Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing - Converting RGB Frame Data to Binary Using Python Native Methods
Converting RGB frame data to its binary representation in Python relies on a few core methods. A common route involves using OpenCV's `cv2.threshold` function. This function, however, first requires converting the RGB frame to grayscale using `cv2.cvtColor`. The thresholding process simplifies the image into a binary representation by assigning pixel values to either 0 or 255, depending on a predetermined cutoff value. While OpenCV provides a robust approach, alternatives exist. For simpler binarization tasks, NumPy can be used to directly manipulate the RGB data as arrays, effectively bypassing OpenCV's functionality. This flexibility within Python's ecosystem makes frame data manipulation accessible for various video processing and analysis goals.
1. RGB frame data, at its core, is a collection of three-value sets, each representing the red, green, and blue intensity of a single pixel. With each intensity ranging from 0 to 255, a single pixel can express a vast spectrum of over 16 million colors. However, this rich color representation comes at a cost in terms of data volume when converted to binary.
2. When translating RGB data to binary, each color intensity component is typically stored as an 8-bit value. Therefore, an entire RGB pixel requires 24 bits to store its color information, leading to substantial data sizes when working with video frames composed of thousands or millions of pixels. This volume presents challenges in terms of storage and processing, especially for higher resolution or long duration recordings.
3. Python's built-in `format()` function offers a convenient approach for directly converting integer RGB values into binary strings. By employing this function, developers can bypass the need for more complex, custom-built routines, leading to a cleaner and more concise codebase. This simplification aids in readability and maintainability.
4. For enhanced efficiency in RGB-to-binary conversion, utilizing NumPy arrays becomes advantageous. The inherent capabilities of NumPy to work with arrays in a vectorized manner allows for significantly faster manipulation of large image datasets compared to conventional Python lists. This performance boost is crucial for time-sensitive applications like real-time video processing or analysis.
5. By using bit manipulation methods, it becomes possible to work directly with RGB values in their binary form without needing to convert to a higher-level representation. This approach is especially useful for tasks like applying masks or isolating specific color channels, potentially speeding up the process. However, careful attention to the bit patterns and their intended manipulation is crucial to avoid unintended consequences.
6. When using Python's native `bin()` function for binary conversion, developers should be aware of the prefix "0b" that it appends to the result. While convenient, this prefix often needs to be removed in scenarios where the output should be solely the raw binary representation, potentially adding a step in the process if a clean representation is desired.
7. One of the hurdles when converting RGB data to binary is handling endianness. Failing to acknowledge endianness – the byte order convention – can lead to incorrect interpretation of the binary data when working across systems with differing architectures. This can manifest as discrepancies in the representation of pixel data. It's thus imperative that developers are cognizant of the byte order used for proper processing of the data.
8. The methods chosen for binary conversion significantly impact the processing speed, particularly in applications related to video processing. Python's efficient built-in tools can bring about substantial improvements in processing speed when applied skillfully, potentially leading to a 2-3 fold increase in performance when compared to less optimized approaches. The choice of approach directly influences how efficiently we can work with the video data.
9. Maintaining color accuracy throughout the binary conversion process is paramount. Any loss of precision in the RGB representation during the conversion can translate into noticeable differences in the visual quality of the processed image or video. The implications of bit-depth reduction, especially when combined with further compression techniques, can degrade the output noticeably. Choosing a suitable bit depth during conversion becomes important to preserve the original appearance of the data.
10. While binary representations inherently take up less space at the level of the data itself, it's important to acknowledge that video file sizes can increase drastically due to other aspects of storage and compression. Container formats and codec implementations can introduce overhead as they add metadata, which can lead to a substantial overall increase in the size of the video file, counteracting the inherent compactness of the binary data itself. The details of how we store and manage these bits can impact our data size unexpectedly.
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing - Frame Processing Memory Optimization Through Binary Operations
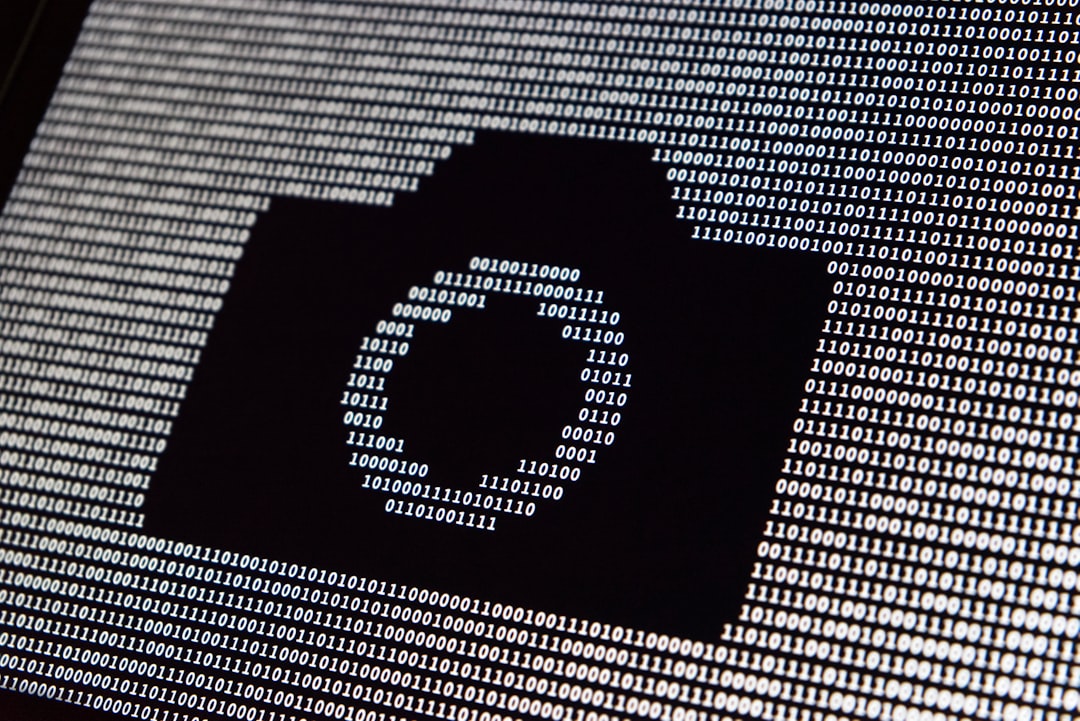
As video data resolution increases, the need for memory optimization in frame processing becomes crucial. Methods like selective frame processing, often using binary classifiers, can reduce the amount of data we need to handle. These classifiers identify important frames, for example, by comparing them to a reference frame and using a simple binary true/false output to signal a change. Leveraging optimized algorithms can also improve how we manage the memory used for storing video data while processing. This is especially useful for platforms like Field-Programmable Gate Arrays (FPGAs) which are designed for processing continuous streams of data. Additionally, using binary techniques like image thresholding can help clean up image data and make it easier to find specific features or areas within a frame. This is important as we increasingly rely on real-time processing for things like video surveillance or autonomous vehicle navigation. Understanding and efficiently employing binary operations in these scenarios is becoming essential to ensure we can manage the growing amounts of data produced by increasingly complex video capture systems and improve the performance of these systems.
1. **Leveraging Bitwise Operations for Color Manipulation**: By applying bitwise operations directly to RGB values, we can efficiently isolate individual color channels or perform blending tasks, leading to faster processing compared to using standard arithmetic. This is an interesting avenue to explore for optimization.
2. **Navigating the Trade-offs of Bit Depth**: The choice of bit depth during conversion impacts both the visual quality of the data and the resulting data size. For example, switching from an 8-bit to a 16-bit structure increases color fidelity but doubles the data size. Understanding these trade-offs is important when designing a frame processing pipeline.
3. **Managing Memory Dynamics in Python**: Python's dynamic memory allocation can hinder performance when working with large frame datasets and binary operations. Using NumPy's capabilities for upfront memory allocation can help prevent repeated memory reallocations, potentially smoothing out processing bottlenecks. This suggests that Python's flexibility can introduce some challenges we need to be aware of when it comes to performance.
4. **Caching to Reduce Redundancy**: Caching previously computed binary values in frame processing can be a powerful optimization. This is especially relevant when working with sequences of frames where pixel data may not vary substantially. Implementing a caching mechanism can potentially significantly reduce the computational workload. It's curious how much speed improvement one could gain here.
5. **Addressing Latency in Real-Time Scenarios**: Inefficient binary conversion can introduce latency in real-time video applications. Methods like parallel processing using libraries like NumPy or GPU acceleration could provide considerable gains in speed, making the difference between a real-time system and something that can't keep up.
6. **Focused Processing with Color Channel Masking**: The ability to apply masks in binary representations allows us to concentrate processing efforts on specific colors or regions within a frame. This focused approach reduces the workload, allowing for optimizations by avoiding unnecessary computations on the whole pixel set. This is another interesting area to investigate for efficiency.
7. **Compression's Influence on Binary Operations**: The compression method used prior to conversion can impact the efficacy of our subsequent binary processing. Lossy compression methods, while reducing data size, might discard important color information, leading to complications when converting to binary. The type of compression matters quite a bit here.
8. **Ensuring Cross-Platform Interoperability**: When transferring binary data between systems, we need to ensure compatibility by addressing differences in endianness. Failure to do so can result in corrupted data and frames that can't be processed correctly on receiving systems. This highlights a challenge with data exchange across platforms.
9. **Optimizing Data Structures for Efficiency**: Utilizing optimized data structures like bit arrays can considerably reduce memory usage when dealing with large volumes of binary data. This can be very beneficial for processing high-resolution video where memory is a scarce resource. There is likely further study one could do here regarding alternative structures.
10. **Leveraging External Libraries for Speed**: Libraries like OpenCV and imageio often provide pre-optimized functions for binary operations that can significantly improve frame processing speed. Using these can also accelerate development time, as compared to writing these functions from scratch. There are definitely advantages to utilizing existing resources in this domain.
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing - Implementing Bitwise Operations for Video Frame Compression
Implementing bitwise operations is a key technique in video frame compression, tackling the immense data volumes that arise from video capture. Bitwise operations like AND, OR, NOT, and shifts allow for efficient manipulation of pixel data at a fundamental level, working directly with the binary representation. These operations are critical for minimizing redundancy and optimizing how video data is stored, particularly when dealing with high-resolution video. While traditional compression methods have proven useful, the emergence of neural networks integrated into existing compression frameworks signifies a shift toward more sophisticated approaches. These advanced techniques are designed to streamline the compression process without negatively impacting video quality. For anyone looking to dig deeper into Python-based video frame processing, grasping bitwise operations is a crucial aspect of the knowledge base. It's important to understand that there can be a degree of trade-off, sometimes sacrificing small amounts of detail for increased efficiency and storage space. Understanding the interplay between these choices and their consequences is fundamental for any developer in the field.
1. **Bitwise Operations for Color Tweaking**: We can use bitwise operations to directly modify individual color components (red, green, and blue) within a frame. This approach allows us to isolate and blend colors more efficiently than traditional arithmetic methods, potentially leading to noticeable speed increases when processing frames.
2. **Balancing Color Accuracy and Data Size**: When converting to binary, the bit depth we select impacts both the visual quality and the size of the resulting data. Going from 8-bit to 16-bit significantly boosts the range of colors, but also doubles the size of the data. It's a tricky balance between image quality and the resources required to manage the information.
3. **Python's Memory Management Quirks**: While Python is flexible, its dynamic memory handling can slow down things when we work with massive video frame datasets and bitwise operations. Using NumPy's ability to pre-allocate memory can help us avoid repeated memory allocations, potentially smoothing out any processing slowdowns that can occur. This shows that convenience can come with a performance price if not handled carefully.
4. **Caching for Frame Repetition**: If we see a lot of similar frames, we could save processing power by implementing a caching system to store the results of our binary operations. This way, when we process a similar frame later, we won't have to redo the same calculations. It is intriguing to think about how much we can improve things with this strategy.
5. **Real-Time Performance Under Pressure**: When speed is critical, like in a live video stream, slow binary conversion can create delays. To overcome this, we can leverage parallel processing using tools like NumPy or GPU acceleration. These methods are vital for pushing our video processing from adequate to truly real-time.
6. **Targeted Frame Processing**: By applying binary masks to select color channels, we can refine the focus of our processing effort. This targeted approach allows us to skip calculations on areas of a frame that aren't relevant. It is curious how much of a performance gain we can achieve using this focused approach.
7. **Compression's Effect on Binary Conversion**: The compression method used on video data beforehand can have a big effect on how well our binary operations work. While lossy compression shrinks the file size, it might throw away important color details that could complicate converting the data to binary afterwards. It's essential to understand how compression interacts with our operations.
8. **Endianness Considerations for Sharing Data**: When exchanging binary video data between computers, we have to be careful about endianness—the way bytes are ordered. If we don't handle this correctly, it can lead to garbled data and frames that won't process properly. This is important to keep in mind for any projects that involve multiple systems.
9. **Memory Efficiency with Bit Arrays**: To work with massive amounts of binary data in high-resolution video processing, we can use optimized structures like bit arrays. This technique can reduce memory usage quite a bit. It's a potential area for deeper research to see what alternative structures exist.
10. **Leveraging Existing Libraries for Faster Results**: Libraries like OpenCV and imageio provide optimized functions for binary operations. Utilizing these can greatly speed up the processing of video frames and also make development quicker. It's clearly beneficial to take advantage of the work already done in this field.
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing - Binary String Manipulation Techniques in Video Stream Processing
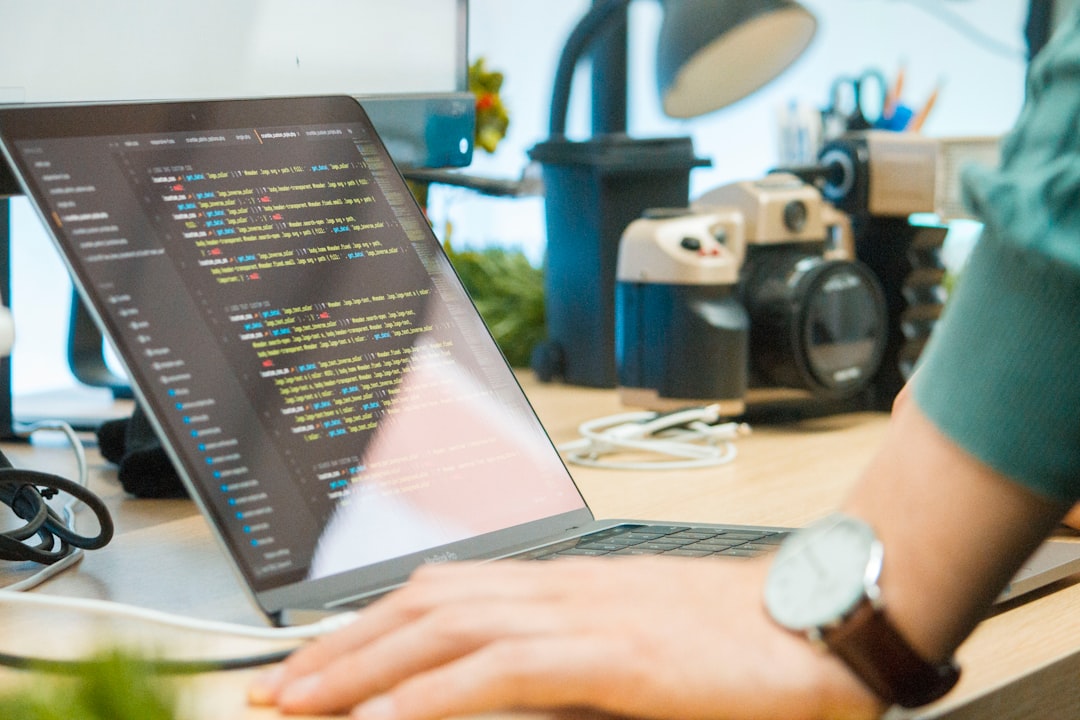
Binary string manipulation is a fundamental aspect of video stream processing, enabling the efficient encoding and decoding of video data. This involves transforming pixel values into their binary representations, a crucial step in managing the immense data volume inherent in high-resolution videos. For instance, a 4K video frame can contain up to 8,294,400 bits of data, highlighting the need for robust techniques to handle such large binary datasets. Python's ecosystem offers a convenient toolkit for manipulating binary strings, incorporating built-in functions like `bin()` and `hex()` as well as powerful libraries like NumPy, which provide tools for bitwise operations that can optimize performance in demanding tasks like real-time video processing. Nonetheless, it's vital to be mindful of potential pitfalls such as bit overflow, which can occur when operations exceed the limits of data types. Further, maintaining data integrity across different systems requires careful attention to issues like endianness, the ordering of bytes in a binary representation, to prevent errors during data exchange. Understanding and effectively utilizing binary string manipulation techniques is essential for building robust and performant video processing systems capable of handling the ever-increasing resolution and data complexity of modern video content.
Binary string manipulation is a powerful tool for handling video streams, particularly when it comes to processing and compressing the massive amounts of data involved. We can convert video data into and out of binary formats using specialized algorithms, essentially encoding and decoding video streams using binary strings. A standard 4K video frame, for instance, can be represented by a binary string of up to 8,294,400 bits, highlighting the large data scales involved.
One interesting aspect is translating values into binary representations, for example, changing a hexadecimal value like 0x27 into a binary string like '000'. This concept extends to color representations where a sequence of binary digits could represent 255-255-255, white. Python offers tools for working with this sort of binary data: functions like `bin()`, `hex()`, and `oct()` are useful for basic conversions, and `binascii.unhexlify()` helps when we need to work with byte sequences and bit-level operations.
It's important to be aware of bit overflow, which can occur when we use fixed-width integer data types in operations. If the results exceed the maximum limit of the data type, we can end up with unexpected values. This is something to watch out for during processing.
We can convert strings into a binary data stream using methods like the BinaryWriter's `WriteString` method. This operation modifies the original byte representation, essentially storing the string in a memory stream object.
Techniques for detecting manipulated video content typically examine pixel data, but rely on descriptors of the stream rather than looking at individual pixels directly.
Understanding how binary representations work is fundamental. We need this knowledge to make sense of how integers, characters, and even images are stored and processed as sequences of bits.
String manipulation techniques play a crucial role here too. These techniques, which include joining strings, extracting substrings, and comparing lengths, all have relevance to our goal of processing binary strings.
A binary string is essentially a sequence of characters representing binary values. These strings can be manipulated across various programming languages and data types, which gives us flexibility when building video processing applications.
While these techniques are essential, it's worth acknowledging some of the practical limitations we face when working with binary representations. For example, when converting RGB data to binary, lossy compression techniques used to shrink data can compromise the integrity of the information. This highlights the tension between storage efficiency and accuracy, a trade-off that's often unavoidable when compressing and decompressing information. In essence, there is no free lunch.
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing - Performance Analysis Between Int and Binary Video Data Structures
Analyzing the performance differences between using integer and binary data structures for video processing is crucial, especially when using Python. This analysis involves assessing both how much space the data takes up (space complexity) and how long it takes to process (time complexity). Because binary structures typically store information more compactly, they can often lead to faster access and manipulation of data, especially in cases where quality standards can be maintained. Importantly, making good use of binary structures can greatly improve performance for tasks that need fast responses, such as in applications that monitor video in real-time, like security systems or computer vision projects. Understanding these performance factors is vital for developers hoping to refine video processing using binary conversions, as they aim to optimize their processing capabilities.
1. **The Scale of Binary Data**: Converting video data from its RGB format to binary can dramatically increase the size of the data we're working with. For instance, a single 4K video frame, with its millions of pixels, could potentially generate a binary representation several megabytes in size. This shift in data volume highlights the importance of finding efficient ways to manage the data throughout the processing pipeline.
2. **Impact of Color Depth on Binary Conversion**: When we convert RGB data to binary, the color depth we choose—like 8-bit or 16-bit—affects both image quality and the amount of data we're dealing with. While a higher bit depth increases the range of colors and visual fidelity, it also substantially increases the size of the binary data, making it crucial to find a balance between quality and efficiency.
3. **Speed Benefits of Direct Bit Manipulation**: Operating directly on the bits of a binary representation, using bitwise operations, can lead to substantial performance gains compared to working with higher-level data structures and arithmetic. This fine-grained control over data becomes important when we're working on systems where speed is a critical requirement.
4. **Bit Overflow: A Potential Pitfall**: One thing to keep in mind when performing binary operations is the possibility of exceeding the limits of the data types we're using. This bit overflow can lead to unintended consequences, potentially resulting in corrupted pixel data or unexpected output. It’s something to be cautious about when manipulating binary data in our programs.
5. **Endianness: A Cross-Platform Issue**: When we transfer binary video data between different systems, we have to consider the byte order (endianness) used by each system. Failing to account for differences in byte order can result in garbled data. It's a subtle detail that can have a significant impact on data integrity when transferring video across platforms.
6. **Caching for Improved Performance**: One way to optimize binary operations is to use a caching mechanism. In situations where a large portion of the frames have similar pixel data, storing previously computed binary values can greatly reduce the number of times we need to repeat those calculations, potentially resulting in a noticeable speed increase.
7. **Python's Dynamic Memory & Performance**: While Python's flexibility is attractive, the dynamic memory allocation can lead to performance challenges when processing large amounts of video data, particularly when dealing with bit operations. To mitigate these issues, we can leverage libraries like NumPy, which enable pre-allocation of memory. This helps smooth out the memory management process and can improve the overall efficiency of our code.
8. **Compression's Influence on Binary Representations**: The compression method we use prior to converting a video to binary format can affect how we're able to process the resulting binary data. Lossy compression, for example, while reducing file sizes, might discard important details about the colors in the video. This potential loss of information can make subsequent processing steps more complex.
9. **Specialized Data Structures for Binary Data**: When working with large quantities of binary video data, using optimized data structures, like bit arrays, can significantly reduce memory usage. This is especially important in processing high-resolution videos where memory resources might be limited. These structures may offer a way to make more efficient use of the available memory.
10. **Variable-Length Encoding for Compact Representation**: There are some interesting encoding schemes that can create more compact binary representations of the data, using what’s known as variable-length encoding. These methods offer the potential to store data in smaller sizes without compromising data integrity. It could be a promising avenue for improving video storage solutions.
Understanding Binary Conversions in Video Data A Deep Dive into Python's Int-to-Binary Methods for Frame Processing - Practical Binary Video Frame Transformations With NumPy Arrays
This section, "Practical Binary Video Frame Transformations With NumPy Arrays," shifts our focus to the practical application of NumPy for efficient video frame manipulations within a binary context. NumPy's array capabilities are central to optimizing video processing, allowing us to work with video frames at the most granular level – the individual pixel. This direct access to pixel data enables us to explore advanced techniques such as bitwise operations and intelligent memory management that can significantly reduce the computational overhead associated with handling large video files.
By using NumPy arrays, developers can optimize frame processing and storage in several key ways. This includes techniques like manipulating pixels directly, using bitwise operators for efficient data modification, and proactively managing memory through pre-allocation strategies. These approaches prove particularly beneficial when dealing with high-resolution video, where even minor gains in efficiency can translate to large improvements in overall processing performance.
Ultimately, understanding and applying these binary transformations lays the groundwork for more advanced tasks in video analysis and processing. NumPy provides a powerful toolkit for implementing machine learning algorithms, real-time video monitoring, and other applications requiring efficient manipulation of vast quantities of video data. Understanding the nuances of how NumPy can optimize binary frame transformations is therefore a key aspect of developing efficient and scalable video processing pipelines.
1. **Boosting Compression with Binary Operations**: When we use binary operations to handle video frames, we can often see a big improvement in how efficiently we compress the data. This is clear in formats like H.264, which use clever binary-based techniques to get rid of redundant information, resulting in smaller file sizes without much loss in quality.
2. **Speeding Up Frame Processing with Direct Bit Tweaking**: Working directly with the individual bits of pixel data using bitwise operations can often deliver speed benefits that are easily overlooked. These low-level manipulations allow for fast color adjustments and filtering, providing a potential speed advantage over more traditional methods that rely on higher-level code.
3. **Variable-Length Encoding for Compact Binary**: Implementing variable-length encoding in our binary representations can optimize how we store video data. Unlike fixed-length encoding, variable-length encoding allows for more compact representations based on how often pixel values occur. This is especially useful for scenes that have repeating patterns or colors.
4. **The Visible Effects of Quantization**: The process of rounding off RGB values when converting to binary can cause artifacts, especially in detailed areas. Understanding how quantization works is important for preserving image quality while still effectively compressing data.
5. **Frame Rate's Influence on Data Size**: The frame rate we choose for our videos directly impacts how much data we need to process. A 30 FPS video, for instance, generates a lot of data when converted to binary, since each frame's RGB values need to be handled individually. This adds up in terms of storage and computational resources.
6. **Binary Trees: A Potential Performance Boost**: Organizing binary data in a binary tree structure can speed up how we encode and decode video. This kind of data structure makes it faster to search and find pixel values, which could be valuable when optimizing video pipelines.
7. **Smart Memory Management**: The way we manage memory during binary operations can make a big difference in performance. Using continuous blocks of memory to store binary data can help reduce cache misses, which in turn means faster access and lower latency in processing.
8. **Meeting the Demands of Real-Time Video**: The processing overhead of converting frames to binary can be a hurdle for real-time video applications. Offloading these intensive operations to GPUs can significantly speed things up, ensuring that applications like live streaming or video calls stay smooth.
9. **Lossless vs. Lossy Compression: A Trade-Off**: When picking methods for binary conversion, the kind of compression we use (lossless vs. lossy) will affect the end result. Lossless methods maintain all the original data but can lead to larger file sizes. We need to carefully weigh our options based on the specific needs of the video application.
10. **Error Correction for Robust Video Transmission**: When sending binary video data between systems, using error correction codes (ECC) is essential for data integrity. ECC helps protect against data loss or corruption, ensuring smooth playback and an accurate representation of the video.
Analyze any video with AI. Uncover insights, transcripts, and more in seconds. (Get started for free)
More Posts from whatsinmy.video: