.
Using Built-in Functions in Python Video Processing Common Errors and Solutions - Troubleshooting Codec Support In Python FFmpeg Integration
When you're using Python to work with video using FFmpeg, you might encounter issues related to codec support. This can happen if the FFmpeg build you're using wasn't configured to include the necessary codec, for example, if you're working with AV1 but your build lacks the `libdav1d` library. You can figure out which codecs your FFmpeg version supports using the command `ffmpeg codecs`, checking for entries like `DEVL av1` that indicate support. It's also crucial to implement proper error handling in your code to prevent unexpected crashes, especially considering that outdated versions of FFmpeg are often the source of problems. To make things simpler, consider using higher-level Python libraries like `pydub` or `moviepy` for basic video processing tasks, as they can streamline FFmpeg integration and minimize the need for complex command-line operations. While FFmpeg provides powerful capabilities, making sure your build includes the appropriate codecs and your code is error-tolerant is crucial for a smooth workflow.
When integrating FFmpeg within Python for video processing, codec support can be a source of frustration. It's not always straightforward, and often depends on the specifics of your FFmpeg build and the system's architecture. A codec that works on one system may fail on another, simply because the necessary libraries or build configurations aren't present.
FFmpeg itself comes packed with a vast collection of decoders and encoders, but it doesn't include them all. Some are excluded due to licensing hurdles, like certain proprietary codecs like H.264 or AAC. This can lead to issues if your Python code tries to use a codec that hasn't been compiled into your FFmpeg version. It's worth noting that this isn't always transparent, and you might get errors that are a bit cryptic.
Beyond the codec itself, the container format also has a role to play. Just because a codec can theoretically handle encoding a video doesn't mean that the chosen container (like AVI, MP4, or MKV) is well-suited to it. Trying to pack a modern codec into an older container format can cause odd behaviors, leading to playback problems.
Adding to the complexity, transcoding or doing real-time video processing with FFmpeg can lead to latency. This latency can be exacerbated by poorly-configured codecs or by incompatible codec settings. This is particularly problematic for applications that depend on speed and precise timing, like live streaming.
There's also the fact that conversions between codecs can result in a decrease in quality. Going from a lossless codec to a lossy codec can visibly damage a video, a major concern for applications that are sensitive to visual fidelity. Carefully considering your codec choices during these transitions is critical.
A lot of troubleshooting with FFmpeg boils down to understanding the abundance of command-line parameters it has. A minor typo in a flag or a misunderstanding of how a parameter functions can easily lead to a cascade of issues related to codec communication. It's quite easy to get lost, even for experienced engineers.
Codec issues can also be exacerbated by corrupted input files. While FFmpeg offers flags to try and recover or ignore errors in corrupted files, relying on these flags can lead to less predictable results.
Another trade-off in using FFmpeg involves speed and compression. High-compression codecs can dramatically reduce file size but at the cost of a potential increase in processing time. This is something you have to consider when writing Python scripts, as it can impact your application's performance.
FFmpeg is a project that undergoes frequent updates, and each release potentially adjusts the supported codecs. This means that an integration that worked fine with an older FFmpeg version could suddenly break after an update. This is a great example of how vital version control is for maintaining stability.
Lastly, if you’re looking for extreme flexibility, FFmpeg lets you create custom codecs. While technically impressive, this adds a new layer of complexity that can make troubleshooting that much more involved when integrating it with Python.
It’s easy to see why codec support can be challenging when working with FFmpeg and Python. It underscores the intricate interaction between codecs, containers, command-line settings, system dependencies, and FFmpeg versions, which can easily lead to unexpected results if not carefully managed.
Using Built-in Functions in Python Video Processing Common Errors and Solutions - Video Frame Extraction Bug Fixes Using Built In Functions
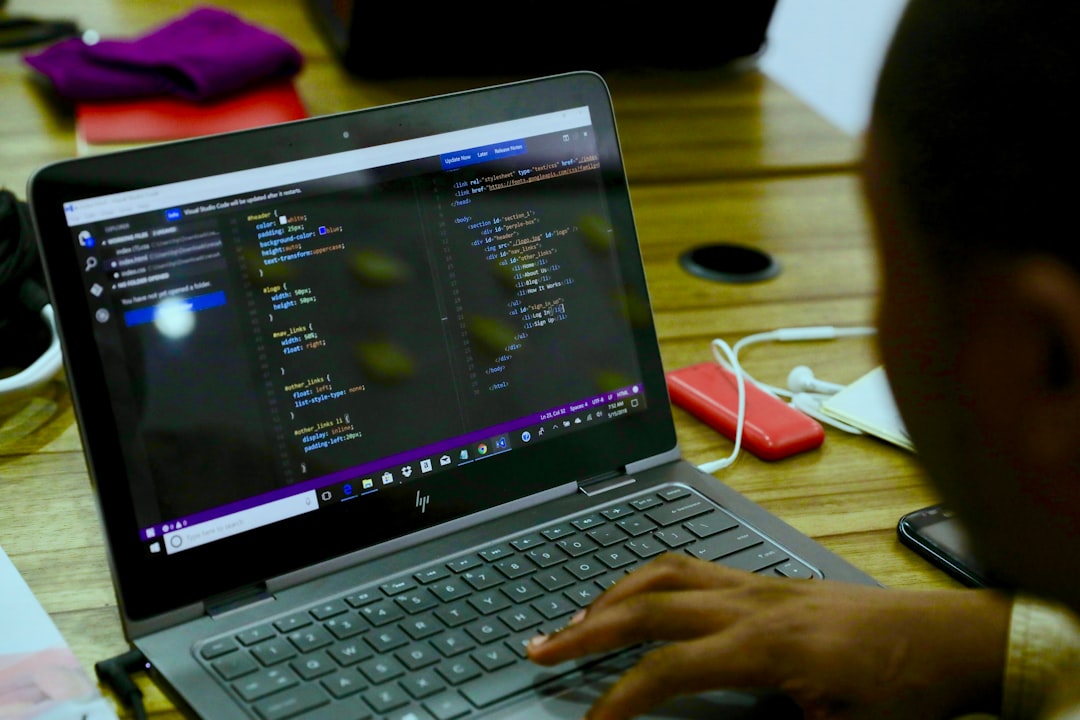
When working with Python for video processing, specifically frame extraction, various bug fixes related to built-in functions have been implemented. These improvements contribute to more reliable and stable results. For instance, the Katna library, a popular tool for video processing, has resolved a multiprocessing error that affected Python versions 3.6 and 3.7. This bug, which could have caused issues with frame extraction, has been fixed in Katna version 0.9 and newer. Another noteworthy change is the resolution of a memory leak in older Katna versions (0.8.2 and below) that arose when processing large amounts of video keyframes. While these fixes are beneficial, users should also be mindful of the fact that certain function names within Katna were altered starting with version 0.5.0. This may mean updating existing code that relies on these functions. When utilizing OpenCV's VideoCapture for frame extraction, it's important to note that the function requires an existing output folder for storing the extracted frames. If the folder doesn't exist, the function will often fail without producing an easily understandable error, leading to unexpected behavior. These bug fixes and modifications reflect a general trend towards improvement in video processing tools and libraries. However, developers should always be cautious of potential issues that can arise from version updates, especially if they're dealing with older codebases. Careful attention to change logs and thorough testing is recommended for seamless transitions.
When working with video frame extraction in Python using built-in functions, a few quirks and potential pitfalls can crop up. One aspect that can cause trouble is the way video codecs often compress frames by relying on information from previous frames. This means if you extract a single frame without properly managing these dependencies, you might not get the intended result, possibly getting an incomplete or corrupted frame. Additionally, video formats use different color spaces, with RGB and YUV being common examples. When extracting frames, OpenCV will typically switch between these color spaces, and this can cause strange color changes if you're not prepared for it in the rest of your processing stages.
Timing can also play a role. The `cv2.VideoCapture` function, which is great for reading video data, isn't always perfectly synced with the actual frame timestamps in the video itself. As a consequence, this lack of perfect synchronization can lead to situations where you skip frames, or you end up extracting corrupted frames that are only partially loaded. It's a good reminder that working with videos involves understanding the different formats and the way each format handles frames. For example, certain formats like AVI or MP4 might have their own quirks when dealing with specific video codecs, leading to extraction errors if a format doesn't support a codec used in a given video.
The quality of the video matters a lot, too. Videos with higher resolutions and bitrates require more computing power and memory. In situations where your system doesn't have ample resources, this can lead to performance slowdowns or the extraction process just failing. You can try to speed things up by using the GPU with tools like CUDA, but setting that up can be quite complex. Even when the extraction process goes as planned, sometimes metadata is lost. Metadata holds useful details, like timestamps or the type of compression used, and if you lose it, it can cause issues for analysis or processing later on.
Multi-threading, a common technique for speeding things up, can create a whole new set of challenges when handling frames. Trying to get several threads to work together can sometimes lead to race conditions which cause frame data corruption, if not properly managed. And just like with other aspects of video processing, errors can sometimes hide until runtime. These errors might be caused by problems in the way a video was created or transferred. In those cases, carefully inspecting the source files before extraction becomes a crucial step in avoiding extraction issues. In live video scenarios, like video conferencing, where frame timing is essential, delays in extraction can be problematic. It can cause audio/video desync, causing a bad user experience.
It's worth considering the complexities involved in built-in function frame extraction in the context of larger video workflows. It's an area where there are various complexities and factors to understand for a successful video processing workflow.
Using Built-in Functions in Python Video Processing Common Errors and Solutions - RGB To BGR Color Space Conversion Error Resolution
OpenCV's reliance on the BGR color space, a historical artifact from its early development, often creates friction in Python video processing. When integrating with other libraries or programs that usually assume RGB, this difference can lead to errors during color space conversions. A common issue is forgetting to include the correct conversion flag within the `cv2.cvtColor()` function. This simple oversight can lead to skewed color output, disrupting your intended visuals. Furthermore, it's important to understand how specific color space conversions affect your application's goal, especially if you are performing tasks such as color segmentation or object detection where accurate color representation is crucial. These steps ensure that color processing is accurate and seamless, whether working with individual frames or entire video sequences. By managing color space conversions thoughtfully, you can improve the final output quality and integrate your processing smoothly with other Python libraries and tools.
### RGB To BGR Color Space Conversion Error Resolution
1. **A Frequent Source of Trouble:** In video processing, switching between RGB (Red, Green, Blue) and BGR (Blue, Green, Red) color spaces can cause visual inconsistencies, particularly in OpenCV because it uses BGR by default. This swap can lead to unexpected color displays in things like image labeling or visual analysis.
2. **A Bit of History:** RGB to BGR conversion is especially important when dealing with older image processing setups, which often used BGR as the default. As a result, engineers frequently encounter color-shifted images, and explicit conversion becomes essential when applying newer methods or libraries.
3. **Performance Matters:** Mismatches between color formats can slow down processing. Making sure color space conversion is done correctly can greatly improve performance by reducing needless calculations and memory usage caused by working with incompatible formats.
4. **Pixel Format Awareness is Key:** Understanding pixel formats is crucial. RGB images typically have 3 channels, but conversions to formats like RGBA (adding an alpha channel) need careful handling to prevent data loss. If an alpha channel exists, the conversion might need extra code to manage it correctly.
5. **The Math Behind It All:** The process of changing between RGB and BGR isn't just about swapping values. In many systems, especially those working with color spaces like YUV or HSV, the mathematical representation can get complicated. If the conversion logic doesn't properly interpret these formats, it can lead to visible image flaws.
6. **Compression's Effect:** Compression methods can significantly impact how color values are represented, particularly at lower bitrates. If an image is poorly compressed, direct RGB to BGR conversion might not produce the right results. This shows that preprocessing is vital.
7. **Sensitivity in Real-Time Systems:** In real-time video processing applications, maintaining a consistent frame rate can be easily disrupted by improper color space conversions. This emphasizes that a thorough approach to matching formats across all processing steps is required.
8. **Workflow Bottlenecks:** Color space conversion bugs often arise from the assumptions made during processing stages. Developers might miss cross-format compatibility errors, causing difficult-to-track inefficiencies later on.
9. **Library Capabilities:** Not all Python libraries smoothly convert between RGB and BGR. Some require explicit handling through custom functions, which can introduce extra opportunities for errors in code, often overlooked during development.
10. **Debugging Headaches:** Troubleshooting color conversion issues can be difficult since the outcomes aren't always immediately obvious. Engineers might spend time tracking down problems that, at first glance, appear unrelated to color handling, making the debugging process complex.
Using Built-in Functions in Python Video Processing Common Errors and Solutions - Video Stream Threading And Buffer Overflow Prevention
**Video Stream Threading and Buffer Overflow Prevention**
When dealing with video streams, especially in Python, threading is a valuable tool for improving performance. By creating separate threads for tasks like capturing frames from a video source and processing those frames, you can significantly decrease the overall lag and delays that often occur during video processing. However, this approach can be tricky if you don't handle the frame buffers effectively. If the buffer isn't managed correctly, your processing might end up using outdated frame data which would naturally cause errors.
To avoid this type of problem, it is beneficial to implement strategies that ensure the buffer is efficiently managed. One way to do this is to control the speed at which frames are read (i.e. frames per second, or FPS) which helps flush old frames out of the buffer. Using a data structure called a queue alongside multithreading is another valuable technique, enabling your code to process frames as they are captured, minimizing the chance of buffer overflow.
Finally, it's important to incorporate proper error handling into your application's design. Video stream processing applications can face unexpected disruptions like cameras becoming disconnected. Without proper error handling, a disconnected camera or other external factors can easily crash your program. To maintain a stable and robust program, always expect potential problems and write code that catches and gracefully recovers from unexpected errors that might arise during the video processing workflow.
### Surprising Facts About Video Stream Threading and Buffer Overflow Prevention
1. **Buffer Overflow Vulnerability**: It's interesting that even in languages like Python, which are often considered higher-level, buffer overflows can be a problem when working with libraries built using C or C++. This reminds us that even when using advanced programming tools, we have to pay attention to memory boundaries. This is especially critical when dealing with a continuous flow of video frames, where a failure could potentially lead to an exploit.
2. **Real-Time Processing Challenges**: When we're dealing with video in real-time, one of the biggest goals is to keep latency as low as possible. However, this pursuit of low latency can also be a source of issues when we're not careful about how threads are managed. Some researchers have discovered that when thread management isn't done correctly, the frame processing time can become a lot slower when the system is under pressure, resulting in a less smooth viewing experience.
3. **Thread Safety in OpenCV**: OpenCV is a fantastic tool for working with video, but it's not always perfectly designed for multithreaded scenarios. In particular, some parts of OpenCV aren't fully thread-safe. If you have multiple threads trying to change the same variables at the same time, you could end up in a race condition which can corrupt data. This tells us that when you're using OpenCV and threads together, you have to be mindful of how to protect shared resources.
4. **Dynamic Buffer Adjustments**: There's an interesting trend where modern video processing systems are able to automatically adjust the buffer size depending on the network conditions. This means algorithms can adapt in real-time to try and keep the streaming quality as high as possible. It highlights how complex the task of balancing the use of memory and the streaming performance can become.
5. **Thread Pool Usage**: One way to improve the performance of video processing is to use a thread pool. Instead of constantly creating and destroying threads, a thread pool lets you reuse a set number of threads for various tasks. This can result in significant improvements, particularly when dealing with something like extracting individual frames.
6. **Memory Overhead**: While threading can help speed up video processing, we also need to recognize that it comes at a cost in terms of memory. The work needed to manage all those threads can sometimes be too large for the potential benefits we get from parallelism. If you create too many threads, they can end up fighting for CPU time, which can lead to delays because of context switching. This suggests a good approach is to carefully consider how many threads to use.
7. **Latency and Buffering Interaction**: Buffering strategies and threading can sometimes affect latency in unexpected ways. For example, if a system is designed to aggressively fetch future frames, it can potentially cause the buffers to get overloaded. This can lead to processing delays which can be particularly troublesome when working with time-sensitive applications such as live streaming.
8. **Non-linear Frame Fetching**: A technique called non-linear frame fetching can be used to improve efficiency, but it creates complications for buffer management. When we're fetching frames out of order to process them more quickly, it means the buffer needs to be managed in a sophisticated way so that we don't get overflow issues. This makes us understand that achieving this kind of optimization demands a solid understanding of threading behavior and data flow.
9. **Error Propagation in Threads**: If we're not careful about how threads share resources, errors in one thread can "infect" other threads. This suggests that robust error handling is a necessity in threaded applications that handle video data, as video processing requires a continuous stream of operations.
10. **Processing Priority**: Sometimes, giving different priorities to video processing threads can have a major impact on performance. Some operations might have a tighter real-time requirement than others. For example, displaying a video frame promptly is often critical. This allows for smooth playback and creates a good experience for the user when carefully handled.
More Posts from whatsinmy.video: